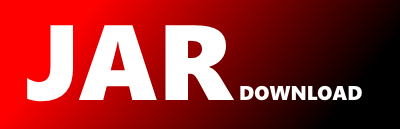
org.jppf.serialization.JPPFSerialization Maven / Gradle / Ivy
Show all versions of jppf-common Show documentation
/*
* JPPF.
* Copyright (C) 2005-2015 JPPF Team.
* http://www.jppf.org
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jppf.serialization;
import java.io.*;
import org.jppf.JPPFError;
import org.jppf.utils.*;
import org.slf4j.*;
/**
* Interface and factory for object serialization and deserialization schemes in JPPF.
* A serialization scheme is defined as an implementation of this interface,
* and configured in a JPPF configuration file using the property definition:
* jppf.object.serialization.class = my.implementation.OfJPPFSerialization
*
The same implementation must be used for all nodes, servers and clients.
* @author Laurent Cohen
*/
public interface JPPFSerialization {
/**
* Configuration property name for object serialization.
*/
String SERIALIZATION_CLASS = "jppf.object.serialization.class";
/**
* Serialize an object into the specified output stream.
* @param o the object to serialize.
* @param os the stream that receives the serialized object.
* @throws Exception if any error occurs.
*/
void serialize(Object o, OutputStream os) throws Exception;
/**
* Deserialize an object from the specified input stream.
* @param is the stream from which to deserialize.
* @return the serialized object.
* @throws Exception if any error occurs.
*/
Object deserialize(InputStream is) throws Exception;
/**
* Factory class for instantiating a default or configured serialization.
*/
public static class Factory {
/**
* Logger for this class.
*/
private static Logger log = LoggerFactory.getLogger(Factory.class);
/**
* Determines whether the debug level is enabled in the log configuration, without the cost of a method call.
*/
private static boolean debugEnabled = LoggingUtils.isDebugEnabled(log);
/**
* The serialization to use.
*/
private static JPPFSerialization serialization = init();
/**
* Initialize the serialization.
* @return the defined {@link JPPFSerialization} instance.
*/
private static JPPFSerialization init() {
String className = JPPFConfiguration.getProperties().getString(SERIALIZATION_CLASS);
if (debugEnabled) log.debug("found " + SERIALIZATION_CLASS + " = " + className);
if (className != null) {
try {
Class> clazz = Class.forName(className);
return (JPPFSerialization) clazz.newInstance();
} catch (Exception e) {
StringBuilder sb = new StringBuilder();
sb.append("Could not instantiate JPPF serialization [").append(SERIALIZATION_CLASS).append(" = ").append(className);
sb.append(", terminating this application");
log.error(sb.toString(), e);
throw new JPPFError(sb.toString(), e);
}
}
if (debugEnabled) log.debug("using DefaultJavaSerialization");
return new DefaultJavaSerialization();
}
/**
* Get the configured serialization.
* @return an instance of {@link JPPFSerialization}.
*/
public static JPPFSerialization getSerialization() {
return serialization;
}
/**
* Reset the configured serialization.
* @exclude
*/
public static void reset() {
serialization = init();
}
}
}