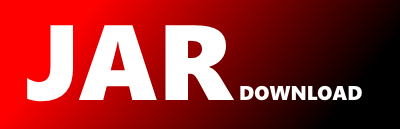
org.jreliability.function.InverseFunction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jreliability Show documentation
Show all versions of jreliability Show documentation
A Java-based reliability evaluation library.
The newest version!
/**
* JReliability is free software: you can redistribute it and/or modify it under the terms of the GNU Lesser General
* Public License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any
* later version.
*
* JReliability is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied
* warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*
* You should have received a copy of the GNU Lesser General Public License along with Opt4J. If not, see
* http://www.gnu.org/licenses/.
*/
package org.jreliability.function;
/**
* The {@code InverseFunction} determines the inverse reliability {@code
* R^-1(x)}. It calculates a {@code y} in {@code x = R(y)} for a given {@code x} and the {@code ReliabilityFunction}
* {@code R(x)} via a bisection approach.
*
* @author glass
*
*/
public class InverseFunction implements Function {
/**
* The {@code ReliabilityFunction} for which the inverse is to determine.
*/
protected final ReliabilityFunction reliabilityFunction;
/**
* The allowed error / {@code epsilon} for embedded bisection method.
*/
protected final double epsilon;
/**
* Constructs an {@code InverseFunction} with a given {@code
* ReliabilityFunction} and an error {@code epsilon} for the embedded bisection method.
*
* @param reliabilityFunction
* the reliabilityFunction
*
* @param epsilon
* the error of the bisection method
*/
public InverseFunction(ReliabilityFunction reliabilityFunction, double epsilon) {
this.reliabilityFunction = reliabilityFunction;
this.epsilon = epsilon;
}
/**
* Constructs an {@code InverseFunction} with a given {@code
* ReliabilityFunction} and an acceptable error of 1.0E-5.
*
* @param reliabilityFunction
* the reliabilityFunction
*/
public InverseFunction(ReliabilityFunction reliabilityFunction) {
this(reliabilityFunction, 1.0E-7);
}
/*
* (non-Javadoc)
*
* @see org.jreliability.function.Function#getY(double)
*/
@Override
public double getY(double x) {
double y;
double diff;
double low = 0;
double high = 2;
// Fast calculation of the bounds
while (reliabilityFunction.getY(high) > x) {
low = high;
high *= 2;
}
// Bisection
do {
y = high - ((high - low) / 2);
double tmpX = reliabilityFunction.getY(y);
if (tmpX < x) {
high = y;
} else {
low = y;
}
diff = Math.abs(x - tmpX);
} while (diff > epsilon);
return y;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy