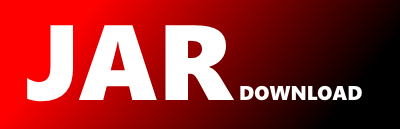
org.jruby.truffle.extra.ffi.PointerLayoutImpl Maven / Gradle / Ivy
The newest version!
package org.jruby.truffle.extra.ffi;
import java.util.EnumSet;
import com.oracle.truffle.api.CompilerAsserts;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.object.DynamicObject;
import com.oracle.truffle.api.object.DynamicObjectFactory;
import com.oracle.truffle.api.object.FinalLocationException;
import com.oracle.truffle.api.object.HiddenKey;
import com.oracle.truffle.api.object.IncompatibleLocationException;
import com.oracle.truffle.api.object.LocationModifier;
import com.oracle.truffle.api.object.ObjectType;
import com.oracle.truffle.api.object.Property;
import com.oracle.truffle.api.object.Shape;
import org.jruby.truffle.extra.ffi.PointerLayout;
import org.jruby.truffle.core.basicobject.BasicObjectLayoutImpl;
@GeneratedBy(org.jruby.truffle.extra.ffi.PointerLayout.class)
public class PointerLayoutImpl extends BasicObjectLayoutImpl implements PointerLayout {
public static final PointerLayout INSTANCE = new PointerLayoutImpl();
public static class PointerType extends BasicObjectLayoutImpl.BasicObjectType {
public PointerType(
com.oracle.truffle.api.object.DynamicObject logicalClass,
com.oracle.truffle.api.object.DynamicObject metaClass) {
super(
logicalClass,
metaClass);
}
@Override
public PointerType setLogicalClass(com.oracle.truffle.api.object.DynamicObject logicalClass) {
return new PointerType(
logicalClass,
metaClass);
}
@Override
public PointerType setMetaClass(com.oracle.truffle.api.object.DynamicObject metaClass) {
return new PointerType(
logicalClass,
metaClass);
}
}
protected static final Shape.Allocator POINTER_ALLOCATOR = LAYOUT.createAllocator();
protected static final HiddenKey POINTER_IDENTIFIER = new HiddenKey("pointer");
protected static final Property POINTER_PROPERTY = Property.create(POINTER_IDENTIFIER, POINTER_ALLOCATOR.locationForType(jnr.ffi.Pointer.class, EnumSet.of(LocationModifier.NonNull)), 0);
protected PointerLayoutImpl() {
}
@Override
public DynamicObjectFactory createPointerShape(
com.oracle.truffle.api.object.DynamicObject logicalClass,
com.oracle.truffle.api.object.DynamicObject metaClass) {
return LAYOUT.createShape(new PointerType(
logicalClass,
metaClass))
.addProperty(POINTER_PROPERTY)
.createFactory();
}
@Override
public DynamicObject createPointer(
DynamicObjectFactory factory,
jnr.ffi.Pointer pointer) {
assert factory != null;
CompilerAsserts.partialEvaluationConstant(factory);
assert createsPointer(factory);
assert factory.getShape().hasProperty(POINTER_IDENTIFIER);
assert pointer != null;
return factory.newInstance(
pointer);
}
@Override
public boolean isPointer(DynamicObject object) {
return isPointer(object.getShape().getObjectType());
}
private static boolean isPointer(ObjectType objectType) {
return objectType instanceof PointerType;
}
private static boolean createsPointer(DynamicObjectFactory factory) {
return isPointer(factory.getShape().getObjectType());
}
@Override
public jnr.ffi.Pointer getPointer(DynamicObject object) {
assert isPointer(object);
assert object.getShape().hasProperty(POINTER_IDENTIFIER);
return (jnr.ffi.Pointer) POINTER_PROPERTY.get(object, isPointer(object));
}
@Override
public void setPointer(DynamicObject object, jnr.ffi.Pointer value) {
assert isPointer(object);
assert object.getShape().hasProperty(POINTER_IDENTIFIER);
assert value != null;
try {
POINTER_PROPERTY.set(object, value, object.getShape());
} catch (IncompatibleLocationException | FinalLocationException e) {
throw new UnsupportedOperationException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy