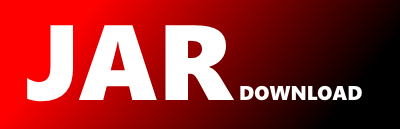
commonMain.org.jraf.klibnotion.client.NotionClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of klibnotion-jvm Show documentation
Show all versions of klibnotion-jvm Show documentation
A Notion API client library for Kotlin, Java and more.
/*
* This source is part of the
* _____ ___ ____
* __ / / _ \/ _ | / __/___ _______ _
* / // / , _/ __ |/ _/_/ _ \/ __/ _ `/
* \___/_/|_/_/ |_/_/ (_)___/_/ \_, /
* /___/
* repository.
*
* Copyright (C) 2021-present Benoit 'BoD' Lubek ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jraf.klibnotion.client
import org.jraf.klibnotion.internal.client.NotionClientImpl
import org.jraf.klibnotion.model.base.UuidString
import org.jraf.klibnotion.model.block.Block
import org.jraf.klibnotion.model.block.BlockListProducer
import org.jraf.klibnotion.model.block.MutableBlockList
import org.jraf.klibnotion.model.database.Database
import org.jraf.klibnotion.model.database.query.DatabaseQuery
import org.jraf.klibnotion.model.database.query.DatabaseQuerySort
import org.jraf.klibnotion.model.page.Page
import org.jraf.klibnotion.model.pagination.Pagination
import org.jraf.klibnotion.model.pagination.ResultPage
import org.jraf.klibnotion.model.property.value.PropertyValueList
import org.jraf.klibnotion.model.user.User
import kotlin.jvm.JvmStatic
interface NotionClient {
companion object {
@JvmStatic
fun newInstance(configuration: ClientConfiguration): NotionClient =
NotionClientImpl(configuration)
}
/**
* User related APIs.
*/
interface Users {
/**
* Retrieve a user.
* @see Retrieve a user
*/
suspend fun getUser(id: UuidString): User
/**
* List all users.
* @see List all users
*/
suspend fun getUserList(pagination: Pagination = Pagination()): ResultPage
}
/**
* Database related APIs.
*/
interface Databases {
/**
* Retrieve a database.
* @see Retrieve a database
*/
suspend fun getDatabase(id: UuidString): Database
/**
* List Databases.
* This lists all the databases that have been shared with your bot.
* @see List Databases
*/
suspend fun getDatabaseList(pagination: Pagination = Pagination()): ResultPage
/**
* Query a database.
* @see Query a database
*/
suspend fun queryDatabase(
id: UuidString,
query: DatabaseQuery? = null,
sort: DatabaseQuerySort? = null,
pagination: Pagination = Pagination(),
): ResultPage
}
/**
* Page related APIs.
*/
interface Pages {
/**
* Retrieve a page.
* @see Retrieve a page
*/
suspend fun getPage(id: UuidString): Page
/**
* Create a page.
* @see Create a page
*/
suspend fun createPage(
parentDatabaseId: UuidString,
properties: PropertyValueList = PropertyValueList(),
content: MutableBlockList? = null,
): Page
/**
* Create a page.
* @see Create a page
*/
suspend fun createPage(
parentDatabaseId: UuidString,
properties: PropertyValueList = PropertyValueList(),
content: BlockListProducer,
): Page
/**
* Update a page.
* @see Update page properties
*/
suspend fun updatePage(id: UuidString, properties: PropertyValueList): Page
}
/**
* Blocks (content) related APIs.
*/
interface Blocks {
/**
* Retrieve children blocks of the specified object.
* @see Retrieve block children
*/
suspend fun getBlockList(parentId: UuidString, pagination: Pagination = Pagination()): ResultPage
/**
* Append blocks to the children of the specified object.
* @see Append block children
*/
suspend fun appendBlockList(parentId: UuidString, blocks: MutableBlockList)
/**
* Append blocks to the children of the specified object.
* @see Append block children
*/
suspend fun appendBlockList(parentId: UuidString, blocks: BlockListProducer)
}
/**
* User related APIs.
*/
val users: Users
/**
* Database related APIs.
*/
val databases: Databases
/**
* Page related APIs.
*/
val pages: Pages
/**
* Page related APIs.
*/
val blocks: Blocks
/**
* Dispose of this client instance.
* This will release some resources so it is recommended to call it after use.
*
* **Note: this client will no longer be usable after this is called.**
*/
fun close()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy