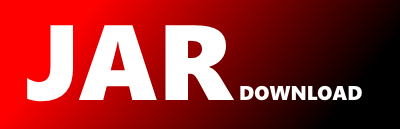
org.jruby.RubyBasicObject Maven / Gradle / Ivy
/***** BEGIN LICENSE BLOCK *****
* Version: EPL 1.0/GPL 2.0/LGPL 2.1
*
* The contents of this file are subject to the Eclipse Public
* License Version 1.0 (the "License"); you may not use this file
* except in compliance with the License. You may obtain a copy of
* the License at http://www.eclipse.org/legal/epl-v10.html
*
* Software distributed under the License is distributed on an "AS
* IS" basis, WITHOUT WARRANTY OF ANY KIND, either express or
* implied. See the License for the specific language governing
* rights and limitations under the License.
*
* Copyright (C) 2008 Thomas E Enebo
*
* Alternatively, the contents of this file may be used under the terms of
* either of the GNU General Public License Version 2 or later (the "GPL"),
* or the GNU Lesser General Public License Version 2.1 or later (the "LGPL"),
* in which case the provisions of the GPL or the LGPL are applicable instead
* of those above. If you wish to allow use of your version of this file only
* under the terms of either the GPL or the LGPL, and not to allow others to
* use your version of this file under the terms of the EPL, indicate your
* decision by deleting the provisions above and replace them with the notice
* and other provisions required by the GPL or the LGPL. If you do not delete
* the provisions above, a recipient may use your version of this file under
* the terms of any one of the EPL, the GPL or the LGPL.
***** END LICENSE BLOCK *****/
package org.jruby;
import org.jruby.runtime.ivars.VariableAccessor;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.atomic.AtomicBoolean;
import org.jruby.anno.JRubyMethod;
import org.jruby.common.IRubyWarnings.ID;
import org.jruby.evaluator.ASTInterpreter;
import org.jruby.exceptions.JumpException;
import org.jruby.internal.runtime.methods.DynamicMethod;
import org.jruby.javasupport.JavaObject;
import org.jruby.javasupport.JavaUtil;
import org.jruby.runtime.Helpers;
import org.jruby.runtime.Block;
import org.jruby.runtime.CallType;
import org.jruby.runtime.ClassIndex;
import org.jruby.runtime.ObjectAllocator;
import org.jruby.runtime.ThreadContext;
import org.jruby.runtime.Visibility;
import static org.jruby.runtime.Visibility.*;
import static org.jruby.CompatVersion.*;
import org.jruby.exceptions.RaiseException;
import org.jruby.runtime.Arity;
import org.jruby.runtime.builtin.IRubyObject;
import org.jruby.runtime.builtin.InstanceVariables;
import org.jruby.runtime.builtin.InternalVariables;
import org.jruby.runtime.builtin.Variable;
import org.jruby.runtime.component.VariableEntry;
import org.jruby.runtime.marshal.CoreObjectType;
import org.jruby.util.IdUtil;
import org.jruby.util.TypeConverter;
import org.jruby.util.log.Logger;
import org.jruby.util.log.LoggerFactory;
import org.jruby.util.unsafe.UnsafeHolder;
import static org.jruby.runtime.Helpers.invokedynamic;
import static org.jruby.runtime.invokedynamic.MethodNames.OP_EQUAL;
import static org.jruby.runtime.invokedynamic.MethodNames.OP_CMP;
import static org.jruby.runtime.invokedynamic.MethodNames.EQL;
import static org.jruby.runtime.invokedynamic.MethodNames.INSPECT;
import org.jruby.runtime.ivars.VariableTableManager;
/**
* RubyBasicObject is the only implementation of the
* {@link org.jruby.runtime.builtin.IRubyObject}. Every Ruby object in JRuby
* is represented by something that is an instance of RubyBasicObject. In
* the core class implementations, this means doing a subclass
* that extends RubyBasicObject. In other cases it means using a simple
* RubyBasicObject instance and its data fields to store specific
* information about the Ruby object.
*
* Some care has been taken to make the implementation be as
* monomorphic as possible, so that the Java Hotspot engine can
* improve performance of it. That is the reason for several patterns
* that might seem odd in this class.
*
* The IRubyObject interface used to have lots of methods for
* different things, but these have now mostly been refactored into
* several interfaces that gives access to that specific part of the
* object. This gives us the possibility to switch out that subsystem
* without changing interfaces again. For example, instance variable
* and internal variables are handled this way, but the implementation
* in RubyObject only returns "this" in {@link #getInstanceVariables()} and
* {@link #getInternalVariables()}.
*
* Methods that are implemented here, such as "initialize" should be implemented
* with care; reification of Ruby classes into Java classes can produce
* conflicting method names in rare cases. See JRUBY-5906 for an example.
*/
public class RubyBasicObject implements Cloneable, IRubyObject, Serializable, Comparable, CoreObjectType, InstanceVariables, InternalVariables {
private static final Logger LOG = LoggerFactory.getLogger("RubyBasicObject");
private static final boolean DEBUG = false;
/** The class of this object */
protected transient RubyClass metaClass;
/** object flags */
protected int flags;
/** variable table, lazily allocated as needed (if needed) */
public transient Object[] varTable;
/** locking stamp for Unsafe ops updating the vartable */
public transient volatile int varTableStamp;
/** offset of the varTable field in RubyBasicObject */
public static final long VAR_TABLE_OFFSET = UnsafeHolder.fieldOffset(RubyBasicObject.class, "varTable");
/** offset of the varTableTamp field in RubyBasicObject */
public static final long STAMP_OFFSET = UnsafeHolder.fieldOffset(RubyBasicObject.class, "varTableStamp");
/**
* The error message used when some one tries to modify an
* instance variable in a high security setting.
*/
public static final String ERR_INSECURE_SET_INST_VAR = "Insecure: can't modify instance variable";
public static final int ALL_F = -1;
public static final int FALSE_F = 1 << 0;
/**
* This flag is a bit funny. It's used to denote that this value
* is nil. It's a bit counterintuitive for a Java programmer to
* not use subclassing to handle this case, since we have a
* RubyNil subclass anyway. Well, the reason for it being a flag
* is that the {@link #isNil()} method is called extremely often. So often
* that it gives a good speed boost to make it monomorphic and
* final. It turns out using a flag for this actually gives us
* better performance than having a polymorphic {@link #isNil()} method.
*/
public static final int NIL_F = 1 << 1;
public static final int FROZEN_F = 1 << 2;
public static final int TAINTED_F = 1 << 3;
public static final int UNTRUSTED_F = 1 << 4;
public static final int FL_USHIFT = 5;
public static final int USER0_F = (1<<(FL_USHIFT+0));
public static final int USER1_F = (1<<(FL_USHIFT+1));
public static final int USER2_F = (1<<(FL_USHIFT+2));
public static final int USER3_F = (1<<(FL_USHIFT+3));
public static final int USER4_F = (1<<(FL_USHIFT+4));
public static final int USER5_F = (1<<(FL_USHIFT+5));
public static final int USER6_F = (1<<(FL_USHIFT+6));
public static final int USER7_F = (1<<(FL_USHIFT+7));
public static final int USER8_F = (1<<(FL_USHIFT+8));
public static final int COMPARE_BY_IDENTITY_F = USER8_F;
/**
* A value that is used as a null sentinel in among other places
* the RubyArray implementation. It will cause large problems to
* call any methods on this object.
*/
public static final IRubyObject NEVER = new RubyBasicObject();
/**
* A value that specifies an undefined value. This value is used
* as a sentinel for undefined constant values, and other places
* where neither null nor NEVER makes sense.
*/
public static final IRubyObject UNDEF = new RubyBasicObject();
/**
* It's not valid to create a totally empty RubyObject. Since the
* RubyObject is always defined in relation to a runtime, that
* means that creating RubyObjects from outside the class might
* cause problems.
*/
private RubyBasicObject(){};
/**
* Default allocator instance for all Ruby objects. The only
* reason to not use this allocator is if you actually need to
* have all instances of something be a subclass of RubyObject.
*
* @see org.jruby.runtime.ObjectAllocator
*/
public static final ObjectAllocator BASICOBJECT_ALLOCATOR = new ObjectAllocator() {
public IRubyObject allocate(Ruby runtime, RubyClass klass) {
return new RubyBasicObject(runtime, klass);
}
};
/**
* Will create the Ruby class Object in the runtime
* specified. This method needs to take the actual class as an
* argument because of the Object class' central part in runtime
* initialization.
*/
public static RubyClass createBasicObjectClass(Ruby runtime, RubyClass objectClass) {
objectClass.index = ClassIndex.OBJECT;
objectClass.defineAnnotatedMethods(RubyBasicObject.class);
recacheBuiltinMethods(runtime);
return objectClass;
}
static void recacheBuiltinMethods(Ruby runtime) {
RubyModule objectClass = runtime.getBasicObject();
if (runtime.is1_9()) { // method_missing is in Kernel in 1.9
runtime.setDefaultMethodMissing(objectClass.searchMethod("method_missing"));
}
}
@JRubyMethod(name = "initialize", visibility = PRIVATE, compat = RUBY1_9)
public IRubyObject initialize19(ThreadContext context) {
return context.nil;
}
/**
* Standard path for object creation. Objects are entered into ObjectSpace
* only if ObjectSpace is enabled.
*/
public RubyBasicObject(Ruby runtime, RubyClass metaClass) {
this.metaClass = metaClass;
runtime.addToObjectSpace(true, this);
}
/**
* Path for objects that don't taint and don't enter objectspace.
*/
public RubyBasicObject(RubyClass metaClass) {
this.metaClass = metaClass;
}
/**
* Path for objects who want to decide whether they don't want to be in
* ObjectSpace even when it is on. (notably used by objects being
* considered immediate, they'll always pass false here)
*/
protected RubyBasicObject(Ruby runtime, RubyClass metaClass, boolean useObjectSpace) {
this.metaClass = metaClass;
runtime.addToObjectSpace(useObjectSpace, this);
}
protected void taint(Ruby runtime) {
if (!isTaint()) {
testFrozen();
setTaint(true);
}
}
/** rb_frozen_class_p
*
* Helper to test whether this object is frozen, and if it is will
* throw an exception based on the message.
*/
protected final void testFrozen(String message) {
if (isFrozen()) {
throw getRuntime().newFrozenError(message);
}
}
/** rb_frozen_class_p
*
* Helper to test whether this object is frozen, and if it is will
* throw an exception based on the message.
*/
protected final void testFrozen() {
if (isFrozen()) {
throw getRuntime().newFrozenError("object");
}
}
/**
* Sets or unsets a flag on this object. The only flags that are
* guaranteed to be valid to use as the first argument is:
*
*
* - {@link #FALSE_F}
* - {@link #NIL_F}
* - {@link #FROZEN_F}
* - {@link #TAINTED_F}
* - {@link #USER0_F}
* - {@link #USER1_F}
* - {@link #USER2_F}
* - {@link #USER3_F}
* - {@link #USER4_F}
* - {@link #USER5_F}
* - {@link #USER6_F}
* - {@link #USER7_F}
*
*
* @param flag the actual flag to set or unset.
* @param set if true, the flag will be set, if false, the flag will be unset.
*/
public final void setFlag(int flag, boolean set) {
if (set) {
flags |= flag;
} else {
flags &= ~flag;
}
}
/**
* Get the value of a custom flag on this object. The only
* guaranteed flags that can be sent in to this method is:
*
*
* - {@link #FALSE_F}
* - {@link #NIL_F}
* - {@link #FROZEN_F}
* - {@link #TAINTED_F}
* - {@link #USER0_F}
* - {@link #USER1_F}
* - {@link #USER2_F}
* - {@link #USER3_F}
* - {@link #USER4_F}
* - {@link #USER5_F}
* - {@link #USER6_F}
* - {@link #USER7_F}
*
*
* @param flag the flag to get
* @return true if the flag is set, false otherwise
*/
public final boolean getFlag(int flag) {
return (flags & flag) != 0;
}
/**
* Will invoke a named method with no arguments and no block if that method or a custom
* method missing exists. Otherwise returns null. 1.9: rb_check_funcall
*/
public final IRubyObject checkCallMethod(ThreadContext context, String name) {
return Helpers.invokeChecked(context, this, name);
}
/**
* Will invoke a named method with no arguments and no block.
*/
public final IRubyObject callMethod(ThreadContext context, String name) {
return Helpers.invoke(context, this, name);
}
/**
* Will invoke a named method with one argument and no block with
* functional invocation.
*/
public final IRubyObject callMethod(ThreadContext context, String name, IRubyObject arg) {
return Helpers.invoke(context, this, name, arg);
}
/**
* Will invoke a named method with the supplied arguments and no
* block with functional invocation.
*/
public final IRubyObject callMethod(ThreadContext context, String name, IRubyObject[] args) {
return Helpers.invoke(context, this, name, args);
}
public final IRubyObject callMethod(String name, IRubyObject... args) {
return Helpers.invoke(getRuntime().getCurrentContext(), this, name, args);
}
public final IRubyObject callMethod(String name) {
return Helpers.invoke(getRuntime().getCurrentContext(), this, name);
}
/**
* Will invoke a named method with the supplied arguments and
* supplied block with functional invocation.
*/
public final IRubyObject callMethod(ThreadContext context, String name, IRubyObject[] args, Block block) {
return Helpers.invoke(context, this, name, args, block);
}
/**
* Does this object represent nil? See the docs for the {@link
* #NIL_F} flag for more information.
*/
public final boolean isNil() {
return (flags & NIL_F) != 0;
}
/**
* Is this value a truthy value or not? Based on the {@link #FALSE_F} flag.
*/
public final boolean isTrue() {
return (flags & FALSE_F) == 0;
}
/**
* Is this value a falsey value or not? Based on the {@link #FALSE_F} flag.
*/
public final boolean isFalse() {
return (flags & FALSE_F) != 0;
}
/**
* Gets the taint. Shortcut for getFlag(TAINTED_F).
*
* @return true if this object is tainted
*/
public boolean isTaint() {
return (flags & TAINTED_F) != 0;
}
/**
* Sets the taint flag. Shortcut for setFlag(TAINTED_F, taint)
*
* @param taint should this object be tainted or not?
*/
public void setTaint(boolean taint) {
// JRUBY-4113: callers should not call setTaint on immediate objects
if (isImmediate()) return;
if (taint) {
flags |= TAINTED_F;
} else {
flags &= ~TAINTED_F;
}
}
/** OBJ_INFECT
*
* Infects this object with traits from the argument obj. In real
* terms this currently means that if obj is tainted, this object
* will get tainted too. It's possible to hijack this method to do
* other infections if that would be interesting.
*/
public IRubyObject infectBy(IRubyObject obj) {
if (obj.isTaint()) setTaint(true);
if (obj.isUntrusted()) setUntrusted(true);
return this;
}
final RubyBasicObject infectBy(RubyBasicObject obj) {
flags |= (obj.flags & (TAINTED_F | UNTRUSTED_F));
return this;
}
final RubyBasicObject infectBy(int tuFlags) {
flags |= (tuFlags & (TAINTED_F | UNTRUSTED_F));
return this;
}
/**
* Is this value frozen or not? Shortcut for doing
* getFlag(FROZEN_F).
*
* @return true if this object is frozen, false otherwise
*/
public boolean isFrozen() {
return (flags & FROZEN_F) != 0;
}
/**
* Sets whether this object is frozen or not. Shortcut for doing
* setFlag(FROZEN_F, frozen).
*
* @param frozen should this object be frozen?
*/
public void setFrozen(boolean frozen) {
if (frozen) {
flags |= FROZEN_F;
} else {
flags &= ~FROZEN_F;
}
}
/**
* Is this value untrusted or not? Shortcut for doing
* getFlag(UNTRUSTED_F).
*
* @return true if this object is frozen, false otherwise
*/
public boolean isUntrusted() {
return (flags & UNTRUSTED_F) != 0;
}
/**
* Sets whether this object is untrusted or not. Shortcut for doing
* setFlag(UNTRUSTED_F, untrusted).
*
* @param untrusted should this object be frozen?
*/
public void setUntrusted(boolean untrusted) {
if (untrusted) {
flags |= UNTRUSTED_F;
} else {
flags &= ~UNTRUSTED_F;
}
}
/**
* Is object immediate (def: Fixnum, Symbol, true, false, nil?).
*/
public boolean isImmediate() {
return false;
}
/**
* if exist return the meta-class else return the type of the object.
*
*/
public final RubyClass getMetaClass() {
return metaClass;
}
/** rb_singleton_class
*
* Note: this method is specialized for RubyFixnum, RubySymbol,
* RubyNil and RubyBoolean
*
* Will either return the existing singleton class for this
* object, or create a new one and return that.
*/
public RubyClass getSingletonClass() {
RubyClass klass;
if (getMetaClass().isSingleton() && ((MetaClass)getMetaClass()).getAttached() == this) {
klass = getMetaClass();
} else {
klass = makeMetaClass(getMetaClass());
}
klass.setTaint(isTaint());
if (isFrozen()) klass.setFrozen(true);
return klass;
}
/** rb_make_metaclass
*
* Will create a new meta class, insert this in the chain of
* classes for this specific object, and return the generated meta
* class.
*/
public RubyClass makeMetaClass(RubyClass superClass) {
MetaClass klass = new MetaClass(getRuntime(), superClass, this); // rb_class_boot
setMetaClass(klass);
klass.setMetaClass(superClass.getRealClass().getMetaClass());
superClass.addSubclass(klass);
return klass;
}
/**
* Makes it possible to change the metaclass of an object. In
* practice, this is a simple version of Smalltalks Become, except
* that it doesn't work when we're dealing with subclasses. In
* practice it's used to change the singleton/meta class used,
* without changing the "real" inheritance chain.
*/
public void setMetaClass(RubyClass metaClass) {
this.metaClass = metaClass;
}
/**
* @see org.jruby.runtime.builtin.IRubyObject#getType()
*/
public RubyClass getType() {
return getMetaClass().getRealClass();
}
/**
* Does this object respond to the specified message? Uses a
* shortcut if it can be proved that respond_to? haven't been
* overridden.
*/
public final boolean respondsTo(String name) {
final Ruby runtime = getRuntime();
if ( runtime.is1_9() ) return respondsTo19(runtime, name);
final DynamicMethod method = getMetaClass().searchMethod("respond_to?");
if ( method.equals(runtime.getRespondToMethod()) ) {
// fastest path; builtin respond_to? which just does isMethodBound
return getMetaClass().isMethodBound(name, false);
}
final RubySymbol mname = runtime.newSymbol(name);
if ( ! method.isUndefined() ) {
// medium path, invoke user's respond_to? if defined
return method.call(runtime.getCurrentContext(), this, metaClass, "respond_to?", mname).isTrue();
}
// slowest path, full callMethod to hit method_missing if present, or produce error
return callMethod(runtime.getCurrentContext(), "respond_to?", mname).isTrue();
}
private boolean respondsTo19(final Ruby runtime, final String name) {
final DynamicMethod respondTo = getMetaClass().searchMethod("respond_to?");
final DynamicMethod respondToMissing = getMetaClass().searchMethod("respond_to_missing?");
if ( respondTo.equals(runtime.getRespondToMethod()) &&
respondToMissing.equals(runtime.getRespondToMissingMethod()) ) {
// fastest path; builtin respond_to? which just does isMethodBound
return getMetaClass().isMethodBound(name, false);
}
final ThreadContext context = runtime.getCurrentContext();
final RubySymbol mname = runtime.newSymbol(name);
final boolean respondToUndefined = respondTo.isUndefined();
if ( ! ( respondToUndefined && respondToMissing.isUndefined() ) ) {
// medium path, invoke user's respond_to?/respond_to_missing? if defined
final DynamicMethod method; final String respondName;
if ( respondToUndefined ) {
method = respondToMissing; respondName = "respond_to_missing?";
} else {
method = respondTo; respondName = "respond_to?";
}
// We have to check and enforce arity
final Arity arity = method.getArity();
if ( arity.isFixed() ) {
if ( arity.required() == 1 ) {
return method.call(context, this, metaClass, respondName, mname).isTrue();
}
//if ( arity.required() != 2 ) {
// throw runtime.newArgumentError(respondName + " must accept 1 or 2 arguments (requires " + arity.getValue() + ")");
//}
}
return method.call(context, this, metaClass, respondName, mname, runtime.getTrue()).isTrue();
}
// slowest path, full callMethod to hit method_missing if present, or produce error
return callMethod(context, "respond_to?", mname).isTrue();
}
/**
* Does this object respond to the specified message via "method_missing?"
*/
public final boolean respondsToMissing(String name) {
return respondsToMissing(name, true);
}
/**
* Does this object respond to the specified message via "method_missing?"
*/
public final boolean respondsToMissing(String name, boolean incPrivate) {
DynamicMethod method = getMetaClass().searchMethod("respond_to_missing?");
// perhaps should try a smart version as for respondsTo above?
if ( method.isUndefined() ) return false;
final Ruby runtime = getRuntime();
return method.call(runtime.getCurrentContext(), this, getMetaClass(),
"respond_to_missing?", runtime.newSymbol(name), runtime.newBoolean(incPrivate)
).isTrue();
}
/**
* Will return the runtime that this object is associated with.
*
* @return current runtime
*/
public final Ruby getRuntime() {
return getMetaClass().getClassRuntime();
}
/**
* Will return the Java interface that most closely can represent
* this object, when working through JAva integration
* translations.
*/
public Class getJavaClass() {
Object obj = dataGetStruct();
if (obj instanceof JavaObject) {
return ((JavaObject)obj).getValue().getClass();
}
return getClass();
}
/** rb_to_id
*
* Will try to convert this object to a String using the Ruby
* "to_str" if the object isn't already a String. If this still
* doesn't work, will throw a Ruby TypeError.
*
*/
public String asJavaString() {
IRubyObject asString = checkStringType();
if(!asString.isNil()) return ((RubyString)asString).asJavaString();
throw getRuntime().newTypeError(inspect().toString() + " is not a string");
}
/** rb_obj_as_string
*
* First converts this object into a String using the "to_s"
* method, infects it with the current taint and returns it. If
* to_s doesn't return a Ruby String, {@link #anyToString} is used
* instead.
*/
public RubyString asString() {
IRubyObject str = Helpers.invoke(getRuntime().getCurrentContext(), this, "to_s");
if (!(str instanceof RubyString)) return (RubyString)anyToString();
if (isTaint()) str.setTaint(true);
return (RubyString) str;
}
/**
* Tries to convert this object to a Ruby Array using the "to_ary"
* method.
*/
public RubyArray convertToArray() {
return (RubyArray) TypeConverter.convertToType(this, getRuntime().getArray(), "to_ary");
}
/**
* Tries to convert this object to a Ruby Hash using the "to_hash"
* method.
*/
public RubyHash convertToHash() {
return (RubyHash)TypeConverter.convertToType(this, getRuntime().getHash(), "to_hash");
}
/**
* Tries to convert this object to a Ruby Float using the "to_f"
* method.
*/
public RubyFloat convertToFloat() {
return (RubyFloat) TypeConverter.convertToType(this, getRuntime().getFloat(), "to_f");
}
/**
* Tries to convert this object to a Ruby Integer using the "to_int"
* method.
*/
public RubyInteger convertToInteger() {
return convertToInteger("to_int");
}
/**
* Tries to convert this object to a Ruby Integer using the
* supplied conversion method.
*/
public RubyInteger convertToInteger(String convertMethod) {
IRubyObject val = TypeConverter.convertToType(this, getRuntime().getInteger(), convertMethod, true);
if (!(val instanceof RubyInteger)) throw getRuntime().newTypeError(getMetaClass().getName() + '#' + convertMethod + " should return Integer");
return (RubyInteger)val;
}
/**
* Tries to convert this object to a Ruby String using the
* "to_str" method.
*/
public RubyString convertToString() {
return (RubyString) TypeConverter.convertToType(this, getRuntime().getString(), "to_str");
}
/**
* Internal method that helps to convert any object into the
* format of a class name and a hex string inside of #<>.
*/
public IRubyObject anyToString() {
String cname = getMetaClass().getRealClass().getName();
/* 6:tags 16:addr 1:eos */
RubyString str = getRuntime().newString("#<" + cname + ":0x" + Integer.toHexString(System.identityHashCode(this)) + '>');
str.setTaint(isTaint());
return str;
}
/** rb_check_string_type
*
* Tries to return a coerced string representation of this object,
* using "to_str". If that returns something other than a String
* or nil, an empty String will be returned.
*
*/
public IRubyObject checkStringType() {
IRubyObject str = TypeConverter.convertToTypeWithCheck(this, getRuntime().getString(), "to_str");
if(!str.isNil() && !(str instanceof RubyString)) {
str = RubyString.newEmptyString(getRuntime());
}
return str;
}
/** rb_check_string_type
*
* Tries to return a coerced string representation of this object,
* using "to_str". If that returns something other than a String
* or nil, an empty String will be returned.
*
*/
public IRubyObject checkStringType19() {
IRubyObject str = TypeConverter.convertToTypeWithCheck19(this, getRuntime().getString(), "to_str");
if(!str.isNil() && !(str instanceof RubyString)) {
str = RubyString.newEmptyString(getRuntime());
}
return str;
}
/** rb_check_array_type
*
* Returns the result of trying to convert this object to an Array
* with "to_ary".
*/
public IRubyObject checkArrayType() {
return TypeConverter.convertToTypeWithCheck(this, getRuntime().getArray(), "to_ary");
}
/**
* @see IRubyObject#toJava
*/
public Object toJava(Class target) {
// for callers that unconditionally pass null retval type (JRUBY-4737)
if (target == void.class) return null;
final Object innerWrapper = dataGetStruct();
if (innerWrapper instanceof JavaObject) {
// for interface impls
final Object value = ((JavaObject) innerWrapper).getValue();
// ensure the object is associated with the wrapper we found it in,
// so that if it comes back we don't re-wrap it
if (target.isAssignableFrom(value.getClass())) {
getRuntime().getJavaSupport().getObjectProxyCache().put(value, this);
return value;
}
}
else if (JavaUtil.isDuckTypeConvertable(getClass(), target)) {
if (!respondsTo("java_object")) {
return JavaUtil.convertProcToInterface(getRuntime().getCurrentContext(), this, target);
}
}
else if (target.isAssignableFrom(getClass())) {
return this;
}
throw getRuntime().newTypeError("cannot convert instance of " + getClass() + " to " + target);
}
public IRubyObject dup() {
Ruby runtime = getRuntime();
if (isImmediate()) throw runtime.newTypeError("can't dup " + getMetaClass().getName());
IRubyObject dup = getMetaClass().getRealClass().allocate();
if (isTaint()) dup.setTaint(true);
if (isUntrusted()) dup.setUntrusted(true);
initCopy(dup, this, runtime.is1_9() ? "initialize_dup" : "initialize_copy");
return dup;
}
/** init_copy
*
* Initializes a copy with variable and special instance variable
* information, and then call the initialize_copy Ruby method.
*/
private static void initCopy(IRubyObject clone, IRubyObject original, String method) {
assert !clone.isFrozen() : "frozen object (" + clone.getMetaClass().getName() + ") allocated";
original.copySpecialInstanceVariables(clone);
if (original.hasVariables()) clone.syncVariables(original);
if (original instanceof RubyModule) {
RubyModule cloneMod = (RubyModule)clone;
cloneMod.syncConstants((RubyModule)original);
cloneMod.syncClassVariables((RubyModule)original);
}
/* FIXME: finalizer should be dupped here */
clone.callMethod(clone.getRuntime().getCurrentContext(), method, original);
}
/**
* Lots of MRI objects keep their state in non-lookupable ivars
* (e:g. Range, Struct, etc). This method is responsible for
* dupping our java field equivalents
*/
public void copySpecialInstanceVariables(IRubyObject clone) {
}
/** rb_inspect
*
* The internal helper that ensures a RubyString instance is returned
* so dangerous casting can be omitted
* Prefered over callMethod(context, "inspect")
*/
static RubyString inspect(ThreadContext context, IRubyObject object) {
return RubyString.objAsString(context, invokedynamic(context, object, INSPECT));
}
public IRubyObject rbClone() {
Ruby runtime = getRuntime();
if (isImmediate()) throw runtime.newTypeError("can't clone " + getMetaClass().getName());
// We're cloning ourselves, so we know the result should be a RubyObject
RubyBasicObject clone = (RubyBasicObject)getMetaClass().getRealClass().allocate();
clone.setMetaClass(getSingletonClassClone());
if (isTaint()) clone.setTaint(true);
initCopy(clone, this, runtime.is1_9() ? "initialize_clone" : "initialize_copy");
if (isFrozen()) clone.setFrozen(true);
if (isUntrusted()) clone.setUntrusted(true);
return clone;
}
/** rb_singleton_class_clone
*
* Will make sure that if the current objects class is a
* singleton, it will get cloned.
*
* @return either a real class, or a clone of the current singleton class
*/
protected RubyClass getSingletonClassClone() {
RubyClass klass = getMetaClass();
if (!klass.isSingleton()) {
return klass;
}
MetaClass clone = new MetaClass(getRuntime(), klass.getSuperClass(), ((MetaClass) klass).getAttached());
clone.flags = flags;
if (this instanceof RubyClass) {
clone.setMetaClass(clone);
} else {
clone.setMetaClass(klass.getSingletonClassClone());
}
if (klass.hasVariables()) {
clone.syncVariables(klass);
}
clone.syncConstants(klass);
klass.cloneMethods(clone);
((MetaClass) clone.getMetaClass()).setAttached(clone);
return clone;
}
/**
* Specifically polymorphic method that are meant to be overridden
* by modules to specify that they are modules in an easy way.
*/
public boolean isModule() {
return false;
}
/**
* Specifically polymorphic method that are meant to be overridden
* by classes to specify that they are classes in an easy way.
*/
public boolean isClass() {
return false;
}
/**
* @see org.jruby.runtime.builtin.IRubyObject#dataWrapStruct(Object)
*/
public synchronized void dataWrapStruct(Object obj) {
if (obj == null) {
removeInternalVariable("__wrap_struct__");
} else {
fastSetInternalVariable("__wrap_struct__", obj);
}
}
// The dataStruct is a place where custom information can be
// contained for core implementations that doesn't necessarily
// want to go to the trouble of creating a subclass of
// RubyObject. The OpenSSL implementation uses this heavily to
// save holder objects containing Java cryptography objects.
// Java integration uses this to store the Java object ref.
//protected transient Object dataStruct;
/**
* @see org.jruby.runtime.builtin.IRubyObject#dataGetStruct()
*/
public synchronized Object dataGetStruct() {
return getInternalVariable("__wrap_struct__");
}
// Equivalent of Data_Get_Struct
// This will first check that the object in question is actually a T_DATA equivalent.
public synchronized Object dataGetStructChecked() {
TypeConverter.checkData(this);
return getInternalVariable("__wrap_struct__");
}
/** rb_obj_id
*
* Return the internal id of an object.
*/
@JRubyMethod(name = {"object_id", "__id__"}, compat = RUBY1_9)
public IRubyObject id() {
return getRuntime().newFixnum(getObjectId());
}
/**
* The logic here is to use the special objectId accessor slot from the
* parent as a lazy store for an object ID. IDs are generated atomically,
* in serial, and guaranteed unique for up to 2^63 objects. The special
* objectId slot is managed separately from the "normal" vars so it
* does not marshal, clone/dup, or refuse to be initially set when the
* object is frozen.
*/
protected long getObjectId() {
return metaClass.getRealClass().getVariableTableManager().getObjectId(this);
}
/** rb_obj_inspect
*
* call-seq:
* obj.inspect => string
*
* Returns a string containing a human-readable representation of
* obj. If not overridden, uses the to_s
method to
* generate the string.
*
* [ 1, 2, 3..4, 'five' ].inspect #=> "[1, 2, 3..4, \"five\"]"
* Time.new.inspect #=> "Wed Apr 09 08:54:39 CDT 2003"
*/
public IRubyObject inspect() {
Ruby runtime = getRuntime();
if ((!isImmediate()) && !(this instanceof RubyModule) && hasVariables()) {
return hashyInspect();
}
if (isNil()) return RubyNil.inspect(this);
return Helpers.invoke(runtime.getCurrentContext(), this, "to_s");
}
public IRubyObject hashyInspect() {
Ruby runtime = getRuntime();
StringBuilder part = new StringBuilder();
String cname = getMetaClass().getRealClass().getName();
part.append("#<").append(cname).append(":0x");
part.append(Integer.toHexString(inspectHashCode()));
if (runtime.isInspecting(this)) {
/* 6:tags 16:addr 1:eos */
part.append(" ...>");
return runtime.newString(part.toString());
}
try {
runtime.registerInspecting(this);
return runtime.newString(inspectObj(part).toString());
} finally {
runtime.unregisterInspecting(this);
}
}
/**
* For most objects, the hash used in the default #inspect is just the
* identity hashcode of the actual object.
*
* See org.jruby.java.proxies.JavaProxy for a divergent case.
*
* @return The identity hashcode of this object
*/
protected int inspectHashCode() {
return System.identityHashCode(this);
}
/** inspect_obj
*
* The internal helper method that takes care of the part of the
* inspection that inspects instance variables.
*/
private StringBuilder inspectObj(StringBuilder part) {
ThreadContext context = getRuntime().getCurrentContext();
String sep = "";
for (Map.Entry entry : metaClass.getVariableTableManager().getVariableAccessorsForRead().entrySet()) {
Object value = entry.getValue().get(this);
if (value == null || !(value instanceof IRubyObject) || !IdUtil.isInstanceVariable(entry.getKey())) continue;
part.append(sep).append(' ').append(entry.getKey()).append('=');
part.append(invokedynamic(context, (IRubyObject)value, INSPECT));
sep = ",";
}
part.append('>');
return part;
}
// Methods of the Object class (rb_obj_*):
@JRubyMethod(name = "!", compat = RUBY1_9)
public IRubyObject op_not(ThreadContext context) {
return context.runtime.newBoolean(!this.isTrue());
}
@JRubyMethod(name = "!=", required = 1, compat = RUBY1_9)
public IRubyObject op_not_equal(ThreadContext context, IRubyObject other) {
return context.runtime.newBoolean(!invokedynamic(context, this, OP_EQUAL, other).isTrue());
}
/**
* Compares this Ruby object with another.
*
* @param other another IRubyObject
* @return 0 if equal,
* < 0 if this is less than other,
* > 0 if this is greater than other
*/
public int compareTo(IRubyObject other) {
final Ruby runtime = getRuntime();
if ( runtime.is1_8() ) return compareTo18(runtime, other);
IRubyObject cmp = invokedynamic(runtime.getCurrentContext(), this, OP_CMP, other);
// if RubyBasicObject#op_cmp is used, the result may be nil (not comparable)
if ( ! cmp.isNil() ) {
return (int) cmp.convertToInteger().getLongValue();
}
/* We used to raise an error if two IRubyObject were not comparable, but
* in order to support the new ConcurrentHashMapV8 and other libraries
* and containers that arbitrarily call compareTo expecting it to always
* succeed, we have opted to return 0 here. This will allow all
* RubyBasicObject subclasses to be compared, but if the comparison is
* not valid we they will appear the same for sorting purposes.
*
* See https://jira.codehaus.org/browse/JRUBY-7013
*/
return 0;
}
// on 1.8 nil (Object in general) is not comparable using <=> by default
private int compareTo18(final Ruby runtime, IRubyObject other) {
final ThreadContext context = runtime.getCurrentContext();
final IRubyObject $ex = context.getErrorInfo();
try {
IRubyObject cmp = invokedynamic(context, this, OP_CMP, other);
if ( ! cmp.isNil() ) {
return (int) cmp.convertToInteger().getLongValue();
}
}
catch (RaiseException e) {
RubyException re = e.getException();
if ( re instanceof RubyNoMethodError ) {
// e.g. NoMethodError: undefined method `<=>' for #
context.setErrorInfo($ex); return 0;
}
throw e;
}
return 0;
}
public IRubyObject op_equal(ThreadContext context, IRubyObject obj) {
return op_equal_19(context, obj);
}
/** rb_obj_equal
*
* Will by default use identity equality to compare objects. This
* follows the Ruby semantics.
*
* The name of this method doesn't follow the convention because hierarchy problems
*/
@JRubyMethod(name = "==", compat = RUBY1_9)
public IRubyObject op_equal_19(ThreadContext context, IRubyObject obj) {
return this == obj ? context.runtime.getTrue() : context.runtime.getFalse();
}
public IRubyObject op_eqq(ThreadContext context, IRubyObject other) {
// Remain unimplemented due to problems with the double java hierarchy
return context.runtime.getNil();
}
@JRubyMethod(name = "equal?", required = 1, compat = RUBY1_9)
public IRubyObject equal_p19(ThreadContext context, IRubyObject other) {
return op_equal_19(context, other);
}
/**
* Helper method for checking equality, first using Java identity
* equality, and then calling the "==" method.
*/
protected static boolean equalInternal(final ThreadContext context, final IRubyObject that, final IRubyObject other){
return that == other || invokedynamic(context, that, OP_EQUAL, other).isTrue();
}
/** method used for Hash key comparison (specialized for String, Symbol and Fixnum)
*
* Will by default just call the Ruby method "eql?"
*/
public boolean eql(IRubyObject other) {
return invokedynamic(getRuntime().getCurrentContext(), this, EQL, other).isTrue();
}
/**
* Adds the specified object as a finalizer for this object.
*/
public void addFinalizer(IRubyObject f) {
Finalizer finalizer = (Finalizer)getInternalVariable("__finalizer__");
if (finalizer == null) {
// since this is the first time we're registering a finalizer, we
// must also register this object in ObjectSpace, so that future
// calls to undefine_finalizer, which takes an object ID, can
// locate the object properly. See JRUBY-4839.
long id = getObjectId();
RubyFixnum fixnumId = (RubyFixnum)id();
getRuntime().getObjectSpace().registerObjectId(id, this);
finalizer = new Finalizer(fixnumId);
fastSetInternalVariable("__finalizer__", finalizer);
getRuntime().addFinalizer(finalizer);
}
finalizer.addFinalizer(f);
}
/**
* Remove all the finalizers for this object.
*/
public void removeFinalizers() {
Finalizer finalizer = (Finalizer)getInternalVariable("__finalizer__");
if (finalizer != null) {
finalizer.removeFinalizers();
removeInternalVariable("__finalizer__");
getRuntime().removeFinalizer(finalizer);
}
}
public Object getVariable(int index) {
return VariableAccessor.getVariable(this, index);
}
public void setVariable(int index, Object value) {
ensureInstanceVariablesSettable();
if (index < 0) return;
metaClass.getVariableTableManager().setVariableInternal(this, index, value);
}
public final Object getNativeHandle() {
return metaClass.getVariableTableManager().getNativeHandle(this);
}
public final void setNativeHandle(Object value) {
metaClass.getVariableTableManager().setNativeHandle(this, value);
}
public final Object getFFIHandle() {
return metaClass.getVariableTableManager().getFFIHandle(this);
}
public final void setFFIHandle(Object value) {
metaClass.getVariableTableManager().setFFIHandle(this, value);
}
//
// COMMON VARIABLE METHODS
//
/**
* Returns true if object has any variables
*
* @see VariableTableManager#hasVariables(org.jruby.RubyBasicObject)
*/
public boolean hasVariables() {
return metaClass.getVariableTableManager().hasVariables(this);
}
/**
* Gets a list of all variables in this object.
*/
// TODO: must override in RubyModule to pick up constants
public List> getVariableList() {
Map ivarAccessors = metaClass.getVariableAccessorsForRead();
ArrayList> list = new ArrayList>();
for (Map.Entry entry : ivarAccessors.entrySet()) {
Object value = entry.getValue().get(this);
if (value == null) continue;
list.add(new VariableEntry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy