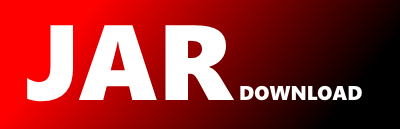
org.jruby.runtime.profile.builtin.HtmlProfilePrinter Maven / Gradle / Ivy
/***** BEGIN LICENSE BLOCK *****
* Version: EPL 1.0/GPL 2.0/LGPL 2.1
*
* The contents of this file are subject to the Eclipse Public
* License Version 1.0 (the "License"); you may not use this file
* except in compliance with the License. You may obtain a copy of
* the License at http://www.eclipse.org/legal/epl-v10.html
*
* Software distributed under the License is distributed on an "AS
* IS" basis, WITHOUT WARRANTY OF ANY KIND, either express or
* implied. See the License for the specific language governing
* rights and limitations under the License.
*
* Alternatively, the contents of this file may be used under the terms of
* either of the GNU General Public License Version 2 or later (the "GPL"),
* or the GNU Lesser General Public License Version 2.1 or later (the "LGPL"),
* in which case the provisions of the GPL or the LGPL are applicable instead
* of those above. If you wish to allow use of your version of this file only
* under the terms of either the GPL or the LGPL, and not to allow others to
* use your version of this file under the terms of the EPL, indicate your
* decision by deleting the provisions above and replace them with the notice
* and other provisions required by the GPL or the LGPL. If you do not delete
* the provisions above, a recipient may use your version of this file under
* the terms of any one of the EPL, the GPL or the LGPL.
***** END LICENSE BLOCK *****/
package org.jruby.runtime.profile.builtin;
import org.jruby.util.collections.IntHashMap;
import java.io.PrintStream;
import java.util.Arrays;
import java.util.Comparator;
public class HtmlProfilePrinter extends ProfilePrinter {
private static final long LIMIT = 100000000;
public HtmlProfilePrinter(ProfileData profileData) {
super(profileData);
}
HtmlProfilePrinter(ProfileData profileData, Invocation topInvocation) {
super(profileData, topInvocation);
}
public void printHeader(PrintStream out) {
out.println(head);
out.println("");
}
public void printFooter(PrintStream out) {
out.println("");
out.println("