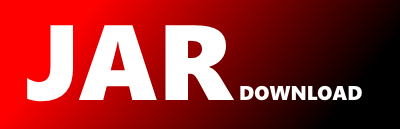
org.jppf.nio.AbstractNioContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jppf-common Show documentation
Show all versions of jppf-common Show documentation
JPPF, the open source grid computing solution
/*
* JPPF.
* Copyright (C) 2005-2015 JPPF Team.
* http://www.jppf.org
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jppf.nio;
import java.util.concurrent.atomic.AtomicBoolean;
/**
* Context associated with an open communication channel.
* @param the type of states associated with this context.
* @author Laurent Cohen
*/
public abstract class AbstractNioContext> implements NioContext {
/**
* The current state of the channel this context is associated with.
*/
protected S state = null;
/**
* Uuid of the remote client or node.
*/
protected String uuid = null;
/**
* Container for the current message data.
*/
protected NioMessage message = null;
/**
* The associated channel.
*/
protected ChannelWrapper> channel = null;
/**
* Unique ID for the corresponding connection on the remote peer.
*/
protected String connectionUuid = null;
/**
* The SSL engine associated with the channel.
*/
protected SSLHandler sslHandler = null;
/**
* Determines whether the associated channel is connected to a peer server.
*/
protected boolean peer = false;
/**
* Determines whether the connection was opened on an SSL port.
*/
protected boolean ssl = false;
/**
* Whether this context is enabled.
*/
protected boolean enabled = true;
/**
* Whether this context has been closed.
*/
protected final AtomicBoolean closed = new AtomicBoolean(false);
@Override
public S getState() {
return state;
}
@Override
public boolean setState(final S state) {
this.state = state;
return true;
}
@Override
public String getUuid() {
return uuid;
}
@Override
public void setUuid(final String uuid) {
this.uuid = uuid;
}
/**
* Give the non qualified name of the class of this instance.
* @return a class name as a string.
*/
protected String getShortClassName() {
String fqn = getClass().getName();
int idx = fqn.lastIndexOf('.');
return fqn.substring(idx + 1);
}
/**
* Get the container for the current message data.
* @return an NioMessage
instance.
*/
public NioMessage getMessage() {
return message;
}
/**
* Set the container for the current message data.
* @param message an NioMessage
instance.
*/
public void setMessage(final NioMessage message) {
this.message = message;
}
@Override
public ChannelWrapper> getChannel() {
return channel;
}
@Override
public void setChannel(final ChannelWrapper> channel) {
this.channel = channel;
}
/**
* Get the unique ID for the corresponding connection on the remote peer.
* @return the id as a string.
*/
public String getConnectionUuid() {
return connectionUuid;
}
/**
* Set the unique ID for the corresponding connection on the remote peer.
* @param connectionUuid the id as a string.
*/
public void setConnectionUuid(final String connectionUuid) {
this.connectionUuid = connectionUuid;
}
@Override
public SSLHandler getSSLHandler() {
return sslHandler;
}
@Override
public void setSSLHandler(final SSLHandler sslHandler) {
this.sslHandler = sslHandler;
}
@Override
public boolean isPeer() {
return peer;
}
@Override
public void setPeer(final boolean peer) {
this.peer = peer;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder(getClass().getSimpleName()).append('[');
sb.append("channel=").append(channel.getClass().getSimpleName()).append("[id=").append(channel.getId()).append(']');
sb.append(", state=").append(getState());
sb.append(", uuid=").append(uuid);
sb.append(", connectionUuid=").append(connectionUuid);
sb.append(", peer=").append(peer);
sb.append(", ssl=").append(ssl);
sb.append(']');
return sb.toString();
}
/**
* Determine whether the associated channel is secured via SSL/TLS.
* @return true
if the channel is secure, false
otherwise.
*/
public boolean isSecure() {
return sslHandler != null;
}
/**
* Determines whether the connection was opened on an SSL port.
* @return true
for an SSL connection, false
otherwise.
*/
@Override
public boolean isSsl() {
return ssl;
}
/**
* Specifies whether the connection was opened on an SSL port.
* @param ssl true
for an SSL connection, false
otherwise.
*/
@Override
public void setSsl(final boolean ssl) {
this.ssl = ssl;
}
@Override
public boolean isEnabled() {
return enabled;
}
@Override
public void setEnabled(final boolean enabled) {
this.enabled = enabled;
}
/**
* Determine whether this channel has been closed.
* @return {@code true} if this channel has been closed, {@code false} otherwise.
*/
public boolean isClosed() {
return closed.get();
}
/**
* Specify whether this channel has been closed.
* @param closed {@code true} if this channel has been closed, {@code false} otherwise.
*/
public void setClosed(final boolean closed) {
this.closed.set(closed);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy