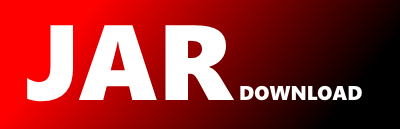
org.jresearch.commons.base.mail.MailSettings Maven / Gradle / Ivy
The newest version!
package org.jresearch.commons.base.mail;
public class MailSettings implements IMailSettings {
private String host;
private int port;
private boolean useTls;
private String from;
private String username;
private String password;
private String portString;
private String useTlsString;
private String errorTo;
public static IMailSettings clone(IMailSettings configuration) {
try {
return configuration == null ? null : configuration.clone();
} catch (CloneNotSupportedException e) {
return new MailSettings(configuration);
}
}
public MailSettings() {
}
public MailSettings(IMailSettings configuration) {
host = configuration.getHost();
from = configuration.getFrom();
username = configuration.getUsername();
password = configuration.getPassword();
port = configuration.getPort();
useTls = configuration.isUseTls();
errorTo = configuration.getErrorTo();
}
@Override
public MailSettings clone() throws CloneNotSupportedException {
return (MailSettings) super.clone();
}
/**
* @param host
* the host to set
*/
public void setHost(String host) {
this.host = host;
}
/**
* @param from
* the from to set
*/
public void setFrom(String from) {
this.from = from;
}
/**
* @param username
* the username to set
*/
public void setUsername(String username) {
this.username = username;
}
/**
* @param password
* the password to set
*/
public void setPassword(String password) {
this.password = password;
}
/**
* @return the host
*/
@Override
public String getHost() {
return host;
}
/**
* @return the from
*/
@Override
public String getFrom() {
return from;
}
/**
* @return the username
*/
@Override
public String getUsername() {
return username;
}
/**
* @return the password
*/
@Override
public String getPassword() {
return password;
}
/**
* @return the port
*/
@Override
public int getPort() {
return port;
}
/**
* @param port
* the port to set
*/
public void setPort(int port) {
this.port = port;
}
/**
* @return the useTls
*/
@Override
public boolean isUseTls() {
return useTls;
}
/**
* @param useTls
* the useTls to set
*/
public void setUseTls(boolean useTls) {
this.useTls = useTls;
}
/**
* @return the portString
*/
public String getPortString() {
return portString;
}
/**
* @param portString
* the portString to set
*/
public void setPortString(String portString) {
this.portString = portString;
}
protected void init() {
if (isCorrect()) {
try {
port = Integer.parseInt(portString);
} catch (NumberFormatException e) {
port = 25;
}
useTls = Boolean.parseBoolean(useTlsString);
}
}
@Override
public boolean isCorrect() {
return host != null && !host.startsWith("@[jrs-email]"); //$NON-NLS-1$
}
/**
* @param useTlsString
* the useTlsString to set
*/
public void setUseTlsString(String useTlsString) {
this.useTlsString = useTlsString;
}
@Override
public String getErrorTo() {
return errorTo;
}
/**
* @param errorTo
* the errorTo to set
*/
public void setErrorTo(String errorTo) {
this.errorTo = errorTo;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy