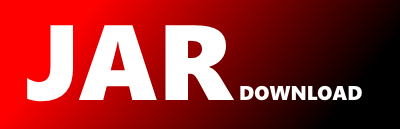
org.jppf.utils.compilation.ErrorReporter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jppf-common Show documentation
Show all versions of jppf-common Show documentation
JPPF, the open source grid computing solution
/*
* JPPF.
* Copyright (C) 2005-2015 JPPF Team.
* http://www.jppf.org
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jppf.utils.compilation;
import java.util.*;
import javax.tools.*;
/**
* A simple DiagnosticListener
which exposes the collected Diagnostic
s via a List
* and allows resetting its state.
* @param the type of source object on which the diagnostics are reported.
* @author Laurent Cohen
*/
class ErrorReporter implements DiagnosticListener
{
/**
* The list of errors generated by the compilation.
*/
private List> errors = new ArrayList<>();
/**
* The list of warnings generated by the compilation.
*/
private List> warnings = new ArrayList<>();
/**
* {@inheritDoc}
*/
@Override
public void report(final Diagnostic extends S> diagnostic)
{
switch (diagnostic.getKind())
{
case ERROR:
errors.add(diagnostic);
break;
case WARNING:
case MANDATORY_WARNING:
warnings.add(diagnostic);
break;
}
}
/**
* Get the diagnostics gathered by this ErrorReporter
.
* @return a list of {@link Diagnostic} objects.
*/
public List> getErrors()
{
return new ArrayList<>(errors);
}
/**
* Get the diagnostics gathered by this ErrorReporter
.
* @return a list of {@link Diagnostic} objects.
*/
public List> getWarnings()
{
return new ArrayList<>(warnings);
}
/**
* Clear the diagnostics previously gathered by this ErrorReporter
.
*/
public void clear()
{
errors.clear();
warnings.clear();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy