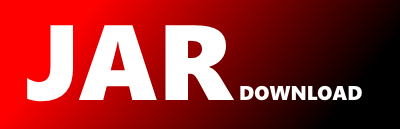
numpy.DType Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pmml-python Show documentation
Show all versions of pmml-python Show documentation
JPMML Python to PMML converter
/*
* Copyright (c) 2015 Villu Ruusmann
*
* This file is part of JPMML-Python
*
* JPMML-Python is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* JPMML-Python is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with JPMML-Python. If not, see .
*/
package numpy;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import numpy.core.TypeDescriptor;
import org.dmg.pmml.DataType;
import org.jpmml.python.CythonObject;
import org.jpmml.python.TypeInfo;
public class DType extends CythonObject implements TypeInfo {
public DType(String module, String name){
super(module, name);
}
@Override
public void __init__(Object[] args){
super.__setstate__(INIT_ATTRIBUTES, args);
}
/**
* https://github.com/numpy/numpy/blob/master/numpy/core/src/multiarray/descriptor.c
*/
@Override
public void __setstate__(Object[] args){
if(args.length == SETSTATE_DATETIME_ATTRIBUTES.length){
super.__setstate__(SETSTATE_DATETIME_ATTRIBUTES, args);
return;
}
super.__setstate__(SETSTATE_ATTRIBUTES, args);
}
@Override
public DataType getDataType(){
TypeDescriptor typeDescriptor = new TypeDescriptor(this);
return typeDescriptor.getDataType();
}
public Object toDescr(){
Map values = getValues();
if(values == null){
String obj = getObj();
String order = getOrder();
return formatDescr(obj, order);
}
Set valueKeys = values.keySet();
List definition = DType.definitions.get(valueKeys);
if(definition != null){
return formatDescr(definition, values);
}
throw new IllegalArgumentException();
}
public boolean hasValues(){
Map values = getValues();
return (values != null);
}
public Map getValues(){
return (Map)getOptionalDict("values");
}
public String getObj(){
return getOptionalString("obj");
}
public String getOrder(){
return getOptionalString("order");
}
public Integer getWSize(){
return getOptionalInteger("w_size");
}
public Object[] getDatetimeData(){
return getOptionalTuple("datetime_data");
}
static
public void addDefinition(List definition){
DType.definitions.put(new HashSet<>(definition), definition);
}
static
public void removeDefinition(List definition){
DType.definitions.remove(new HashSet<>(definition));
}
static
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy