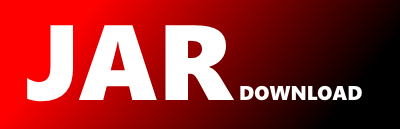
org.jppf.management.JPPFSystemInformation Maven / Gradle / Ivy
Show all versions of jppf-common Show documentation
/*
* JPPF.
* Copyright (C) 2005-2015 JPPF Team.
* http://www.jppf.org
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jppf.management;
import java.util.*;
import org.jppf.utils.*;
import org.slf4j.*;
/**
* This class encapsulates the system information for a node.
* It includes:
*
* - System properties, including -D flags
* - Runtime information such as available processors and memory usage
* - Environment variables
* - JPPF configuration properties
* - IPV4 and IPV6 addresses assigned to the JVM host
* - Disk space information (JDK 1.6 or later only)
*
* @author Laurent Cohen
*/
public class JPPFSystemInformation implements PropertiesCollection {
/**
* Explicit serialVersionUID.
*/
private static final long serialVersionUID = 1L;
/**
* Logger for this class.
*/
private static Logger log = LoggerFactory.getLogger(JPPFSystemInformation.class);
/**
* Determines whether the trace level is enabled in the log configuration, without the cost of a method call.
*/
private static boolean traceEnabled = log.isTraceEnabled();
/**
* Mapping of all properties containers.
*/
private final Map map = new LinkedHashMap<>();
/**
* Holds the latests set of properties maps.
*/
private TypedProperties[] propertiesArray = new TypedProperties[0];
/**
* true
if the JPPF component is local (local node or local client executor), false
otherwise.
*/
private final boolean local;
/**
* If true
, then the name resolution for InetAddress
es should occur immediately,
*/
private final boolean resolveInetAddressesNow;
/**
* Initialize this system information object with the specified uuid.
* @param uuid the uuid of the corresponding JPPF component.
* @param local true
if the JPPF component is local (local node or local client executor), false
otherwise.
* @param resolveInetAddressesNow if true
, then name resolution for InetAddress
es should occur immediately,
* otherwise it is different and executed in a separate thread.
*/
public JPPFSystemInformation(final String uuid, final boolean local, final boolean resolveInetAddressesNow) {
this.local = local;
this.resolveInetAddressesNow = resolveInetAddressesNow;
TypedProperties uuidProps = new TypedProperties();
uuidProps.setProperty("jppf.uuid", (uuid == null) ? "" : uuid);
uuidProps.setInt("jppf.pid", SystemUtils.getPID());
VersionUtils.Version v = VersionUtils.getVersion();
uuidProps.setProperty("jppf.version.number", v.getVersionNumber());
uuidProps.setProperty("jppf.build.number", v.getBuildNumber());
uuidProps.setProperty("jppf.build.date", v.getBuildDate());
addProperties("uuid", uuidProps);
populate();
}
/**
* Get the map holding the system properties.
* @return a TypedProperties
instance.
* @see org.jppf.utils.SystemUtils#getSystemProperties()
*/
public TypedProperties getSystem() {
return getProperties("system");
}
/**
* Get the map holding the runtime information.
* The resulting map will contain the following properties:
*
* - availableProcessors = number of processors available to the JVM
* - freeMemory = current free heap size in bytes
* - totalMemory = current total heap size in bytes
* - maxMemory = maximum heap size in bytes (i.e. as specified by -Xmx JVM option)
*
* Some or all of these properties may be missing if a security manager is installed
* that does not grant access to the related {@link java.lang.Runtime} APIs.
* @return a TypedProperties
instance.
* @see org.jppf.utils.SystemUtils#getRuntimeInformation()
*/
public TypedProperties getRuntime() {
return getProperties("runtime");
}
/**
* Get the map holding the environment variables.
* @return a TypedProperties
instance.
* @see org.jppf.utils.SystemUtils#getEnvironment()
*/
public TypedProperties getEnv() {
return getProperties("env");
}
/**
* Get the map of the network configuration.
*
The resulting map will contain the following properties:
*
* - ipv4.addresses = hostname_1|ipv4_address_1 ... hostname_n|ipv4_address_n
* - ipv6.addresses = hostname_1|ipv6_address_1 ... hostname_p|ipv6_address_p
*
* Each property is a space-separated list of hostname|ip_address pairs,
* the hostname and ip address being separated by a pipe symbol "|".
* @return a TypedProperties
instance.
* @see org.jppf.utils.SystemUtils#getNetwork()
*/
public TypedProperties getNetwork() {
return getProperties("network");
}
/**
* Get the map holding the JPPF configuration properties.
* @return a TypedProperties
instance.
* @see org.jppf.utils.JPPFConfiguration
*/
public TypedProperties getJppf() {
return getProperties("jppf");
}
/**
* Get the map holding the host storage information.
*
The map will contain the following information:
*
* - host.roots.names = root_name_0 ... root_name_n-1 : the names of all accessible file system roots
* - host.roots.number = n : the number of accessible file system roots
* - For each root i:
* - root.i.name = root_name : for instance "C:\" on Windows or "/" on Unix
* - root.i.space.free = space_in_bytes : current free space for the root
* - root.i.space.total = space_in_bytes : total space for the root
* - root.i.space.usable = space_in_bytes : space available to the user the JVM is running under
*
* If the JVM version is prior to 1.6, the space information will not be available.
* @return a TypedProperties
instance.
* @see org.jppf.utils.SystemUtils#getStorageInformation()
*/
public TypedProperties getStorage() {
return getProperties("storage");
}
/**
* Get the properties object holding the JPPF uuid and version information.
* The following properties are provided:
*
* - "jppf.uuid" : the uuid of the node or driver
* - "jppf.version.number" : the current JPPF version number
* - "jppf.build.number" : the current build number
* - "jppf.build.date" : the build date, including the time zone, in the format "yyyy-MM-dd hh:mm z"
*
* @return a TypedProperties
wrapper for the uuid and version information of the corresponding JPPF component.
*/
public TypedProperties getUuid() {
return getProperties("uuid");
}
/**
* Get the properties object holding the operating system information.
* The following properties are provided:
*
* - "os.TotalPhysicalMemorySize" : total physical RAM (long value)
* - "os.FreePhysicalMemorySize" : available physical RAM (long value)
* - "os.TotalSwapSpaceSize" : total swap space (long value)
* - "os.FreeSwapSpaceSize" : available swap space (long value)
* - "os.CommittedVirtualMemorySize" : total committed virtual memory of the process (long value)
* - "os.ProcessCpuLoad" : process CPU load (double value in range [0 ... 1])
* - "os.ProcessCpuTime" : process CPU time (long value)
* - "os.SystemCpuLoad" : system total CPU load (double value in range [0 ... 1])
* - "os.Name" : the name of the OS (string value, ex: "Windows 7")
* - "os.Version" : the OS version (string value, ex: "6.1")
* - "os.Arch" : the OS architecture (string value, ex: "amd64")
* - "os.AvailableProcessors" : number of processors available to the OS (int value)
* - "os.SystemLoadAverage" : average system CPU load over the last minute (double value in the range [0 ... 1], always returns -1 on Windows)
*
* @return a TypedProperties
wrapper for the operating system information of the corresponding JPPF component.
*/
public TypedProperties getOS() {
return getProperties("os");
}
/**
* Get all the properties as an array.
* @return an array of all the sets of properties.
*/
@Override
public TypedProperties[] getPropertiesArray() {
synchronized(map) {
return propertiesArray;
}
}
@Override
public void addProperties(final String key, final TypedProperties properties) {
synchronized(map) {
map.put(key, properties);
propertiesArray = map.values().toArray(new TypedProperties[map.size()]);
}
}
@Override
public TypedProperties getProperties(final String key) {
synchronized(map) {
return map.get(key);
}
}
/**
* Populate this system information object.
* @return this JPPFSystemInformation
object.
*/
public JPPFSystemInformation populate() {
if (traceEnabled) {
Exception e = new Exception("call stack for JPPFSystemInformation.populate(" + resolveInetAddressesNow + ")");
log.trace(e.getMessage(), e);
}
addProperties("system", SystemUtils.getSystemProperties());
addProperties("runtime", SystemUtils.getRuntimeInformation());
addProperties("env", SystemUtils.getEnvironment());
addProperties("jppf", new TypedProperties(JPPFConfiguration.getProperties()));
getJppf().setProperty("jppf.channel.local", String.valueOf(local));
Runnable r = new Runnable() {
@Override
public void run() {
addProperties("network", SystemUtils.getNetwork());
}
};
if (resolveInetAddressesNow) r.run();
else new Thread(r).start();
addProperties("storage", SystemUtils.getStorageInformation());
if (getProperties("uuid") == null) addProperties("uuid", new TypedProperties());
addProperties("os", SystemUtils.getOS());
return this;
}
/**
* Parse the list of IP v4 addresses contained in this JPPFSystemInformation instance.
* This method is provided as a convenience so developers don't have to do the parsing themselves.
* @return an array of HostIP
instances.
* @exclude
*/
public HostIP[] parseIPV4Addresses() {
return NetworkUtils.parseAddresses(getNetwork().getString("ipv4.addresses"));
}
/**
* Parse the list of IP v6 addresses contained in this JPPFSystemInformation instance.
* This method is provided as a convenience so developers don't have to do the parsing themselves.
* @return an array of HostIP
instances.
* @exclude
*/
public HostIP[] parseIPV6Addresses() {
return NetworkUtils.parseAddresses(getNetwork().getString("ipv6.addresses"));
}
}