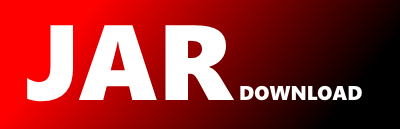
org.jruby.RubyInstanceConfig Maven / Gradle / Ivy
/***** BEGIN LICENSE BLOCK *****
* Version: EPL 1.0/GPL 2.0/LGPL 2.1
*
* The contents of this file are subject to the Eclipse Public
* License Version 1.0 (the "License"); you may not use this file
* except in compliance with the License. You may obtain a copy of
* the License at http://www.eclipse.org/legal/epl-v10.html
*
* Software distributed under the License is distributed on an "AS
* IS" basis, WITHOUT WARRANTY OF ANY KIND, either express or
* implied. See the License for the specific language governing
* rights and limitations under the License.
*
* Copyright (C) 2007-2011 Nick Sieger
* Copyright (C) 2009 Joseph LaFata
*
* Alternatively, the contents of this file may be used under the terms of
* either of the GNU General Public License Version 2 or later (the "GPL"),
* or the GNU Lesser General Public License Version 2.1 or later (the "LGPL"),
* in which case the provisions of the GPL or the LGPL are applicable instead
* of those above. If you wish to allow use of your version of this file only
* under the terms of either the GPL or the LGPL, and not to allow others to
* use your version of this file under the terms of the EPL, indicate your
* decision by deleting the provisions above and replace them with the notice
* and other provisions required by the GPL or the LGPL. If you do not delete
* the provisions above, a recipient may use your version of this file under
* the terms of any one of the EPL, the GPL or the LGPL.
***** END LICENSE BLOCK *****/
package org.jruby;
import jnr.posix.util.Platform;
import org.jruby.ast.executable.Script;
import org.jruby.compiler.ASTCompiler;
import org.jruby.compiler.ASTCompiler19;
import org.jruby.embed.util.SystemPropertyCatcher;
import org.jruby.exceptions.MainExitException;
import org.jruby.runtime.Constants;
import org.jruby.runtime.backtrace.TraceType;
import org.jruby.runtime.load.LoadService;
import org.jruby.runtime.load.LoadService19;
import org.jruby.runtime.profile.builtin.ProfileOutput;
import org.jruby.util.ClassCache;
import org.jruby.util.ClasspathLauncher;
import org.jruby.util.FileResource;
import org.jruby.util.InputStreamMarkCursor;
import org.jruby.util.JRubyFile;
import org.jruby.util.KCode;
import org.jruby.util.SafePropertyAccessor;
import org.jruby.util.URLResource;
import org.jruby.util.cli.ArgumentProcessor;
import org.jruby.util.cli.Options;
import org.jruby.util.cli.OutputStrings;
import org.objectweb.asm.Opcodes;
import java.io.BufferedInputStream;
import java.io.BufferedReader;
import java.io.ByteArrayInputStream;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.PrintStream;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.regex.Pattern;
/**
* A structure used to configure new JRuby instances. All publicly-tweakable
* aspects of Ruby can be modified here, including those settable by command-
* line options, those available through JVM properties, and those suitable for
* embedding.
*/
public class RubyInstanceConfig {
public RubyInstanceConfig() {
currentDirectory = Ruby.isSecurityRestricted() ? "/" : JRubyFile.getFileProperty("user.dir");
String compatString = Options.COMPAT_VERSION.load();
compatVersion = CompatVersion.getVersionFromString(compatString);
if (compatVersion == null) {
error.println("Compatibility version `" + compatString + "' invalid; use 1.8, 1.9, or 2.0. Using default 1.9.");
compatVersion = CompatVersion.RUBY1_9;
}
if (Ruby.isSecurityRestricted()) {
compileMode = CompileMode.OFF;
jitLogging = false;
jitDumping = false;
jitLoggingVerbose = false;
jitLogEvery = 0;
jitThreshold = -1;
jitMax = 0;
jitMaxSize = -1;
managementEnabled = false;
} else {
if (COMPILE_EXCLUDE != null) {
String[] elements = COMPILE_EXCLUDE.split(",");
excludedMethods.addAll(Arrays.asList(elements));
}
managementEnabled = Options.MANAGEMENT_ENABLED.load();
runRubyInProcess = Options.LAUNCH_INPROC.load();
String jitModeProperty = Options.COMPILE_MODE.load();
if (jitModeProperty.equals("OFF")) {
compileMode = CompileMode.OFF;
} else if (jitModeProperty.equals("OFFIR")) {
compileMode = CompileMode.OFFIR;
} else if (jitModeProperty.equals("JIT")) {
compileMode = CompileMode.JIT;
} else if (jitModeProperty.equals("FORCE")) {
compileMode = CompileMode.FORCE;
} else {
error.print(Options.COMPILE_MODE + " property must be OFF, JIT, FORCE, or unset; defaulting to JIT");
compileMode = CompileMode.JIT;
}
jitLogging = Options.JIT_LOGGING.load();
jitDumping = Options.JIT_DUMPING.load();
jitLoggingVerbose = Options.JIT_LOGGING_VERBOSE.load();
jitLogEvery = Options.JIT_LOGEVERY.load();
jitThreshold = Options.JIT_THRESHOLD.load();
jitMax = Options.JIT_MAX.load();
jitMaxSize = Options.JIT_MAXSIZE.load();
}
// default ClassCache using jitMax as a soft upper bound
classCache = new ClassCache