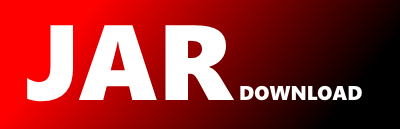
org.jruby.truffle.extra.ffi.PointerPrimitiveNodesFactory Maven / Gradle / Ivy
// CheckStyle: start generated
package org.jruby.truffle.extra.ffi;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NodeFactory;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.internal.SpecializationNode;
import com.oracle.truffle.api.dsl.internal.SpecializedNode;
import com.oracle.truffle.api.dsl.internal.SuppressFBWarnings;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.nodes.UnexpectedResultException;
import com.oracle.truffle.api.object.DynamicObject;
import java.util.Arrays;
import java.util.List;
import org.jruby.truffle.builtins.PrimitiveArrayArgumentsNode;
import org.jruby.truffle.extra.ffi.PointerPrimitiveNodes.PointerAddPrimitiveNode;
import org.jruby.truffle.extra.ffi.PointerPrimitiveNodes.PointerAddressPrimitiveNode;
import org.jruby.truffle.extra.ffi.PointerPrimitiveNodes.PointerAllocatePrimitiveNode;
import org.jruby.truffle.extra.ffi.PointerPrimitiveNodes.PointerFreePrimitiveNode;
import org.jruby.truffle.extra.ffi.PointerPrimitiveNodes.PointerGetAtOffsetPrimitiveNode;
import org.jruby.truffle.extra.ffi.PointerPrimitiveNodes.PointerMallocPrimitiveNode;
import org.jruby.truffle.extra.ffi.PointerPrimitiveNodes.PointerReadIntPrimitiveNode;
import org.jruby.truffle.extra.ffi.PointerPrimitiveNodes.PointerReadPointerPrimitiveNode;
import org.jruby.truffle.extra.ffi.PointerPrimitiveNodes.PointerReadStringPrimitiveNode;
import org.jruby.truffle.extra.ffi.PointerPrimitiveNodes.PointerReadStringToNullPrimitiveNode;
import org.jruby.truffle.extra.ffi.PointerPrimitiveNodes.PointerSetAddressPrimitiveNode;
import org.jruby.truffle.extra.ffi.PointerPrimitiveNodes.PointerSetAtOffsetPrimitiveNode;
import org.jruby.truffle.extra.ffi.PointerPrimitiveNodes.PointerSetAutoreleasePrimitiveNode;
import org.jruby.truffle.extra.ffi.PointerPrimitiveNodes.PointerWriteIntPrimitiveNode;
import org.jruby.truffle.extra.ffi.PointerPrimitiveNodes.PointerWriteStringPrimitiveNode;
import org.jruby.truffle.language.RubyGuards;
import org.jruby.truffle.language.RubyNode;
import org.jruby.truffle.language.RubyTypes;
import org.jruby.truffle.language.RubyTypesGen;
import org.jruby.truffle.platform.RubiniusTypes;
@GeneratedBy(PointerPrimitiveNodes.class)
public final class PointerPrimitiveNodesFactory {
public static List> getFactories() {
return Arrays.asList(PointerAllocatePrimitiveNodeFactory.getInstance(), PointerMallocPrimitiveNodeFactory.getInstance(), PointerFreePrimitiveNodeFactory.getInstance(), PointerSetAddressPrimitiveNodeFactory.getInstance(), PointerAddPrimitiveNodeFactory.getInstance(), PointerReadIntPrimitiveNodeFactory.getInstance(), PointerReadStringPrimitiveNodeFactory.getInstance(), PointerSetAutoreleasePrimitiveNodeFactory.getInstance(), PointerSetAtOffsetPrimitiveNodeFactory.getInstance(), PointerReadPointerPrimitiveNodeFactory.getInstance(), PointerAddressPrimitiveNodeFactory.getInstance(), PointerGetAtOffsetPrimitiveNodeFactory.getInstance(), PointerWriteStringPrimitiveNodeFactory.getInstance(), PointerReadStringToNullPrimitiveNodeFactory.getInstance(), PointerWriteIntPrimitiveNodeFactory.getInstance());
}
@GeneratedBy(PointerAllocatePrimitiveNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class PointerAllocatePrimitiveNodeFactory implements NodeFactory {
private static PointerAllocatePrimitiveNodeFactory pointerAllocatePrimitiveNodeFactoryInstance;
private PointerAllocatePrimitiveNodeFactory() {
}
@Override
public Class getNodeClass() {
return PointerAllocatePrimitiveNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public PointerAllocatePrimitiveNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (pointerAllocatePrimitiveNodeFactoryInstance == null) {
pointerAllocatePrimitiveNodeFactoryInstance = new PointerAllocatePrimitiveNodeFactory();
}
return pointerAllocatePrimitiveNodeFactoryInstance;
}
public static PointerAllocatePrimitiveNode create(RubyNode[] arguments) {
return new PointerAllocatePrimitiveNodeGen(arguments);
}
@GeneratedBy(PointerAllocatePrimitiveNode.class)
public static final class PointerAllocatePrimitiveNodeGen extends PointerAllocatePrimitiveNode {
@Child private RubyNode arguments0_;
@CompilationFinal private boolean seenUnsupported0;
private PointerAllocatePrimitiveNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(ex.getResult());
}
return this.allocate(arguments0Value_);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
private UnsupportedSpecializationException unsupported(Object arguments0Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_}, arguments0Value);
}
}
}
@GeneratedBy(PointerMallocPrimitiveNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class PointerMallocPrimitiveNodeFactory implements NodeFactory {
private static PointerMallocPrimitiveNodeFactory pointerMallocPrimitiveNodeFactoryInstance;
private PointerMallocPrimitiveNodeFactory() {
}
@Override
public Class getNodeClass() {
return PointerMallocPrimitiveNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public PointerMallocPrimitiveNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (pointerMallocPrimitiveNodeFactoryInstance == null) {
pointerMallocPrimitiveNodeFactoryInstance = new PointerMallocPrimitiveNodeFactory();
}
return pointerMallocPrimitiveNodeFactoryInstance;
}
public static PointerMallocPrimitiveNode create(RubyNode[] arguments) {
return new PointerMallocPrimitiveNodeGen(arguments);
}
@GeneratedBy(PointerMallocPrimitiveNode.class)
public static final class PointerMallocPrimitiveNodeGen extends PointerMallocPrimitiveNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@CompilationFinal private Class> arguments1Type_;
@Child private BaseNode_ specialization_;
private PointerMallocPrimitiveNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
return specialization_.executeDynamicObject(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(PointerMallocPrimitiveNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected PointerMallocPrimitiveNodeGen root;
BaseNode_(PointerMallocPrimitiveNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (PointerMallocPrimitiveNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_, root.arguments1_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value, Object arguments1Value) {
return this.executeDynamicObject_((VirtualFrame) frameValue, arguments0Value, arguments1Value);
}
public abstract DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = executeArguments1_(frameValue);
return executeDynamicObject_(frameValue, arguments0Value_, arguments1Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
return (DynamicObject) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject) {
if (RubyTypesGen.isImplicitInteger(arguments1Value)) {
return Malloc0Node_.create(root, arguments1Value);
}
if (RubyTypesGen.isImplicitLong(arguments1Value)) {
return Malloc1Node_.create(root, arguments1Value);
}
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments1_(Frame frameValue) {
Class> arguments1Type_ = root.arguments1Type_;
try {
if (arguments1Type_ == int.class) {
return root.arguments1_.executeInteger((VirtualFrame) frameValue);
} else if (arguments1Type_ == long.class) {
return root.arguments1_.executeLong((VirtualFrame) frameValue);
} else if (arguments1Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments1_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else if (_value instanceof Long) {
_type = long.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments1Type_ = _type;
}
} else {
return root.arguments1_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments1Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(PointerMallocPrimitiveNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(PointerMallocPrimitiveNodeGen root) {
super(root, 2147483647);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
return (DynamicObject) uninitialized(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(PointerMallocPrimitiveNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(PointerMallocPrimitiveNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(PointerMallocPrimitiveNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value, arguments1Value));
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(PointerMallocPrimitiveNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "malloc(DynamicObject, int)", value = PointerMallocPrimitiveNode.class)
private static final class Malloc0Node_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
Malloc0Node_(PointerMallocPrimitiveNodeGen root, Object arguments1Value) {
super(root, 1);
this.arguments1ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments1Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((Malloc0Node_) other).arguments1ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject(frameValue);
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
return getNext().executeDynamicObject_(frameValue, ex.getResult(), arguments1Value);
}
int arguments1Value_;
try {
if (arguments1ImplicitType == int.class) {
arguments1Value_ = root.arguments1_.executeInteger(frameValue);
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitInteger(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeDynamicObject_(frameValue, arguments0Value_, ex.getResult());
}
return root.malloc(arguments0Value_, arguments1Value_);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value, arguments1ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
int arguments1Value_ = RubyTypesGen.asImplicitInteger(arguments1Value, arguments1ImplicitType);
return root.malloc(arguments0Value_, arguments1Value_);
}
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(PointerMallocPrimitiveNodeGen root, Object arguments1Value) {
return new Malloc0Node_(root, arguments1Value);
}
}
@GeneratedBy(methodName = "malloc(DynamicObject, long)", value = PointerMallocPrimitiveNode.class)
private static final class Malloc1Node_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
Malloc1Node_(PointerMallocPrimitiveNodeGen root, Object arguments1Value) {
super(root, 2);
this.arguments1ImplicitType = RubyTypesGen.getImplicitLongClass(arguments1Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((Malloc1Node_) other).arguments1ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject(frameValue);
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
return getNext().executeDynamicObject_(frameValue, ex.getResult(), arguments1Value);
}
long arguments1Value_;
try {
if (arguments1ImplicitType == long.class) {
arguments1Value_ = root.arguments1_.executeLong(frameValue);
} else if (arguments1ImplicitType == int.class) {
arguments1Value_ = RubyTypes.promoteToLong(root.arguments1_.executeInteger(frameValue));
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitLong(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeDynamicObject_(frameValue, arguments0Value_, ex.getResult());
}
return root.malloc(arguments0Value_, arguments1Value_);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitLong(arguments1Value, arguments1ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
long arguments1Value_ = RubyTypesGen.asImplicitLong(arguments1Value, arguments1ImplicitType);
return root.malloc(arguments0Value_, arguments1Value_);
}
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(PointerMallocPrimitiveNodeGen root, Object arguments1Value) {
return new Malloc1Node_(root, arguments1Value);
}
}
}
}
@GeneratedBy(PointerFreePrimitiveNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class PointerFreePrimitiveNodeFactory implements NodeFactory {
private static PointerFreePrimitiveNodeFactory pointerFreePrimitiveNodeFactoryInstance;
private PointerFreePrimitiveNodeFactory() {
}
@Override
public Class getNodeClass() {
return PointerFreePrimitiveNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public PointerFreePrimitiveNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (pointerFreePrimitiveNodeFactoryInstance == null) {
pointerFreePrimitiveNodeFactoryInstance = new PointerFreePrimitiveNodeFactory();
}
return pointerFreePrimitiveNodeFactoryInstance;
}
public static PointerFreePrimitiveNode create(RubyNode[] arguments) {
return new PointerFreePrimitiveNodeGen(arguments);
}
@GeneratedBy(PointerFreePrimitiveNode.class)
public static final class PointerFreePrimitiveNodeGen extends PointerFreePrimitiveNode {
@Child private RubyNode arguments0_;
@CompilationFinal private boolean seenUnsupported0;
private PointerFreePrimitiveNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(ex.getResult());
}
return this.free(arguments0Value_);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
private UnsupportedSpecializationException unsupported(Object arguments0Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_}, arguments0Value);
}
}
}
@GeneratedBy(PointerSetAddressPrimitiveNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class PointerSetAddressPrimitiveNodeFactory implements NodeFactory {
private static PointerSetAddressPrimitiveNodeFactory pointerSetAddressPrimitiveNodeFactoryInstance;
private PointerSetAddressPrimitiveNodeFactory() {
}
@Override
public Class getNodeClass() {
return PointerSetAddressPrimitiveNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public PointerSetAddressPrimitiveNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (pointerSetAddressPrimitiveNodeFactoryInstance == null) {
pointerSetAddressPrimitiveNodeFactoryInstance = new PointerSetAddressPrimitiveNodeFactory();
}
return pointerSetAddressPrimitiveNodeFactoryInstance;
}
public static PointerSetAddressPrimitiveNode create(RubyNode[] arguments) {
return new PointerSetAddressPrimitiveNodeGen(arguments);
}
@GeneratedBy(PointerSetAddressPrimitiveNode.class)
public static final class PointerSetAddressPrimitiveNodeGen extends PointerSetAddressPrimitiveNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@CompilationFinal private Class> arguments1Type_;
@Child private BaseNode_ specialization_;
private PointerSetAddressPrimitiveNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public long executeLong(VirtualFrame frameValue) {
return specialization_.executeLong(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(PointerSetAddressPrimitiveNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected PointerSetAddressPrimitiveNodeGen root;
BaseNode_(PointerSetAddressPrimitiveNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (PointerSetAddressPrimitiveNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_, root.arguments1_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value, Object arguments1Value) {
return this.executeLong_((VirtualFrame) frameValue, arguments0Value, arguments1Value);
}
public abstract long executeLong_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = executeArguments1_(frameValue);
return executeLong_(frameValue, arguments0Value_, arguments1Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public long executeLong(VirtualFrame frameValue) {
return (long) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject) {
if (RubyTypesGen.isImplicitInteger(arguments1Value)) {
return SetAddress0Node_.create(root, arguments1Value);
}
if (RubyTypesGen.isImplicitLong(arguments1Value)) {
return SetAddress1Node_.create(root, arguments1Value);
}
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments1_(Frame frameValue) {
Class> arguments1Type_ = root.arguments1Type_;
try {
if (arguments1Type_ == int.class) {
return root.arguments1_.executeInteger((VirtualFrame) frameValue);
} else if (arguments1Type_ == long.class) {
return root.arguments1_.executeLong((VirtualFrame) frameValue);
} else if (arguments1Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments1_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else if (_value instanceof Long) {
_type = long.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments1Type_ = _type;
}
} else {
return root.arguments1_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments1Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(PointerSetAddressPrimitiveNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(PointerSetAddressPrimitiveNodeGen root) {
super(root, 2147483647);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
return (long) uninitialized(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(PointerSetAddressPrimitiveNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(PointerSetAddressPrimitiveNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(PointerSetAddressPrimitiveNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value, arguments1Value));
}
@Override
public long executeLong(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = executeArguments1_(frameValue);
return getNext().executeLong_(frameValue, arguments0Value_, arguments1Value_);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
return getNext().executeLong_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(PointerSetAddressPrimitiveNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "setAddress(DynamicObject, int)", value = PointerSetAddressPrimitiveNode.class)
private static final class SetAddress0Node_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
SetAddress0Node_(PointerSetAddressPrimitiveNodeGen root, Object arguments1Value) {
super(root, 1);
this.arguments1ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments1Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((SetAddress0Node_) other).arguments1ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeLong(frameValue);
}
@Override
public long executeLong(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
return getNext().executeLong_(frameValue, ex.getResult(), arguments1Value);
}
int arguments1Value_;
try {
if (arguments1ImplicitType == int.class) {
arguments1Value_ = root.arguments1_.executeInteger(frameValue);
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitInteger(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeLong_(frameValue, arguments0Value_, ex.getResult());
}
return root.setAddress(arguments0Value_, arguments1Value_);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value, arguments1ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
int arguments1Value_ = RubyTypesGen.asImplicitInteger(arguments1Value, arguments1ImplicitType);
return root.setAddress(arguments0Value_, arguments1Value_);
}
return getNext().executeLong_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(PointerSetAddressPrimitiveNodeGen root, Object arguments1Value) {
return new SetAddress0Node_(root, arguments1Value);
}
}
@GeneratedBy(methodName = "setAddress(DynamicObject, long)", value = PointerSetAddressPrimitiveNode.class)
private static final class SetAddress1Node_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
SetAddress1Node_(PointerSetAddressPrimitiveNodeGen root, Object arguments1Value) {
super(root, 2);
this.arguments1ImplicitType = RubyTypesGen.getImplicitLongClass(arguments1Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((SetAddress1Node_) other).arguments1ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeLong(frameValue);
}
@Override
public long executeLong(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
return getNext().executeLong_(frameValue, ex.getResult(), arguments1Value);
}
long arguments1Value_;
try {
if (arguments1ImplicitType == long.class) {
arguments1Value_ = root.arguments1_.executeLong(frameValue);
} else if (arguments1ImplicitType == int.class) {
arguments1Value_ = RubyTypes.promoteToLong(root.arguments1_.executeInteger(frameValue));
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitLong(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeLong_(frameValue, arguments0Value_, ex.getResult());
}
return root.setAddress(arguments0Value_, arguments1Value_);
}
@Override
public long executeLong_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitLong(arguments1Value, arguments1ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
long arguments1Value_ = RubyTypesGen.asImplicitLong(arguments1Value, arguments1ImplicitType);
return root.setAddress(arguments0Value_, arguments1Value_);
}
return getNext().executeLong_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(PointerSetAddressPrimitiveNodeGen root, Object arguments1Value) {
return new SetAddress1Node_(root, arguments1Value);
}
}
}
}
@GeneratedBy(PointerAddPrimitiveNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class PointerAddPrimitiveNodeFactory implements NodeFactory {
private static PointerAddPrimitiveNodeFactory pointerAddPrimitiveNodeFactoryInstance;
private PointerAddPrimitiveNodeFactory() {
}
@Override
public Class getNodeClass() {
return PointerAddPrimitiveNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public PointerAddPrimitiveNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (pointerAddPrimitiveNodeFactoryInstance == null) {
pointerAddPrimitiveNodeFactoryInstance = new PointerAddPrimitiveNodeFactory();
}
return pointerAddPrimitiveNodeFactoryInstance;
}
public static PointerAddPrimitiveNode create(RubyNode[] arguments) {
return new PointerAddPrimitiveNodeGen(arguments);
}
@GeneratedBy(PointerAddPrimitiveNode.class)
public static final class PointerAddPrimitiveNodeGen extends PointerAddPrimitiveNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@CompilationFinal private Class> arguments1Type_;
@Child private BaseNode_ specialization_;
private PointerAddPrimitiveNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
return specialization_.executeDynamicObject(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(PointerAddPrimitiveNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected PointerAddPrimitiveNodeGen root;
BaseNode_(PointerAddPrimitiveNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (PointerAddPrimitiveNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_, root.arguments1_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value, Object arguments1Value) {
return this.executeDynamicObject_((VirtualFrame) frameValue, arguments0Value, arguments1Value);
}
public abstract DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = executeArguments1_(frameValue);
return executeDynamicObject_(frameValue, arguments0Value_, arguments1Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
return (DynamicObject) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject) {
if (RubyTypesGen.isImplicitInteger(arguments1Value)) {
return Add0Node_.create(root, arguments1Value);
}
if (RubyTypesGen.isImplicitLong(arguments1Value)) {
return Add1Node_.create(root, arguments1Value);
}
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments1_(Frame frameValue) {
Class> arguments1Type_ = root.arguments1Type_;
try {
if (arguments1Type_ == int.class) {
return root.arguments1_.executeInteger((VirtualFrame) frameValue);
} else if (arguments1Type_ == long.class) {
return root.arguments1_.executeLong((VirtualFrame) frameValue);
} else if (arguments1Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments1_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else if (_value instanceof Long) {
_type = long.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments1Type_ = _type;
}
} else {
return root.arguments1_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments1Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(PointerAddPrimitiveNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(PointerAddPrimitiveNodeGen root) {
super(root, 2147483647);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
return (DynamicObject) uninitialized(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(PointerAddPrimitiveNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(PointerAddPrimitiveNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(PointerAddPrimitiveNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value, arguments1Value));
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(PointerAddPrimitiveNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "add(DynamicObject, int)", value = PointerAddPrimitiveNode.class)
private static final class Add0Node_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
Add0Node_(PointerAddPrimitiveNodeGen root, Object arguments1Value) {
super(root, 1);
this.arguments1ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments1Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((Add0Node_) other).arguments1ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject(frameValue);
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
return getNext().executeDynamicObject_(frameValue, ex.getResult(), arguments1Value);
}
int arguments1Value_;
try {
if (arguments1ImplicitType == int.class) {
arguments1Value_ = root.arguments1_.executeInteger(frameValue);
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitInteger(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeDynamicObject_(frameValue, arguments0Value_, ex.getResult());
}
return root.add(arguments0Value_, arguments1Value_);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value, arguments1ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
int arguments1Value_ = RubyTypesGen.asImplicitInteger(arguments1Value, arguments1ImplicitType);
return root.add(arguments0Value_, arguments1Value_);
}
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(PointerAddPrimitiveNodeGen root, Object arguments1Value) {
return new Add0Node_(root, arguments1Value);
}
}
@GeneratedBy(methodName = "add(DynamicObject, long)", value = PointerAddPrimitiveNode.class)
private static final class Add1Node_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
Add1Node_(PointerAddPrimitiveNodeGen root, Object arguments1Value) {
super(root, 2);
this.arguments1ImplicitType = RubyTypesGen.getImplicitLongClass(arguments1Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((Add1Node_) other).arguments1ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject(frameValue);
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
return getNext().executeDynamicObject_(frameValue, ex.getResult(), arguments1Value);
}
long arguments1Value_;
try {
if (arguments1ImplicitType == long.class) {
arguments1Value_ = root.arguments1_.executeLong(frameValue);
} else if (arguments1ImplicitType == int.class) {
arguments1Value_ = RubyTypes.promoteToLong(root.arguments1_.executeInteger(frameValue));
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitLong(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeDynamicObject_(frameValue, arguments0Value_, ex.getResult());
}
return root.add(arguments0Value_, arguments1Value_);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitLong(arguments1Value, arguments1ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
long arguments1Value_ = RubyTypesGen.asImplicitLong(arguments1Value, arguments1ImplicitType);
return root.add(arguments0Value_, arguments1Value_);
}
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(PointerAddPrimitiveNodeGen root, Object arguments1Value) {
return new Add1Node_(root, arguments1Value);
}
}
}
}
@GeneratedBy(PointerReadIntPrimitiveNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class PointerReadIntPrimitiveNodeFactory implements NodeFactory {
private static PointerReadIntPrimitiveNodeFactory pointerReadIntPrimitiveNodeFactoryInstance;
private PointerReadIntPrimitiveNodeFactory() {
}
@Override
public Class getNodeClass() {
return PointerReadIntPrimitiveNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public PointerReadIntPrimitiveNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (pointerReadIntPrimitiveNodeFactoryInstance == null) {
pointerReadIntPrimitiveNodeFactoryInstance = new PointerReadIntPrimitiveNodeFactory();
}
return pointerReadIntPrimitiveNodeFactoryInstance;
}
public static PointerReadIntPrimitiveNode create(RubyNode[] arguments) {
return new PointerReadIntPrimitiveNodeGen(arguments);
}
@GeneratedBy(PointerReadIntPrimitiveNode.class)
public static final class PointerReadIntPrimitiveNodeGen extends PointerReadIntPrimitiveNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@CompilationFinal private boolean seenUnsupported0;
private PointerReadIntPrimitiveNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeInteger(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
executeInteger(frameValue);
return;
}
@Override
public int executeInteger(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = arguments1_.execute(frameValue);
throw unsupported(ex.getResult(), arguments1Value);
}
boolean arguments1Value_;
try {
arguments1Value_ = arguments1_.executeBoolean(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(arguments0Value_, ex.getResult());
}
if ((this.isSigned(arguments1Value_))) {
return this.readInt(arguments0Value_, arguments1Value_);
}
throw unsupported(arguments0Value_, arguments1Value_);
}
private UnsupportedSpecializationException unsupported(Object arguments0Value, Object arguments1Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_, arguments1_}, arguments0Value, arguments1Value);
}
}
}
@GeneratedBy(PointerReadStringPrimitiveNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class PointerReadStringPrimitiveNodeFactory implements NodeFactory {
private static PointerReadStringPrimitiveNodeFactory pointerReadStringPrimitiveNodeFactoryInstance;
private PointerReadStringPrimitiveNodeFactory() {
}
@Override
public Class getNodeClass() {
return PointerReadStringPrimitiveNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public PointerReadStringPrimitiveNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (pointerReadStringPrimitiveNodeFactoryInstance == null) {
pointerReadStringPrimitiveNodeFactoryInstance = new PointerReadStringPrimitiveNodeFactory();
}
return pointerReadStringPrimitiveNodeFactoryInstance;
}
public static PointerReadStringPrimitiveNode create(RubyNode[] arguments) {
return new PointerReadStringPrimitiveNodeGen(arguments);
}
@GeneratedBy(PointerReadStringPrimitiveNode.class)
public static final class PointerReadStringPrimitiveNodeGen extends PointerReadStringPrimitiveNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@CompilationFinal private Class> arguments1Type_;
@Child private BaseNode_ specialization_;
private PointerReadStringPrimitiveNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
return specialization_.executeDynamicObject(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(PointerReadStringPrimitiveNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected PointerReadStringPrimitiveNodeGen root;
BaseNode_(PointerReadStringPrimitiveNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (PointerReadStringPrimitiveNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_, root.arguments1_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value, Object arguments1Value) {
return this.executeDynamicObject_((VirtualFrame) frameValue, arguments0Value, arguments1Value);
}
public abstract DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = executeArguments1_(frameValue);
return executeDynamicObject_(frameValue, arguments0Value_, arguments1Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
return (DynamicObject) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value)) {
return ReadStringNode_.create(root, arguments1Value);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments1_(Frame frameValue) {
Class> arguments1Type_ = root.arguments1Type_;
try {
if (arguments1Type_ == int.class) {
return root.arguments1_.executeInteger((VirtualFrame) frameValue);
} else if (arguments1Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments1_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments1Type_ = _type;
}
} else {
return root.arguments1_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments1Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(PointerReadStringPrimitiveNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(PointerReadStringPrimitiveNodeGen root) {
super(root, 2147483647);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
return (DynamicObject) uninitialized(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(PointerReadStringPrimitiveNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(PointerReadStringPrimitiveNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(PointerReadStringPrimitiveNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value, arguments1Value));
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(PointerReadStringPrimitiveNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "readString(DynamicObject, int)", value = PointerReadStringPrimitiveNode.class)
private static final class ReadStringNode_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
ReadStringNode_(PointerReadStringPrimitiveNodeGen root, Object arguments1Value) {
super(root, 1);
this.arguments1ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments1Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((ReadStringNode_) other).arguments1ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject(frameValue);
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
return getNext().executeDynamicObject_(frameValue, ex.getResult(), arguments1Value);
}
int arguments1Value_;
try {
if (arguments1ImplicitType == int.class) {
arguments1Value_ = root.arguments1_.executeInteger(frameValue);
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitInteger(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeDynamicObject_(frameValue, arguments0Value_, ex.getResult());
}
return root.readString(arguments0Value_, arguments1Value_);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value, arguments1ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
int arguments1Value_ = RubyTypesGen.asImplicitInteger(arguments1Value, arguments1ImplicitType);
return root.readString(arguments0Value_, arguments1Value_);
}
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(PointerReadStringPrimitiveNodeGen root, Object arguments1Value) {
return new ReadStringNode_(root, arguments1Value);
}
}
}
}
@GeneratedBy(PointerSetAutoreleasePrimitiveNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class PointerSetAutoreleasePrimitiveNodeFactory implements NodeFactory {
private static PointerSetAutoreleasePrimitiveNodeFactory pointerSetAutoreleasePrimitiveNodeFactoryInstance;
private PointerSetAutoreleasePrimitiveNodeFactory() {
}
@Override
public Class getNodeClass() {
return PointerSetAutoreleasePrimitiveNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public PointerSetAutoreleasePrimitiveNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (pointerSetAutoreleasePrimitiveNodeFactoryInstance == null) {
pointerSetAutoreleasePrimitiveNodeFactoryInstance = new PointerSetAutoreleasePrimitiveNodeFactory();
}
return pointerSetAutoreleasePrimitiveNodeFactoryInstance;
}
public static PointerSetAutoreleasePrimitiveNode create(RubyNode[] arguments) {
return new PointerSetAutoreleasePrimitiveNodeGen(arguments);
}
@GeneratedBy(PointerSetAutoreleasePrimitiveNode.class)
public static final class PointerSetAutoreleasePrimitiveNodeGen extends PointerSetAutoreleasePrimitiveNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@CompilationFinal private boolean seenUnsupported0;
private PointerSetAutoreleasePrimitiveNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeBoolean(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
executeBoolean(frameValue);
return;
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = arguments1_.execute(frameValue);
throw unsupported(ex.getResult(), arguments1Value);
}
boolean arguments1Value_;
try {
arguments1Value_ = arguments1_.executeBoolean(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(arguments0Value_, ex.getResult());
}
return this.setAutorelease(arguments0Value_, arguments1Value_);
}
private UnsupportedSpecializationException unsupported(Object arguments0Value, Object arguments1Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_, arguments1_}, arguments0Value, arguments1Value);
}
}
}
@GeneratedBy(PointerSetAtOffsetPrimitiveNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class PointerSetAtOffsetPrimitiveNodeFactory implements NodeFactory {
private static PointerSetAtOffsetPrimitiveNodeFactory pointerSetAtOffsetPrimitiveNodeFactoryInstance;
private PointerSetAtOffsetPrimitiveNodeFactory() {
}
@Override
public Class getNodeClass() {
return PointerSetAtOffsetPrimitiveNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class, RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public PointerSetAtOffsetPrimitiveNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (pointerSetAtOffsetPrimitiveNodeFactoryInstance == null) {
pointerSetAtOffsetPrimitiveNodeFactoryInstance = new PointerSetAtOffsetPrimitiveNodeFactory();
}
return pointerSetAtOffsetPrimitiveNodeFactoryInstance;
}
public static PointerSetAtOffsetPrimitiveNode create(RubyNode[] arguments) {
return new PointerSetAtOffsetPrimitiveNodeGen(arguments);
}
@GeneratedBy(PointerSetAtOffsetPrimitiveNode.class)
@SuppressFBWarnings("SA_LOCAL_SELF_COMPARISON")
public static final class PointerSetAtOffsetPrimitiveNodeGen extends PointerSetAtOffsetPrimitiveNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@Child private RubyNode arguments2_;
@Child private RubyNode arguments3_;
@CompilationFinal private Class> arguments1Type_;
@CompilationFinal private Class> arguments2Type_;
@CompilationFinal private Class> arguments3Type_;
@Child private BaseNode_ specialization_;
private PointerSetAtOffsetPrimitiveNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.arguments2_ = arguments != null && 2 < arguments.length ? arguments[2] : null;
this.arguments3_ = arguments != null && 3 < arguments.length ? arguments[3] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public int executeInteger(VirtualFrame frameValue) throws UnexpectedResultException {
return specialization_.executeInt(frameValue);
}
@Override
public long executeLong(VirtualFrame frameValue) throws UnexpectedResultException {
return specialization_.executeLong(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
private static int expectInteger(Object value) throws UnexpectedResultException {
if (value instanceof Integer) {
return (int) value;
}
throw new UnexpectedResultException(value);
}
private static long expectLong(Object value) throws UnexpectedResultException {
if (value instanceof Long) {
return (long) value;
}
throw new UnexpectedResultException(value);
}
@GeneratedBy(PointerSetAtOffsetPrimitiveNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected PointerSetAtOffsetPrimitiveNodeGen root;
BaseNode_(PointerSetAtOffsetPrimitiveNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (PointerSetAtOffsetPrimitiveNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_, root.arguments1_, root.arguments2_, root.arguments3_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value) {
return this.execute_((VirtualFrame) frameValue, arguments0Value, arguments1Value, arguments2Value, arguments3Value);
}
public abstract Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = executeArguments1_(frameValue);
Object arguments2Value_ = executeArguments2_(frameValue);
Object arguments3Value_ = executeArguments3_(frameValue);
return execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public int executeInt(VirtualFrame frameValue) throws UnexpectedResultException {
return expectInteger(execute(frameValue));
}
public long executeLong(VirtualFrame frameValue) throws UnexpectedResultException {
return expectLong(execute(frameValue));
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value) && RubyTypesGen.isImplicitInteger(arguments2Value)) {
int arguments2Value_ = RubyTypesGen.asImplicitInteger(arguments2Value);
if (RubyTypesGen.isImplicitInteger(arguments3Value)) {
if ((arguments2Value_ == RubiniusTypes.TYPE_INT)) {
return SetAtOffsetIntNode_.create(root, arguments1Value, arguments2Value, arguments3Value);
}
}
if (RubyTypesGen.isImplicitLong(arguments3Value)) {
if ((arguments2Value_ == RubiniusTypes.TYPE_LONG)) {
return SetAtOffsetLongNode_.create(root, arguments1Value, arguments2Value, arguments3Value);
}
if ((arguments2Value_ == RubiniusTypes.TYPE_ULONG)) {
return SetAtOffsetULongNode_.create(root, arguments1Value, arguments2Value, arguments3Value);
}
if ((arguments2Value_ == RubiniusTypes.TYPE_ULL)) {
return SetAtOffsetULLNode_.create(root, arguments1Value, arguments2Value, arguments3Value);
}
}
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments1_(Frame frameValue) {
Class> arguments1Type_ = root.arguments1Type_;
try {
if (arguments1Type_ == int.class) {
return root.arguments1_.executeInteger((VirtualFrame) frameValue);
} else if (arguments1Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments1_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments1Type_ = _type;
}
} else {
return root.arguments1_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments1Type_ = Object.class;
return ex.getResult();
}
}
protected final Object executeArguments2_(Frame frameValue) {
Class> arguments2Type_ = root.arguments2Type_;
try {
if (arguments2Type_ == int.class) {
return root.arguments2_.executeInteger((VirtualFrame) frameValue);
} else if (arguments2Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments2_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments2Type_ = _type;
}
} else {
return root.arguments2_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments2Type_ = Object.class;
return ex.getResult();
}
}
protected final long executeArguments3Long_(Frame frameValue, Class> arguments3ImplicitType) throws UnexpectedResultException {
if (arguments3ImplicitType == long.class) {
return root.arguments3_.executeLong((VirtualFrame) frameValue);
} else if (arguments3ImplicitType == int.class) {
return RubyTypes.promoteToLong(root.arguments3_.executeInteger((VirtualFrame) frameValue));
} else {
Object arguments3Value_ = executeArguments3_(frameValue);
return RubyTypesGen.expectImplicitLong(arguments3Value_, arguments3ImplicitType);
}
}
protected final Object executeArguments3_(Frame frameValue) {
Class> arguments3Type_ = root.arguments3Type_;
try {
if (arguments3Type_ == int.class) {
return root.arguments3_.executeInteger((VirtualFrame) frameValue);
} else if (arguments3Type_ == long.class) {
return root.arguments3_.executeLong((VirtualFrame) frameValue);
} else if (arguments3Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments3_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else if (_value instanceof Long) {
_type = long.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments3Type_ = _type;
}
} else {
return root.arguments3_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments3Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(PointerSetAtOffsetPrimitiveNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(PointerSetAtOffsetPrimitiveNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value) {
return uninitialized(frameValue, arguments0Value, arguments1Value, arguments2Value, arguments3Value);
}
static BaseNode_ create(PointerSetAtOffsetPrimitiveNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(PointerSetAtOffsetPrimitiveNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(PointerSetAtOffsetPrimitiveNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value, arguments1Value, arguments2Value, arguments3Value));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value) {
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value, arguments3Value);
}
static BaseNode_ create(PointerSetAtOffsetPrimitiveNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "setAtOffsetInt(DynamicObject, int, int, int)", value = PointerSetAtOffsetPrimitiveNode.class)
private static final class SetAtOffsetIntNode_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
private final Class> arguments2ImplicitType;
private final Class> arguments3ImplicitType;
SetAtOffsetIntNode_(PointerSetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value, Object arguments3Value) {
super(root, 1);
this.arguments1ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments1Value);
this.arguments2ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments2Value);
this.arguments3ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments3Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((SetAtOffsetIntNode_) other).arguments1ImplicitType && this.arguments2ImplicitType == ((SetAtOffsetIntNode_) other).arguments2ImplicitType && this.arguments3ImplicitType == ((SetAtOffsetIntNode_) other).arguments3ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
try {
return executeInt(frameValue);
} catch (UnexpectedResultException ex) {
return ex.getResult();
}
}
@Override
public int executeInt(VirtualFrame frameValue) throws UnexpectedResultException {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
Object arguments2Value = executeArguments2_(frameValue);
Object arguments3Value = executeArguments3_(frameValue);
return expectInteger(getNext().execute_(frameValue, ex.getResult(), arguments1Value, arguments2Value, arguments3Value));
}
int arguments1Value_;
try {
if (arguments1ImplicitType == int.class) {
arguments1Value_ = root.arguments1_.executeInteger(frameValue);
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitInteger(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
Object arguments2Value = executeArguments2_(frameValue);
Object arguments3Value = executeArguments3_(frameValue);
return expectInteger(getNext().execute_(frameValue, arguments0Value_, ex.getResult(), arguments2Value, arguments3Value));
}
int arguments2Value_;
try {
if (arguments2ImplicitType == int.class) {
arguments2Value_ = root.arguments2_.executeInteger(frameValue);
} else {
Object arguments2Value__ = executeArguments2_(frameValue);
arguments2Value_ = RubyTypesGen.expectImplicitInteger(arguments2Value__, arguments2ImplicitType);
}
} catch (UnexpectedResultException ex) {
Object arguments3Value = executeArguments3_(frameValue);
return expectInteger(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, ex.getResult(), arguments3Value));
}
int arguments3Value_;
try {
if (arguments3ImplicitType == int.class) {
arguments3Value_ = root.arguments3_.executeInteger(frameValue);
} else {
Object arguments3Value__ = executeArguments3_(frameValue);
arguments3Value_ = RubyTypesGen.expectImplicitInteger(arguments3Value__, arguments3ImplicitType);
}
} catch (UnexpectedResultException ex) {
return expectInteger(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, ex.getResult()));
}
if ((arguments2Value_ == RubiniusTypes.TYPE_INT)) {
return root.setAtOffsetInt(arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_);
}
return expectInteger(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value, arguments1ImplicitType) && RubyTypesGen.isImplicitInteger(arguments2Value, arguments2ImplicitType) && RubyTypesGen.isImplicitInteger(arguments3Value, arguments3ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
int arguments1Value_ = RubyTypesGen.asImplicitInteger(arguments1Value, arguments1ImplicitType);
int arguments2Value_ = RubyTypesGen.asImplicitInteger(arguments2Value, arguments2ImplicitType);
int arguments3Value_ = RubyTypesGen.asImplicitInteger(arguments3Value, arguments3ImplicitType);
if ((arguments2Value_ == RubiniusTypes.TYPE_INT)) {
return root.setAtOffsetInt(arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value, arguments3Value);
}
static BaseNode_ create(PointerSetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value, Object arguments3Value) {
return new SetAtOffsetIntNode_(root, arguments1Value, arguments2Value, arguments3Value);
}
}
@GeneratedBy(methodName = "setAtOffsetLong(DynamicObject, int, int, long)", value = PointerSetAtOffsetPrimitiveNode.class)
private static final class SetAtOffsetLongNode_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
private final Class> arguments2ImplicitType;
private final Class> arguments3ImplicitType;
SetAtOffsetLongNode_(PointerSetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value, Object arguments3Value) {
super(root, 2);
this.arguments1ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments1Value);
this.arguments2ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments2Value);
this.arguments3ImplicitType = RubyTypesGen.getImplicitLongClass(arguments3Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((SetAtOffsetLongNode_) other).arguments1ImplicitType && this.arguments2ImplicitType == ((SetAtOffsetLongNode_) other).arguments2ImplicitType && this.arguments3ImplicitType == ((SetAtOffsetLongNode_) other).arguments3ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
try {
return executeLong(frameValue);
} catch (UnexpectedResultException ex) {
return ex.getResult();
}
}
@Override
public long executeLong(VirtualFrame frameValue) throws UnexpectedResultException {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
Object arguments2Value = executeArguments2_(frameValue);
Object arguments3Value = executeArguments3_(frameValue);
return expectLong(getNext().execute_(frameValue, ex.getResult(), arguments1Value, arguments2Value, arguments3Value));
}
int arguments1Value_;
try {
if (arguments1ImplicitType == int.class) {
arguments1Value_ = root.arguments1_.executeInteger(frameValue);
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitInteger(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
Object arguments2Value = executeArguments2_(frameValue);
Object arguments3Value = executeArguments3_(frameValue);
return expectLong(getNext().execute_(frameValue, arguments0Value_, ex.getResult(), arguments2Value, arguments3Value));
}
int arguments2Value_;
try {
if (arguments2ImplicitType == int.class) {
arguments2Value_ = root.arguments2_.executeInteger(frameValue);
} else {
Object arguments2Value__ = executeArguments2_(frameValue);
arguments2Value_ = RubyTypesGen.expectImplicitInteger(arguments2Value__, arguments2ImplicitType);
}
} catch (UnexpectedResultException ex) {
Object arguments3Value = executeArguments3_(frameValue);
return expectLong(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, ex.getResult(), arguments3Value));
}
long arguments3Value_;
try {
arguments3Value_ = executeArguments3Long_(frameValue, arguments3ImplicitType);
} catch (UnexpectedResultException ex) {
return expectLong(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, ex.getResult()));
}
if ((arguments2Value_ == RubiniusTypes.TYPE_LONG)) {
return root.setAtOffsetLong(arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_);
}
return expectLong(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value, arguments1ImplicitType) && RubyTypesGen.isImplicitInteger(arguments2Value, arguments2ImplicitType) && RubyTypesGen.isImplicitLong(arguments3Value, arguments3ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
int arguments1Value_ = RubyTypesGen.asImplicitInteger(arguments1Value, arguments1ImplicitType);
int arguments2Value_ = RubyTypesGen.asImplicitInteger(arguments2Value, arguments2ImplicitType);
long arguments3Value_ = RubyTypesGen.asImplicitLong(arguments3Value, arguments3ImplicitType);
if ((arguments2Value_ == RubiniusTypes.TYPE_LONG)) {
return root.setAtOffsetLong(arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value, arguments3Value);
}
static BaseNode_ create(PointerSetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value, Object arguments3Value) {
return new SetAtOffsetLongNode_(root, arguments1Value, arguments2Value, arguments3Value);
}
}
@GeneratedBy(methodName = "setAtOffsetULong(DynamicObject, int, int, long)", value = PointerSetAtOffsetPrimitiveNode.class)
private static final class SetAtOffsetULongNode_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
private final Class> arguments2ImplicitType;
private final Class> arguments3ImplicitType;
SetAtOffsetULongNode_(PointerSetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value, Object arguments3Value) {
super(root, 3);
this.arguments1ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments1Value);
this.arguments2ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments2Value);
this.arguments3ImplicitType = RubyTypesGen.getImplicitLongClass(arguments3Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((SetAtOffsetULongNode_) other).arguments1ImplicitType && this.arguments2ImplicitType == ((SetAtOffsetULongNode_) other).arguments2ImplicitType && this.arguments3ImplicitType == ((SetAtOffsetULongNode_) other).arguments3ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
try {
return executeLong(frameValue);
} catch (UnexpectedResultException ex) {
return ex.getResult();
}
}
@Override
public long executeLong(VirtualFrame frameValue) throws UnexpectedResultException {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
Object arguments2Value = executeArguments2_(frameValue);
Object arguments3Value = executeArguments3_(frameValue);
return expectLong(getNext().execute_(frameValue, ex.getResult(), arguments1Value, arguments2Value, arguments3Value));
}
int arguments1Value_;
try {
if (arguments1ImplicitType == int.class) {
arguments1Value_ = root.arguments1_.executeInteger(frameValue);
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitInteger(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
Object arguments2Value = executeArguments2_(frameValue);
Object arguments3Value = executeArguments3_(frameValue);
return expectLong(getNext().execute_(frameValue, arguments0Value_, ex.getResult(), arguments2Value, arguments3Value));
}
int arguments2Value_;
try {
if (arguments2ImplicitType == int.class) {
arguments2Value_ = root.arguments2_.executeInteger(frameValue);
} else {
Object arguments2Value__ = executeArguments2_(frameValue);
arguments2Value_ = RubyTypesGen.expectImplicitInteger(arguments2Value__, arguments2ImplicitType);
}
} catch (UnexpectedResultException ex) {
Object arguments3Value = executeArguments3_(frameValue);
return expectLong(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, ex.getResult(), arguments3Value));
}
long arguments3Value_;
try {
arguments3Value_ = executeArguments3Long_(frameValue, arguments3ImplicitType);
} catch (UnexpectedResultException ex) {
return expectLong(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, ex.getResult()));
}
if ((arguments2Value_ == RubiniusTypes.TYPE_ULONG)) {
return root.setAtOffsetULong(arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_);
}
return expectLong(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value, arguments1ImplicitType) && RubyTypesGen.isImplicitInteger(arguments2Value, arguments2ImplicitType) && RubyTypesGen.isImplicitLong(arguments3Value, arguments3ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
int arguments1Value_ = RubyTypesGen.asImplicitInteger(arguments1Value, arguments1ImplicitType);
int arguments2Value_ = RubyTypesGen.asImplicitInteger(arguments2Value, arguments2ImplicitType);
long arguments3Value_ = RubyTypesGen.asImplicitLong(arguments3Value, arguments3ImplicitType);
if ((arguments2Value_ == RubiniusTypes.TYPE_ULONG)) {
return root.setAtOffsetULong(arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value, arguments3Value);
}
static BaseNode_ create(PointerSetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value, Object arguments3Value) {
return new SetAtOffsetULongNode_(root, arguments1Value, arguments2Value, arguments3Value);
}
}
@GeneratedBy(methodName = "setAtOffsetULL(DynamicObject, int, int, long)", value = PointerSetAtOffsetPrimitiveNode.class)
private static final class SetAtOffsetULLNode_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
private final Class> arguments2ImplicitType;
private final Class> arguments3ImplicitType;
SetAtOffsetULLNode_(PointerSetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value, Object arguments3Value) {
super(root, 4);
this.arguments1ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments1Value);
this.arguments2ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments2Value);
this.arguments3ImplicitType = RubyTypesGen.getImplicitLongClass(arguments3Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((SetAtOffsetULLNode_) other).arguments1ImplicitType && this.arguments2ImplicitType == ((SetAtOffsetULLNode_) other).arguments2ImplicitType && this.arguments3ImplicitType == ((SetAtOffsetULLNode_) other).arguments3ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
try {
return executeLong(frameValue);
} catch (UnexpectedResultException ex) {
return ex.getResult();
}
}
@Override
public long executeLong(VirtualFrame frameValue) throws UnexpectedResultException {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
Object arguments2Value = executeArguments2_(frameValue);
Object arguments3Value = executeArguments3_(frameValue);
return expectLong(getNext().execute_(frameValue, ex.getResult(), arguments1Value, arguments2Value, arguments3Value));
}
int arguments1Value_;
try {
if (arguments1ImplicitType == int.class) {
arguments1Value_ = root.arguments1_.executeInteger(frameValue);
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitInteger(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
Object arguments2Value = executeArguments2_(frameValue);
Object arguments3Value = executeArguments3_(frameValue);
return expectLong(getNext().execute_(frameValue, arguments0Value_, ex.getResult(), arguments2Value, arguments3Value));
}
int arguments2Value_;
try {
if (arguments2ImplicitType == int.class) {
arguments2Value_ = root.arguments2_.executeInteger(frameValue);
} else {
Object arguments2Value__ = executeArguments2_(frameValue);
arguments2Value_ = RubyTypesGen.expectImplicitInteger(arguments2Value__, arguments2ImplicitType);
}
} catch (UnexpectedResultException ex) {
Object arguments3Value = executeArguments3_(frameValue);
return expectLong(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, ex.getResult(), arguments3Value));
}
long arguments3Value_;
try {
arguments3Value_ = executeArguments3Long_(frameValue, arguments3ImplicitType);
} catch (UnexpectedResultException ex) {
return expectLong(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, ex.getResult()));
}
if ((arguments2Value_ == RubiniusTypes.TYPE_ULL)) {
return root.setAtOffsetULL(arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_);
}
return expectLong(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value, arguments1ImplicitType) && RubyTypesGen.isImplicitInteger(arguments2Value, arguments2ImplicitType) && RubyTypesGen.isImplicitLong(arguments3Value, arguments3ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
int arguments1Value_ = RubyTypesGen.asImplicitInteger(arguments1Value, arguments1ImplicitType);
int arguments2Value_ = RubyTypesGen.asImplicitInteger(arguments2Value, arguments2ImplicitType);
long arguments3Value_ = RubyTypesGen.asImplicitLong(arguments3Value, arguments3ImplicitType);
if ((arguments2Value_ == RubiniusTypes.TYPE_ULL)) {
return root.setAtOffsetULL(arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value, arguments3Value);
}
static BaseNode_ create(PointerSetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value, Object arguments3Value) {
return new SetAtOffsetULLNode_(root, arguments1Value, arguments2Value, arguments3Value);
}
}
}
}
@GeneratedBy(PointerReadPointerPrimitiveNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class PointerReadPointerPrimitiveNodeFactory implements NodeFactory {
private static PointerReadPointerPrimitiveNodeFactory pointerReadPointerPrimitiveNodeFactoryInstance;
private PointerReadPointerPrimitiveNodeFactory() {
}
@Override
public Class getNodeClass() {
return PointerReadPointerPrimitiveNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public PointerReadPointerPrimitiveNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (pointerReadPointerPrimitiveNodeFactoryInstance == null) {
pointerReadPointerPrimitiveNodeFactoryInstance = new PointerReadPointerPrimitiveNodeFactory();
}
return pointerReadPointerPrimitiveNodeFactoryInstance;
}
public static PointerReadPointerPrimitiveNode create(RubyNode[] arguments) {
return new PointerReadPointerPrimitiveNodeGen(arguments);
}
@GeneratedBy(PointerReadPointerPrimitiveNode.class)
public static final class PointerReadPointerPrimitiveNodeGen extends PointerReadPointerPrimitiveNode {
@Child private RubyNode arguments0_;
@CompilationFinal private boolean seenUnsupported0;
private PointerReadPointerPrimitiveNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(ex.getResult());
}
return this.readPointer(arguments0Value_);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
private UnsupportedSpecializationException unsupported(Object arguments0Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_}, arguments0Value);
}
}
}
@GeneratedBy(PointerAddressPrimitiveNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class PointerAddressPrimitiveNodeFactory implements NodeFactory {
private static PointerAddressPrimitiveNodeFactory pointerAddressPrimitiveNodeFactoryInstance;
private PointerAddressPrimitiveNodeFactory() {
}
@Override
public Class getNodeClass() {
return PointerAddressPrimitiveNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public PointerAddressPrimitiveNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (pointerAddressPrimitiveNodeFactoryInstance == null) {
pointerAddressPrimitiveNodeFactoryInstance = new PointerAddressPrimitiveNodeFactory();
}
return pointerAddressPrimitiveNodeFactoryInstance;
}
public static PointerAddressPrimitiveNode create(RubyNode[] arguments) {
return new PointerAddressPrimitiveNodeGen(arguments);
}
@GeneratedBy(PointerAddressPrimitiveNode.class)
public static final class PointerAddressPrimitiveNodeGen extends PointerAddressPrimitiveNode {
@Child private RubyNode arguments0_;
@CompilationFinal private boolean seenUnsupported0;
private PointerAddressPrimitiveNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeLong(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
executeLong(frameValue);
return;
}
@Override
public long executeLong(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(ex.getResult());
}
return this.address(arguments0Value_);
}
private UnsupportedSpecializationException unsupported(Object arguments0Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_}, arguments0Value);
}
}
}
@GeneratedBy(PointerGetAtOffsetPrimitiveNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class PointerGetAtOffsetPrimitiveNodeFactory implements NodeFactory {
private static PointerGetAtOffsetPrimitiveNodeFactory pointerGetAtOffsetPrimitiveNodeFactoryInstance;
private PointerGetAtOffsetPrimitiveNodeFactory() {
}
@Override
public Class getNodeClass() {
return PointerGetAtOffsetPrimitiveNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public PointerGetAtOffsetPrimitiveNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (pointerGetAtOffsetPrimitiveNodeFactoryInstance == null) {
pointerGetAtOffsetPrimitiveNodeFactoryInstance = new PointerGetAtOffsetPrimitiveNodeFactory();
}
return pointerGetAtOffsetPrimitiveNodeFactoryInstance;
}
public static PointerGetAtOffsetPrimitiveNode create(RubyNode[] arguments) {
return new PointerGetAtOffsetPrimitiveNodeGen(arguments);
}
@GeneratedBy(PointerGetAtOffsetPrimitiveNode.class)
@SuppressFBWarnings("SA_LOCAL_SELF_COMPARISON")
public static final class PointerGetAtOffsetPrimitiveNodeGen extends PointerGetAtOffsetPrimitiveNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@Child private RubyNode arguments2_;
@CompilationFinal private Class> arguments1Type_;
@CompilationFinal private Class> arguments2Type_;
@Child private BaseNode_ specialization_;
private PointerGetAtOffsetPrimitiveNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.arguments2_ = arguments != null && 2 < arguments.length ? arguments[2] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) throws UnexpectedResultException {
return specialization_.executeDynamicObject(frameValue);
}
@Override
public int executeInteger(VirtualFrame frameValue) throws UnexpectedResultException {
return specialization_.executeInt(frameValue);
}
@Override
public long executeLong(VirtualFrame frameValue) throws UnexpectedResultException {
return specialization_.executeLong(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
private static DynamicObject expectDynamicObject(Object value) throws UnexpectedResultException {
if (value instanceof DynamicObject) {
return (DynamicObject) value;
}
throw new UnexpectedResultException(value);
}
private static int expectInteger(Object value) throws UnexpectedResultException {
if (value instanceof Integer) {
return (int) value;
}
throw new UnexpectedResultException(value);
}
private static long expectLong(Object value) throws UnexpectedResultException {
if (value instanceof Long) {
return (long) value;
}
throw new UnexpectedResultException(value);
}
@GeneratedBy(PointerGetAtOffsetPrimitiveNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected PointerGetAtOffsetPrimitiveNodeGen root;
BaseNode_(PointerGetAtOffsetPrimitiveNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (PointerGetAtOffsetPrimitiveNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_, root.arguments1_, root.arguments2_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return this.execute_((VirtualFrame) frameValue, arguments0Value, arguments1Value, arguments2Value);
}
public abstract Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = executeArguments1_(frameValue);
Object arguments2Value_ = executeArguments2_(frameValue);
return execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public DynamicObject executeDynamicObject(VirtualFrame frameValue) throws UnexpectedResultException {
return expectDynamicObject(execute(frameValue));
}
public int executeInt(VirtualFrame frameValue) throws UnexpectedResultException {
return expectInteger(execute(frameValue));
}
public long executeLong(VirtualFrame frameValue) throws UnexpectedResultException {
return expectLong(execute(frameValue));
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value) && RubyTypesGen.isImplicitInteger(arguments2Value)) {
int arguments2Value_ = RubyTypesGen.asImplicitInteger(arguments2Value);
if ((arguments2Value_ == RubiniusTypes.TYPE_CHAR)) {
return GetAtOffsetCharNode_.create(root, arguments1Value, arguments2Value);
}
if ((arguments2Value_ == RubiniusTypes.TYPE_UCHAR)) {
return GetAtOffsetUCharNode_.create(root, arguments1Value, arguments2Value);
}
if ((arguments2Value_ == RubiniusTypes.TYPE_INT)) {
return GetAtOffsetIntNode_.create(root, arguments1Value, arguments2Value);
}
if ((arguments2Value_ == RubiniusTypes.TYPE_SHORT)) {
return GetAtOffsetShortNode_.create(root, arguments1Value, arguments2Value);
}
if ((arguments2Value_ == RubiniusTypes.TYPE_USHORT)) {
return GetAtOffsetUShortNode_.create(root, arguments1Value, arguments2Value);
}
if ((arguments2Value_ == RubiniusTypes.TYPE_LONG)) {
return GetAtOffsetLongNode_.create(root, arguments1Value, arguments2Value);
}
if ((arguments2Value_ == RubiniusTypes.TYPE_ULONG)) {
return GetAtOffsetULongNode_.create(root, arguments1Value, arguments2Value);
}
if ((arguments2Value_ == RubiniusTypes.TYPE_ULL)) {
return GetAtOffsetULLNode_.create(root, arguments1Value, arguments2Value);
}
if ((arguments2Value_ == RubiniusTypes.TYPE_STRING)) {
return GetAtOffsetStringNode_.create(root, arguments1Value, arguments2Value);
}
if ((arguments2Value_ == RubiniusTypes.TYPE_PTR)) {
return GetAtOffsetPointerNode_.create(root, arguments1Value, arguments2Value);
}
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments1_(Frame frameValue) {
Class> arguments1Type_ = root.arguments1Type_;
try {
if (arguments1Type_ == int.class) {
return root.arguments1_.executeInteger((VirtualFrame) frameValue);
} else if (arguments1Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments1_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments1Type_ = _type;
}
} else {
return root.arguments1_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments1Type_ = Object.class;
return ex.getResult();
}
}
protected final Object executeArguments2_(Frame frameValue) {
Class> arguments2Type_ = root.arguments2Type_;
try {
if (arguments2Type_ == int.class) {
return root.arguments2_.executeInteger((VirtualFrame) frameValue);
} else if (arguments2Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments2_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments2Type_ = _type;
}
} else {
return root.arguments2_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments2Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(PointerGetAtOffsetPrimitiveNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(PointerGetAtOffsetPrimitiveNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return uninitialized(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(PointerGetAtOffsetPrimitiveNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(PointerGetAtOffsetPrimitiveNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(PointerGetAtOffsetPrimitiveNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value, arguments1Value, arguments2Value));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(PointerGetAtOffsetPrimitiveNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "getAtOffsetChar(DynamicObject, int, int)", value = PointerGetAtOffsetPrimitiveNode.class)
private static final class GetAtOffsetCharNode_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
private final Class> arguments2ImplicitType;
GetAtOffsetCharNode_(PointerGetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value) {
super(root, 1);
this.arguments1ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments1Value);
this.arguments2ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments2Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((GetAtOffsetCharNode_) other).arguments1ImplicitType && this.arguments2ImplicitType == ((GetAtOffsetCharNode_) other).arguments2ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
try {
return executeInt(frameValue);
} catch (UnexpectedResultException ex) {
return ex.getResult();
}
}
@Override
public int executeInt(VirtualFrame frameValue) throws UnexpectedResultException {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
Object arguments2Value = executeArguments2_(frameValue);
return expectInteger(getNext().execute_(frameValue, ex.getResult(), arguments1Value, arguments2Value));
}
int arguments1Value_;
try {
if (arguments1ImplicitType == int.class) {
arguments1Value_ = root.arguments1_.executeInteger(frameValue);
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitInteger(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
Object arguments2Value = executeArguments2_(frameValue);
return expectInteger(getNext().execute_(frameValue, arguments0Value_, ex.getResult(), arguments2Value));
}
int arguments2Value_;
try {
if (arguments2ImplicitType == int.class) {
arguments2Value_ = root.arguments2_.executeInteger(frameValue);
} else {
Object arguments2Value__ = executeArguments2_(frameValue);
arguments2Value_ = RubyTypesGen.expectImplicitInteger(arguments2Value__, arguments2ImplicitType);
}
} catch (UnexpectedResultException ex) {
return expectInteger(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, ex.getResult()));
}
if ((arguments2Value_ == RubiniusTypes.TYPE_CHAR)) {
return root.getAtOffsetChar(arguments0Value_, arguments1Value_, arguments2Value_);
}
return expectInteger(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value, arguments1ImplicitType) && RubyTypesGen.isImplicitInteger(arguments2Value, arguments2ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
int arguments1Value_ = RubyTypesGen.asImplicitInteger(arguments1Value, arguments1ImplicitType);
int arguments2Value_ = RubyTypesGen.asImplicitInteger(arguments2Value, arguments2ImplicitType);
if ((arguments2Value_ == RubiniusTypes.TYPE_CHAR)) {
return root.getAtOffsetChar(arguments0Value_, arguments1Value_, arguments2Value_);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(PointerGetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value) {
return new GetAtOffsetCharNode_(root, arguments1Value, arguments2Value);
}
}
@GeneratedBy(methodName = "getAtOffsetUChar(DynamicObject, int, int)", value = PointerGetAtOffsetPrimitiveNode.class)
private static final class GetAtOffsetUCharNode_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
private final Class> arguments2ImplicitType;
GetAtOffsetUCharNode_(PointerGetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value) {
super(root, 2);
this.arguments1ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments1Value);
this.arguments2ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments2Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((GetAtOffsetUCharNode_) other).arguments1ImplicitType && this.arguments2ImplicitType == ((GetAtOffsetUCharNode_) other).arguments2ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
try {
return executeInt(frameValue);
} catch (UnexpectedResultException ex) {
return ex.getResult();
}
}
@Override
public int executeInt(VirtualFrame frameValue) throws UnexpectedResultException {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
Object arguments2Value = executeArguments2_(frameValue);
return expectInteger(getNext().execute_(frameValue, ex.getResult(), arguments1Value, arguments2Value));
}
int arguments1Value_;
try {
if (arguments1ImplicitType == int.class) {
arguments1Value_ = root.arguments1_.executeInteger(frameValue);
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitInteger(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
Object arguments2Value = executeArguments2_(frameValue);
return expectInteger(getNext().execute_(frameValue, arguments0Value_, ex.getResult(), arguments2Value));
}
int arguments2Value_;
try {
if (arguments2ImplicitType == int.class) {
arguments2Value_ = root.arguments2_.executeInteger(frameValue);
} else {
Object arguments2Value__ = executeArguments2_(frameValue);
arguments2Value_ = RubyTypesGen.expectImplicitInteger(arguments2Value__, arguments2ImplicitType);
}
} catch (UnexpectedResultException ex) {
return expectInteger(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, ex.getResult()));
}
if ((arguments2Value_ == RubiniusTypes.TYPE_UCHAR)) {
return root.getAtOffsetUChar(arguments0Value_, arguments1Value_, arguments2Value_);
}
return expectInteger(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value, arguments1ImplicitType) && RubyTypesGen.isImplicitInteger(arguments2Value, arguments2ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
int arguments1Value_ = RubyTypesGen.asImplicitInteger(arguments1Value, arguments1ImplicitType);
int arguments2Value_ = RubyTypesGen.asImplicitInteger(arguments2Value, arguments2ImplicitType);
if ((arguments2Value_ == RubiniusTypes.TYPE_UCHAR)) {
return root.getAtOffsetUChar(arguments0Value_, arguments1Value_, arguments2Value_);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(PointerGetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value) {
return new GetAtOffsetUCharNode_(root, arguments1Value, arguments2Value);
}
}
@GeneratedBy(methodName = "getAtOffsetInt(DynamicObject, int, int)", value = PointerGetAtOffsetPrimitiveNode.class)
private static final class GetAtOffsetIntNode_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
private final Class> arguments2ImplicitType;
GetAtOffsetIntNode_(PointerGetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value) {
super(root, 3);
this.arguments1ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments1Value);
this.arguments2ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments2Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((GetAtOffsetIntNode_) other).arguments1ImplicitType && this.arguments2ImplicitType == ((GetAtOffsetIntNode_) other).arguments2ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
try {
return executeInt(frameValue);
} catch (UnexpectedResultException ex) {
return ex.getResult();
}
}
@Override
public int executeInt(VirtualFrame frameValue) throws UnexpectedResultException {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
Object arguments2Value = executeArguments2_(frameValue);
return expectInteger(getNext().execute_(frameValue, ex.getResult(), arguments1Value, arguments2Value));
}
int arguments1Value_;
try {
if (arguments1ImplicitType == int.class) {
arguments1Value_ = root.arguments1_.executeInteger(frameValue);
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitInteger(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
Object arguments2Value = executeArguments2_(frameValue);
return expectInteger(getNext().execute_(frameValue, arguments0Value_, ex.getResult(), arguments2Value));
}
int arguments2Value_;
try {
if (arguments2ImplicitType == int.class) {
arguments2Value_ = root.arguments2_.executeInteger(frameValue);
} else {
Object arguments2Value__ = executeArguments2_(frameValue);
arguments2Value_ = RubyTypesGen.expectImplicitInteger(arguments2Value__, arguments2ImplicitType);
}
} catch (UnexpectedResultException ex) {
return expectInteger(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, ex.getResult()));
}
if ((arguments2Value_ == RubiniusTypes.TYPE_INT)) {
return root.getAtOffsetInt(arguments0Value_, arguments1Value_, arguments2Value_);
}
return expectInteger(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value, arguments1ImplicitType) && RubyTypesGen.isImplicitInteger(arguments2Value, arguments2ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
int arguments1Value_ = RubyTypesGen.asImplicitInteger(arguments1Value, arguments1ImplicitType);
int arguments2Value_ = RubyTypesGen.asImplicitInteger(arguments2Value, arguments2ImplicitType);
if ((arguments2Value_ == RubiniusTypes.TYPE_INT)) {
return root.getAtOffsetInt(arguments0Value_, arguments1Value_, arguments2Value_);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(PointerGetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value) {
return new GetAtOffsetIntNode_(root, arguments1Value, arguments2Value);
}
}
@GeneratedBy(methodName = "getAtOffsetShort(DynamicObject, int, int)", value = PointerGetAtOffsetPrimitiveNode.class)
private static final class GetAtOffsetShortNode_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
private final Class> arguments2ImplicitType;
GetAtOffsetShortNode_(PointerGetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value) {
super(root, 4);
this.arguments1ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments1Value);
this.arguments2ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments2Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((GetAtOffsetShortNode_) other).arguments1ImplicitType && this.arguments2ImplicitType == ((GetAtOffsetShortNode_) other).arguments2ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
try {
return executeInt(frameValue);
} catch (UnexpectedResultException ex) {
return ex.getResult();
}
}
@Override
public int executeInt(VirtualFrame frameValue) throws UnexpectedResultException {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
Object arguments2Value = executeArguments2_(frameValue);
return expectInteger(getNext().execute_(frameValue, ex.getResult(), arguments1Value, arguments2Value));
}
int arguments1Value_;
try {
if (arguments1ImplicitType == int.class) {
arguments1Value_ = root.arguments1_.executeInteger(frameValue);
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitInteger(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
Object arguments2Value = executeArguments2_(frameValue);
return expectInteger(getNext().execute_(frameValue, arguments0Value_, ex.getResult(), arguments2Value));
}
int arguments2Value_;
try {
if (arguments2ImplicitType == int.class) {
arguments2Value_ = root.arguments2_.executeInteger(frameValue);
} else {
Object arguments2Value__ = executeArguments2_(frameValue);
arguments2Value_ = RubyTypesGen.expectImplicitInteger(arguments2Value__, arguments2ImplicitType);
}
} catch (UnexpectedResultException ex) {
return expectInteger(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, ex.getResult()));
}
if ((arguments2Value_ == RubiniusTypes.TYPE_SHORT)) {
return root.getAtOffsetShort(arguments0Value_, arguments1Value_, arguments2Value_);
}
return expectInteger(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value, arguments1ImplicitType) && RubyTypesGen.isImplicitInteger(arguments2Value, arguments2ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
int arguments1Value_ = RubyTypesGen.asImplicitInteger(arguments1Value, arguments1ImplicitType);
int arguments2Value_ = RubyTypesGen.asImplicitInteger(arguments2Value, arguments2ImplicitType);
if ((arguments2Value_ == RubiniusTypes.TYPE_SHORT)) {
return root.getAtOffsetShort(arguments0Value_, arguments1Value_, arguments2Value_);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(PointerGetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value) {
return new GetAtOffsetShortNode_(root, arguments1Value, arguments2Value);
}
}
@GeneratedBy(methodName = "getAtOffsetUShort(DynamicObject, int, int)", value = PointerGetAtOffsetPrimitiveNode.class)
private static final class GetAtOffsetUShortNode_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
private final Class> arguments2ImplicitType;
GetAtOffsetUShortNode_(PointerGetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value) {
super(root, 5);
this.arguments1ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments1Value);
this.arguments2ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments2Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((GetAtOffsetUShortNode_) other).arguments1ImplicitType && this.arguments2ImplicitType == ((GetAtOffsetUShortNode_) other).arguments2ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
try {
return executeInt(frameValue);
} catch (UnexpectedResultException ex) {
return ex.getResult();
}
}
@Override
public int executeInt(VirtualFrame frameValue) throws UnexpectedResultException {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
Object arguments2Value = executeArguments2_(frameValue);
return expectInteger(getNext().execute_(frameValue, ex.getResult(), arguments1Value, arguments2Value));
}
int arguments1Value_;
try {
if (arguments1ImplicitType == int.class) {
arguments1Value_ = root.arguments1_.executeInteger(frameValue);
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitInteger(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
Object arguments2Value = executeArguments2_(frameValue);
return expectInteger(getNext().execute_(frameValue, arguments0Value_, ex.getResult(), arguments2Value));
}
int arguments2Value_;
try {
if (arguments2ImplicitType == int.class) {
arguments2Value_ = root.arguments2_.executeInteger(frameValue);
} else {
Object arguments2Value__ = executeArguments2_(frameValue);
arguments2Value_ = RubyTypesGen.expectImplicitInteger(arguments2Value__, arguments2ImplicitType);
}
} catch (UnexpectedResultException ex) {
return expectInteger(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, ex.getResult()));
}
if ((arguments2Value_ == RubiniusTypes.TYPE_USHORT)) {
return root.getAtOffsetUShort(arguments0Value_, arguments1Value_, arguments2Value_);
}
return expectInteger(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value, arguments1ImplicitType) && RubyTypesGen.isImplicitInteger(arguments2Value, arguments2ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
int arguments1Value_ = RubyTypesGen.asImplicitInteger(arguments1Value, arguments1ImplicitType);
int arguments2Value_ = RubyTypesGen.asImplicitInteger(arguments2Value, arguments2ImplicitType);
if ((arguments2Value_ == RubiniusTypes.TYPE_USHORT)) {
return root.getAtOffsetUShort(arguments0Value_, arguments1Value_, arguments2Value_);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(PointerGetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value) {
return new GetAtOffsetUShortNode_(root, arguments1Value, arguments2Value);
}
}
@GeneratedBy(methodName = "getAtOffsetLong(DynamicObject, int, int)", value = PointerGetAtOffsetPrimitiveNode.class)
private static final class GetAtOffsetLongNode_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
private final Class> arguments2ImplicitType;
GetAtOffsetLongNode_(PointerGetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value) {
super(root, 6);
this.arguments1ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments1Value);
this.arguments2ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments2Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((GetAtOffsetLongNode_) other).arguments1ImplicitType && this.arguments2ImplicitType == ((GetAtOffsetLongNode_) other).arguments2ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
try {
return executeLong(frameValue);
} catch (UnexpectedResultException ex) {
return ex.getResult();
}
}
@Override
public long executeLong(VirtualFrame frameValue) throws UnexpectedResultException {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
Object arguments2Value = executeArguments2_(frameValue);
return expectLong(getNext().execute_(frameValue, ex.getResult(), arguments1Value, arguments2Value));
}
int arguments1Value_;
try {
if (arguments1ImplicitType == int.class) {
arguments1Value_ = root.arguments1_.executeInteger(frameValue);
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitInteger(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
Object arguments2Value = executeArguments2_(frameValue);
return expectLong(getNext().execute_(frameValue, arguments0Value_, ex.getResult(), arguments2Value));
}
int arguments2Value_;
try {
if (arguments2ImplicitType == int.class) {
arguments2Value_ = root.arguments2_.executeInteger(frameValue);
} else {
Object arguments2Value__ = executeArguments2_(frameValue);
arguments2Value_ = RubyTypesGen.expectImplicitInteger(arguments2Value__, arguments2ImplicitType);
}
} catch (UnexpectedResultException ex) {
return expectLong(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, ex.getResult()));
}
if ((arguments2Value_ == RubiniusTypes.TYPE_LONG)) {
return root.getAtOffsetLong(arguments0Value_, arguments1Value_, arguments2Value_);
}
return expectLong(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value, arguments1ImplicitType) && RubyTypesGen.isImplicitInteger(arguments2Value, arguments2ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
int arguments1Value_ = RubyTypesGen.asImplicitInteger(arguments1Value, arguments1ImplicitType);
int arguments2Value_ = RubyTypesGen.asImplicitInteger(arguments2Value, arguments2ImplicitType);
if ((arguments2Value_ == RubiniusTypes.TYPE_LONG)) {
return root.getAtOffsetLong(arguments0Value_, arguments1Value_, arguments2Value_);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(PointerGetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value) {
return new GetAtOffsetLongNode_(root, arguments1Value, arguments2Value);
}
}
@GeneratedBy(methodName = "getAtOffsetULong(DynamicObject, int, int)", value = PointerGetAtOffsetPrimitiveNode.class)
private static final class GetAtOffsetULongNode_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
private final Class> arguments2ImplicitType;
GetAtOffsetULongNode_(PointerGetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value) {
super(root, 7);
this.arguments1ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments1Value);
this.arguments2ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments2Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((GetAtOffsetULongNode_) other).arguments1ImplicitType && this.arguments2ImplicitType == ((GetAtOffsetULongNode_) other).arguments2ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
try {
return executeLong(frameValue);
} catch (UnexpectedResultException ex) {
return ex.getResult();
}
}
@Override
public long executeLong(VirtualFrame frameValue) throws UnexpectedResultException {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
Object arguments2Value = executeArguments2_(frameValue);
return expectLong(getNext().execute_(frameValue, ex.getResult(), arguments1Value, arguments2Value));
}
int arguments1Value_;
try {
if (arguments1ImplicitType == int.class) {
arguments1Value_ = root.arguments1_.executeInteger(frameValue);
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitInteger(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
Object arguments2Value = executeArguments2_(frameValue);
return expectLong(getNext().execute_(frameValue, arguments0Value_, ex.getResult(), arguments2Value));
}
int arguments2Value_;
try {
if (arguments2ImplicitType == int.class) {
arguments2Value_ = root.arguments2_.executeInteger(frameValue);
} else {
Object arguments2Value__ = executeArguments2_(frameValue);
arguments2Value_ = RubyTypesGen.expectImplicitInteger(arguments2Value__, arguments2ImplicitType);
}
} catch (UnexpectedResultException ex) {
return expectLong(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, ex.getResult()));
}
if ((arguments2Value_ == RubiniusTypes.TYPE_ULONG)) {
return root.getAtOffsetULong(arguments0Value_, arguments1Value_, arguments2Value_);
}
return expectLong(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value, arguments1ImplicitType) && RubyTypesGen.isImplicitInteger(arguments2Value, arguments2ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
int arguments1Value_ = RubyTypesGen.asImplicitInteger(arguments1Value, arguments1ImplicitType);
int arguments2Value_ = RubyTypesGen.asImplicitInteger(arguments2Value, arguments2ImplicitType);
if ((arguments2Value_ == RubiniusTypes.TYPE_ULONG)) {
return root.getAtOffsetULong(arguments0Value_, arguments1Value_, arguments2Value_);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(PointerGetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value) {
return new GetAtOffsetULongNode_(root, arguments1Value, arguments2Value);
}
}
@GeneratedBy(methodName = "getAtOffsetULL(DynamicObject, int, int)", value = PointerGetAtOffsetPrimitiveNode.class)
private static final class GetAtOffsetULLNode_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
private final Class> arguments2ImplicitType;
GetAtOffsetULLNode_(PointerGetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value) {
super(root, 8);
this.arguments1ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments1Value);
this.arguments2ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments2Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((GetAtOffsetULLNode_) other).arguments1ImplicitType && this.arguments2ImplicitType == ((GetAtOffsetULLNode_) other).arguments2ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
try {
return executeLong(frameValue);
} catch (UnexpectedResultException ex) {
return ex.getResult();
}
}
@Override
public long executeLong(VirtualFrame frameValue) throws UnexpectedResultException {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
Object arguments2Value = executeArguments2_(frameValue);
return expectLong(getNext().execute_(frameValue, ex.getResult(), arguments1Value, arguments2Value));
}
int arguments1Value_;
try {
if (arguments1ImplicitType == int.class) {
arguments1Value_ = root.arguments1_.executeInteger(frameValue);
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitInteger(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
Object arguments2Value = executeArguments2_(frameValue);
return expectLong(getNext().execute_(frameValue, arguments0Value_, ex.getResult(), arguments2Value));
}
int arguments2Value_;
try {
if (arguments2ImplicitType == int.class) {
arguments2Value_ = root.arguments2_.executeInteger(frameValue);
} else {
Object arguments2Value__ = executeArguments2_(frameValue);
arguments2Value_ = RubyTypesGen.expectImplicitInteger(arguments2Value__, arguments2ImplicitType);
}
} catch (UnexpectedResultException ex) {
return expectLong(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, ex.getResult()));
}
if ((arguments2Value_ == RubiniusTypes.TYPE_ULL)) {
return root.getAtOffsetULL(arguments0Value_, arguments1Value_, arguments2Value_);
}
return expectLong(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value, arguments1ImplicitType) && RubyTypesGen.isImplicitInteger(arguments2Value, arguments2ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
int arguments1Value_ = RubyTypesGen.asImplicitInteger(arguments1Value, arguments1ImplicitType);
int arguments2Value_ = RubyTypesGen.asImplicitInteger(arguments2Value, arguments2ImplicitType);
if ((arguments2Value_ == RubiniusTypes.TYPE_ULL)) {
return root.getAtOffsetULL(arguments0Value_, arguments1Value_, arguments2Value_);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(PointerGetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value) {
return new GetAtOffsetULLNode_(root, arguments1Value, arguments2Value);
}
}
@GeneratedBy(methodName = "getAtOffsetString(DynamicObject, int, int)", value = PointerGetAtOffsetPrimitiveNode.class)
private static final class GetAtOffsetStringNode_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
private final Class> arguments2ImplicitType;
GetAtOffsetStringNode_(PointerGetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value) {
super(root, 9);
this.arguments1ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments1Value);
this.arguments2ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments2Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((GetAtOffsetStringNode_) other).arguments1ImplicitType && this.arguments2ImplicitType == ((GetAtOffsetStringNode_) other).arguments2ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
try {
return executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
return ex.getResult();
}
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) throws UnexpectedResultException {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
Object arguments2Value = executeArguments2_(frameValue);
return expectDynamicObject(getNext().execute_(frameValue, ex.getResult(), arguments1Value, arguments2Value));
}
int arguments1Value_;
try {
if (arguments1ImplicitType == int.class) {
arguments1Value_ = root.arguments1_.executeInteger(frameValue);
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitInteger(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
Object arguments2Value = executeArguments2_(frameValue);
return expectDynamicObject(getNext().execute_(frameValue, arguments0Value_, ex.getResult(), arguments2Value));
}
int arguments2Value_;
try {
if (arguments2ImplicitType == int.class) {
arguments2Value_ = root.arguments2_.executeInteger(frameValue);
} else {
Object arguments2Value__ = executeArguments2_(frameValue);
arguments2Value_ = RubyTypesGen.expectImplicitInteger(arguments2Value__, arguments2ImplicitType);
}
} catch (UnexpectedResultException ex) {
return expectDynamicObject(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, ex.getResult()));
}
if ((arguments2Value_ == RubiniusTypes.TYPE_STRING)) {
return root.getAtOffsetString(arguments0Value_, arguments1Value_, arguments2Value_);
}
return expectDynamicObject(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value, arguments1ImplicitType) && RubyTypesGen.isImplicitInteger(arguments2Value, arguments2ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
int arguments1Value_ = RubyTypesGen.asImplicitInteger(arguments1Value, arguments1ImplicitType);
int arguments2Value_ = RubyTypesGen.asImplicitInteger(arguments2Value, arguments2ImplicitType);
if ((arguments2Value_ == RubiniusTypes.TYPE_STRING)) {
return root.getAtOffsetString(arguments0Value_, arguments1Value_, arguments2Value_);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(PointerGetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value) {
return new GetAtOffsetStringNode_(root, arguments1Value, arguments2Value);
}
}
@GeneratedBy(methodName = "getAtOffsetPointer(DynamicObject, int, int)", value = PointerGetAtOffsetPrimitiveNode.class)
private static final class GetAtOffsetPointerNode_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
private final Class> arguments2ImplicitType;
GetAtOffsetPointerNode_(PointerGetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value) {
super(root, 10);
this.arguments1ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments1Value);
this.arguments2ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments2Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((GetAtOffsetPointerNode_) other).arguments1ImplicitType && this.arguments2ImplicitType == ((GetAtOffsetPointerNode_) other).arguments2ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
try {
return executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
return ex.getResult();
}
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) throws UnexpectedResultException {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
Object arguments2Value = executeArguments2_(frameValue);
return expectDynamicObject(getNext().execute_(frameValue, ex.getResult(), arguments1Value, arguments2Value));
}
int arguments1Value_;
try {
if (arguments1ImplicitType == int.class) {
arguments1Value_ = root.arguments1_.executeInteger(frameValue);
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitInteger(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
Object arguments2Value = executeArguments2_(frameValue);
return expectDynamicObject(getNext().execute_(frameValue, arguments0Value_, ex.getResult(), arguments2Value));
}
int arguments2Value_;
try {
if (arguments2ImplicitType == int.class) {
arguments2Value_ = root.arguments2_.executeInteger(frameValue);
} else {
Object arguments2Value__ = executeArguments2_(frameValue);
arguments2Value_ = RubyTypesGen.expectImplicitInteger(arguments2Value__, arguments2ImplicitType);
}
} catch (UnexpectedResultException ex) {
return expectDynamicObject(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, ex.getResult()));
}
if ((arguments2Value_ == RubiniusTypes.TYPE_PTR)) {
return root.getAtOffsetPointer(arguments0Value_, arguments1Value_, arguments2Value_);
}
return expectDynamicObject(getNext().execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value, arguments1ImplicitType) && RubyTypesGen.isImplicitInteger(arguments2Value, arguments2ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
int arguments1Value_ = RubyTypesGen.asImplicitInteger(arguments1Value, arguments1ImplicitType);
int arguments2Value_ = RubyTypesGen.asImplicitInteger(arguments2Value, arguments2ImplicitType);
if ((arguments2Value_ == RubiniusTypes.TYPE_PTR)) {
return root.getAtOffsetPointer(arguments0Value_, arguments1Value_, arguments2Value_);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(PointerGetAtOffsetPrimitiveNodeGen root, Object arguments1Value, Object arguments2Value) {
return new GetAtOffsetPointerNode_(root, arguments1Value, arguments2Value);
}
}
}
}
@GeneratedBy(PointerWriteStringPrimitiveNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class PointerWriteStringPrimitiveNodeFactory implements NodeFactory {
private static PointerWriteStringPrimitiveNodeFactory pointerWriteStringPrimitiveNodeFactoryInstance;
private PointerWriteStringPrimitiveNodeFactory() {
}
@Override
public Class getNodeClass() {
return PointerWriteStringPrimitiveNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public PointerWriteStringPrimitiveNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (pointerWriteStringPrimitiveNodeFactoryInstance == null) {
pointerWriteStringPrimitiveNodeFactoryInstance = new PointerWriteStringPrimitiveNodeFactory();
}
return pointerWriteStringPrimitiveNodeFactoryInstance;
}
public static PointerWriteStringPrimitiveNode create(RubyNode[] arguments) {
return new PointerWriteStringPrimitiveNodeGen(arguments);
}
@GeneratedBy(PointerWriteStringPrimitiveNode.class)
public static final class PointerWriteStringPrimitiveNodeGen extends PointerWriteStringPrimitiveNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@Child private RubyNode arguments2_;
@CompilationFinal private Class> arguments2Type_;
@Child private BaseNode_ specialization_;
private PointerWriteStringPrimitiveNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.arguments2_ = arguments != null && 2 < arguments.length ? arguments[2] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
return specialization_.executeDynamicObject(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(PointerWriteStringPrimitiveNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected PointerWriteStringPrimitiveNodeGen root;
BaseNode_(PointerWriteStringPrimitiveNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (PointerWriteStringPrimitiveNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_, root.arguments1_, root.arguments2_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return this.executeDynamicObject_((VirtualFrame) frameValue, arguments0Value, arguments1Value, arguments2Value);
}
public abstract DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = root.arguments1_.execute(frameValue);
Object arguments2Value_ = executeArguments2_(frameValue);
return executeDynamicObject_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
return (DynamicObject) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof DynamicObject && arguments1Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments2Value)) {
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
if ((RubyGuards.isRubyString(arguments1Value_))) {
return AddressNode_.create(root, arguments2Value);
}
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments2_(Frame frameValue) {
Class> arguments2Type_ = root.arguments2Type_;
try {
if (arguments2Type_ == int.class) {
return root.arguments2_.executeInteger((VirtualFrame) frameValue);
} else if (arguments2Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments2_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments2Type_ = _type;
}
} else {
return root.arguments2_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments2Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(PointerWriteStringPrimitiveNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(PointerWriteStringPrimitiveNodeGen root) {
super(root, 2147483647);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return (DynamicObject) uninitialized(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(PointerWriteStringPrimitiveNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(PointerWriteStringPrimitiveNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(PointerWriteStringPrimitiveNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value, arguments1Value, arguments2Value));
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(PointerWriteStringPrimitiveNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "address(DynamicObject, DynamicObject, int)", value = PointerWriteStringPrimitiveNode.class)
private static final class AddressNode_ extends BaseNode_ {
private final Class> arguments2ImplicitType;
AddressNode_(PointerWriteStringPrimitiveNodeGen root, Object arguments2Value) {
super(root, 1);
this.arguments2ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments2Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments2ImplicitType == ((AddressNode_) other).arguments2ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject(frameValue);
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = root.arguments1_.execute(frameValue);
Object arguments2Value = executeArguments2_(frameValue);
return getNext().executeDynamicObject_(frameValue, ex.getResult(), arguments1Value, arguments2Value);
}
DynamicObject arguments1Value_;
try {
arguments1Value_ = root.arguments1_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments2Value = executeArguments2_(frameValue);
return getNext().executeDynamicObject_(frameValue, arguments0Value_, ex.getResult(), arguments2Value);
}
int arguments2Value_;
try {
if (arguments2ImplicitType == int.class) {
arguments2Value_ = root.arguments2_.executeInteger(frameValue);
} else {
Object arguments2Value__ = executeArguments2_(frameValue);
arguments2Value_ = RubyTypesGen.expectImplicitInteger(arguments2Value__, arguments2ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeDynamicObject_(frameValue, arguments0Value_, arguments1Value_, ex.getResult());
}
if ((RubyGuards.isRubyString(arguments1Value_))) {
return root.address(arguments0Value_, arguments1Value_, arguments2Value_);
}
return getNext().executeDynamicObject_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof DynamicObject && arguments1Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments2Value, arguments2ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
int arguments2Value_ = RubyTypesGen.asImplicitInteger(arguments2Value, arguments2ImplicitType);
if ((RubyGuards.isRubyString(arguments1Value_))) {
return root.address(arguments0Value_, arguments1Value_, arguments2Value_);
}
}
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(PointerWriteStringPrimitiveNodeGen root, Object arguments2Value) {
return new AddressNode_(root, arguments2Value);
}
}
}
}
@GeneratedBy(PointerReadStringToNullPrimitiveNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class PointerReadStringToNullPrimitiveNodeFactory implements NodeFactory {
private static PointerReadStringToNullPrimitiveNodeFactory pointerReadStringToNullPrimitiveNodeFactoryInstance;
private PointerReadStringToNullPrimitiveNodeFactory() {
}
@Override
public Class getNodeClass() {
return PointerReadStringToNullPrimitiveNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public PointerReadStringToNullPrimitiveNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (pointerReadStringToNullPrimitiveNodeFactoryInstance == null) {
pointerReadStringToNullPrimitiveNodeFactoryInstance = new PointerReadStringToNullPrimitiveNodeFactory();
}
return pointerReadStringToNullPrimitiveNodeFactoryInstance;
}
public static PointerReadStringToNullPrimitiveNode create(RubyNode[] arguments) {
return new PointerReadStringToNullPrimitiveNodeGen(arguments);
}
@GeneratedBy(PointerReadStringToNullPrimitiveNode.class)
public static final class PointerReadStringToNullPrimitiveNodeGen extends PointerReadStringToNullPrimitiveNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private BaseNode_ specialization_;
private PointerReadStringToNullPrimitiveNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(PointerReadStringToNullPrimitiveNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected PointerReadStringToNullPrimitiveNodeGen root;
BaseNode_(PointerReadStringToNullPrimitiveNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (PointerReadStringToNullPrimitiveNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.executeDynamicObject_((VirtualFrame) frameValue, arguments0Value);
}
public abstract DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
return executeDynamicObject_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (arguments0Value instanceof DynamicObject) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
if ((RubyGuards.isNullPointer(arguments0Value_))) {
return ReadNullPointerNode_.create(root);
} else {
return ReadStringToNullNode_.create(root);
}
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(PointerReadStringToNullPrimitiveNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(PointerReadStringToNullPrimitiveNodeGen root) {
super(root, 2147483647);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value) {
return (DynamicObject) uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(PointerReadStringToNullPrimitiveNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(PointerReadStringToNullPrimitiveNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(PointerReadStringToNullPrimitiveNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().executeDynamicObject_(frameValue, arguments0Value);
}
static BaseNode_ create(PointerReadStringToNullPrimitiveNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "readNullPointer(DynamicObject)", value = PointerReadStringToNullPrimitiveNode.class)
private static final class ReadNullPointerNode_ extends BaseNode_ {
ReadNullPointerNode_(PointerReadStringToNullPrimitiveNodeGen root) {
super(root, 1);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value) {
if (arguments0Value instanceof DynamicObject) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
if ((RubyGuards.isNullPointer(arguments0Value_))) {
return root.readNullPointer(arguments0Value_);
}
}
return getNext().executeDynamicObject_(frameValue, arguments0Value);
}
static BaseNode_ create(PointerReadStringToNullPrimitiveNodeGen root) {
return new ReadNullPointerNode_(root);
}
}
@GeneratedBy(methodName = "readStringToNull(DynamicObject)", value = PointerReadStringToNullPrimitiveNode.class)
private static final class ReadStringToNullNode_ extends BaseNode_ {
ReadStringToNullNode_(PointerReadStringToNullPrimitiveNodeGen root) {
super(root, 2);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value) {
if (arguments0Value instanceof DynamicObject) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
if ((!(RubyGuards.isNullPointer(arguments0Value_)))) {
return root.readStringToNull(arguments0Value_);
}
}
return getNext().executeDynamicObject_(frameValue, arguments0Value);
}
static BaseNode_ create(PointerReadStringToNullPrimitiveNodeGen root) {
return new ReadStringToNullNode_(root);
}
}
}
}
@GeneratedBy(PointerWriteIntPrimitiveNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class PointerWriteIntPrimitiveNodeFactory implements NodeFactory {
private static PointerWriteIntPrimitiveNodeFactory pointerWriteIntPrimitiveNodeFactoryInstance;
private PointerWriteIntPrimitiveNodeFactory() {
}
@Override
public Class getNodeClass() {
return PointerWriteIntPrimitiveNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public PointerWriteIntPrimitiveNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (pointerWriteIntPrimitiveNodeFactoryInstance == null) {
pointerWriteIntPrimitiveNodeFactoryInstance = new PointerWriteIntPrimitiveNodeFactory();
}
return pointerWriteIntPrimitiveNodeFactoryInstance;
}
public static PointerWriteIntPrimitiveNode create(RubyNode[] arguments) {
return new PointerWriteIntPrimitiveNodeGen(arguments);
}
@GeneratedBy(PointerWriteIntPrimitiveNode.class)
public static final class PointerWriteIntPrimitiveNodeGen extends PointerWriteIntPrimitiveNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@CompilationFinal private Class> arguments1Type_;
@Child private BaseNode_ specialization_;
private PointerWriteIntPrimitiveNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
return specialization_.executeDynamicObject(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(PointerWriteIntPrimitiveNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected PointerWriteIntPrimitiveNodeGen root;
BaseNode_(PointerWriteIntPrimitiveNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (PointerWriteIntPrimitiveNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_, root.arguments1_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value, Object arguments1Value) {
return this.executeDynamicObject_((VirtualFrame) frameValue, arguments0Value, arguments1Value);
}
public abstract DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = executeArguments1_(frameValue);
return executeDynamicObject_(frameValue, arguments0Value_, arguments1Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
return (DynamicObject) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value)) {
return AddressNode_.create(root, arguments1Value);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments1_(Frame frameValue) {
Class> arguments1Type_ = root.arguments1Type_;
try {
if (arguments1Type_ == int.class) {
return root.arguments1_.executeInteger((VirtualFrame) frameValue);
} else if (arguments1Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments1_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments1Type_ = _type;
}
} else {
return root.arguments1_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments1Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(PointerWriteIntPrimitiveNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(PointerWriteIntPrimitiveNodeGen root) {
super(root, 2147483647);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
return (DynamicObject) uninitialized(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(PointerWriteIntPrimitiveNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(PointerWriteIntPrimitiveNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(PointerWriteIntPrimitiveNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value, arguments1Value));
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(PointerWriteIntPrimitiveNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "address(DynamicObject, int)", value = PointerWriteIntPrimitiveNode.class)
private static final class AddressNode_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
AddressNode_(PointerWriteIntPrimitiveNodeGen root, Object arguments1Value) {
super(root, 1);
this.arguments1ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments1Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((AddressNode_) other).arguments1ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject(frameValue);
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
return getNext().executeDynamicObject_(frameValue, ex.getResult(), arguments1Value);
}
int arguments1Value_;
try {
if (arguments1ImplicitType == int.class) {
arguments1Value_ = root.arguments1_.executeInteger(frameValue);
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitInteger(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeDynamicObject_(frameValue, arguments0Value_, ex.getResult());
}
return root.address(arguments0Value_, arguments1Value_);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value, arguments1ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
int arguments1Value_ = RubyTypesGen.asImplicitInteger(arguments1Value, arguments1ImplicitType);
return root.address(arguments0Value_, arguments1Value_);
}
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(PointerWriteIntPrimitiveNodeGen root, Object arguments1Value) {
return new AddressNode_(root, arguments1Value);
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy