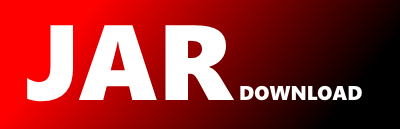
org.jppf.client.concurrent.AbstractJobConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jppf-client Show documentation
Show all versions of jppf-client Show documentation
JPPF, the open source grid computing solution
/*
* JPPF.
* Copyright (C) 2005-2015 JPPF Team.
* http://www.jppf.org
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jppf.client.concurrent;
import java.util.*;
import org.jppf.client.event.JobListener;
import org.jppf.client.persistence.JobPersistence;
import org.jppf.node.protocol.*;
/**
* Abstract implementation of the JobConfiguration
interface.
* @author Laurent Cohen
* @exclude
*/
abstract class AbstractJobConfiguration implements JobConfiguration {
/**
* The service level agreement between the job and the server.
*/
private JobSLA jobSLA = new JPPFJobSLA();
/**
* The service level agreement between the job and the server.
*/
private JobClientSLA jobClientSLA = new JPPFJobClientSLA();
/**
* The user-defined metadata associated with this job.
*/
private JobMetadata jobMetadata = new JPPFJobMetadata();
/**
* The persistence manager that enables saving and restoring the state of this job.
*/
private JobPersistence> persistenceManager = null;
/**
* The data provider to set onto the job.
*/
private DataProvider dataProvider = null;
/**
* The list of listeners to register with the job.
*/
private List listeners = new LinkedList<>();
/**
* A list of class loaders used to load the classes needed to run the jobs.
*/
protected final List classLoaders = new ArrayList<>();
/**
* Default constructor.
*/
protected AbstractJobConfiguration() {
}
/**
* Copy constructor.
* @param sla the sla configuration.
* @param metadata the metadata configuration to use.
* @param persistenceManager the persistence manager to use.
*/
AbstractJobConfiguration(final JobSLA sla, final JobMetadata metadata, final JobPersistence persistenceManager) {
this.jobSLA = sla;
this.jobMetadata = metadata;
this.persistenceManager = persistenceManager;
}
@Override
public JobSLA getSLA() {
return jobSLA;
}
/**
* Get the service level agreement between the job and the server.
* @param jobSLA an instance of JPPFJobSLA
.
*/
public void setSLA(final JobSLA jobSLA) {
this.jobSLA = jobSLA;
}
@Override
public JobClientSLA getClientSLA() {
return jobClientSLA;
}
/**
* Get the service level agreement between the job and the server.
* @param jobClientSLA an instance of JPPFJobSLA
.
*/
public void setClientSLA(final JobClientSLA jobClientSLA) {
this.jobClientSLA = jobClientSLA;
}
@Override
public JobMetadata getMetadata() {
return jobMetadata;
}
/**
* Set this job's metadata.
* @param jobMetadata a {@link JPPFJobMetadata} instance.
*/
public void setMetadata(final JobMetadata jobMetadata) {
this.jobMetadata = jobMetadata;
}
@Override
@SuppressWarnings("unchecked")
public JobPersistence getPersistenceManager() {
return (JobPersistence) persistenceManager;
}
@Override
public void setPersistenceManager(final JobPersistence persistenceManager) {
this.persistenceManager = persistenceManager;
}
@Override
public DataProvider getDataProvider() {
return dataProvider;
}
@Override
public void setDataProvider(final DataProvider dataProvider) {
this.dataProvider = dataProvider;
}
@Override
public void addJobListener(final JobListener listener) {
synchronized(listeners) {
listeners.add(listener);
}
}
@Override
public void removeJobListener(final JobListener listener) {
synchronized(listeners) {
listeners.remove(listener);
}
}
@Override
public List getAllJobListeners() {
synchronized(listeners) {
return listeners;
}
}
@Override
public List getClassLoaders() {
return classLoaders;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy