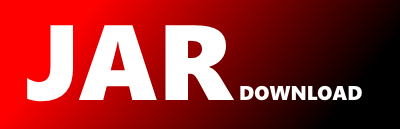
de.saumya.mojo.gems.spec.GemSpecification Maven / Gradle / Ivy
package de.saumya.mojo.gems.spec;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
/**
* Gem::Specification
*
* @author cstamas
*/
public class GemSpecification {
private List authors;
@Deprecated
private String autorequire;
private String bindir;
private List cert_chain;
private Date date;
private String default_executable;
private List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy