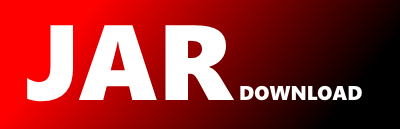
org.jppf.node.protocol.JPPFExceptionResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jppf-common Show documentation
Show all versions of jppf-common Show documentation
JPPF, the open source grid computing solution
/*
* JPPF.
* Copyright (C) 2005-2015 JPPF Team.
* http://www.jppf.org
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jppf.node.protocol;
import org.jppf.utils.ExceptionUtils;
/**
* Instances of this class are used to signal that a task could not be sent back by the node to the server.
* This generally happens when a task cannot be serialized after its execution, or if a data transformation is
* applied and fails with an exception. An instance of this class captures the context of the error, including the exception
* that occurred, the object's toString()
descriptor and its class name.
*
When such an error occurs, an instance of this class will be sent instead of the initial JPPF task.
* @author Laurent Cohen
*/
public final class JPPFExceptionResult extends AbstractTask
© 2015 - 2025 Weber Informatics LLC | Privacy Policy