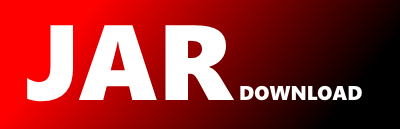
org.dmg.pmml.time_series.TimeAnchor Maven / Gradle / Ivy
package org.dmg.pmml.time_series;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import org.dmg.pmml.Visitor;
import org.dmg.pmml.VisitorAction;
import org.jpmml.schema.Added;
import org.jpmml.schema.Version;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://www.dmg.org/PMML-4_3}TimeCycle" maxOccurs="unbounded" minOccurs="0"/>
* <element ref="{http://www.dmg.org/PMML-4_3}TimeException" maxOccurs="2" minOccurs="0"/>
* </sequence>
* <attribute name="type">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <enumeration value="dateTimeMillisecondsSince[0]"/>
* <enumeration value="dateTimeMillisecondsSince[1960]"/>
* <enumeration value="dateTimeMillisecondsSince[1970]"/>
* <enumeration value="dateTimeMillisecondsSince[1980]"/>
* <enumeration value="dateTimeSecondsSince[0]"/>
* <enumeration value="dateTimeSecondsSince[1960]"/>
* <enumeration value="dateTimeSecondsSince[1970]"/>
* <enumeration value="dateTimeSecondsSince[1980]"/>
* <enumeration value="dateDaysSince[0]"/>
* <enumeration value="dateDaysSince[1960]"/>
* <enumeration value="dateDaysSince[1970]"/>
* <enumeration value="dateDaysSince[1980]"/>
* <enumeration value="dateMonthsSince[0]"/>
* <enumeration value="dateMonthsSince[1960]"/>
* <enumeration value="dateMonthsSince[1970]"/>
* <enumeration value="dateMonthsSince[1980]"/>
* <enumeration value="dateYearsSince[0]"/>
* </restriction>
* </simpleType>
* </attribute>
* <attribute name="offset" type="{http://www.dmg.org/PMML-4_3}INT-NUMBER" />
* <attribute name="stepsize" type="{http://www.dmg.org/PMML-4_3}INT-NUMBER" />
* <attribute name="displayName" type="{http://www.w3.org/2001/XMLSchema}anySimpleType" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"timeCycles",
"timeExceptions"
})
@XmlRootElement(name = "TimeAnchor", namespace = "http://www.dmg.org/PMML-4_3")
@Added(Version.PMML_4_0)
public class TimeAnchor
extends org.dmg.pmml.PMMLObject
{
@XmlAttribute(name = "type")
private TimeAnchor.Type type;
@XmlAttribute(name = "offset")
private Integer offset;
@XmlAttribute(name = "stepsize")
private Integer stepsize;
@XmlAttribute(name = "displayName")
@XmlSchemaType(name = "anySimpleType")
private String displayName;
@XmlElement(name = "TimeCycle", namespace = "http://www.dmg.org/PMML-4_3")
private List timeCycles;
@XmlElement(name = "TimeException", namespace = "http://www.dmg.org/PMML-4_3")
private List timeExceptions;
/**
* Gets the value of the type property.
*
* @return
* possible object is
* {@link TimeAnchor.Type }
*
*/
public TimeAnchor.Type getType() {
return type;
}
/**
* Sets the value of the type property.
*
* @param type
* allowed object is
* {@link TimeAnchor.Type }
*
*/
public TimeAnchor setType(TimeAnchor.Type type) {
this.type = type;
return this;
}
/**
* Gets the value of the offset property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getOffset() {
return offset;
}
/**
* Sets the value of the offset property.
*
* @param offset
* allowed object is
* {@link Integer }
*
*/
public TimeAnchor setOffset(Integer offset) {
this.offset = offset;
return this;
}
/**
* Gets the value of the stepsize property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getStepsize() {
return stepsize;
}
/**
* Sets the value of the stepsize property.
*
* @param stepsize
* allowed object is
* {@link Integer }
*
*/
public TimeAnchor setStepsize(Integer stepsize) {
this.stepsize = stepsize;
return this;
}
/**
* Gets the value of the displayName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDisplayName() {
return displayName;
}
/**
* Sets the value of the displayName property.
*
* @param displayName
* allowed object is
* {@link String }
*
*/
public TimeAnchor setDisplayName(String displayName) {
this.displayName = displayName;
return this;
}
/**
* Gets the value of the timeCycles property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the timeCycles property.
*
*
* For example, to add a new item, do as follows:
*
* getTimeCycles().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link TimeCycle }
*
*
*/
public List getTimeCycles() {
if (timeCycles == null) {
timeCycles = new ArrayList();
}
return this.timeCycles;
}
/**
* Gets the value of the timeExceptions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the timeExceptions property.
*
*
* For example, to add a new item, do as follows:
*
* getTimeExceptions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link TimeException }
*
*
*/
public List getTimeExceptions() {
if (timeExceptions == null) {
timeExceptions = new ArrayList();
}
return this.timeExceptions;
}
public boolean hasTimeCycles() {
return ((this.timeCycles!= null)&&(this.timeCycles.size()> 0));
}
public TimeAnchor addTimeCycles(TimeCycle... timeCycles) {
getTimeCycles().addAll(Arrays.asList(timeCycles));
return this;
}
public boolean hasTimeExceptions() {
return ((this.timeExceptions!= null)&&(this.timeExceptions.size()> 0));
}
public TimeAnchor addTimeExceptions(TimeException... timeExceptions) {
getTimeExceptions().addAll(Arrays.asList(timeExceptions));
return this;
}
@Override
public VisitorAction accept(Visitor visitor) {
VisitorAction status = visitor.visit(this);
if (status == VisitorAction.CONTINUE) {
visitor.pushParent(this);
if ((status == VisitorAction.CONTINUE)&&hasTimeCycles()) {
status = org.dmg.pmml.PMMLObject.traverse(visitor, getTimeCycles());
}
if ((status == VisitorAction.CONTINUE)&&hasTimeExceptions()) {
status = org.dmg.pmml.PMMLObject.traverse(visitor, getTimeExceptions());
}
visitor.popParent();
}
if (status == VisitorAction.TERMINATE) {
return VisitorAction.TERMINATE;
}
return VisitorAction.CONTINUE;
}
/**
* Java class for null.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <enumeration value="dateTimeMillisecondsSince[0]"/>
* <enumeration value="dateTimeMillisecondsSince[1960]"/>
* <enumeration value="dateTimeMillisecondsSince[1970]"/>
* <enumeration value="dateTimeMillisecondsSince[1980]"/>
* <enumeration value="dateTimeSecondsSince[0]"/>
* <enumeration value="dateTimeSecondsSince[1960]"/>
* <enumeration value="dateTimeSecondsSince[1970]"/>
* <enumeration value="dateTimeSecondsSince[1980]"/>
* <enumeration value="dateDaysSince[0]"/>
* <enumeration value="dateDaysSince[1960]"/>
* <enumeration value="dateDaysSince[1970]"/>
* <enumeration value="dateDaysSince[1980]"/>
* <enumeration value="dateMonthsSince[0]"/>
* <enumeration value="dateMonthsSince[1960]"/>
* <enumeration value="dateMonthsSince[1970]"/>
* <enumeration value="dateMonthsSince[1980]"/>
* <enumeration value="dateYearsSince[0]"/>
* </restriction>
* </simpleType>
*
*
*/
@XmlType(name = "")
@XmlEnum
@Added(Version.PMML_4_0)
public enum Type {
@XmlEnumValue("dateTimeMillisecondsSince[0]")
DATE_TIME_MILLISECONDS_SINCE_0("dateTimeMillisecondsSince[0]"),
@XmlEnumValue("dateTimeMillisecondsSince[1960]")
DATE_TIME_MILLISECONDS_SINCE_1960("dateTimeMillisecondsSince[1960]"),
@XmlEnumValue("dateTimeMillisecondsSince[1970]")
DATE_TIME_MILLISECONDS_SINCE_1970("dateTimeMillisecondsSince[1970]"),
@XmlEnumValue("dateTimeMillisecondsSince[1980]")
DATE_TIME_MILLISECONDS_SINCE_1980("dateTimeMillisecondsSince[1980]"),
@XmlEnumValue("dateTimeSecondsSince[0]")
DATE_TIME_SECONDS_SINCE_0("dateTimeSecondsSince[0]"),
@XmlEnumValue("dateTimeSecondsSince[1960]")
DATE_TIME_SECONDS_SINCE_1960("dateTimeSecondsSince[1960]"),
@XmlEnumValue("dateTimeSecondsSince[1970]")
DATE_TIME_SECONDS_SINCE_1970("dateTimeSecondsSince[1970]"),
@XmlEnumValue("dateTimeSecondsSince[1980]")
DATE_TIME_SECONDS_SINCE_1980("dateTimeSecondsSince[1980]"),
@XmlEnumValue("dateDaysSince[0]")
DATE_DAYS_SINCE_0("dateDaysSince[0]"),
@XmlEnumValue("dateDaysSince[1960]")
DATE_DAYS_SINCE_1960("dateDaysSince[1960]"),
@XmlEnumValue("dateDaysSince[1970]")
DATE_DAYS_SINCE_1970("dateDaysSince[1970]"),
@XmlEnumValue("dateDaysSince[1980]")
DATE_DAYS_SINCE_1980("dateDaysSince[1980]"),
@XmlEnumValue("dateMonthsSince[0]")
DATE_MONTHS_SINCE_0("dateMonthsSince[0]"),
@XmlEnumValue("dateMonthsSince[1960]")
DATE_MONTHS_SINCE_1960("dateMonthsSince[1960]"),
@XmlEnumValue("dateMonthsSince[1970]")
DATE_MONTHS_SINCE_1970("dateMonthsSince[1970]"),
@XmlEnumValue("dateMonthsSince[1980]")
DATE_MONTHS_SINCE_1980("dateMonthsSince[1980]"),
@XmlEnumValue("dateYearsSince[0]")
DATE_YEARS_SINCE_0("dateYearsSince[0]");
private final String value;
Type(String v) {
value = v;
}
public String value() {
return value;
}
public static TimeAnchor.Type fromValue(String v) {
for (TimeAnchor.Type c: TimeAnchor.Type.values()) {
if (c.value.equals(v)) {
return c;
}
}
throw new IllegalArgumentException(v);
}
}
}