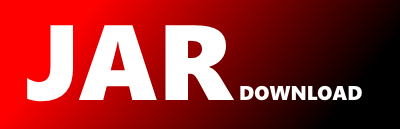
numpy.core.NDArrayUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pmml-python Show documentation
Show all versions of pmml-python Show documentation
JPMML Python to PMML converter
/*
* Copyright (c) 2015 Villu Ruusmann
*
* This file is part of JPMML-Python
*
* JPMML-Python is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* JPMML-Python is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with JPMML-Python. If not, see .
*/
package numpy.core;
import java.io.EOFException;
import java.io.IOException;
import java.io.InputStream;
import java.nio.ByteOrder;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import com.google.common.io.ByteStreams;
import com.google.common.primitives.Ints;
import com.google.common.primitives.Longs;
import net.razorvine.pickle.Unpickler;
import net.razorvine.serpent.Parser;
import net.razorvine.serpent.ast.Ast;
import numpy.DType;
import org.jpmml.converter.ValueUtil;
import org.jpmml.python.CustomUnpickler;
import org.jpmml.python.TupleUtil;
public class NDArrayUtil {
private NDArrayUtil(){
}
static
public int[] getShape(NDArray array){
Object[] shape = array.getShape();
List extends Number> values = (List)Arrays.asList(shape);
return Ints.toArray(ValueUtil.asIntegers(values));
}
/**
* Gets the payload of a one-dimensional array.
*/
static
public List> getContent(NDArray array){
Object content = array.getContent();
return asJavaList(array, (List>)content);
}
/**
* Gets the payload of the specified dimension of a multi-dimensional array.
*
* @param key The dimension.
*/
static
public List> getContent(NDArray array, String key){
Map content = (Map)array.getContent();
return asJavaList(array, (List>)content.get(key));
}
static
public NDArray toArray(List> data){
NDArray result = new NDArray();
result.put("data", data);
result.put("fortran_order", Boolean.FALSE);
result.put("shape", new Object[]{data.size()});
return result;
}
static
private List asJavaList(NDArray array, List values){
return asJavaList(array, values, false);
}
static
private List asJavaList(NDArray array, List values, boolean fortranOrderShape){
boolean fortranOrder = array.getFortranOrder();
if(fortranOrder){
int[] shape = getShape(array);
switch(shape.length){
case 1:
return values;
case 2:
if(fortranOrderShape){
shape = new int[]{shape[1], shape[0]};
}
return toJavaList(values, shape[0], shape[1]);
default:
throw new IllegalArgumentException();
}
}
return values;
}
/**
* Translates a column-major (ie. Fortran-type) array to a row-major (ie. C-type) array.
*/
static
private List toJavaList(List values, int rows, int columns){
List result = new ArrayList<>(values.size());
for(int i = 0; i < values.size(); i++){
int row = i / columns;
int column = i % columns;
E value = values.get((column * rows) + row);
result.add(value);
}
return result;
}
/**
* https://numpy.org/doc/stable/reference/generated/numpy.lib.format.html
*/
static
public NDArray parseNpy(InputStream is) throws IOException {
byte[] magicBytes = new byte[MAGIC_STRING.length];
ByteStreams.readFully(is, magicBytes);
if(!Arrays.equals(magicBytes, MAGIC_STRING)){
throw new IOException();
}
int majorVersion = readUnsignedByte(is);
int minorVersion = readUnsignedByte(is);
if(majorVersion != 1 || minorVersion != 0){
throw new IOException();
}
int headerLength = readUnsignedShort(is, ByteOrder.LITTLE_ENDIAN);
if(headerLength < 0){
throw new IOException();
}
byte[] headerBytes = new byte[headerLength];
ByteStreams.readFully(is, headerBytes);
String header = new String(headerBytes);
// Remove trailing whitespace
header = header.trim();
Map headerDict = parseDict(header);
Object descr = headerDict.get("descr");
Boolean fortranOrder = (Boolean)headerDict.get("fortran_order");
Object[] shape = (Object[])headerDict.get("shape");
byte[] data = ByteStreams.toByteArray(is);
NDArray array = new NDArray();
array.__setstate__(new Object[]{Arrays.asList(majorVersion, minorVersion), shape, descr, fortranOrder, data});
return array;
}
static
public Object parseData(InputStream is, Object descr, Object[] shape) throws IOException {
return parseData(is, descr, shape, null);
}
static
public Object parseData(InputStream is, Object descr, Object[] shape, Integer numpyArrayAlignmentBytes) throws IOException {
boolean simple = false;
Integer wSize = null;
if(descr instanceof DType){
DType dtype = (DType)descr;
simple = !dtype.hasValues();
wSize = dtype.getWSize();
}
int length = 1;
for(int i = 0; i < shape.length; i++){
length *= ValueUtil.asInt((Number)shape[i]);
} // End if
if(simple){
return parseArray(is, descr, length, numpyArrayAlignmentBytes);
} else
{
if(descr instanceof DType){
DType dtype = (DType)descr;
descr = dtype.toDescr();
}
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy