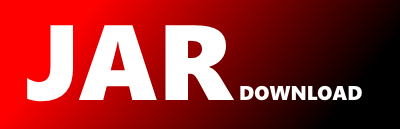
org.jresearch.flexess.client.context.UserContextManager Maven / Gradle / Ivy
package org.jresearch.flexess.client.context;
import java.util.Optional;
import org.jresearch.flexess.umi.api.IUmiUserManager;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* This class is responsible for associating user information with the current
* thread by binding the user context to ThreadLocal object.
*
* @see org.jresearch.flexess.client.context.IUserContext
*
*/
public class UserContextManager {
private final static Logger logger = LoggerFactory.getLogger(UserContextManager.class);
private static InheritableThreadLocal currentInstance = new InheritableThreadLocal<>();
private UserContextManager() {
super();
}
/**
* Binds given UserContext to the current thread.
*
* @param context
* the user context
*/
public static void setContext(final IUserContext context) {
logger.info("Init user context for user {}, thread {}", context == null ? null : context.getUserId(), Thread.currentThread()); //$NON-NLS-1$
currentInstance.set(context);
}
/**
* Retrieves the user context from the current thread. If no context exists
* returns the context for anonymous user
*
* @return the user context
*/
public static IUserContext getContext() {
IUserContext userContext = currentInstance.get();
if (userContext == null) {
setContext(userContext = new UserContext(IUmiUserManager.ANONYMOUS_USER_NAME));
}
return userContext;
}
/**
* Retrieves the user context from the current thread.
*
* @return the user context
*/
public static Optional getContextRav() {
return Optional.ofNullable(currentInstance.get());
}
/**
* Clears current user context (removes binding from the current thread).
*/
public static void clearContext() {
currentInstance.set(null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy