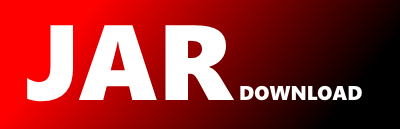
org.jresearch.commons.base.domain.AttributeValue Maven / Gradle / Ivy
The newest version!
package org.jresearch.commons.base.domain;
import javax.persistence.Column;
import javax.persistence.ManyToOne;
import javax.persistence.MappedSuperclass;
import org.jresearch.commons.base.domain.ref.BusinessType;
import org.jresearch.commons.base.domain.ref.ParamType;
import com.google.common.base.MoreObjects;
@MappedSuperclass
public abstract class AttributeValue extends ExtDomainJpa {
@Column(name = "ATTR_VALUE_STR")
private String valueStr;
@Column(name = "ATTR_VALUE_INT")
private Integer valueInt;
@Column(name = "ATTR_VALUE_DOUBLE")
private Double valueDouble;
@ManyToOne
private BusinessType businessType;
public String getValueStr() {
return valueStr;
}
public void setValueStr(final String valueStr) {
this.valueStr = valueStr;
}
public Integer getValueInt() {
return valueInt;
}
public void setValueInt(final Integer valueInt) {
this.valueInt = valueInt;
}
public Double getValueDouble() {
return valueDouble;
}
public void setValueDouble(final Double valueDouble) {
this.valueDouble = valueDouble;
}
public BusinessType getBusinessType() {
return businessType;
}
public void setBusinessType(final BusinessType businessType) {
this.businessType = businessType;
}
public static void setTypedValue(final AttributeValue attr) {
final BusinessType type = attr.getBusinessType();
final String valueStr = attr.getValueStr();
if (type != null && valueStr != null) {
if (type.getParameterType() == null) {
throw new IllegalArgumentException("ParameterType is not set for BusinessType"); //$NON-NLS-1$
}
if (type.getParameterType().getCode() == null) {
throw new IllegalArgumentException("ParameterType code is not set for ParamType"); //$NON-NLS-1$
}
final String paramCode = type.getParameterType().getCode();
switch (paramCode) {
case ParamType.STRING:
attr.setValueStr(valueStr);
break;
case ParamType.DECIMAL:
attr.setValueDouble(Double.valueOf(valueStr));
break;
case ParamType.LONG:
case ParamType.DICTIONARY:
attr.setValueInt(Integer.valueOf(valueStr));
break;
default:
throw new IllegalArgumentException("This parameter type is currently unsupported for custom attributes"); //$NON-NLS-1$
}
}
}
@SuppressWarnings({ "nls", "null" })
@Override
public String toString() {
return MoreObjects.toStringHelper(this)
.omitNullValues()
.add("super", super.toString())
.add("valueStr", valueStr)
.add("valueInt", valueInt)
.add("valueDouble", valueDouble)
.add("businessType", id(businessType))
.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy