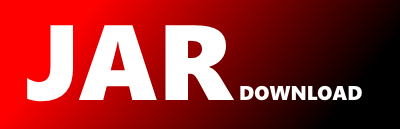
org.jruby.truffle.cext.CExtNodesFactory Maven / Gradle / Ivy
The newest version!
// CheckStyle: start generated
package org.jruby.truffle.cext;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NodeFactory;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.internal.SpecializationNode;
import com.oracle.truffle.api.dsl.internal.SpecializedNode;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.MaterializedFrame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.nodes.UnexpectedResultException;
import com.oracle.truffle.api.object.DynamicObject;
import com.oracle.truffle.api.profiles.ConditionProfile;
import java.util.Arrays;
import java.util.List;
import org.jruby.truffle.builtins.CoreMethodNode;
import org.jruby.truffle.cext.CExtNodes.AdaptRDataNode;
import org.jruby.truffle.cext.CExtNodes.BlockGivenNode;
import org.jruby.truffle.cext.CExtNodes.CLASSOFNode;
import org.jruby.truffle.cext.CExtNodes.CallerFrameVisibilityNode;
import org.jruby.truffle.cext.CExtNodes.CextModuleFunctionNode;
import org.jruby.truffle.cext.CExtNodes.ConstGetFromNode;
import org.jruby.truffle.cext.CExtNodes.FIX2INTNode;
import org.jruby.truffle.cext.CExtNodes.FIX2LONGNode;
import org.jruby.truffle.cext.CExtNodes.FIX2UINTNode;
import org.jruby.truffle.cext.CExtNodes.FromNativeHandleNode;
import org.jruby.truffle.cext.CExtNodes.GetBlockNode;
import org.jruby.truffle.cext.CExtNodes.INT2FIXNode;
import org.jruby.truffle.cext.CExtNodes.INT2NUMNode;
import org.jruby.truffle.cext.CExtNodes.IOHandleNode;
import org.jruby.truffle.cext.CExtNodes.LONG2FIXNode;
import org.jruby.truffle.cext.CExtNodes.LONG2NUMNode;
import org.jruby.truffle.cext.CExtNodes.Long2Int;
import org.jruby.truffle.cext.CExtNodes.NUM2DBLNode;
import org.jruby.truffle.cext.CExtNodes.NUM2INTNode;
import org.jruby.truffle.cext.CExtNodes.NUM2LONGNode;
import org.jruby.truffle.cext.CExtNodes.NUM2UINTNode;
import org.jruby.truffle.cext.CExtNodes.NUM2ULONGNode;
import org.jruby.truffle.cext.CExtNodes.StringPointerNode;
import org.jruby.truffle.cext.CExtNodes.ToNativeHandleNode;
import org.jruby.truffle.cext.CExtNodes.ToRubyStringNode;
import org.jruby.truffle.cext.CExtNodes.UINT2NUMNode;
import org.jruby.truffle.cext.CExtNodes.ULONG2NUMNode;
import org.jruby.truffle.language.NotProvided;
import org.jruby.truffle.language.RubyGuards;
import org.jruby.truffle.language.RubyNode;
import org.jruby.truffle.language.RubyTypes;
import org.jruby.truffle.language.RubyTypesGen;
import org.jruby.truffle.language.objects.MetaClassNode;
@GeneratedBy(CExtNodes.class)
public final class CExtNodesFactory {
public static List> getFactories() {
return Arrays.asList(NUM2INTNodeFactory.getInstance(), NUM2UINTNodeFactory.getInstance(), NUM2LONGNodeFactory.getInstance(), NUM2ULONGNodeFactory.getInstance(), NUM2DBLNodeFactory.getInstance(), FIX2INTNodeFactory.getInstance(), FIX2UINTNodeFactory.getInstance(), FIX2LONGNodeFactory.getInstance(), INT2NUMNodeFactory.getInstance(), INT2FIXNodeFactory.getInstance(), UINT2NUMNodeFactory.getInstance(), LONG2NUMNodeFactory.getInstance(), ULONG2NUMNodeFactory.getInstance(), LONG2FIXNodeFactory.getInstance(), CLASSOFNodeFactory.getInstance(), Long2IntFactory.getInstance(), StringPointerNodeFactory.getInstance(), ToRubyStringNodeFactory.getInstance(), BlockGivenNodeFactory.getInstance(), GetBlockNodeFactory.getInstance(), ConstGetFromNodeFactory.getInstance(), IOHandleNodeFactory.getInstance(), CextModuleFunctionNodeFactory.getInstance(), CallerFrameVisibilityNodeFactory.getInstance(), AdaptRDataNodeFactory.getInstance(), ToNativeHandleNodeFactory.getInstance(), FromNativeHandleNodeFactory.getInstance());
}
@GeneratedBy(NUM2INTNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class NUM2INTNodeFactory implements NodeFactory {
private static NUM2INTNodeFactory nUM2INTNodeFactoryInstance;
private NUM2INTNodeFactory() {
}
@Override
public Class getNodeClass() {
return NUM2INTNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public NUM2INTNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (nUM2INTNodeFactoryInstance == null) {
nUM2INTNodeFactoryInstance = new NUM2INTNodeFactory();
}
return nUM2INTNodeFactoryInstance;
}
public static NUM2INTNode create(RubyNode[] arguments) {
return new NUM2INTNodeGen(arguments);
}
@GeneratedBy(NUM2INTNode.class)
public static final class NUM2INTNodeGen extends NUM2INTNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@CompilationFinal private Class> arguments0Type_;
@Child private BaseNode_ specialization_;
private NUM2INTNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public int executeInteger(VirtualFrame frameValue) {
return specialization_.executeInt(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(NUM2INTNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected NUM2INTNodeGen root;
BaseNode_(NUM2INTNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (NUM2INTNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.executeInt_((VirtualFrame) frameValue, arguments0Value);
}
public abstract int executeInt_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return executeInt_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public int executeInt(VirtualFrame frameValue) {
return (int) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value)) {
return Num2int0Node_.create(root, arguments0Value);
}
if (RubyTypesGen.isImplicitLong(arguments0Value)) {
return Num2int1Node_.create(root, arguments0Value);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments0_(Frame frameValue) {
Class> arguments0Type_ = root.arguments0Type_;
try {
if (arguments0Type_ == int.class) {
return root.arguments0_.executeInteger((VirtualFrame) frameValue);
} else if (arguments0Type_ == long.class) {
return root.arguments0_.executeLong((VirtualFrame) frameValue);
} else if (arguments0Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments0_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else if (_value instanceof Long) {
_type = long.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments0Type_ = _type;
}
} else {
return root.arguments0_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments0Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(NUM2INTNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(NUM2INTNodeGen root) {
super(root, 2147483647);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value) {
return (int) uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(NUM2INTNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(NUM2INTNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(NUM2INTNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public int executeInt(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return getNext().executeInt_(frameValue, arguments0Value_);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().executeInt_(frameValue, arguments0Value);
}
static BaseNode_ create(NUM2INTNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "num2int(int)", value = NUM2INTNode.class)
private static final class Num2int0Node_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
Num2int0Node_(NUM2INTNodeGen root, Object arguments0Value) {
super(root, 1);
this.arguments0ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((Num2int0Node_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeInt(frameValue);
}
@Override
public int executeInt(VirtualFrame frameValue) {
int arguments0Value_;
try {
if (arguments0ImplicitType == int.class) {
arguments0Value_ = root.arguments0_.executeInteger(frameValue);
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitInteger(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeInt_(frameValue, ex.getResult());
}
return root.num2int(arguments0Value_);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value, arguments0ImplicitType)) {
int arguments0Value_ = RubyTypesGen.asImplicitInteger(arguments0Value, arguments0ImplicitType);
return root.num2int(arguments0Value_);
}
return getNext().executeInt_(frameValue, arguments0Value);
}
static BaseNode_ create(NUM2INTNodeGen root, Object arguments0Value) {
return new Num2int0Node_(root, arguments0Value);
}
}
@GeneratedBy(methodName = "num2int(long)", value = NUM2INTNode.class)
private static final class Num2int1Node_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
Num2int1Node_(NUM2INTNodeGen root, Object arguments0Value) {
super(root, 2);
this.arguments0ImplicitType = RubyTypesGen.getImplicitLongClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((Num2int1Node_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeInt(frameValue);
}
@Override
public int executeInt(VirtualFrame frameValue) {
long arguments0Value_;
try {
if (arguments0ImplicitType == long.class) {
arguments0Value_ = root.arguments0_.executeLong(frameValue);
} else if (arguments0ImplicitType == int.class) {
arguments0Value_ = RubyTypes.promoteToLong(root.arguments0_.executeInteger(frameValue));
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitLong(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeInt_(frameValue, ex.getResult());
}
return root.num2int(arguments0Value_);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitLong(arguments0Value, arguments0ImplicitType)) {
long arguments0Value_ = RubyTypesGen.asImplicitLong(arguments0Value, arguments0ImplicitType);
return root.num2int(arguments0Value_);
}
return getNext().executeInt_(frameValue, arguments0Value);
}
static BaseNode_ create(NUM2INTNodeGen root, Object arguments0Value) {
return new Num2int1Node_(root, arguments0Value);
}
}
}
}
@GeneratedBy(NUM2UINTNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class NUM2UINTNodeFactory implements NodeFactory {
private static NUM2UINTNodeFactory nUM2UINTNodeFactoryInstance;
private NUM2UINTNodeFactory() {
}
@Override
public Class getNodeClass() {
return NUM2UINTNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public NUM2UINTNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (nUM2UINTNodeFactoryInstance == null) {
nUM2UINTNodeFactoryInstance = new NUM2UINTNodeFactory();
}
return nUM2UINTNodeFactoryInstance;
}
public static NUM2UINTNode create(RubyNode[] arguments) {
return new NUM2UINTNodeGen(arguments);
}
@GeneratedBy(NUM2UINTNode.class)
public static final class NUM2UINTNodeGen extends NUM2UINTNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@CompilationFinal private Class> arguments0Type_;
@Child private BaseNode_ specialization_;
private NUM2UINTNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public int executeInteger(VirtualFrame frameValue) {
return specialization_.executeInt(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(NUM2UINTNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected NUM2UINTNodeGen root;
BaseNode_(NUM2UINTNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (NUM2UINTNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.executeInt_((VirtualFrame) frameValue, arguments0Value);
}
public abstract int executeInt_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return executeInt_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public int executeInt(VirtualFrame frameValue) {
return (int) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value)) {
return Num2uintNode_.create(root, arguments0Value);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments0_(Frame frameValue) {
Class> arguments0Type_ = root.arguments0Type_;
try {
if (arguments0Type_ == int.class) {
return root.arguments0_.executeInteger((VirtualFrame) frameValue);
} else if (arguments0Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments0_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments0Type_ = _type;
}
} else {
return root.arguments0_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments0Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(NUM2UINTNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(NUM2UINTNodeGen root) {
super(root, 2147483647);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value) {
return (int) uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(NUM2UINTNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(NUM2UINTNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(NUM2UINTNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public int executeInt(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return getNext().executeInt_(frameValue, arguments0Value_);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().executeInt_(frameValue, arguments0Value);
}
static BaseNode_ create(NUM2UINTNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "num2uint(int)", value = NUM2UINTNode.class)
private static final class Num2uintNode_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
Num2uintNode_(NUM2UINTNodeGen root, Object arguments0Value) {
super(root, 1);
this.arguments0ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((Num2uintNode_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeInt(frameValue);
}
@Override
public int executeInt(VirtualFrame frameValue) {
int arguments0Value_;
try {
if (arguments0ImplicitType == int.class) {
arguments0Value_ = root.arguments0_.executeInteger(frameValue);
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitInteger(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeInt_(frameValue, ex.getResult());
}
return root.num2uint(arguments0Value_);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value, arguments0ImplicitType)) {
int arguments0Value_ = RubyTypesGen.asImplicitInteger(arguments0Value, arguments0ImplicitType);
return root.num2uint(arguments0Value_);
}
return getNext().executeInt_(frameValue, arguments0Value);
}
static BaseNode_ create(NUM2UINTNodeGen root, Object arguments0Value) {
return new Num2uintNode_(root, arguments0Value);
}
}
}
}
@GeneratedBy(NUM2LONGNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class NUM2LONGNodeFactory implements NodeFactory {
private static NUM2LONGNodeFactory nUM2LONGNodeFactoryInstance;
private NUM2LONGNodeFactory() {
}
@Override
public Class getNodeClass() {
return NUM2LONGNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public NUM2LONGNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (nUM2LONGNodeFactoryInstance == null) {
nUM2LONGNodeFactoryInstance = new NUM2LONGNodeFactory();
}
return nUM2LONGNodeFactoryInstance;
}
public static NUM2LONGNode create(RubyNode[] arguments) {
return new NUM2LONGNodeGen(arguments);
}
@GeneratedBy(NUM2LONGNode.class)
public static final class NUM2LONGNodeGen extends NUM2LONGNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@CompilationFinal private Class> arguments0Type_;
@Child private BaseNode_ specialization_;
private NUM2LONGNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public long executeLong(VirtualFrame frameValue) {
return specialization_.executeLong(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(NUM2LONGNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected NUM2LONGNodeGen root;
BaseNode_(NUM2LONGNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (NUM2LONGNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.executeLong_((VirtualFrame) frameValue, arguments0Value);
}
public abstract long executeLong_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return executeLong_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public long executeLong(VirtualFrame frameValue) {
return (long) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value)) {
return Num2long0Node_.create(root, arguments0Value);
}
if (RubyTypesGen.isImplicitLong(arguments0Value)) {
return Num2long1Node_.create(root, arguments0Value);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments0_(Frame frameValue) {
Class> arguments0Type_ = root.arguments0Type_;
try {
if (arguments0Type_ == int.class) {
return root.arguments0_.executeInteger((VirtualFrame) frameValue);
} else if (arguments0Type_ == long.class) {
return root.arguments0_.executeLong((VirtualFrame) frameValue);
} else if (arguments0Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments0_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else if (_value instanceof Long) {
_type = long.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments0Type_ = _type;
}
} else {
return root.arguments0_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments0Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(NUM2LONGNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(NUM2LONGNodeGen root) {
super(root, 2147483647);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value) {
return (long) uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(NUM2LONGNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(NUM2LONGNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(NUM2LONGNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public long executeLong(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return getNext().executeLong_(frameValue, arguments0Value_);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().executeLong_(frameValue, arguments0Value);
}
static BaseNode_ create(NUM2LONGNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "num2long(int)", value = NUM2LONGNode.class)
private static final class Num2long0Node_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
Num2long0Node_(NUM2LONGNodeGen root, Object arguments0Value) {
super(root, 1);
this.arguments0ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((Num2long0Node_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeLong(frameValue);
}
@Override
public long executeLong(VirtualFrame frameValue) {
int arguments0Value_;
try {
if (arguments0ImplicitType == int.class) {
arguments0Value_ = root.arguments0_.executeInteger(frameValue);
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitInteger(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeLong_(frameValue, ex.getResult());
}
return root.num2long(arguments0Value_);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value, arguments0ImplicitType)) {
int arguments0Value_ = RubyTypesGen.asImplicitInteger(arguments0Value, arguments0ImplicitType);
return root.num2long(arguments0Value_);
}
return getNext().executeLong_(frameValue, arguments0Value);
}
static BaseNode_ create(NUM2LONGNodeGen root, Object arguments0Value) {
return new Num2long0Node_(root, arguments0Value);
}
}
@GeneratedBy(methodName = "num2long(long)", value = NUM2LONGNode.class)
private static final class Num2long1Node_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
Num2long1Node_(NUM2LONGNodeGen root, Object arguments0Value) {
super(root, 2);
this.arguments0ImplicitType = RubyTypesGen.getImplicitLongClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((Num2long1Node_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeLong(frameValue);
}
@Override
public long executeLong(VirtualFrame frameValue) {
long arguments0Value_;
try {
if (arguments0ImplicitType == long.class) {
arguments0Value_ = root.arguments0_.executeLong(frameValue);
} else if (arguments0ImplicitType == int.class) {
arguments0Value_ = RubyTypes.promoteToLong(root.arguments0_.executeInteger(frameValue));
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitLong(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeLong_(frameValue, ex.getResult());
}
return root.num2long(arguments0Value_);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitLong(arguments0Value, arguments0ImplicitType)) {
long arguments0Value_ = RubyTypesGen.asImplicitLong(arguments0Value, arguments0ImplicitType);
return root.num2long(arguments0Value_);
}
return getNext().executeLong_(frameValue, arguments0Value);
}
static BaseNode_ create(NUM2LONGNodeGen root, Object arguments0Value) {
return new Num2long1Node_(root, arguments0Value);
}
}
}
}
@GeneratedBy(NUM2ULONGNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class NUM2ULONGNodeFactory implements NodeFactory {
private static NUM2ULONGNodeFactory nUM2ULONGNodeFactoryInstance;
private NUM2ULONGNodeFactory() {
}
@Override
public Class getNodeClass() {
return NUM2ULONGNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public NUM2ULONGNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (nUM2ULONGNodeFactoryInstance == null) {
nUM2ULONGNodeFactoryInstance = new NUM2ULONGNodeFactory();
}
return nUM2ULONGNodeFactoryInstance;
}
public static NUM2ULONGNode create(RubyNode[] arguments) {
return new NUM2ULONGNodeGen(arguments);
}
@GeneratedBy(NUM2ULONGNode.class)
public static final class NUM2ULONGNodeGen extends NUM2ULONGNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@CompilationFinal private Class> arguments0Type_;
@Child private BaseNode_ specialization_;
private NUM2ULONGNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public long executeLong(VirtualFrame frameValue) {
return specialization_.executeLong(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(NUM2ULONGNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected NUM2ULONGNodeGen root;
BaseNode_(NUM2ULONGNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (NUM2ULONGNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.executeLong_((VirtualFrame) frameValue, arguments0Value);
}
public abstract long executeLong_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return executeLong_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public long executeLong(VirtualFrame frameValue) {
return (long) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value)) {
return Num2ulongNode_.create(root, arguments0Value);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments0_(Frame frameValue) {
Class> arguments0Type_ = root.arguments0Type_;
try {
if (arguments0Type_ == int.class) {
return root.arguments0_.executeInteger((VirtualFrame) frameValue);
} else if (arguments0Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments0_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments0Type_ = _type;
}
} else {
return root.arguments0_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments0Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(NUM2ULONGNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(NUM2ULONGNodeGen root) {
super(root, 2147483647);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value) {
return (long) uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(NUM2ULONGNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(NUM2ULONGNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(NUM2ULONGNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public long executeLong(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return getNext().executeLong_(frameValue, arguments0Value_);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().executeLong_(frameValue, arguments0Value);
}
static BaseNode_ create(NUM2ULONGNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "num2ulong(int)", value = NUM2ULONGNode.class)
private static final class Num2ulongNode_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
Num2ulongNode_(NUM2ULONGNodeGen root, Object arguments0Value) {
super(root, 1);
this.arguments0ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((Num2ulongNode_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeLong(frameValue);
}
@Override
public long executeLong(VirtualFrame frameValue) {
int arguments0Value_;
try {
if (arguments0ImplicitType == int.class) {
arguments0Value_ = root.arguments0_.executeInteger(frameValue);
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitInteger(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeLong_(frameValue, ex.getResult());
}
return root.num2ulong(arguments0Value_);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value, arguments0ImplicitType)) {
int arguments0Value_ = RubyTypesGen.asImplicitInteger(arguments0Value, arguments0ImplicitType);
return root.num2ulong(arguments0Value_);
}
return getNext().executeLong_(frameValue, arguments0Value);
}
static BaseNode_ create(NUM2ULONGNodeGen root, Object arguments0Value) {
return new Num2ulongNode_(root, arguments0Value);
}
}
}
}
@GeneratedBy(NUM2DBLNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class NUM2DBLNodeFactory implements NodeFactory {
private static NUM2DBLNodeFactory nUM2DBLNodeFactoryInstance;
private NUM2DBLNodeFactory() {
}
@Override
public Class getNodeClass() {
return NUM2DBLNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public NUM2DBLNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (nUM2DBLNodeFactoryInstance == null) {
nUM2DBLNodeFactoryInstance = new NUM2DBLNodeFactory();
}
return nUM2DBLNodeFactoryInstance;
}
public static NUM2DBLNode create(RubyNode[] arguments) {
return new NUM2DBLNodeGen(arguments);
}
@GeneratedBy(NUM2DBLNode.class)
public static final class NUM2DBLNodeGen extends NUM2DBLNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@CompilationFinal private Class> arguments0Type_;
@Child private BaseNode_ specialization_;
private NUM2DBLNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public double executeDouble(VirtualFrame frameValue) {
return specialization_.executeDouble(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(NUM2DBLNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected NUM2DBLNodeGen root;
BaseNode_(NUM2DBLNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (NUM2DBLNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.executeDouble_((VirtualFrame) frameValue, arguments0Value);
}
public abstract double executeDouble_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return executeDouble_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public double executeDouble(VirtualFrame frameValue) {
return (double) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value)) {
return Num2dblNode_.create(root, arguments0Value);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments0_(Frame frameValue) {
Class> arguments0Type_ = root.arguments0Type_;
try {
if (arguments0Type_ == int.class) {
return root.arguments0_.executeInteger((VirtualFrame) frameValue);
} else if (arguments0Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments0_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments0Type_ = _type;
}
} else {
return root.arguments0_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments0Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(NUM2DBLNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(NUM2DBLNodeGen root) {
super(root, 2147483647);
}
@Override
public double executeDouble_(VirtualFrame frameValue, Object arguments0Value) {
return (double) uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(NUM2DBLNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(NUM2DBLNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(NUM2DBLNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public double executeDouble(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return getNext().executeDouble_(frameValue, arguments0Value_);
}
@Override
public double executeDouble_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().executeDouble_(frameValue, arguments0Value);
}
static BaseNode_ create(NUM2DBLNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "num2dbl(int)", value = NUM2DBLNode.class)
private static final class Num2dblNode_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
Num2dblNode_(NUM2DBLNodeGen root, Object arguments0Value) {
super(root, 1);
this.arguments0ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((Num2dblNode_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDouble(frameValue);
}
@Override
public double executeDouble(VirtualFrame frameValue) {
int arguments0Value_;
try {
if (arguments0ImplicitType == int.class) {
arguments0Value_ = root.arguments0_.executeInteger(frameValue);
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitInteger(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeDouble_(frameValue, ex.getResult());
}
return root.num2dbl(arguments0Value_);
}
@Override
public double executeDouble_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value, arguments0ImplicitType)) {
int arguments0Value_ = RubyTypesGen.asImplicitInteger(arguments0Value, arguments0ImplicitType);
return root.num2dbl(arguments0Value_);
}
return getNext().executeDouble_(frameValue, arguments0Value);
}
static BaseNode_ create(NUM2DBLNodeGen root, Object arguments0Value) {
return new Num2dblNode_(root, arguments0Value);
}
}
}
}
@GeneratedBy(FIX2INTNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class FIX2INTNodeFactory implements NodeFactory {
private static FIX2INTNodeFactory fIX2INTNodeFactoryInstance;
private FIX2INTNodeFactory() {
}
@Override
public Class getNodeClass() {
return FIX2INTNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public FIX2INTNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (fIX2INTNodeFactoryInstance == null) {
fIX2INTNodeFactoryInstance = new FIX2INTNodeFactory();
}
return fIX2INTNodeFactoryInstance;
}
public static FIX2INTNode create(RubyNode[] arguments) {
return new FIX2INTNodeGen(arguments);
}
@GeneratedBy(FIX2INTNode.class)
public static final class FIX2INTNodeGen extends FIX2INTNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@CompilationFinal private Class> arguments0Type_;
@Child private BaseNode_ specialization_;
private FIX2INTNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public int executeInteger(VirtualFrame frameValue) {
return specialization_.executeInt(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(FIX2INTNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected FIX2INTNodeGen root;
BaseNode_(FIX2INTNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (FIX2INTNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.executeInt_((VirtualFrame) frameValue, arguments0Value);
}
public abstract int executeInt_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return executeInt_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public int executeInt(VirtualFrame frameValue) {
return (int) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value)) {
return Fix2intNode_.create(root, arguments0Value);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments0_(Frame frameValue) {
Class> arguments0Type_ = root.arguments0Type_;
try {
if (arguments0Type_ == int.class) {
return root.arguments0_.executeInteger((VirtualFrame) frameValue);
} else if (arguments0Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments0_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments0Type_ = _type;
}
} else {
return root.arguments0_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments0Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(FIX2INTNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(FIX2INTNodeGen root) {
super(root, 2147483647);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value) {
return (int) uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(FIX2INTNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(FIX2INTNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(FIX2INTNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public int executeInt(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return getNext().executeInt_(frameValue, arguments0Value_);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().executeInt_(frameValue, arguments0Value);
}
static BaseNode_ create(FIX2INTNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "fix2int(int)", value = FIX2INTNode.class)
private static final class Fix2intNode_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
Fix2intNode_(FIX2INTNodeGen root, Object arguments0Value) {
super(root, 1);
this.arguments0ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((Fix2intNode_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeInt(frameValue);
}
@Override
public int executeInt(VirtualFrame frameValue) {
int arguments0Value_;
try {
if (arguments0ImplicitType == int.class) {
arguments0Value_ = root.arguments0_.executeInteger(frameValue);
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitInteger(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeInt_(frameValue, ex.getResult());
}
return root.fix2int(arguments0Value_);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value, arguments0ImplicitType)) {
int arguments0Value_ = RubyTypesGen.asImplicitInteger(arguments0Value, arguments0ImplicitType);
return root.fix2int(arguments0Value_);
}
return getNext().executeInt_(frameValue, arguments0Value);
}
static BaseNode_ create(FIX2INTNodeGen root, Object arguments0Value) {
return new Fix2intNode_(root, arguments0Value);
}
}
}
}
@GeneratedBy(FIX2UINTNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class FIX2UINTNodeFactory implements NodeFactory {
private static FIX2UINTNodeFactory fIX2UINTNodeFactoryInstance;
private FIX2UINTNodeFactory() {
}
@Override
public Class getNodeClass() {
return FIX2UINTNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public FIX2UINTNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (fIX2UINTNodeFactoryInstance == null) {
fIX2UINTNodeFactoryInstance = new FIX2UINTNodeFactory();
}
return fIX2UINTNodeFactoryInstance;
}
public static FIX2UINTNode create(RubyNode[] arguments) {
return new FIX2UINTNodeGen(arguments);
}
@GeneratedBy(FIX2UINTNode.class)
public static final class FIX2UINTNodeGen extends FIX2UINTNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@CompilationFinal private Class> arguments0Type_;
@Child private BaseNode_ specialization_;
private FIX2UINTNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public int executeInteger(VirtualFrame frameValue) throws UnexpectedResultException {
return specialization_.executeInt(frameValue);
}
@Override
public long executeLong(VirtualFrame frameValue) throws UnexpectedResultException {
return specialization_.executeLong(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
private static int expectInteger(Object value) throws UnexpectedResultException {
if (value instanceof Integer) {
return (int) value;
}
throw new UnexpectedResultException(value);
}
private static long expectLong(Object value) throws UnexpectedResultException {
if (value instanceof Long) {
return (long) value;
}
throw new UnexpectedResultException(value);
}
@GeneratedBy(FIX2UINTNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected FIX2UINTNodeGen root;
BaseNode_(FIX2UINTNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (FIX2UINTNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.execute_((VirtualFrame) frameValue, arguments0Value);
}
public abstract Object execute_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return execute_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public int executeInt(VirtualFrame frameValue) throws UnexpectedResultException {
return expectInteger(execute(frameValue));
}
public long executeLong(VirtualFrame frameValue) throws UnexpectedResultException {
return expectLong(execute(frameValue));
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value)) {
return Fix2uint0Node_.create(root, arguments0Value);
}
if (RubyTypesGen.isImplicitLong(arguments0Value)) {
return Fix2uint1Node_.create(root, arguments0Value);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments0_(Frame frameValue) {
Class> arguments0Type_ = root.arguments0Type_;
try {
if (arguments0Type_ == int.class) {
return root.arguments0_.executeInteger((VirtualFrame) frameValue);
} else if (arguments0Type_ == long.class) {
return root.arguments0_.executeLong((VirtualFrame) frameValue);
} else if (arguments0Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments0_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else if (_value instanceof Long) {
_type = long.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments0Type_ = _type;
}
} else {
return root.arguments0_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments0Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(FIX2UINTNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(FIX2UINTNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
return uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(FIX2UINTNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(FIX2UINTNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(FIX2UINTNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().execute_(frameValue, arguments0Value);
}
static BaseNode_ create(FIX2UINTNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "fix2uint(int)", value = FIX2UINTNode.class)
private static final class Fix2uint0Node_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
Fix2uint0Node_(FIX2UINTNodeGen root, Object arguments0Value) {
super(root, 1);
this.arguments0ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((Fix2uint0Node_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
try {
return executeInt(frameValue);
} catch (UnexpectedResultException ex) {
return ex.getResult();
}
}
@Override
public int executeInt(VirtualFrame frameValue) throws UnexpectedResultException {
int arguments0Value_;
try {
if (arguments0ImplicitType == int.class) {
arguments0Value_ = root.arguments0_.executeInteger(frameValue);
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitInteger(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return expectInteger(getNext().execute_(frameValue, ex.getResult()));
}
return root.fix2uint(arguments0Value_);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value, arguments0ImplicitType)) {
int arguments0Value_ = RubyTypesGen.asImplicitInteger(arguments0Value, arguments0ImplicitType);
return root.fix2uint(arguments0Value_);
}
return getNext().execute_(frameValue, arguments0Value);
}
static BaseNode_ create(FIX2UINTNodeGen root, Object arguments0Value) {
return new Fix2uint0Node_(root, arguments0Value);
}
}
@GeneratedBy(methodName = "fix2uint(long)", value = FIX2UINTNode.class)
private static final class Fix2uint1Node_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
Fix2uint1Node_(FIX2UINTNodeGen root, Object arguments0Value) {
super(root, 2);
this.arguments0ImplicitType = RubyTypesGen.getImplicitLongClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((Fix2uint1Node_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
try {
return executeLong(frameValue);
} catch (UnexpectedResultException ex) {
return ex.getResult();
}
}
@Override
public long executeLong(VirtualFrame frameValue) throws UnexpectedResultException {
long arguments0Value_;
try {
if (arguments0ImplicitType == long.class) {
arguments0Value_ = root.arguments0_.executeLong(frameValue);
} else if (arguments0ImplicitType == int.class) {
arguments0Value_ = RubyTypes.promoteToLong(root.arguments0_.executeInteger(frameValue));
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitLong(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return expectLong(getNext().execute_(frameValue, ex.getResult()));
}
return root.fix2uint(arguments0Value_);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitLong(arguments0Value, arguments0ImplicitType)) {
long arguments0Value_ = RubyTypesGen.asImplicitLong(arguments0Value, arguments0ImplicitType);
return root.fix2uint(arguments0Value_);
}
return getNext().execute_(frameValue, arguments0Value);
}
static BaseNode_ create(FIX2UINTNodeGen root, Object arguments0Value) {
return new Fix2uint1Node_(root, arguments0Value);
}
}
}
}
@GeneratedBy(FIX2LONGNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class FIX2LONGNodeFactory implements NodeFactory {
private static FIX2LONGNodeFactory fIX2LONGNodeFactoryInstance;
private FIX2LONGNodeFactory() {
}
@Override
public Class getNodeClass() {
return FIX2LONGNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public FIX2LONGNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (fIX2LONGNodeFactoryInstance == null) {
fIX2LONGNodeFactoryInstance = new FIX2LONGNodeFactory();
}
return fIX2LONGNodeFactoryInstance;
}
public static FIX2LONGNode create(RubyNode[] arguments) {
return new FIX2LONGNodeGen(arguments);
}
@GeneratedBy(FIX2LONGNode.class)
public static final class FIX2LONGNodeGen extends FIX2LONGNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@CompilationFinal private Class> arguments0Type_;
@Child private BaseNode_ specialization_;
private FIX2LONGNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public long executeLong(VirtualFrame frameValue) {
return specialization_.executeLong(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(FIX2LONGNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected FIX2LONGNodeGen root;
BaseNode_(FIX2LONGNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (FIX2LONGNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.executeLong_((VirtualFrame) frameValue, arguments0Value);
}
public abstract long executeLong_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return executeLong_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public long executeLong(VirtualFrame frameValue) {
return (long) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value)) {
return Fix2longNode_.create(root, arguments0Value);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments0_(Frame frameValue) {
Class> arguments0Type_ = root.arguments0Type_;
try {
if (arguments0Type_ == int.class) {
return root.arguments0_.executeInteger((VirtualFrame) frameValue);
} else if (arguments0Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments0_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments0Type_ = _type;
}
} else {
return root.arguments0_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments0Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(FIX2LONGNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(FIX2LONGNodeGen root) {
super(root, 2147483647);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value) {
return (long) uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(FIX2LONGNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(FIX2LONGNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(FIX2LONGNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public long executeLong(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return getNext().executeLong_(frameValue, arguments0Value_);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().executeLong_(frameValue, arguments0Value);
}
static BaseNode_ create(FIX2LONGNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "fix2long(int)", value = FIX2LONGNode.class)
private static final class Fix2longNode_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
Fix2longNode_(FIX2LONGNodeGen root, Object arguments0Value) {
super(root, 1);
this.arguments0ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((Fix2longNode_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeLong(frameValue);
}
@Override
public long executeLong(VirtualFrame frameValue) {
int arguments0Value_;
try {
if (arguments0ImplicitType == int.class) {
arguments0Value_ = root.arguments0_.executeInteger(frameValue);
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitInteger(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeLong_(frameValue, ex.getResult());
}
return root.fix2long(arguments0Value_);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value, arguments0ImplicitType)) {
int arguments0Value_ = RubyTypesGen.asImplicitInteger(arguments0Value, arguments0ImplicitType);
return root.fix2long(arguments0Value_);
}
return getNext().executeLong_(frameValue, arguments0Value);
}
static BaseNode_ create(FIX2LONGNodeGen root, Object arguments0Value) {
return new Fix2longNode_(root, arguments0Value);
}
}
}
}
@GeneratedBy(INT2NUMNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class INT2NUMNodeFactory implements NodeFactory {
private static INT2NUMNodeFactory iNT2NUMNodeFactoryInstance;
private INT2NUMNodeFactory() {
}
@Override
public Class getNodeClass() {
return INT2NUMNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public INT2NUMNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (iNT2NUMNodeFactoryInstance == null) {
iNT2NUMNodeFactoryInstance = new INT2NUMNodeFactory();
}
return iNT2NUMNodeFactoryInstance;
}
public static INT2NUMNode create(RubyNode[] arguments) {
return new INT2NUMNodeGen(arguments);
}
@GeneratedBy(INT2NUMNode.class)
public static final class INT2NUMNodeGen extends INT2NUMNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@CompilationFinal private Class> arguments0Type_;
@Child private BaseNode_ specialization_;
private INT2NUMNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public int executeInteger(VirtualFrame frameValue) throws UnexpectedResultException {
return specialization_.executeInt(frameValue);
}
@Override
public long executeLong(VirtualFrame frameValue) throws UnexpectedResultException {
return specialization_.executeLong(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
private static int expectInteger(Object value) throws UnexpectedResultException {
if (value instanceof Integer) {
return (int) value;
}
throw new UnexpectedResultException(value);
}
private static long expectLong(Object value) throws UnexpectedResultException {
if (value instanceof Long) {
return (long) value;
}
throw new UnexpectedResultException(value);
}
@GeneratedBy(INT2NUMNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected INT2NUMNodeGen root;
BaseNode_(INT2NUMNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (INT2NUMNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.execute_((VirtualFrame) frameValue, arguments0Value);
}
public abstract Object execute_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return execute_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public int executeInt(VirtualFrame frameValue) throws UnexpectedResultException {
return expectInteger(execute(frameValue));
}
public long executeLong(VirtualFrame frameValue) throws UnexpectedResultException {
return expectLong(execute(frameValue));
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value)) {
return Int2num0Node_.create(root, arguments0Value);
}
if (RubyTypesGen.isImplicitLong(arguments0Value)) {
return Int2num1Node_.create(root, arguments0Value);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments0_(Frame frameValue) {
Class> arguments0Type_ = root.arguments0Type_;
try {
if (arguments0Type_ == int.class) {
return root.arguments0_.executeInteger((VirtualFrame) frameValue);
} else if (arguments0Type_ == long.class) {
return root.arguments0_.executeLong((VirtualFrame) frameValue);
} else if (arguments0Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments0_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else if (_value instanceof Long) {
_type = long.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments0Type_ = _type;
}
} else {
return root.arguments0_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments0Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(INT2NUMNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(INT2NUMNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
return uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(INT2NUMNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(INT2NUMNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(INT2NUMNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().execute_(frameValue, arguments0Value);
}
static BaseNode_ create(INT2NUMNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "int2num(int)", value = INT2NUMNode.class)
private static final class Int2num0Node_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
Int2num0Node_(INT2NUMNodeGen root, Object arguments0Value) {
super(root, 1);
this.arguments0ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((Int2num0Node_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
try {
return executeInt(frameValue);
} catch (UnexpectedResultException ex) {
return ex.getResult();
}
}
@Override
public int executeInt(VirtualFrame frameValue) throws UnexpectedResultException {
int arguments0Value_;
try {
if (arguments0ImplicitType == int.class) {
arguments0Value_ = root.arguments0_.executeInteger(frameValue);
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitInteger(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return expectInteger(getNext().execute_(frameValue, ex.getResult()));
}
return root.int2num(arguments0Value_);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value, arguments0ImplicitType)) {
int arguments0Value_ = RubyTypesGen.asImplicitInteger(arguments0Value, arguments0ImplicitType);
return root.int2num(arguments0Value_);
}
return getNext().execute_(frameValue, arguments0Value);
}
static BaseNode_ create(INT2NUMNodeGen root, Object arguments0Value) {
return new Int2num0Node_(root, arguments0Value);
}
}
@GeneratedBy(methodName = "int2num(long)", value = INT2NUMNode.class)
private static final class Int2num1Node_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
Int2num1Node_(INT2NUMNodeGen root, Object arguments0Value) {
super(root, 2);
this.arguments0ImplicitType = RubyTypesGen.getImplicitLongClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((Int2num1Node_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
try {
return executeLong(frameValue);
} catch (UnexpectedResultException ex) {
return ex.getResult();
}
}
@Override
public long executeLong(VirtualFrame frameValue) throws UnexpectedResultException {
long arguments0Value_;
try {
if (arguments0ImplicitType == long.class) {
arguments0Value_ = root.arguments0_.executeLong(frameValue);
} else if (arguments0ImplicitType == int.class) {
arguments0Value_ = RubyTypes.promoteToLong(root.arguments0_.executeInteger(frameValue));
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitLong(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return expectLong(getNext().execute_(frameValue, ex.getResult()));
}
return root.int2num(arguments0Value_);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitLong(arguments0Value, arguments0ImplicitType)) {
long arguments0Value_ = RubyTypesGen.asImplicitLong(arguments0Value, arguments0ImplicitType);
return root.int2num(arguments0Value_);
}
return getNext().execute_(frameValue, arguments0Value);
}
static BaseNode_ create(INT2NUMNodeGen root, Object arguments0Value) {
return new Int2num1Node_(root, arguments0Value);
}
}
}
}
@GeneratedBy(INT2FIXNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class INT2FIXNodeFactory implements NodeFactory {
private static INT2FIXNodeFactory iNT2FIXNodeFactoryInstance;
private INT2FIXNodeFactory() {
}
@Override
public Class getNodeClass() {
return INT2FIXNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public INT2FIXNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (iNT2FIXNodeFactoryInstance == null) {
iNT2FIXNodeFactoryInstance = new INT2FIXNodeFactory();
}
return iNT2FIXNodeFactoryInstance;
}
public static INT2FIXNode create(RubyNode[] arguments) {
return new INT2FIXNodeGen(arguments);
}
@GeneratedBy(INT2FIXNode.class)
public static final class INT2FIXNodeGen extends INT2FIXNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@CompilationFinal private Class> arguments0Type_;
@Child private BaseNode_ specialization_;
private INT2FIXNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public int executeInteger(VirtualFrame frameValue) throws UnexpectedResultException {
return specialization_.executeInt(frameValue);
}
@Override
public long executeLong(VirtualFrame frameValue) throws UnexpectedResultException {
return specialization_.executeLong(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
private static int expectInteger(Object value) throws UnexpectedResultException {
if (value instanceof Integer) {
return (int) value;
}
throw new UnexpectedResultException(value);
}
private static long expectLong(Object value) throws UnexpectedResultException {
if (value instanceof Long) {
return (long) value;
}
throw new UnexpectedResultException(value);
}
@GeneratedBy(INT2FIXNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected INT2FIXNodeGen root;
BaseNode_(INT2FIXNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (INT2FIXNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.execute_((VirtualFrame) frameValue, arguments0Value);
}
public abstract Object execute_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return execute_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public int executeInt(VirtualFrame frameValue) throws UnexpectedResultException {
return expectInteger(execute(frameValue));
}
public long executeLong(VirtualFrame frameValue) throws UnexpectedResultException {
return expectLong(execute(frameValue));
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value)) {
return Int2fix0Node_.create(root, arguments0Value);
}
if (RubyTypesGen.isImplicitLong(arguments0Value)) {
return Int2fix1Node_.create(root, arguments0Value);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments0_(Frame frameValue) {
Class> arguments0Type_ = root.arguments0Type_;
try {
if (arguments0Type_ == int.class) {
return root.arguments0_.executeInteger((VirtualFrame) frameValue);
} else if (arguments0Type_ == long.class) {
return root.arguments0_.executeLong((VirtualFrame) frameValue);
} else if (arguments0Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments0_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else if (_value instanceof Long) {
_type = long.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments0Type_ = _type;
}
} else {
return root.arguments0_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments0Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(INT2FIXNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(INT2FIXNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
return uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(INT2FIXNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(INT2FIXNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(INT2FIXNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().execute_(frameValue, arguments0Value);
}
static BaseNode_ create(INT2FIXNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "int2fix(int)", value = INT2FIXNode.class)
private static final class Int2fix0Node_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
Int2fix0Node_(INT2FIXNodeGen root, Object arguments0Value) {
super(root, 1);
this.arguments0ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((Int2fix0Node_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
try {
return executeInt(frameValue);
} catch (UnexpectedResultException ex) {
return ex.getResult();
}
}
@Override
public int executeInt(VirtualFrame frameValue) throws UnexpectedResultException {
int arguments0Value_;
try {
if (arguments0ImplicitType == int.class) {
arguments0Value_ = root.arguments0_.executeInteger(frameValue);
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitInteger(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return expectInteger(getNext().execute_(frameValue, ex.getResult()));
}
return root.int2fix(arguments0Value_);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value, arguments0ImplicitType)) {
int arguments0Value_ = RubyTypesGen.asImplicitInteger(arguments0Value, arguments0ImplicitType);
return root.int2fix(arguments0Value_);
}
return getNext().execute_(frameValue, arguments0Value);
}
static BaseNode_ create(INT2FIXNodeGen root, Object arguments0Value) {
return new Int2fix0Node_(root, arguments0Value);
}
}
@GeneratedBy(methodName = "int2fix(long)", value = INT2FIXNode.class)
private static final class Int2fix1Node_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
Int2fix1Node_(INT2FIXNodeGen root, Object arguments0Value) {
super(root, 2);
this.arguments0ImplicitType = RubyTypesGen.getImplicitLongClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((Int2fix1Node_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
try {
return executeLong(frameValue);
} catch (UnexpectedResultException ex) {
return ex.getResult();
}
}
@Override
public long executeLong(VirtualFrame frameValue) throws UnexpectedResultException {
long arguments0Value_;
try {
if (arguments0ImplicitType == long.class) {
arguments0Value_ = root.arguments0_.executeLong(frameValue);
} else if (arguments0ImplicitType == int.class) {
arguments0Value_ = RubyTypes.promoteToLong(root.arguments0_.executeInteger(frameValue));
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitLong(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return expectLong(getNext().execute_(frameValue, ex.getResult()));
}
return root.int2fix(arguments0Value_);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitLong(arguments0Value, arguments0ImplicitType)) {
long arguments0Value_ = RubyTypesGen.asImplicitLong(arguments0Value, arguments0ImplicitType);
return root.int2fix(arguments0Value_);
}
return getNext().execute_(frameValue, arguments0Value);
}
static BaseNode_ create(INT2FIXNodeGen root, Object arguments0Value) {
return new Int2fix1Node_(root, arguments0Value);
}
}
}
}
@GeneratedBy(UINT2NUMNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class UINT2NUMNodeFactory implements NodeFactory {
private static UINT2NUMNodeFactory uINT2NUMNodeFactoryInstance;
private UINT2NUMNodeFactory() {
}
@Override
public Class getNodeClass() {
return UINT2NUMNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public UINT2NUMNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (uINT2NUMNodeFactoryInstance == null) {
uINT2NUMNodeFactoryInstance = new UINT2NUMNodeFactory();
}
return uINT2NUMNodeFactoryInstance;
}
public static UINT2NUMNode create(RubyNode[] arguments) {
return new UINT2NUMNodeGen(arguments);
}
@GeneratedBy(UINT2NUMNode.class)
public static final class UINT2NUMNodeGen extends UINT2NUMNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@CompilationFinal private Class> arguments0Type_;
@Child private BaseNode_ specialization_;
private UINT2NUMNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public int executeInteger(VirtualFrame frameValue) {
return specialization_.executeInt(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(UINT2NUMNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected UINT2NUMNodeGen root;
BaseNode_(UINT2NUMNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (UINT2NUMNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.executeInt_((VirtualFrame) frameValue, arguments0Value);
}
public abstract int executeInt_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return executeInt_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public int executeInt(VirtualFrame frameValue) {
return (int) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value)) {
return Uint2numNode_.create(root, arguments0Value);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments0_(Frame frameValue) {
Class> arguments0Type_ = root.arguments0Type_;
try {
if (arguments0Type_ == int.class) {
return root.arguments0_.executeInteger((VirtualFrame) frameValue);
} else if (arguments0Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments0_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments0Type_ = _type;
}
} else {
return root.arguments0_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments0Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(UINT2NUMNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(UINT2NUMNodeGen root) {
super(root, 2147483647);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value) {
return (int) uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(UINT2NUMNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(UINT2NUMNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(UINT2NUMNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public int executeInt(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return getNext().executeInt_(frameValue, arguments0Value_);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().executeInt_(frameValue, arguments0Value);
}
static BaseNode_ create(UINT2NUMNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "uint2num(int)", value = UINT2NUMNode.class)
private static final class Uint2numNode_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
Uint2numNode_(UINT2NUMNodeGen root, Object arguments0Value) {
super(root, 1);
this.arguments0ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((Uint2numNode_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeInt(frameValue);
}
@Override
public int executeInt(VirtualFrame frameValue) {
int arguments0Value_;
try {
if (arguments0ImplicitType == int.class) {
arguments0Value_ = root.arguments0_.executeInteger(frameValue);
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitInteger(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeInt_(frameValue, ex.getResult());
}
return root.uint2num(arguments0Value_);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value, arguments0ImplicitType)) {
int arguments0Value_ = RubyTypesGen.asImplicitInteger(arguments0Value, arguments0ImplicitType);
return root.uint2num(arguments0Value_);
}
return getNext().executeInt_(frameValue, arguments0Value);
}
static BaseNode_ create(UINT2NUMNodeGen root, Object arguments0Value) {
return new Uint2numNode_(root, arguments0Value);
}
}
}
}
@GeneratedBy(LONG2NUMNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class LONG2NUMNodeFactory implements NodeFactory {
private static LONG2NUMNodeFactory lONG2NUMNodeFactoryInstance;
private LONG2NUMNodeFactory() {
}
@Override
public Class getNodeClass() {
return LONG2NUMNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public LONG2NUMNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (lONG2NUMNodeFactoryInstance == null) {
lONG2NUMNodeFactoryInstance = new LONG2NUMNodeFactory();
}
return lONG2NUMNodeFactoryInstance;
}
public static LONG2NUMNode create(RubyNode[] arguments) {
return new LONG2NUMNodeGen(arguments);
}
@GeneratedBy(LONG2NUMNode.class)
public static final class LONG2NUMNodeGen extends LONG2NUMNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@CompilationFinal private Class> arguments0Type_;
@Child private BaseNode_ specialization_;
private LONG2NUMNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public long executeLong(VirtualFrame frameValue) {
return specialization_.executeLong(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(LONG2NUMNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected LONG2NUMNodeGen root;
BaseNode_(LONG2NUMNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (LONG2NUMNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.executeLong_((VirtualFrame) frameValue, arguments0Value);
}
public abstract long executeLong_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return executeLong_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public long executeLong(VirtualFrame frameValue) {
return (long) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitLong(arguments0Value)) {
return Long2numNode_.create(root, arguments0Value);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments0_(Frame frameValue) {
Class> arguments0Type_ = root.arguments0Type_;
try {
if (arguments0Type_ == int.class) {
return root.arguments0_.executeInteger((VirtualFrame) frameValue);
} else if (arguments0Type_ == long.class) {
return root.arguments0_.executeLong((VirtualFrame) frameValue);
} else if (arguments0Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments0_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else if (_value instanceof Long) {
_type = long.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments0Type_ = _type;
}
} else {
return root.arguments0_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments0Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(LONG2NUMNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(LONG2NUMNodeGen root) {
super(root, 2147483647);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value) {
return (long) uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(LONG2NUMNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(LONG2NUMNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(LONG2NUMNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public long executeLong(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return getNext().executeLong_(frameValue, arguments0Value_);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().executeLong_(frameValue, arguments0Value);
}
static BaseNode_ create(LONG2NUMNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "long2num(long)", value = LONG2NUMNode.class)
private static final class Long2numNode_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
Long2numNode_(LONG2NUMNodeGen root, Object arguments0Value) {
super(root, 1);
this.arguments0ImplicitType = RubyTypesGen.getImplicitLongClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((Long2numNode_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeLong(frameValue);
}
@Override
public long executeLong(VirtualFrame frameValue) {
long arguments0Value_;
try {
if (arguments0ImplicitType == long.class) {
arguments0Value_ = root.arguments0_.executeLong(frameValue);
} else if (arguments0ImplicitType == int.class) {
arguments0Value_ = RubyTypes.promoteToLong(root.arguments0_.executeInteger(frameValue));
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitLong(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeLong_(frameValue, ex.getResult());
}
return root.long2num(arguments0Value_);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitLong(arguments0Value, arguments0ImplicitType)) {
long arguments0Value_ = RubyTypesGen.asImplicitLong(arguments0Value, arguments0ImplicitType);
return root.long2num(arguments0Value_);
}
return getNext().executeLong_(frameValue, arguments0Value);
}
static BaseNode_ create(LONG2NUMNodeGen root, Object arguments0Value) {
return new Long2numNode_(root, arguments0Value);
}
}
}
}
@GeneratedBy(ULONG2NUMNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class ULONG2NUMNodeFactory implements NodeFactory {
private static ULONG2NUMNodeFactory uLONG2NUMNodeFactoryInstance;
private ULONG2NUMNodeFactory() {
}
@Override
public Class getNodeClass() {
return ULONG2NUMNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public ULONG2NUMNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (uLONG2NUMNodeFactoryInstance == null) {
uLONG2NUMNodeFactoryInstance = new ULONG2NUMNodeFactory();
}
return uLONG2NUMNodeFactoryInstance;
}
public static ULONG2NUMNode create(RubyNode[] arguments) {
return new ULONG2NUMNodeGen(arguments);
}
@GeneratedBy(ULONG2NUMNode.class)
public static final class ULONG2NUMNodeGen extends ULONG2NUMNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@CompilationFinal private Class> arguments0Type_;
@Child private BaseNode_ specialization_;
private ULONG2NUMNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public long executeLong(VirtualFrame frameValue) {
return specialization_.executeLong(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(ULONG2NUMNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected ULONG2NUMNodeGen root;
BaseNode_(ULONG2NUMNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (ULONG2NUMNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.executeLong_((VirtualFrame) frameValue, arguments0Value);
}
public abstract long executeLong_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return executeLong_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public long executeLong(VirtualFrame frameValue) {
return (long) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitLong(arguments0Value)) {
return Ulong2numNode_.create(root, arguments0Value);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments0_(Frame frameValue) {
Class> arguments0Type_ = root.arguments0Type_;
try {
if (arguments0Type_ == int.class) {
return root.arguments0_.executeInteger((VirtualFrame) frameValue);
} else if (arguments0Type_ == long.class) {
return root.arguments0_.executeLong((VirtualFrame) frameValue);
} else if (arguments0Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments0_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else if (_value instanceof Long) {
_type = long.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments0Type_ = _type;
}
} else {
return root.arguments0_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments0Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(ULONG2NUMNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(ULONG2NUMNodeGen root) {
super(root, 2147483647);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value) {
return (long) uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(ULONG2NUMNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(ULONG2NUMNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(ULONG2NUMNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public long executeLong(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return getNext().executeLong_(frameValue, arguments0Value_);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().executeLong_(frameValue, arguments0Value);
}
static BaseNode_ create(ULONG2NUMNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "ulong2num(long)", value = ULONG2NUMNode.class)
private static final class Ulong2numNode_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
Ulong2numNode_(ULONG2NUMNodeGen root, Object arguments0Value) {
super(root, 1);
this.arguments0ImplicitType = RubyTypesGen.getImplicitLongClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((Ulong2numNode_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeLong(frameValue);
}
@Override
public long executeLong(VirtualFrame frameValue) {
long arguments0Value_;
try {
if (arguments0ImplicitType == long.class) {
arguments0Value_ = root.arguments0_.executeLong(frameValue);
} else if (arguments0ImplicitType == int.class) {
arguments0Value_ = RubyTypes.promoteToLong(root.arguments0_.executeInteger(frameValue));
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitLong(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeLong_(frameValue, ex.getResult());
}
return root.ulong2num(arguments0Value_);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitLong(arguments0Value, arguments0ImplicitType)) {
long arguments0Value_ = RubyTypesGen.asImplicitLong(arguments0Value, arguments0ImplicitType);
return root.ulong2num(arguments0Value_);
}
return getNext().executeLong_(frameValue, arguments0Value);
}
static BaseNode_ create(ULONG2NUMNodeGen root, Object arguments0Value) {
return new Ulong2numNode_(root, arguments0Value);
}
}
}
}
@GeneratedBy(LONG2FIXNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class LONG2FIXNodeFactory implements NodeFactory {
private static LONG2FIXNodeFactory lONG2FIXNodeFactoryInstance;
private LONG2FIXNodeFactory() {
}
@Override
public Class getNodeClass() {
return LONG2FIXNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public LONG2FIXNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (lONG2FIXNodeFactoryInstance == null) {
lONG2FIXNodeFactoryInstance = new LONG2FIXNodeFactory();
}
return lONG2FIXNodeFactoryInstance;
}
public static LONG2FIXNode create(RubyNode[] arguments) {
return new LONG2FIXNodeGen(arguments);
}
@GeneratedBy(LONG2FIXNode.class)
public static final class LONG2FIXNodeGen extends LONG2FIXNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@CompilationFinal private Class> arguments0Type_;
@Child private BaseNode_ specialization_;
private LONG2FIXNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public long executeLong(VirtualFrame frameValue) {
return specialization_.executeLong(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(LONG2FIXNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected LONG2FIXNodeGen root;
BaseNode_(LONG2FIXNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (LONG2FIXNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.executeLong_((VirtualFrame) frameValue, arguments0Value);
}
public abstract long executeLong_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return executeLong_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public long executeLong(VirtualFrame frameValue) {
return (long) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitLong(arguments0Value)) {
return Long2fixNode_.create(root, arguments0Value);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments0_(Frame frameValue) {
Class> arguments0Type_ = root.arguments0Type_;
try {
if (arguments0Type_ == int.class) {
return root.arguments0_.executeInteger((VirtualFrame) frameValue);
} else if (arguments0Type_ == long.class) {
return root.arguments0_.executeLong((VirtualFrame) frameValue);
} else if (arguments0Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments0_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else if (_value instanceof Long) {
_type = long.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments0Type_ = _type;
}
} else {
return root.arguments0_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments0Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(LONG2FIXNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(LONG2FIXNodeGen root) {
super(root, 2147483647);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value) {
return (long) uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(LONG2FIXNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(LONG2FIXNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(LONG2FIXNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public long executeLong(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return getNext().executeLong_(frameValue, arguments0Value_);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().executeLong_(frameValue, arguments0Value);
}
static BaseNode_ create(LONG2FIXNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "long2fix(long)", value = LONG2FIXNode.class)
private static final class Long2fixNode_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
Long2fixNode_(LONG2FIXNodeGen root, Object arguments0Value) {
super(root, 1);
this.arguments0ImplicitType = RubyTypesGen.getImplicitLongClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((Long2fixNode_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeLong(frameValue);
}
@Override
public long executeLong(VirtualFrame frameValue) {
long arguments0Value_;
try {
if (arguments0ImplicitType == long.class) {
arguments0Value_ = root.arguments0_.executeLong(frameValue);
} else if (arguments0ImplicitType == int.class) {
arguments0Value_ = RubyTypes.promoteToLong(root.arguments0_.executeInteger(frameValue));
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitLong(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeLong_(frameValue, ex.getResult());
}
return root.long2fix(arguments0Value_);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitLong(arguments0Value, arguments0ImplicitType)) {
long arguments0Value_ = RubyTypesGen.asImplicitLong(arguments0Value, arguments0ImplicitType);
return root.long2fix(arguments0Value_);
}
return getNext().executeLong_(frameValue, arguments0Value);
}
static BaseNode_ create(LONG2FIXNodeGen root, Object arguments0Value) {
return new Long2fixNode_(root, arguments0Value);
}
}
}
}
@GeneratedBy(CLASSOFNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class CLASSOFNodeFactory implements NodeFactory {
private static CLASSOFNodeFactory cLASSOFNodeFactoryInstance;
private CLASSOFNodeFactory() {
}
@Override
public Class getNodeClass() {
return CLASSOFNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public CLASSOFNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (cLASSOFNodeFactoryInstance == null) {
cLASSOFNodeFactoryInstance = new CLASSOFNodeFactory();
}
return cLASSOFNodeFactoryInstance;
}
public static CLASSOFNode create(RubyNode[] arguments) {
return new CLASSOFNodeGen(arguments);
}
@GeneratedBy(CLASSOFNode.class)
public static final class CLASSOFNodeGen extends CLASSOFNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private BaseNode_ specialization_;
private CLASSOFNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(CLASSOFNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected CLASSOFNodeGen root;
BaseNode_(CLASSOFNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (CLASSOFNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.executeDynamicObject_((VirtualFrame) frameValue, arguments0Value);
}
public abstract DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
return executeDynamicObject_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (arguments0Value instanceof DynamicObject) {
MetaClassNode metaClassNode1 = (MetaClassNode.create());
return Class_ofNode_.create(root, metaClassNode1);
}
return null;
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(CLASSOFNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(CLASSOFNodeGen root) {
super(root, 2147483647);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value) {
return (DynamicObject) uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(CLASSOFNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(methodName = "class_of(DynamicObject, MetaClassNode)", value = CLASSOFNode.class)
private static final class Class_ofNode_ extends BaseNode_ {
@Child private MetaClassNode metaClassNode;
Class_ofNode_(CLASSOFNodeGen root, MetaClassNode metaClassNode) {
super(root, 1);
this.metaClassNode = metaClassNode;
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value) {
if (arguments0Value instanceof DynamicObject) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
return root.class_of(arguments0Value_, this.metaClassNode);
}
return getNext().executeDynamicObject_(frameValue, arguments0Value);
}
static BaseNode_ create(CLASSOFNodeGen root, MetaClassNode metaClassNode) {
return new Class_ofNode_(root, metaClassNode);
}
}
}
}
@GeneratedBy(Long2Int.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class Long2IntFactory implements NodeFactory {
private static Long2IntFactory long2IntFactoryInstance;
private Long2IntFactory() {
}
@Override
public Class getNodeClass() {
return Long2Int.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public Long2Int createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (long2IntFactoryInstance == null) {
long2IntFactoryInstance = new Long2IntFactory();
}
return long2IntFactoryInstance;
}
public static Long2Int create(RubyNode[] arguments) {
return new Long2IntNodeGen(arguments);
}
@GeneratedBy(Long2Int.class)
public static final class Long2IntNodeGen extends Long2Int implements SpecializedNode {
@Child private RubyNode arguments0_;
@CompilationFinal private Class> arguments0Type_;
@Child private BaseNode_ specialization_;
private Long2IntNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public int executeInteger(VirtualFrame frameValue) {
return specialization_.executeInt(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(Long2Int.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected Long2IntNodeGen root;
BaseNode_(Long2IntNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (Long2IntNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.executeInt_((VirtualFrame) frameValue, arguments0Value);
}
public abstract int executeInt_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return executeInt_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public int executeInt(VirtualFrame frameValue) {
return (int) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value)) {
return Long2fixNode_.create(root, arguments0Value);
}
if (RubyTypesGen.isImplicitLong(arguments0Value)) {
long arguments0Value_ = RubyTypesGen.asImplicitLong(arguments0Value);
if ((root.fitsIntoInteger(arguments0Value_))) {
return Long2fixInRangeNode_.create(root, arguments0Value);
} else {
return Long2fixOutOfRangeNode_.create(root, arguments0Value);
}
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final long executeArguments0Long_(Frame frameValue, Class> arguments0ImplicitType) throws UnexpectedResultException {
if (arguments0ImplicitType == long.class) {
return root.arguments0_.executeLong((VirtualFrame) frameValue);
} else if (arguments0ImplicitType == int.class) {
return RubyTypes.promoteToLong(root.arguments0_.executeInteger((VirtualFrame) frameValue));
} else {
Object arguments0Value_ = executeArguments0_(frameValue);
return RubyTypesGen.expectImplicitLong(arguments0Value_, arguments0ImplicitType);
}
}
protected final Object executeArguments0_(Frame frameValue) {
Class> arguments0Type_ = root.arguments0Type_;
try {
if (arguments0Type_ == int.class) {
return root.arguments0_.executeInteger((VirtualFrame) frameValue);
} else if (arguments0Type_ == long.class) {
return root.arguments0_.executeLong((VirtualFrame) frameValue);
} else if (arguments0Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments0_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else if (_value instanceof Long) {
_type = long.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments0Type_ = _type;
}
} else {
return root.arguments0_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments0Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(Long2Int.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(Long2IntNodeGen root) {
super(root, 2147483647);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value) {
return (int) uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(Long2IntNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(Long2Int.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(Long2IntNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public int executeInt(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return getNext().executeInt_(frameValue, arguments0Value_);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().executeInt_(frameValue, arguments0Value);
}
static BaseNode_ create(Long2IntNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "long2fix(int)", value = Long2Int.class)
private static final class Long2fixNode_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
Long2fixNode_(Long2IntNodeGen root, Object arguments0Value) {
super(root, 1);
this.arguments0ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((Long2fixNode_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeInt(frameValue);
}
@Override
public int executeInt(VirtualFrame frameValue) {
int arguments0Value_;
try {
if (arguments0ImplicitType == int.class) {
arguments0Value_ = root.arguments0_.executeInteger(frameValue);
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitInteger(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeInt_(frameValue, ex.getResult());
}
return root.long2fix(arguments0Value_);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value, arguments0ImplicitType)) {
int arguments0Value_ = RubyTypesGen.asImplicitInteger(arguments0Value, arguments0ImplicitType);
return root.long2fix(arguments0Value_);
}
return getNext().executeInt_(frameValue, arguments0Value);
}
static BaseNode_ create(Long2IntNodeGen root, Object arguments0Value) {
return new Long2fixNode_(root, arguments0Value);
}
}
@GeneratedBy(methodName = "long2fixInRange(long)", value = Long2Int.class)
private static final class Long2fixInRangeNode_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
Long2fixInRangeNode_(Long2IntNodeGen root, Object arguments0Value) {
super(root, 2);
this.arguments0ImplicitType = RubyTypesGen.getImplicitLongClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((Long2fixInRangeNode_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeInt(frameValue);
}
@Override
public int executeInt(VirtualFrame frameValue) {
long arguments0Value_;
try {
arguments0Value_ = executeArguments0Long_(frameValue, arguments0ImplicitType);
} catch (UnexpectedResultException ex) {
return getNext().executeInt_(frameValue, ex.getResult());
}
if ((root.fitsIntoInteger(arguments0Value_))) {
return root.long2fixInRange(arguments0Value_);
}
return getNext().executeInt_(frameValue, arguments0Value_);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitLong(arguments0Value, arguments0ImplicitType)) {
long arguments0Value_ = RubyTypesGen.asImplicitLong(arguments0Value, arguments0ImplicitType);
if ((root.fitsIntoInteger(arguments0Value_))) {
return root.long2fixInRange(arguments0Value_);
}
}
return getNext().executeInt_(frameValue, arguments0Value);
}
static BaseNode_ create(Long2IntNodeGen root, Object arguments0Value) {
return new Long2fixInRangeNode_(root, arguments0Value);
}
}
@GeneratedBy(methodName = "long2fixOutOfRange(long)", value = Long2Int.class)
private static final class Long2fixOutOfRangeNode_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
Long2fixOutOfRangeNode_(Long2IntNodeGen root, Object arguments0Value) {
super(root, 3);
this.arguments0ImplicitType = RubyTypesGen.getImplicitLongClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((Long2fixOutOfRangeNode_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeInt(frameValue);
}
@Override
public int executeInt(VirtualFrame frameValue) {
long arguments0Value_;
try {
arguments0Value_ = executeArguments0Long_(frameValue, arguments0ImplicitType);
} catch (UnexpectedResultException ex) {
return getNext().executeInt_(frameValue, ex.getResult());
}
if ((!(root.fitsIntoInteger(arguments0Value_)))) {
return root.long2fixOutOfRange(arguments0Value_);
}
return getNext().executeInt_(frameValue, arguments0Value_);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitLong(arguments0Value, arguments0ImplicitType)) {
long arguments0Value_ = RubyTypesGen.asImplicitLong(arguments0Value, arguments0ImplicitType);
if ((!(root.fitsIntoInteger(arguments0Value_)))) {
return root.long2fixOutOfRange(arguments0Value_);
}
}
return getNext().executeInt_(frameValue, arguments0Value);
}
static BaseNode_ create(Long2IntNodeGen root, Object arguments0Value) {
return new Long2fixOutOfRangeNode_(root, arguments0Value);
}
}
}
}
@GeneratedBy(StringPointerNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class StringPointerNodeFactory implements NodeFactory {
private static StringPointerNodeFactory stringPointerNodeFactoryInstance;
private StringPointerNodeFactory() {
}
@Override
public Class getNodeClass() {
return StringPointerNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public StringPointerNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (stringPointerNodeFactoryInstance == null) {
stringPointerNodeFactoryInstance = new StringPointerNodeFactory();
}
return stringPointerNodeFactoryInstance;
}
public static StringPointerNode create(RubyNode[] arguments) {
return new StringPointerNodeGen(arguments);
}
@GeneratedBy(StringPointerNode.class)
public static final class StringPointerNodeGen extends StringPointerNode {
@Child private RubyNode arguments0_;
@CompilationFinal private boolean seenUnsupported0;
private StringPointerNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(ex.getResult());
}
if ((RubyGuards.isRubyString(arguments0Value_))) {
return this.stringPointer(arguments0Value_);
}
throw unsupported(arguments0Value_);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
private UnsupportedSpecializationException unsupported(Object arguments0Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_}, arguments0Value);
}
}
}
@GeneratedBy(ToRubyStringNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class ToRubyStringNodeFactory implements NodeFactory {
private static ToRubyStringNodeFactory toRubyStringNodeFactoryInstance;
private ToRubyStringNodeFactory() {
}
@Override
public Class getNodeClass() {
return ToRubyStringNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public ToRubyStringNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (toRubyStringNodeFactoryInstance == null) {
toRubyStringNodeFactoryInstance = new ToRubyStringNodeFactory();
}
return toRubyStringNodeFactoryInstance;
}
public static ToRubyStringNode create(RubyNode[] arguments) {
return new ToRubyStringNodeGen(arguments);
}
@GeneratedBy(ToRubyStringNode.class)
public static final class ToRubyStringNodeGen extends ToRubyStringNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private BaseNode_ specialization_;
private ToRubyStringNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(ToRubyStringNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected ToRubyStringNodeGen root;
BaseNode_(ToRubyStringNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (ToRubyStringNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.executeDynamicObject_((VirtualFrame) frameValue, arguments0Value);
}
public abstract DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
return executeDynamicObject_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (arguments0Value instanceof StringCharPointerAdapter) {
return ToRubyString0Node_.create(root);
}
if (arguments0Value instanceof DynamicObject) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
if ((RubyGuards.isRubyString(arguments0Value_))) {
return ToRubyString1Node_.create(root);
}
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(ToRubyStringNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(ToRubyStringNodeGen root) {
super(root, 2147483647);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value) {
return (DynamicObject) uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(ToRubyStringNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(ToRubyStringNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(ToRubyStringNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().executeDynamicObject_(frameValue, arguments0Value);
}
static BaseNode_ create(ToRubyStringNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "toRubyString(StringCharPointerAdapter)", value = ToRubyStringNode.class)
private static final class ToRubyString0Node_ extends BaseNode_ {
ToRubyString0Node_(ToRubyStringNodeGen root) {
super(root, 1);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value) {
if (arguments0Value instanceof StringCharPointerAdapter) {
StringCharPointerAdapter arguments0Value_ = (StringCharPointerAdapter) arguments0Value;
return root.toRubyString(arguments0Value_);
}
return getNext().executeDynamicObject_(frameValue, arguments0Value);
}
static BaseNode_ create(ToRubyStringNodeGen root) {
return new ToRubyString0Node_(root);
}
}
@GeneratedBy(methodName = "toRubyString(DynamicObject)", value = ToRubyStringNode.class)
private static final class ToRubyString1Node_ extends BaseNode_ {
ToRubyString1Node_(ToRubyStringNodeGen root) {
super(root, 2);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value) {
if (arguments0Value instanceof DynamicObject) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
if ((RubyGuards.isRubyString(arguments0Value_))) {
return root.toRubyString(arguments0Value_);
}
}
return getNext().executeDynamicObject_(frameValue, arguments0Value);
}
static BaseNode_ create(ToRubyStringNodeGen root) {
return new ToRubyString1Node_(root);
}
}
}
}
@GeneratedBy(BlockGivenNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class BlockGivenNodeFactory implements NodeFactory {
private static BlockGivenNodeFactory blockGivenNodeFactoryInstance;
private BlockGivenNodeFactory() {
}
@Override
public Class getNodeClass() {
return BlockGivenNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public BlockGivenNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (blockGivenNodeFactoryInstance == null) {
blockGivenNodeFactoryInstance = new BlockGivenNodeFactory();
}
return blockGivenNodeFactoryInstance;
}
public static BlockGivenNode create(RubyNode[] arguments) {
return new BlockGivenNodeGen(arguments);
}
@GeneratedBy(BlockGivenNode.class)
public static final class BlockGivenNodeGen extends BlockGivenNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private BaseNode_ specialization_;
private BlockGivenNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public int executeInteger(VirtualFrame frameValue) {
return specialization_.executeInt(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
private static MaterializedFrame expectMaterializedFrame(Object value) throws UnexpectedResultException {
if (value instanceof MaterializedFrame) {
return (MaterializedFrame) value;
}
throw new UnexpectedResultException(value);
}
@GeneratedBy(BlockGivenNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected BlockGivenNodeGen root;
BaseNode_(BlockGivenNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (BlockGivenNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.executeInt_((VirtualFrame) frameValue, arguments0Value);
}
public abstract int executeInt_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
return executeInt_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public int executeInt(VirtualFrame frameValue) {
return (int) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (arguments0Value instanceof MaterializedFrame) {
ConditionProfile blockProfile1 = (ConditionProfile.createBinaryProfile());
return BlockGiven0Node_.create(root, blockProfile1);
}
if (arguments0Value instanceof NotProvided) {
return BlockGiven1Node_.create(root);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(BlockGivenNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(BlockGivenNodeGen root) {
super(root, 2147483647);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value) {
return (int) uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(BlockGivenNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(BlockGivenNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(BlockGivenNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public int executeInt(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
return getNext().executeInt_(frameValue, arguments0Value_);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().executeInt_(frameValue, arguments0Value);
}
static BaseNode_ create(BlockGivenNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "blockGiven(MaterializedFrame, ConditionProfile)", value = BlockGivenNode.class)
private static final class BlockGiven0Node_ extends BaseNode_ {
private final ConditionProfile blockProfile;
BlockGiven0Node_(BlockGivenNodeGen root, ConditionProfile blockProfile) {
super(root, 1);
this.blockProfile = blockProfile;
}
@Override
public int executeInt(VirtualFrame frameValue) {
MaterializedFrame arguments0Value_;
try {
arguments0Value_ = expectMaterializedFrame(root.arguments0_.execute(frameValue));
} catch (UnexpectedResultException ex) {
return getNext().executeInt_(frameValue, ex.getResult());
}
return root.blockGiven(arguments0Value_, this.blockProfile);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value) {
if (arguments0Value instanceof MaterializedFrame) {
MaterializedFrame arguments0Value_ = (MaterializedFrame) arguments0Value;
return root.blockGiven(arguments0Value_, this.blockProfile);
}
return getNext().executeInt_(frameValue, arguments0Value);
}
static BaseNode_ create(BlockGivenNodeGen root, ConditionProfile blockProfile) {
return new BlockGiven0Node_(root, blockProfile);
}
}
@GeneratedBy(methodName = "blockGiven(NotProvided)", value = BlockGivenNode.class)
private static final class BlockGiven1Node_ extends BaseNode_ {
BlockGiven1Node_(BlockGivenNodeGen root) {
super(root, 2);
}
@Override
public int executeInt(VirtualFrame frameValue) {
NotProvided arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeNotProvided(frameValue);
} catch (UnexpectedResultException ex) {
return getNext().executeInt_(frameValue, ex.getResult());
}
return root.blockGiven(arguments0Value_);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value) {
if (arguments0Value instanceof NotProvided) {
NotProvided arguments0Value_ = (NotProvided) arguments0Value;
return root.blockGiven(arguments0Value_);
}
return getNext().executeInt_(frameValue, arguments0Value);
}
static BaseNode_ create(BlockGivenNodeGen root) {
return new BlockGiven1Node_(root);
}
}
}
}
@GeneratedBy(GetBlockNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class GetBlockNodeFactory implements NodeFactory {
private static GetBlockNodeFactory getBlockNodeFactoryInstance;
private GetBlockNodeFactory() {
}
@Override
public Class getNodeClass() {
return GetBlockNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList();
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public GetBlockNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (getBlockNodeFactoryInstance == null) {
getBlockNodeFactoryInstance = new GetBlockNodeFactory();
}
return getBlockNodeFactoryInstance;
}
public static GetBlockNode create(RubyNode[] arguments) {
return new GetBlockNodeGen(arguments);
}
@GeneratedBy(GetBlockNode.class)
public static final class GetBlockNodeGen extends GetBlockNode {
@SuppressWarnings("unused")
private GetBlockNodeGen(RubyNode[] arguments) {
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
return this.getBlock();
}
@Override
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
}
}
@GeneratedBy(ConstGetFromNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class ConstGetFromNodeFactory implements NodeFactory {
private static ConstGetFromNodeFactory constGetFromNodeFactoryInstance;
private ConstGetFromNodeFactory() {
}
@Override
public Class getNodeClass() {
return ConstGetFromNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode.class, RubyNode.class));
}
@Override
public ConstGetFromNode createNode(Object... arguments) {
if (arguments.length == 2 && (arguments[0] == null || arguments[0] instanceof RubyNode) && (arguments[1] == null || arguments[1] instanceof RubyNode)) {
return create((RubyNode) arguments[0], (RubyNode) arguments[1]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (constGetFromNodeFactoryInstance == null) {
constGetFromNodeFactoryInstance = new ConstGetFromNodeFactory();
}
return constGetFromNodeFactoryInstance;
}
public static ConstGetFromNode create(RubyNode module, RubyNode name) {
return new ConstGetFromNodeGen(module, name);
}
@GeneratedBy(ConstGetFromNode.class)
public static final class ConstGetFromNodeGen extends ConstGetFromNode {
@Child private RubyNode module_;
@Child private RubyNode name_;
@CompilationFinal private boolean seenUnsupported0;
private ConstGetFromNodeGen(RubyNode module, RubyNode name) {
this.module_ = module;
this.name_ = coerceToString(name);
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
DynamicObject moduleValue_;
try {
moduleValue_ = module_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object nameValue = name_.execute(frameValue);
throw unsupported(ex.getResult(), nameValue);
}
String nameValue_;
try {
nameValue_ = expectString(name_.execute(frameValue));
} catch (UnexpectedResultException ex) {
throw unsupported(moduleValue_, ex.getResult());
}
return this.constGetFrom(frameValue, moduleValue_, nameValue_);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
private UnsupportedSpecializationException unsupported(Object moduleValue, Object nameValue) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {module_, name_}, moduleValue, nameValue);
}
private static String expectString(Object value) throws UnexpectedResultException {
if (value instanceof String) {
return (String) value;
}
throw new UnexpectedResultException(value);
}
}
}
@GeneratedBy(IOHandleNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class IOHandleNodeFactory implements NodeFactory {
private static IOHandleNodeFactory iOHandleNodeFactoryInstance;
private IOHandleNodeFactory() {
}
@Override
public Class getNodeClass() {
return IOHandleNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public IOHandleNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (iOHandleNodeFactoryInstance == null) {
iOHandleNodeFactoryInstance = new IOHandleNodeFactory();
}
return iOHandleNodeFactoryInstance;
}
public static IOHandleNode create(RubyNode[] arguments) {
return new IOHandleNodeGen(arguments);
}
@GeneratedBy(IOHandleNode.class)
public static final class IOHandleNodeGen extends IOHandleNode {
@Child private RubyNode arguments0_;
@CompilationFinal private boolean seenUnsupported0;
private IOHandleNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeInteger(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
executeInteger(frameValue);
return;
}
@Override
public int executeInteger(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(ex.getResult());
}
if ((RubyGuards.isRubyIO(arguments0Value_))) {
return this.ioHandle(arguments0Value_);
}
throw unsupported(arguments0Value_);
}
private UnsupportedSpecializationException unsupported(Object arguments0Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_}, arguments0Value);
}
}
}
@GeneratedBy(CextModuleFunctionNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class CextModuleFunctionNodeFactory implements NodeFactory {
private static CextModuleFunctionNodeFactory cextModuleFunctionNodeFactoryInstance;
private CextModuleFunctionNodeFactory() {
}
@Override
public Class getNodeClass() {
return CextModuleFunctionNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public CextModuleFunctionNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (cextModuleFunctionNodeFactoryInstance == null) {
cextModuleFunctionNodeFactoryInstance = new CextModuleFunctionNodeFactory();
}
return cextModuleFunctionNodeFactoryInstance;
}
public static CextModuleFunctionNode create(RubyNode[] arguments) {
return new CextModuleFunctionNodeGen(arguments);
}
@GeneratedBy(CextModuleFunctionNode.class)
public static final class CextModuleFunctionNodeGen extends CextModuleFunctionNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@CompilationFinal private boolean seenUnsupported0;
private CextModuleFunctionNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = arguments1_.execute(frameValue);
throw unsupported(ex.getResult(), arguments1Value);
}
DynamicObject arguments1Value_;
try {
arguments1Value_ = arguments1_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(arguments0Value_, ex.getResult());
}
if ((RubyGuards.isRubyModule(arguments0Value_)) && (RubyGuards.isRubySymbol(arguments1Value_))) {
return this.cextModuleFunction(frameValue, arguments0Value_, arguments1Value_);
}
throw unsupported(arguments0Value_, arguments1Value_);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
private UnsupportedSpecializationException unsupported(Object arguments0Value, Object arguments1Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_, arguments1_}, arguments0Value, arguments1Value);
}
}
}
@GeneratedBy(CallerFrameVisibilityNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class CallerFrameVisibilityNodeFactory implements NodeFactory {
private static CallerFrameVisibilityNodeFactory callerFrameVisibilityNodeFactoryInstance;
private CallerFrameVisibilityNodeFactory() {
}
@Override
public Class getNodeClass() {
return CallerFrameVisibilityNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public CallerFrameVisibilityNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (callerFrameVisibilityNodeFactoryInstance == null) {
callerFrameVisibilityNodeFactoryInstance = new CallerFrameVisibilityNodeFactory();
}
return callerFrameVisibilityNodeFactoryInstance;
}
public static CallerFrameVisibilityNode create(RubyNode[] arguments) {
return new CallerFrameVisibilityNodeGen(arguments);
}
@GeneratedBy(CallerFrameVisibilityNode.class)
public static final class CallerFrameVisibilityNodeGen extends CallerFrameVisibilityNode {
@Child private RubyNode arguments0_;
@CompilationFinal private boolean seenUnsupported0;
private CallerFrameVisibilityNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeBoolean(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
executeBoolean(frameValue);
return;
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(ex.getResult());
}
if ((RubyGuards.isRubySymbol(arguments0Value_))) {
return this.toRubyString(arguments0Value_);
}
throw unsupported(arguments0Value_);
}
private UnsupportedSpecializationException unsupported(Object arguments0Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_}, arguments0Value);
}
}
}
@GeneratedBy(AdaptRDataNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class AdaptRDataNodeFactory implements NodeFactory {
private static AdaptRDataNodeFactory adaptRDataNodeFactoryInstance;
private AdaptRDataNodeFactory() {
}
@Override
public Class getNodeClass() {
return AdaptRDataNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public AdaptRDataNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (adaptRDataNodeFactoryInstance == null) {
adaptRDataNodeFactoryInstance = new AdaptRDataNodeFactory();
}
return adaptRDataNodeFactoryInstance;
}
public static AdaptRDataNode create(RubyNode[] arguments) {
return new AdaptRDataNodeGen(arguments);
}
@GeneratedBy(AdaptRDataNode.class)
public static final class AdaptRDataNodeGen extends AdaptRDataNode {
@Child private RubyNode arguments0_;
@CompilationFinal private boolean seenUnsupported0;
private AdaptRDataNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(ex.getResult());
}
return this.adaptRData(arguments0Value_);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
private UnsupportedSpecializationException unsupported(Object arguments0Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_}, arguments0Value);
}
}
}
@GeneratedBy(ToNativeHandleNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class ToNativeHandleNodeFactory implements NodeFactory {
private static ToNativeHandleNodeFactory toNativeHandleNodeFactoryInstance;
private ToNativeHandleNodeFactory() {
}
@Override
public Class getNodeClass() {
return ToNativeHandleNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public ToNativeHandleNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (toNativeHandleNodeFactoryInstance == null) {
toNativeHandleNodeFactoryInstance = new ToNativeHandleNodeFactory();
}
return toNativeHandleNodeFactoryInstance;
}
public static ToNativeHandleNode create(RubyNode[] arguments) {
return new ToNativeHandleNodeGen(arguments);
}
@GeneratedBy(ToNativeHandleNode.class)
public static final class ToNativeHandleNodeGen extends ToNativeHandleNode {
@Child private RubyNode arguments0_;
@CompilationFinal private boolean seenUnsupported0;
private ToNativeHandleNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeLong(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
executeLong(frameValue);
return;
}
@Override
public long executeLong(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(ex.getResult());
}
return this.toNativeHandle(arguments0Value_);
}
private UnsupportedSpecializationException unsupported(Object arguments0Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_}, arguments0Value);
}
}
}
@GeneratedBy(FromNativeHandleNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class FromNativeHandleNodeFactory implements NodeFactory {
private static FromNativeHandleNodeFactory fromNativeHandleNodeFactoryInstance;
private FromNativeHandleNodeFactory() {
}
@Override
public Class getNodeClass() {
return FromNativeHandleNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public FromNativeHandleNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (fromNativeHandleNodeFactoryInstance == null) {
fromNativeHandleNodeFactoryInstance = new FromNativeHandleNodeFactory();
}
return fromNativeHandleNodeFactoryInstance;
}
public static FromNativeHandleNode create(RubyNode[] arguments) {
return new FromNativeHandleNodeGen(arguments);
}
@GeneratedBy(FromNativeHandleNode.class)
public static final class FromNativeHandleNodeGen extends FromNativeHandleNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@CompilationFinal private Class> arguments0Type_;
@Child private BaseNode_ specialization_;
private FromNativeHandleNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
return specialization_.executeDynamicObject(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(FromNativeHandleNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected FromNativeHandleNodeGen root;
BaseNode_(FromNativeHandleNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (FromNativeHandleNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.executeDynamicObject_((VirtualFrame) frameValue, arguments0Value);
}
public abstract DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return executeDynamicObject_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
return (DynamicObject) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitLong(arguments0Value)) {
return FromNativeHandleNode_.create(root, arguments0Value);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments0_(Frame frameValue) {
Class> arguments0Type_ = root.arguments0Type_;
try {
if (arguments0Type_ == int.class) {
return root.arguments0_.executeInteger((VirtualFrame) frameValue);
} else if (arguments0Type_ == long.class) {
return root.arguments0_.executeLong((VirtualFrame) frameValue);
} else if (arguments0Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments0_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else if (_value instanceof Long) {
_type = long.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments0Type_ = _type;
}
} else {
return root.arguments0_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments0Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(FromNativeHandleNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(FromNativeHandleNodeGen root) {
super(root, 2147483647);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value) {
return (DynamicObject) uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(FromNativeHandleNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(FromNativeHandleNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(FromNativeHandleNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().executeDynamicObject_(frameValue, arguments0Value);
}
static BaseNode_ create(FromNativeHandleNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "fromNativeHandle(long)", value = FromNativeHandleNode.class)
private static final class FromNativeHandleNode_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
FromNativeHandleNode_(FromNativeHandleNodeGen root, Object arguments0Value) {
super(root, 1);
this.arguments0ImplicitType = RubyTypesGen.getImplicitLongClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((FromNativeHandleNode_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject(frameValue);
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
long arguments0Value_;
try {
if (arguments0ImplicitType == long.class) {
arguments0Value_ = root.arguments0_.executeLong(frameValue);
} else if (arguments0ImplicitType == int.class) {
arguments0Value_ = RubyTypes.promoteToLong(root.arguments0_.executeInteger(frameValue));
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitLong(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeDynamicObject_(frameValue, ex.getResult());
}
return root.fromNativeHandle(arguments0Value_);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitLong(arguments0Value, arguments0ImplicitType)) {
long arguments0Value_ = RubyTypesGen.asImplicitLong(arguments0Value, arguments0ImplicitType);
return root.fromNativeHandle(arguments0Value_);
}
return getNext().executeDynamicObject_(frameValue, arguments0Value);
}
static BaseNode_ create(FromNativeHandleNodeGen root, Object arguments0Value) {
return new FromNativeHandleNode_(root, arguments0Value);
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy