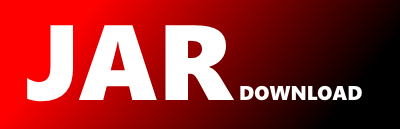
org.jruby.ext.etc.RubyEtc Maven / Gradle / Ivy
package org.jruby.ext.etc;
import java.util.concurrent.atomic.AtomicBoolean;
import org.jruby.anno.JRubyMethod;
import org.jruby.anno.JRubyModule;
import org.jruby.common.IRubyWarnings.ID;
import org.jruby.exceptions.RaiseException;
import jnr.posix.Passwd;
import jnr.posix.Group;
import jnr.posix.POSIX;
import jnr.posix.util.Platform;
import org.jruby.CompatVersion;
import org.jruby.Ruby;
import org.jruby.RubyModule;
import org.jruby.RubyNumeric;
import org.jruby.RubyString;
import org.jruby.RubyStruct;
import org.jruby.ext.rbconfig.RbConfigLibrary;
import org.jruby.runtime.Block;
import org.jruby.runtime.ThreadContext;
import org.jruby.runtime.builtin.IRubyObject;
import org.jruby.util.ByteList;
import org.jruby.util.NormalizedFile;
@JRubyModule(name="Etc")
public class RubyEtc {
public static RubyModule createEtcModule(Ruby runtime) {
RubyModule etcModule = runtime.defineModule("Etc");
runtime.setEtc(etcModule);
etcModule.defineAnnotatedMethods(RubyEtc.class);
definePasswdStruct(runtime);
defineGroupStruct(runtime);
return etcModule;
}
private static void definePasswdStruct(Ruby runtime) {
IRubyObject[] args = new IRubyObject[] {
runtime.newString("Passwd"),
runtime.newSymbol("name"),
runtime.newSymbol("passwd"),
runtime.newSymbol("uid"),
runtime.newSymbol("gid"),
runtime.newSymbol("gecos"),
runtime.newSymbol("dir"),
runtime.newSymbol("shell"),
runtime.newSymbol("change"),
runtime.newSymbol("uclass"),
runtime.newSymbol("expire")
};
runtime.setPasswdStruct(RubyStruct.newInstance(runtime.getStructClass(), args, Block.NULL_BLOCK));
if (runtime.is1_9()) {
runtime.getEtc().defineConstant("Passwd", runtime.getPasswdStruct());
}
}
private static void defineGroupStruct(Ruby runtime) {
IRubyObject[] args = new IRubyObject[] {
runtime.newString("Group"),
runtime.newSymbol("name"),
runtime.newSymbol("passwd"),
runtime.newSymbol("gid"),
runtime.newSymbol("mem")
};
runtime.setGroupStruct(RubyStruct.newInstance(runtime.getStructClass(), args, Block.NULL_BLOCK));
if (runtime.is1_9()) {
runtime.getEtc().defineConstant("Group", runtime.getGroupStruct());
}
}
private static IRubyObject setupPasswd(Ruby runtime, Passwd passwd) {
IRubyObject[] args = new IRubyObject[] {
runtime.newString(passwd.getLoginName()),
runtime.newString(passwd.getPassword()),
runtime.newFixnum(passwd.getUID()),
runtime.newFixnum(passwd.getGID()),
runtime.newString(passwd.getGECOS()),
runtime.newString(passwd.getHome()),
runtime.newString(passwd.getShell()),
runtime.newFixnum(passwd.getPasswdChangeTime()),
runtime.newString(passwd.getAccessClass()),
runtime.newFixnum(passwd.getExpire())
};
return RubyStruct.newStruct(runtime.getPasswdStruct(), args, Block.NULL_BLOCK);
}
private static IRubyObject setupGroup(Ruby runtime, Group group) {
IRubyObject[] args = new IRubyObject[] {
runtime.newString(group.getName()),
runtime.newString(group.getPassword()),
runtime.newFixnum(group.getGID()),
intoStringArray(runtime, group.getMembers())
};
return RubyStruct.newStruct(runtime.getGroupStruct(), args, Block.NULL_BLOCK);
}
private static IRubyObject intoStringArray(Ruby runtime, String[] members) {
IRubyObject[] arr = new IRubyObject[members.length];
for(int i = 0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy