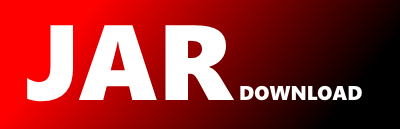
org.jruby.truffle.interop.InteropNodesFactory Maven / Gradle / Ivy
// CheckStyle: start generated
package org.jruby.truffle.interop;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NodeFactory;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.internal.SpecializationNode;
import com.oracle.truffle.api.dsl.internal.SpecializedNode;
import com.oracle.truffle.api.dsl.internal.SuppressFBWarnings;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.interop.TruffleObject;
import com.oracle.truffle.api.nodes.DirectCallNode;
import com.oracle.truffle.api.nodes.IndirectCallNode;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.nodes.UnexpectedResultException;
import com.oracle.truffle.api.object.DynamicObject;
import com.oracle.truffle.api.profiles.BranchProfile;
import java.util.Arrays;
import java.util.List;
import org.jruby.truffle.builtins.CoreMethodNode;
import org.jruby.truffle.core.cast.NameToJavaStringNode;
import org.jruby.truffle.core.rope.Rope;
import org.jruby.truffle.core.string.StringCachingGuards;
import org.jruby.truffle.interop.InteropNodes.BoxedNode;
import org.jruby.truffle.interop.InteropNodes.EvalNode;
import org.jruby.truffle.interop.InteropNodes.ExecuteNode;
import org.jruby.truffle.interop.InteropNodes.ExportNode;
import org.jruby.truffle.interop.InteropNodes.HasSizeNode;
import org.jruby.truffle.interop.InteropNodes.ImportFileNode;
import org.jruby.truffle.interop.InteropNodes.ImportNode;
import org.jruby.truffle.interop.InteropNodes.InteropFromJavaStringNode;
import org.jruby.truffle.interop.InteropNodes.InteropToJavaStringNode;
import org.jruby.truffle.interop.InteropNodes.InvokeNode;
import org.jruby.truffle.interop.InteropNodes.IsExecutableNode;
import org.jruby.truffle.interop.InteropNodes.KeysNode;
import org.jruby.truffle.interop.InteropNodes.MimeTypeSupportedNode;
import org.jruby.truffle.interop.InteropNodes.NullNode;
import org.jruby.truffle.interop.InteropNodes.ReadNode;
import org.jruby.truffle.interop.InteropNodes.SizeNode;
import org.jruby.truffle.interop.InteropNodes.UnboxNode;
import org.jruby.truffle.interop.InteropNodes.WriteNode;
import org.jruby.truffle.language.RubyGuards;
import org.jruby.truffle.language.RubyNode;
import org.jruby.truffle.language.RubyTypes;
import org.jruby.truffle.language.RubyTypesGen;
import org.jruby.truffle.language.SnippetNode;
@GeneratedBy(InteropNodes.class)
public final class InteropNodesFactory {
public static List> getFactories() {
return Arrays.asList(ImportFileNodeFactory.getInstance(), IsExecutableNodeFactory.getInstance(), ExecuteNodeFactory.getInstance(), InvokeNodeFactory.getInstance(), HasSizeNodeFactory.getInstance(), SizeNodeFactory.getInstance(), BoxedNodeFactory.getInstance(), UnboxNodeFactory.getInstance(), NullNodeFactory.getInstance(), ReadNodeFactory.getInstance(), WriteNodeFactory.getInstance(), KeysNodeFactory.getInstance(), ExportNodeFactory.getInstance(), ImportNodeFactory.getInstance(), MimeTypeSupportedNodeFactory.getInstance(), EvalNodeFactory.getInstance(), InteropToJavaStringNodeFactory.getInstance(), InteropFromJavaStringNodeFactory.getInstance());
}
@GeneratedBy(ImportFileNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class ImportFileNodeFactory implements NodeFactory {
private static ImportFileNodeFactory importFileNodeFactoryInstance;
private ImportFileNodeFactory() {
}
@Override
public Class getNodeClass() {
return ImportFileNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public ImportFileNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (importFileNodeFactoryInstance == null) {
importFileNodeFactoryInstance = new ImportFileNodeFactory();
}
return importFileNodeFactoryInstance;
}
public static ImportFileNode create(RubyNode[] arguments) {
return new ImportFileNodeGen(arguments);
}
@GeneratedBy(ImportFileNode.class)
public static final class ImportFileNodeGen extends ImportFileNode {
@Child private RubyNode arguments0_;
@CompilationFinal private boolean seenUnsupported0;
private ImportFileNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(ex.getResult());
}
if ((RubyGuards.isRubyString(arguments0Value_))) {
return this.importFile(arguments0Value_);
}
throw unsupported(arguments0Value_);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
private UnsupportedSpecializationException unsupported(Object arguments0Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_}, arguments0Value);
}
}
}
@GeneratedBy(IsExecutableNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class IsExecutableNodeFactory implements NodeFactory {
private static IsExecutableNodeFactory isExecutableNodeFactoryInstance;
private IsExecutableNodeFactory() {
}
@Override
public Class getNodeClass() {
return IsExecutableNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public IsExecutableNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (isExecutableNodeFactoryInstance == null) {
isExecutableNodeFactoryInstance = new IsExecutableNodeFactory();
}
return isExecutableNodeFactoryInstance;
}
public static IsExecutableNode create(RubyNode[] arguments) {
return new IsExecutableNodeGen(arguments);
}
@GeneratedBy(IsExecutableNode.class)
public static final class IsExecutableNodeGen extends IsExecutableNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private BaseNode_ specialization_;
private IsExecutableNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
return specialization_.executeBoolean(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
private static TruffleObject expectTruffleObject(Object value) throws UnexpectedResultException {
if (value instanceof TruffleObject) {
return (TruffleObject) value;
}
throw new UnexpectedResultException(value);
}
@GeneratedBy(IsExecutableNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected IsExecutableNodeGen root;
BaseNode_(IsExecutableNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (IsExecutableNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.executeBoolean_((VirtualFrame) frameValue, arguments0Value);
}
public abstract boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
return executeBoolean_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public boolean executeBoolean(VirtualFrame frameValue) {
return (boolean) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (arguments0Value instanceof TruffleObject) {
Node isExecutableNode1 = (root.createIsExecutableNode());
return IsExecutableNode_.create(root, isExecutableNode1);
}
return null;
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(IsExecutableNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(IsExecutableNodeGen root) {
super(root, 2147483647);
}
@Override
public boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value) {
return (boolean) uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(IsExecutableNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(methodName = "isExecutable(VirtualFrame, TruffleObject, Node)", value = IsExecutableNode.class)
private static final class IsExecutableNode_ extends BaseNode_ {
@Child private Node isExecutableNode;
IsExecutableNode_(IsExecutableNodeGen root, Node isExecutableNode) {
super(root, 1);
this.isExecutableNode = isExecutableNode;
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
TruffleObject arguments0Value_;
try {
arguments0Value_ = expectTruffleObject(root.arguments0_.execute(frameValue));
} catch (UnexpectedResultException ex) {
return getNext().executeBoolean_(frameValue, ex.getResult());
}
return root.isExecutable(frameValue, arguments0Value_, this.isExecutableNode);
}
@Override
public boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value) {
if (arguments0Value instanceof TruffleObject) {
TruffleObject arguments0Value_ = (TruffleObject) arguments0Value;
return root.isExecutable(frameValue, arguments0Value_, this.isExecutableNode);
}
return getNext().executeBoolean_(frameValue, arguments0Value);
}
static BaseNode_ create(IsExecutableNodeGen root, Node isExecutableNode) {
return new IsExecutableNode_(root, isExecutableNode);
}
}
}
}
@GeneratedBy(ExecuteNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class ExecuteNodeFactory implements NodeFactory {
private static ExecuteNodeFactory executeNodeFactoryInstance;
private ExecuteNodeFactory() {
}
@Override
public Class getNodeClass() {
return ExecuteNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public ExecuteNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (executeNodeFactoryInstance == null) {
executeNodeFactoryInstance = new ExecuteNodeFactory();
}
return executeNodeFactoryInstance;
}
public static ExecuteNode create(RubyNode[] arguments) {
return new ExecuteNodeGen(arguments);
}
@GeneratedBy(ExecuteNode.class)
@SuppressFBWarnings("SA_LOCAL_SELF_COMPARISON")
public static final class ExecuteNodeGen extends ExecuteNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@CompilationFinal private boolean excludeExecuteForeignCached_;
@Child private BaseNode_ specialization_;
private ExecuteNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(ExecuteNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected ExecuteNodeGen root;
BaseNode_(ExecuteNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (ExecuteNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_, root.arguments1_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value, Object arguments1Value) {
return this.execute_((VirtualFrame) frameValue, arguments0Value, arguments1Value);
}
public abstract Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = root.arguments1_.execute(frameValue);
return execute_(frameValue, arguments0Value_, arguments1Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof TruffleObject && arguments1Value instanceof Object[]) {
Object[] arguments1Value_ = (Object[]) arguments1Value;
int cachedArgsLength1 = (arguments1Value_.length);
if ((arguments1Value_.length == cachedArgsLength1)) {
if (!root.excludeExecuteForeignCached_) {
Node executeNode1 = (root.createExecuteNode(cachedArgsLength1));
BranchProfile exceptionProfile1 = (BranchProfile.create());
SpecializationNode s = ExecuteForeignCachedNode_.create(root, cachedArgsLength1, executeNode1, exceptionProfile1);
if (countSame(s) < (root.getCacheLimit())) {
return s;
}
}
}
root.excludeExecuteForeignCached_ = true;
return ExecuteForeignUncachedNode_.create(root);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(ExecuteNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(ExecuteNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
return uninitialized(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(ExecuteNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(ExecuteNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(ExecuteNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value, arguments1Value));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
return getNext().execute_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(ExecuteNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "executeForeignCached(VirtualFrame, TruffleObject, Object[], int, Node, BranchProfile)", value = ExecuteNode.class)
private static final class ExecuteForeignCachedNode_ extends BaseNode_ {
private final int cachedArgsLength;
@Child private Node executeNode;
private final BranchProfile exceptionProfile;
ExecuteForeignCachedNode_(ExecuteNodeGen root, int cachedArgsLength, Node executeNode, BranchProfile exceptionProfile) {
super(root, 1);
this.cachedArgsLength = cachedArgsLength;
this.executeNode = executeNode;
this.exceptionProfile = exceptionProfile;
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value) {
if (newNode.getClass() == ExecuteForeignUncachedNode_.class) {
removeSame("Contained by executeForeignUncached(VirtualFrame, TruffleObject, Object[])");
}
return super.merge(newNode, frameValue, arguments0Value, arguments1Value);
}
@Override
public boolean isIdentical(SpecializationNode other, Frame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof TruffleObject && arguments1Value instanceof Object[]) {
Object[] arguments1Value_ = (Object[]) arguments1Value;
if ((arguments1Value_.length == this.cachedArgsLength)) {
return true;
}
}
return false;
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof TruffleObject && arguments1Value instanceof Object[]) {
TruffleObject arguments0Value_ = (TruffleObject) arguments0Value;
Object[] arguments1Value_ = (Object[]) arguments1Value;
if ((arguments1Value_.length == this.cachedArgsLength)) {
return root.executeForeignCached(frameValue, arguments0Value_, arguments1Value_, this.cachedArgsLength, this.executeNode, this.exceptionProfile);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(ExecuteNodeGen root, int cachedArgsLength, Node executeNode, BranchProfile exceptionProfile) {
return new ExecuteForeignCachedNode_(root, cachedArgsLength, executeNode, exceptionProfile);
}
}
@GeneratedBy(methodName = "executeForeignUncached(VirtualFrame, TruffleObject, Object[])", value = ExecuteNode.class)
private static final class ExecuteForeignUncachedNode_ extends BaseNode_ {
ExecuteForeignUncachedNode_(ExecuteNodeGen root) {
super(root, 2);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof TruffleObject && arguments1Value instanceof Object[]) {
TruffleObject arguments0Value_ = (TruffleObject) arguments0Value;
Object[] arguments1Value_ = (Object[]) arguments1Value;
return root.executeForeignUncached(frameValue, arguments0Value_, arguments1Value_);
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(ExecuteNodeGen root) {
return new ExecuteForeignUncachedNode_(root);
}
}
}
}
@GeneratedBy(InvokeNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class InvokeNodeFactory implements NodeFactory {
private static InvokeNodeFactory invokeNodeFactoryInstance;
private InvokeNodeFactory() {
}
@Override
public Class getNodeClass() {
return InvokeNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public InvokeNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (invokeNodeFactoryInstance == null) {
invokeNodeFactoryInstance = new InvokeNodeFactory();
}
return invokeNodeFactoryInstance;
}
public static InvokeNode create(RubyNode[] arguments) {
return new InvokeNodeGen(arguments);
}
@GeneratedBy(InvokeNode.class)
@SuppressFBWarnings("SA_LOCAL_SELF_COMPARISON")
public static final class InvokeNodeGen extends InvokeNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@Child private RubyNode arguments2_;
@CompilationFinal private boolean excludeInvokeCached_;
@Child private BaseNode_ specialization_;
private InvokeNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.arguments2_ = arguments != null && 2 < arguments.length ? arguments[2] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(InvokeNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected InvokeNodeGen root;
BaseNode_(InvokeNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (InvokeNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_, root.arguments1_, root.arguments2_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return this.execute_((VirtualFrame) frameValue, arguments0Value, arguments1Value, arguments2Value);
}
public abstract Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = root.arguments1_.execute(frameValue);
Object arguments2Value_ = root.arguments2_.execute(frameValue);
return execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof TruffleObject && arguments1Value instanceof DynamicObject && arguments2Value instanceof Object[]) {
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
Object[] arguments2Value_ = (Object[]) arguments2Value;
int cachedArgsLength1 = (arguments2Value_.length);
if ((RubyGuards.isRubyString(arguments1Value_) || RubyGuards.isRubySymbol(arguments1Value_)) && (arguments2Value_.length == cachedArgsLength1)) {
if (!root.excludeInvokeCached_) {
Node invokeNode1 = (root.createInvokeNode(cachedArgsLength1));
NameToJavaStringNode toJavaStringNode1 = (NameToJavaStringNode.create());
BranchProfile exceptionProfile1 = (BranchProfile.create());
SpecializationNode s = InvokeCachedNode_.create(root, cachedArgsLength1, invokeNode1, toJavaStringNode1, exceptionProfile1);
if (countSame(s) < (root.getCacheLimit())) {
return s;
}
}
}
if ((RubyGuards.isRubyString(arguments1Value_) || RubyGuards.isRubySymbol(arguments1Value_))) {
root.excludeInvokeCached_ = true;
return InvokeUncachedNode_.create(root);
}
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(InvokeNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(InvokeNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return uninitialized(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(InvokeNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(InvokeNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(InvokeNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value, arguments1Value, arguments2Value));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(InvokeNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "invokeCached(VirtualFrame, TruffleObject, DynamicObject, Object[], int, Node, NameToJavaStringNode, BranchProfile)", value = InvokeNode.class)
private static final class InvokeCachedNode_ extends BaseNode_ {
private final int cachedArgsLength;
@Child private Node invokeNode;
@Child private NameToJavaStringNode toJavaStringNode;
private final BranchProfile exceptionProfile;
InvokeCachedNode_(InvokeNodeGen root, int cachedArgsLength, Node invokeNode, NameToJavaStringNode toJavaStringNode, BranchProfile exceptionProfile) {
super(root, 1);
this.cachedArgsLength = cachedArgsLength;
this.invokeNode = invokeNode;
this.toJavaStringNode = toJavaStringNode;
this.exceptionProfile = exceptionProfile;
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (newNode.getClass() == InvokeUncachedNode_.class) {
removeSame("Contained by invokeUncached(VirtualFrame, TruffleObject, DynamicObject, Object[])");
}
return super.merge(newNode, frameValue, arguments0Value, arguments1Value, arguments2Value);
}
@Override
public boolean isIdentical(SpecializationNode other, Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof TruffleObject && arguments1Value instanceof DynamicObject && arguments2Value instanceof Object[]) {
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
Object[] arguments2Value_ = (Object[]) arguments2Value;
if ((RubyGuards.isRubyString(arguments1Value_) || RubyGuards.isRubySymbol(arguments1Value_)) && (arguments2Value_.length == this.cachedArgsLength)) {
return true;
}
}
return false;
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof TruffleObject && arguments1Value instanceof DynamicObject && arguments2Value instanceof Object[]) {
TruffleObject arguments0Value_ = (TruffleObject) arguments0Value;
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
Object[] arguments2Value_ = (Object[]) arguments2Value;
if ((RubyGuards.isRubyString(arguments1Value_) || RubyGuards.isRubySymbol(arguments1Value_)) && (arguments2Value_.length == this.cachedArgsLength)) {
return root.invokeCached(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, this.cachedArgsLength, this.invokeNode, this.toJavaStringNode, this.exceptionProfile);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(InvokeNodeGen root, int cachedArgsLength, Node invokeNode, NameToJavaStringNode toJavaStringNode, BranchProfile exceptionProfile) {
return new InvokeCachedNode_(root, cachedArgsLength, invokeNode, toJavaStringNode, exceptionProfile);
}
}
@GeneratedBy(methodName = "invokeUncached(VirtualFrame, TruffleObject, DynamicObject, Object[])", value = InvokeNode.class)
private static final class InvokeUncachedNode_ extends BaseNode_ {
InvokeUncachedNode_(InvokeNodeGen root) {
super(root, 2);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof TruffleObject && arguments1Value instanceof DynamicObject && arguments2Value instanceof Object[]) {
TruffleObject arguments0Value_ = (TruffleObject) arguments0Value;
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
Object[] arguments2Value_ = (Object[]) arguments2Value;
if ((RubyGuards.isRubyString(arguments1Value_) || RubyGuards.isRubySymbol(arguments1Value_))) {
return root.invokeUncached(frameValue, arguments0Value_, arguments1Value_, arguments2Value_);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(InvokeNodeGen root) {
return new InvokeUncachedNode_(root);
}
}
}
}
@GeneratedBy(HasSizeNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class HasSizeNodeFactory implements NodeFactory {
private static HasSizeNodeFactory hasSizeNodeFactoryInstance;
private HasSizeNodeFactory() {
}
@Override
public Class getNodeClass() {
return HasSizeNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public HasSizeNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (hasSizeNodeFactoryInstance == null) {
hasSizeNodeFactoryInstance = new HasSizeNodeFactory();
}
return hasSizeNodeFactoryInstance;
}
public static HasSizeNode create(RubyNode[] arguments) {
return new HasSizeNodeGen(arguments);
}
@GeneratedBy(HasSizeNode.class)
public static final class HasSizeNodeGen extends HasSizeNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private BaseNode_ specialization_;
private HasSizeNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
return specialization_.executeBoolean(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
private static TruffleObject expectTruffleObject(Object value) throws UnexpectedResultException {
if (value instanceof TruffleObject) {
return (TruffleObject) value;
}
throw new UnexpectedResultException(value);
}
@GeneratedBy(HasSizeNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected HasSizeNodeGen root;
BaseNode_(HasSizeNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (HasSizeNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.executeBoolean_((VirtualFrame) frameValue, arguments0Value);
}
public abstract boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
return executeBoolean_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public boolean executeBoolean(VirtualFrame frameValue) {
return (boolean) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (arguments0Value instanceof TruffleObject) {
Node hasSizeNode1 = (root.createHasSizeNode());
return HasSizeNode_.create(root, hasSizeNode1);
}
return null;
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(HasSizeNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(HasSizeNodeGen root) {
super(root, 2147483647);
}
@Override
public boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value) {
return (boolean) uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(HasSizeNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(methodName = "hasSize(VirtualFrame, TruffleObject, Node)", value = HasSizeNode.class)
private static final class HasSizeNode_ extends BaseNode_ {
@Child private Node hasSizeNode;
HasSizeNode_(HasSizeNodeGen root, Node hasSizeNode) {
super(root, 1);
this.hasSizeNode = hasSizeNode;
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
TruffleObject arguments0Value_;
try {
arguments0Value_ = expectTruffleObject(root.arguments0_.execute(frameValue));
} catch (UnexpectedResultException ex) {
return getNext().executeBoolean_(frameValue, ex.getResult());
}
return root.hasSize(frameValue, arguments0Value_, this.hasSizeNode);
}
@Override
public boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value) {
if (arguments0Value instanceof TruffleObject) {
TruffleObject arguments0Value_ = (TruffleObject) arguments0Value;
return root.hasSize(frameValue, arguments0Value_, this.hasSizeNode);
}
return getNext().executeBoolean_(frameValue, arguments0Value);
}
static BaseNode_ create(HasSizeNodeGen root, Node hasSizeNode) {
return new HasSizeNode_(root, hasSizeNode);
}
}
}
}
@GeneratedBy(SizeNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class SizeNodeFactory implements NodeFactory {
private static SizeNodeFactory sizeNodeFactoryInstance;
private SizeNodeFactory() {
}
@Override
public Class getNodeClass() {
return SizeNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public SizeNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (sizeNodeFactoryInstance == null) {
sizeNodeFactoryInstance = new SizeNodeFactory();
}
return sizeNodeFactoryInstance;
}
public static SizeNode create(RubyNode[] arguments) {
return new SizeNodeGen(arguments);
}
@GeneratedBy(SizeNode.class)
public static final class SizeNodeGen extends SizeNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private BaseNode_ specialization_;
private SizeNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(SizeNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected SizeNodeGen root;
BaseNode_(SizeNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (SizeNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.execute_((VirtualFrame) frameValue, arguments0Value);
}
public abstract Object execute_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
return execute_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (arguments0Value instanceof String) {
return Size0Node_.create(root);
}
if (arguments0Value instanceof TruffleObject) {
Node getSizeNode2 = (root.createGetSizeNode());
BranchProfile exceptionProfile2 = (BranchProfile.create());
return Size1Node_.create(root, getSizeNode2, exceptionProfile2);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(SizeNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(SizeNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
return uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(SizeNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(SizeNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(SizeNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().execute_(frameValue, arguments0Value);
}
static BaseNode_ create(SizeNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "size(String)", value = SizeNode.class)
private static final class Size0Node_ extends BaseNode_ {
Size0Node_(SizeNodeGen root) {
super(root, 1);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
if (arguments0Value instanceof String) {
String arguments0Value_ = (String) arguments0Value;
return root.size(arguments0Value_);
}
return getNext().execute_(frameValue, arguments0Value);
}
static BaseNode_ create(SizeNodeGen root) {
return new Size0Node_(root);
}
}
@GeneratedBy(methodName = "size(VirtualFrame, TruffleObject, Node, BranchProfile)", value = SizeNode.class)
private static final class Size1Node_ extends BaseNode_ {
@Child private Node getSizeNode;
private final BranchProfile exceptionProfile;
Size1Node_(SizeNodeGen root, Node getSizeNode, BranchProfile exceptionProfile) {
super(root, 2);
this.getSizeNode = getSizeNode;
this.exceptionProfile = exceptionProfile;
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
if (arguments0Value instanceof TruffleObject) {
TruffleObject arguments0Value_ = (TruffleObject) arguments0Value;
return root.size(frameValue, arguments0Value_, this.getSizeNode, this.exceptionProfile);
}
return getNext().execute_(frameValue, arguments0Value);
}
static BaseNode_ create(SizeNodeGen root, Node getSizeNode, BranchProfile exceptionProfile) {
return new Size1Node_(root, getSizeNode, exceptionProfile);
}
}
}
}
@GeneratedBy(BoxedNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class BoxedNodeFactory implements NodeFactory {
private static BoxedNodeFactory boxedNodeFactoryInstance;
private BoxedNodeFactory() {
}
@Override
public Class getNodeClass() {
return BoxedNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public BoxedNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (boxedNodeFactoryInstance == null) {
boxedNodeFactoryInstance = new BoxedNodeFactory();
}
return boxedNodeFactoryInstance;
}
public static BoxedNode create(RubyNode[] arguments) {
return new BoxedNodeGen(arguments);
}
@GeneratedBy(BoxedNode.class)
public static final class BoxedNodeGen extends BoxedNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@CompilationFinal private Class> arguments0Type_;
@Child private BaseNode_ specialization_;
private BoxedNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
return specialization_.executeBoolean(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
private static CharSequence expectCharSequence(Object value) throws UnexpectedResultException {
if (value instanceof CharSequence) {
return (CharSequence) value;
}
throw new UnexpectedResultException(value);
}
private static TruffleObject expectTruffleObject(Object value) throws UnexpectedResultException {
if (value instanceof TruffleObject) {
return (TruffleObject) value;
}
throw new UnexpectedResultException(value);
}
private static byte expectByte(Object value) throws UnexpectedResultException {
if (value instanceof Byte) {
return (byte) value;
}
throw new UnexpectedResultException(value);
}
private static float expectFloat(Object value) throws UnexpectedResultException {
if (value instanceof Float) {
return (float) value;
}
throw new UnexpectedResultException(value);
}
private static short expectShort(Object value) throws UnexpectedResultException {
if (value instanceof Short) {
return (short) value;
}
throw new UnexpectedResultException(value);
}
@GeneratedBy(BoxedNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected BoxedNodeGen root;
BaseNode_(BoxedNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (BoxedNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.executeBoolean_((VirtualFrame) frameValue, arguments0Value);
}
public abstract boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return executeBoolean_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public boolean executeBoolean(VirtualFrame frameValue) {
return (boolean) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (arguments0Value instanceof Boolean) {
return IsBoxed0Node_.create(root);
}
if (arguments0Value instanceof Byte) {
return IsBoxed1Node_.create(root);
}
if (arguments0Value instanceof Short) {
return IsBoxed2Node_.create(root);
}
if (RubyTypesGen.isImplicitInteger(arguments0Value)) {
return IsBoxed3Node_.create(root, arguments0Value);
}
if (RubyTypesGen.isImplicitLong(arguments0Value)) {
return IsBoxed4Node_.create(root, arguments0Value);
}
if (arguments0Value instanceof Float) {
return IsBoxed5Node_.create(root);
}
if (RubyTypesGen.isImplicitDouble(arguments0Value)) {
return IsBoxed6Node_.create(root, arguments0Value);
}
if (arguments0Value instanceof CharSequence) {
return IsBoxed7Node_.create(root);
}
if (arguments0Value instanceof TruffleObject) {
Node isBoxedNode9 = (root.createIsBoxedNode());
BranchProfile exceptionProfile9 = (BranchProfile.create());
return IsBoxed8Node_.create(root, isBoxedNode9, exceptionProfile9);
}
return null;
}
@Override
protected final SpecializationNode createFallback() {
return FallbackNode_.create(root);
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments0_(Frame frameValue) {
Class> arguments0Type_ = root.arguments0Type_;
try {
if (arguments0Type_ == boolean.class) {
return root.arguments0_.executeBoolean((VirtualFrame) frameValue);
} else if (arguments0Type_ == double.class) {
return root.arguments0_.executeDouble((VirtualFrame) frameValue);
} else if (arguments0Type_ == int.class) {
return root.arguments0_.executeInteger((VirtualFrame) frameValue);
} else if (arguments0Type_ == long.class) {
return root.arguments0_.executeLong((VirtualFrame) frameValue);
} else if (arguments0Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments0_.execute((VirtualFrame) frameValue);
if (_value instanceof Boolean) {
_type = boolean.class;
} else if (_value instanceof Double) {
_type = double.class;
} else if (_value instanceof Integer) {
_type = int.class;
} else if (_value instanceof Long) {
_type = long.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments0Type_ = _type;
}
} else {
return root.arguments0_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments0Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(BoxedNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(BoxedNodeGen root) {
super(root, 2147483647);
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return (boolean) uninitialized(frameValue, arguments0Value_);
}
@Override
public boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value) {
return (boolean) uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(BoxedNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(BoxedNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(BoxedNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
return getNext().executeBoolean_(frameValue, arguments0Value_);
}
@Override
public boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().executeBoolean_(frameValue, arguments0Value);
}
static BaseNode_ create(BoxedNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "isBoxed(boolean)", value = BoxedNode.class)
private static final class IsBoxed0Node_ extends BaseNode_ {
IsBoxed0Node_(BoxedNodeGen root) {
super(root, 1);
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeBoolean(frameValue);
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
boolean arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeBoolean(frameValue);
} catch (UnexpectedResultException ex) {
return getNext().executeBoolean_(frameValue, ex.getResult());
}
return root.isBoxed(arguments0Value_);
}
@Override
public boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value) {
if (arguments0Value instanceof Boolean) {
boolean arguments0Value_ = (boolean) arguments0Value;
return root.isBoxed(arguments0Value_);
}
return getNext().executeBoolean_(frameValue, arguments0Value);
}
static BaseNode_ create(BoxedNodeGen root) {
return new IsBoxed0Node_(root);
}
}
@GeneratedBy(methodName = "isBoxed(byte)", value = BoxedNode.class)
private static final class IsBoxed1Node_ extends BaseNode_ {
IsBoxed1Node_(BoxedNodeGen root) {
super(root, 2);
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
byte arguments0Value_;
try {
arguments0Value_ = expectByte(root.arguments0_.execute(frameValue));
} catch (UnexpectedResultException ex) {
return getNext().executeBoolean_(frameValue, ex.getResult());
}
return root.isBoxed(arguments0Value_);
}
@Override
public boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value) {
if (arguments0Value instanceof Byte) {
byte arguments0Value_ = (byte) arguments0Value;
return root.isBoxed(arguments0Value_);
}
return getNext().executeBoolean_(frameValue, arguments0Value);
}
static BaseNode_ create(BoxedNodeGen root) {
return new IsBoxed1Node_(root);
}
}
@GeneratedBy(methodName = "isBoxed(short)", value = BoxedNode.class)
private static final class IsBoxed2Node_ extends BaseNode_ {
IsBoxed2Node_(BoxedNodeGen root) {
super(root, 3);
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
short arguments0Value_;
try {
arguments0Value_ = expectShort(root.arguments0_.execute(frameValue));
} catch (UnexpectedResultException ex) {
return getNext().executeBoolean_(frameValue, ex.getResult());
}
return root.isBoxed(arguments0Value_);
}
@Override
public boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value) {
if (arguments0Value instanceof Short) {
short arguments0Value_ = (short) arguments0Value;
return root.isBoxed(arguments0Value_);
}
return getNext().executeBoolean_(frameValue, arguments0Value);
}
static BaseNode_ create(BoxedNodeGen root) {
return new IsBoxed2Node_(root);
}
}
@GeneratedBy(methodName = "isBoxed(int)", value = BoxedNode.class)
private static final class IsBoxed3Node_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
IsBoxed3Node_(BoxedNodeGen root, Object arguments0Value) {
super(root, 4);
this.arguments0ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((IsBoxed3Node_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeBoolean(frameValue);
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
int arguments0Value_;
try {
if (arguments0ImplicitType == int.class) {
arguments0Value_ = root.arguments0_.executeInteger(frameValue);
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitInteger(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeBoolean_(frameValue, ex.getResult());
}
return root.isBoxed(arguments0Value_);
}
@Override
public boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitInteger(arguments0Value, arguments0ImplicitType)) {
int arguments0Value_ = RubyTypesGen.asImplicitInteger(arguments0Value, arguments0ImplicitType);
return root.isBoxed(arguments0Value_);
}
return getNext().executeBoolean_(frameValue, arguments0Value);
}
static BaseNode_ create(BoxedNodeGen root, Object arguments0Value) {
return new IsBoxed3Node_(root, arguments0Value);
}
}
@GeneratedBy(methodName = "isBoxed(long)", value = BoxedNode.class)
private static final class IsBoxed4Node_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
IsBoxed4Node_(BoxedNodeGen root, Object arguments0Value) {
super(root, 5);
this.arguments0ImplicitType = RubyTypesGen.getImplicitLongClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((IsBoxed4Node_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeBoolean(frameValue);
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
long arguments0Value_;
try {
if (arguments0ImplicitType == long.class) {
arguments0Value_ = root.arguments0_.executeLong(frameValue);
} else if (arguments0ImplicitType == int.class) {
arguments0Value_ = RubyTypes.promoteToLong(root.arguments0_.executeInteger(frameValue));
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitLong(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeBoolean_(frameValue, ex.getResult());
}
return root.isBoxed(arguments0Value_);
}
@Override
public boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitLong(arguments0Value, arguments0ImplicitType)) {
long arguments0Value_ = RubyTypesGen.asImplicitLong(arguments0Value, arguments0ImplicitType);
return root.isBoxed(arguments0Value_);
}
return getNext().executeBoolean_(frameValue, arguments0Value);
}
static BaseNode_ create(BoxedNodeGen root, Object arguments0Value) {
return new IsBoxed4Node_(root, arguments0Value);
}
}
@GeneratedBy(methodName = "isBoxed(float)", value = BoxedNode.class)
private static final class IsBoxed5Node_ extends BaseNode_ {
IsBoxed5Node_(BoxedNodeGen root) {
super(root, 6);
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
float arguments0Value_;
try {
arguments0Value_ = expectFloat(root.arguments0_.execute(frameValue));
} catch (UnexpectedResultException ex) {
return getNext().executeBoolean_(frameValue, ex.getResult());
}
return root.isBoxed(arguments0Value_);
}
@Override
public boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value) {
if (arguments0Value instanceof Float) {
float arguments0Value_ = (float) arguments0Value;
return root.isBoxed(arguments0Value_);
}
return getNext().executeBoolean_(frameValue, arguments0Value);
}
static BaseNode_ create(BoxedNodeGen root) {
return new IsBoxed5Node_(root);
}
}
@GeneratedBy(methodName = "isBoxed(double)", value = BoxedNode.class)
private static final class IsBoxed6Node_ extends BaseNode_ {
private final Class> arguments0ImplicitType;
IsBoxed6Node_(BoxedNodeGen root, Object arguments0Value) {
super(root, 7);
this.arguments0ImplicitType = RubyTypesGen.getImplicitDoubleClass(arguments0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments0ImplicitType == ((IsBoxed6Node_) other).arguments0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeBoolean(frameValue);
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
double arguments0Value_;
try {
if (arguments0ImplicitType == double.class) {
arguments0Value_ = root.arguments0_.executeDouble(frameValue);
} else {
Object arguments0Value__ = executeArguments0_(frameValue);
arguments0Value_ = RubyTypesGen.expectImplicitDouble(arguments0Value__, arguments0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeBoolean_(frameValue, ex.getResult());
}
return root.isBoxed(arguments0Value_);
}
@Override
public boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value) {
if (RubyTypesGen.isImplicitDouble(arguments0Value, arguments0ImplicitType)) {
double arguments0Value_ = RubyTypesGen.asImplicitDouble(arguments0Value, arguments0ImplicitType);
return root.isBoxed(arguments0Value_);
}
return getNext().executeBoolean_(frameValue, arguments0Value);
}
static BaseNode_ create(BoxedNodeGen root, Object arguments0Value) {
return new IsBoxed6Node_(root, arguments0Value);
}
}
@GeneratedBy(methodName = "isBoxed(CharSequence)", value = BoxedNode.class)
private static final class IsBoxed7Node_ extends BaseNode_ {
IsBoxed7Node_(BoxedNodeGen root) {
super(root, 8);
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
CharSequence arguments0Value_;
try {
arguments0Value_ = expectCharSequence(root.arguments0_.execute(frameValue));
} catch (UnexpectedResultException ex) {
return getNext().executeBoolean_(frameValue, ex.getResult());
}
return root.isBoxed(arguments0Value_);
}
@Override
public boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value) {
if (arguments0Value instanceof CharSequence) {
CharSequence arguments0Value_ = (CharSequence) arguments0Value;
return root.isBoxed(arguments0Value_);
}
return getNext().executeBoolean_(frameValue, arguments0Value);
}
static BaseNode_ create(BoxedNodeGen root) {
return new IsBoxed7Node_(root);
}
}
@GeneratedBy(methodName = "isBoxed(VirtualFrame, TruffleObject, Node, BranchProfile)", value = BoxedNode.class)
private static final class IsBoxed8Node_ extends BaseNode_ {
@Child private Node isBoxedNode;
private final BranchProfile exceptionProfile;
IsBoxed8Node_(BoxedNodeGen root, Node isBoxedNode, BranchProfile exceptionProfile) {
super(root, 9);
this.isBoxedNode = isBoxedNode;
this.exceptionProfile = exceptionProfile;
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
TruffleObject arguments0Value_;
try {
arguments0Value_ = expectTruffleObject(root.arguments0_.execute(frameValue));
} catch (UnexpectedResultException ex) {
return getNext().executeBoolean_(frameValue, ex.getResult());
}
return root.isBoxed(frameValue, arguments0Value_, this.isBoxedNode, this.exceptionProfile);
}
@Override
public boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value) {
if (arguments0Value instanceof TruffleObject) {
TruffleObject arguments0Value_ = (TruffleObject) arguments0Value;
return root.isBoxed(frameValue, arguments0Value_, this.isBoxedNode, this.exceptionProfile);
}
return getNext().executeBoolean_(frameValue, arguments0Value);
}
static BaseNode_ create(BoxedNodeGen root, Node isBoxedNode, BranchProfile exceptionProfile) {
return new IsBoxed8Node_(root, isBoxedNode, exceptionProfile);
}
}
@GeneratedBy(methodName = "isBoxed(Object)", value = BoxedNode.class)
private static final class FallbackNode_ extends BaseNode_ {
FallbackNode_(BoxedNodeGen root) {
super(root, 2147483646);
}
@TruffleBoundary
private boolean guardFallback(Object arguments0Value) {
return createNext(null, arguments0Value) == null;
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
Object arguments0Value_ = executeArguments0_(frameValue);
if (guardFallback(arguments0Value_)) {
return root.isBoxed(arguments0Value_);
}
return getNext().executeBoolean_(frameValue, arguments0Value_);
}
@Override
public boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value) {
if (guardFallback(arguments0Value)) {
return root.isBoxed(arguments0Value);
}
return getNext().executeBoolean_(frameValue, arguments0Value);
}
static BaseNode_ create(BoxedNodeGen root) {
return new FallbackNode_(root);
}
}
}
}
@GeneratedBy(UnboxNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class UnboxNodeFactory implements NodeFactory {
private static UnboxNodeFactory unboxNodeFactoryInstance;
private UnboxNodeFactory() {
}
@Override
public Class getNodeClass() {
return UnboxNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public UnboxNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (unboxNodeFactoryInstance == null) {
unboxNodeFactoryInstance = new UnboxNodeFactory();
}
return unboxNodeFactoryInstance;
}
public static UnboxNode create(RubyNode[] arguments) {
return new UnboxNodeGen(arguments);
}
@GeneratedBy(UnboxNode.class)
public static final class UnboxNodeGen extends UnboxNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private BaseNode_ specialization_;
private UnboxNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(UnboxNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected UnboxNodeGen root;
BaseNode_(UnboxNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (UnboxNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.execute_((VirtualFrame) frameValue, arguments0Value);
}
public abstract Object execute_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
return execute_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (arguments0Value instanceof CharSequence) {
return Unbox0Node_.create(root);
}
if (arguments0Value instanceof TruffleObject) {
Node unboxNode2 = (root.createUnboxNode());
BranchProfile exceptionProfile2 = (BranchProfile.create());
return Unbox1Node_.create(root, unboxNode2, exceptionProfile2);
}
return null;
}
@Override
protected final SpecializationNode createFallback() {
return FallbackNode_.create(root);
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(UnboxNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(UnboxNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
return uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(UnboxNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(UnboxNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(UnboxNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().execute_(frameValue, arguments0Value);
}
static BaseNode_ create(UnboxNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "unbox(CharSequence)", value = UnboxNode.class)
private static final class Unbox0Node_ extends BaseNode_ {
Unbox0Node_(UnboxNodeGen root) {
super(root, 1);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
if (arguments0Value instanceof CharSequence) {
CharSequence arguments0Value_ = (CharSequence) arguments0Value;
return root.unbox(arguments0Value_);
}
return getNext().execute_(frameValue, arguments0Value);
}
static BaseNode_ create(UnboxNodeGen root) {
return new Unbox0Node_(root);
}
}
@GeneratedBy(methodName = "unbox(VirtualFrame, TruffleObject, Node, BranchProfile)", value = UnboxNode.class)
private static final class Unbox1Node_ extends BaseNode_ {
@Child private Node unboxNode;
private final BranchProfile exceptionProfile;
Unbox1Node_(UnboxNodeGen root, Node unboxNode, BranchProfile exceptionProfile) {
super(root, 2);
this.unboxNode = unboxNode;
this.exceptionProfile = exceptionProfile;
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
if (arguments0Value instanceof TruffleObject) {
TruffleObject arguments0Value_ = (TruffleObject) arguments0Value;
return root.unbox(frameValue, arguments0Value_, this.unboxNode, this.exceptionProfile);
}
return getNext().execute_(frameValue, arguments0Value);
}
static BaseNode_ create(UnboxNodeGen root, Node unboxNode, BranchProfile exceptionProfile) {
return new Unbox1Node_(root, unboxNode, exceptionProfile);
}
}
@GeneratedBy(methodName = "unbox(Object)", value = UnboxNode.class)
private static final class FallbackNode_ extends BaseNode_ {
FallbackNode_(UnboxNodeGen root) {
super(root, 2147483646);
}
@TruffleBoundary
private boolean guardFallback(Object arguments0Value) {
return createNext(null, arguments0Value) == null;
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
if (guardFallback(arguments0Value)) {
return root.unbox(arguments0Value);
}
return getNext().execute_(frameValue, arguments0Value);
}
static BaseNode_ create(UnboxNodeGen root) {
return new FallbackNode_(root);
}
}
}
}
@GeneratedBy(NullNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class NullNodeFactory implements NodeFactory {
private static NullNodeFactory nullNodeFactoryInstance;
private NullNodeFactory() {
}
@Override
public Class getNodeClass() {
return NullNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public NullNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (nullNodeFactoryInstance == null) {
nullNodeFactoryInstance = new NullNodeFactory();
}
return nullNodeFactoryInstance;
}
public static NullNode create(RubyNode[] arguments) {
return new NullNodeGen(arguments);
}
@GeneratedBy(NullNode.class)
public static final class NullNodeGen extends NullNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private BaseNode_ specialization_;
private NullNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
return specialization_.executeBoolean(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
private static TruffleObject expectTruffleObject(Object value) throws UnexpectedResultException {
if (value instanceof TruffleObject) {
return (TruffleObject) value;
}
throw new UnexpectedResultException(value);
}
@GeneratedBy(NullNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected NullNodeGen root;
BaseNode_(NullNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (NullNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.executeBoolean_((VirtualFrame) frameValue, arguments0Value);
}
public abstract boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
return executeBoolean_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public boolean executeBoolean(VirtualFrame frameValue) {
return (boolean) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (arguments0Value instanceof TruffleObject) {
Node isNullNode1 = (root.createIsNullNode());
return IsNullNode_.create(root, isNullNode1);
}
return null;
}
@Override
protected final SpecializationNode createFallback() {
return FallbackNode_.create(root);
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(NullNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(NullNodeGen root) {
super(root, 2147483647);
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
return (boolean) uninitialized(frameValue, arguments0Value_);
}
@Override
public boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value) {
return (boolean) uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(NullNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(NullNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(NullNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value));
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
return getNext().executeBoolean_(frameValue, arguments0Value_);
}
@Override
public boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value) {
return getNext().executeBoolean_(frameValue, arguments0Value);
}
static BaseNode_ create(NullNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "isNull(VirtualFrame, TruffleObject, Node)", value = NullNode.class)
private static final class IsNullNode_ extends BaseNode_ {
@Child private Node isNullNode;
IsNullNode_(NullNodeGen root, Node isNullNode) {
super(root, 1);
this.isNullNode = isNullNode;
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
TruffleObject arguments0Value_;
try {
arguments0Value_ = expectTruffleObject(root.arguments0_.execute(frameValue));
} catch (UnexpectedResultException ex) {
return getNext().executeBoolean_(frameValue, ex.getResult());
}
return root.isNull(frameValue, arguments0Value_, this.isNullNode);
}
@Override
public boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value) {
if (arguments0Value instanceof TruffleObject) {
TruffleObject arguments0Value_ = (TruffleObject) arguments0Value;
return root.isNull(frameValue, arguments0Value_, this.isNullNode);
}
return getNext().executeBoolean_(frameValue, arguments0Value);
}
static BaseNode_ create(NullNodeGen root, Node isNullNode) {
return new IsNullNode_(root, isNullNode);
}
}
@GeneratedBy(methodName = "isNull(Object)", value = NullNode.class)
private static final class FallbackNode_ extends BaseNode_ {
FallbackNode_(NullNodeGen root) {
super(root, 2147483646);
}
@TruffleBoundary
private boolean guardFallback(Object arguments0Value) {
return createNext(null, arguments0Value) == null;
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
if (guardFallback(arguments0Value_)) {
return root.isNull(arguments0Value_);
}
return getNext().executeBoolean_(frameValue, arguments0Value_);
}
@Override
public boolean executeBoolean_(VirtualFrame frameValue, Object arguments0Value) {
if (guardFallback(arguments0Value)) {
return root.isNull(arguments0Value);
}
return getNext().executeBoolean_(frameValue, arguments0Value);
}
static BaseNode_ create(NullNodeGen root) {
return new FallbackNode_(root);
}
}
}
}
@GeneratedBy(ReadNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class ReadNodeFactory implements NodeFactory {
private static ReadNodeFactory readNodeFactoryInstance;
private ReadNodeFactory() {
}
@Override
public Class getNodeClass() {
return ReadNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public ReadNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (readNodeFactoryInstance == null) {
readNodeFactoryInstance = new ReadNodeFactory();
}
return readNodeFactoryInstance;
}
public static ReadNode create(RubyNode[] arguments) {
return new ReadNodeGen(arguments);
}
@GeneratedBy(ReadNode.class)
@SuppressFBWarnings("SA_LOCAL_SELF_COMPARISON")
public static final class ReadNodeGen extends ReadNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@CompilationFinal private boolean excludeReadCached_;
@Child private BaseNode_ specialization_;
private ReadNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(ReadNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected ReadNodeGen root;
BaseNode_(ReadNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (ReadNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_, root.arguments1_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value, Object arguments1Value) {
return this.execute_((VirtualFrame) frameValue, arguments0Value, arguments1Value);
}
public abstract Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = root.arguments1_.execute(frameValue);
return execute_(frameValue, arguments0Value_, arguments1Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof TruffleObject) {
if ((!(RubyGuards.isRubySymbol(arguments1Value))) && (!(RubyGuards.isRubyString(arguments1Value)))) {
Node readNode1 = (ReadNode.createReadNode());
BranchProfile exceptionProfile1 = (BranchProfile.create());
return Read0Node_.create(root, readNode1, exceptionProfile1);
}
if (arguments1Value instanceof DynamicObject) {
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
DynamicObject cachedIdentifier2 = (arguments1Value_);
if ((arguments1Value_ == cachedIdentifier2) && (RubyGuards.isRubySymbol(cachedIdentifier2))) {
String identifierString2 = (cachedIdentifier2.toString());
Node readNode2 = (ReadNode.createReadNode());
BranchProfile exceptionProfile2 = (BranchProfile.create());
SpecializationNode s = Read1Node_.create(root, cachedIdentifier2, identifierString2, readNode2, exceptionProfile2);
if (countSame(s) < (3)) {
return s;
}
}
Rope cachedIdentifier3 = (StringCachingGuards.privatizeRope(arguments1Value_));
if ((RubyGuards.isRubyString(arguments1Value_)) && (StringCachingGuards.ropesEqual(arguments1Value_, cachedIdentifier3))) {
if (!root.excludeReadCached_) {
String identifierString3 = (cachedIdentifier3.toString());
Node readNode3 = (ReadNode.createReadNode());
BranchProfile exceptionProfile3 = (BranchProfile.create());
SpecializationNode s = ReadCachedNode_.create(root, cachedIdentifier3, identifierString3, readNode3, exceptionProfile3);
if (countSame(s) < (root.getCacheLimit())) {
return s;
}
}
}
if ((RubyGuards.isRubyString(arguments1Value_))) {
Node readNode4 = (ReadNode.createReadNode());
BranchProfile exceptionProfile4 = (BranchProfile.create());
root.excludeReadCached_ = true;
return ReadUncachedNode_.create(root, readNode4, exceptionProfile4);
}
}
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(ReadNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(ReadNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
return uninitialized(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(ReadNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(ReadNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(ReadNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value, arguments1Value));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
return getNext().execute_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(ReadNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "read(VirtualFrame, TruffleObject, Object, Node, BranchProfile)", value = ReadNode.class)
private static final class Read0Node_ extends BaseNode_ {
@Child private Node readNode;
private final BranchProfile exceptionProfile;
Read0Node_(ReadNodeGen root, Node readNode, BranchProfile exceptionProfile) {
super(root, 1);
this.readNode = readNode;
this.exceptionProfile = exceptionProfile;
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof TruffleObject) {
TruffleObject arguments0Value_ = (TruffleObject) arguments0Value;
if ((!(RubyGuards.isRubySymbol(arguments1Value))) && (!(RubyGuards.isRubyString(arguments1Value)))) {
return root.read(frameValue, arguments0Value_, arguments1Value, this.readNode, this.exceptionProfile);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(ReadNodeGen root, Node readNode, BranchProfile exceptionProfile) {
return new Read0Node_(root, readNode, exceptionProfile);
}
}
@GeneratedBy(methodName = "read(VirtualFrame, TruffleObject, DynamicObject, DynamicObject, String, Node, BranchProfile)", value = ReadNode.class)
private static final class Read1Node_ extends BaseNode_ {
private final DynamicObject cachedIdentifier;
private final String identifierString;
@Child private Node readNode;
private final BranchProfile exceptionProfile;
Read1Node_(ReadNodeGen root, DynamicObject cachedIdentifier, String identifierString, Node readNode, BranchProfile exceptionProfile) {
super(root, 2);
this.cachedIdentifier = cachedIdentifier;
this.identifierString = identifierString;
this.readNode = readNode;
this.exceptionProfile = exceptionProfile;
}
@Override
public boolean isIdentical(SpecializationNode other, Frame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof TruffleObject && arguments1Value instanceof DynamicObject) {
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
if ((arguments1Value_ == this.cachedIdentifier)) {
assert (RubyGuards.isRubySymbol(this.cachedIdentifier));
return true;
}
}
return false;
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof TruffleObject && arguments1Value instanceof DynamicObject) {
TruffleObject arguments0Value_ = (TruffleObject) arguments0Value;
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
if ((arguments1Value_ == this.cachedIdentifier)) {
assert (RubyGuards.isRubySymbol(this.cachedIdentifier));
return root.read(frameValue, arguments0Value_, arguments1Value_, this.cachedIdentifier, this.identifierString, this.readNode, this.exceptionProfile);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(ReadNodeGen root, DynamicObject cachedIdentifier, String identifierString, Node readNode, BranchProfile exceptionProfile) {
return new Read1Node_(root, cachedIdentifier, identifierString, readNode, exceptionProfile);
}
}
@GeneratedBy(methodName = "readCached(VirtualFrame, TruffleObject, DynamicObject, Rope, String, Node, BranchProfile)", value = ReadNode.class)
private static final class ReadCachedNode_ extends BaseNode_ {
private final Rope cachedIdentifier;
private final String identifierString;
@Child private Node readNode;
private final BranchProfile exceptionProfile;
ReadCachedNode_(ReadNodeGen root, Rope cachedIdentifier, String identifierString, Node readNode, BranchProfile exceptionProfile) {
super(root, 3);
this.cachedIdentifier = cachedIdentifier;
this.identifierString = identifierString;
this.readNode = readNode;
this.exceptionProfile = exceptionProfile;
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value) {
if (newNode.getClass() == ReadUncachedNode_.class) {
removeSame("Contained by readUncached(VirtualFrame, TruffleObject, DynamicObject, Node, BranchProfile)");
}
return super.merge(newNode, frameValue, arguments0Value, arguments1Value);
}
@Override
public boolean isIdentical(SpecializationNode other, Frame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof TruffleObject && arguments1Value instanceof DynamicObject) {
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
if ((RubyGuards.isRubyString(arguments1Value_)) && (StringCachingGuards.ropesEqual(arguments1Value_, this.cachedIdentifier))) {
return true;
}
}
return false;
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof TruffleObject && arguments1Value instanceof DynamicObject) {
TruffleObject arguments0Value_ = (TruffleObject) arguments0Value;
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
if ((RubyGuards.isRubyString(arguments1Value_)) && (StringCachingGuards.ropesEqual(arguments1Value_, this.cachedIdentifier))) {
return root.readCached(frameValue, arguments0Value_, arguments1Value_, this.cachedIdentifier, this.identifierString, this.readNode, this.exceptionProfile);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(ReadNodeGen root, Rope cachedIdentifier, String identifierString, Node readNode, BranchProfile exceptionProfile) {
return new ReadCachedNode_(root, cachedIdentifier, identifierString, readNode, exceptionProfile);
}
}
@GeneratedBy(methodName = "readUncached(VirtualFrame, TruffleObject, DynamicObject, Node, BranchProfile)", value = ReadNode.class)
private static final class ReadUncachedNode_ extends BaseNode_ {
@Child private Node readNode;
private final BranchProfile exceptionProfile;
ReadUncachedNode_(ReadNodeGen root, Node readNode, BranchProfile exceptionProfile) {
super(root, 4);
this.readNode = readNode;
this.exceptionProfile = exceptionProfile;
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof TruffleObject && arguments1Value instanceof DynamicObject) {
TruffleObject arguments0Value_ = (TruffleObject) arguments0Value;
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
if ((RubyGuards.isRubyString(arguments1Value_))) {
return root.readUncached(frameValue, arguments0Value_, arguments1Value_, this.readNode, this.exceptionProfile);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(ReadNodeGen root, Node readNode, BranchProfile exceptionProfile) {
return new ReadUncachedNode_(root, readNode, exceptionProfile);
}
}
}
}
@GeneratedBy(WriteNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class WriteNodeFactory implements NodeFactory {
private static WriteNodeFactory writeNodeFactoryInstance;
private WriteNodeFactory() {
}
@Override
public Class getNodeClass() {
return WriteNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public WriteNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (writeNodeFactoryInstance == null) {
writeNodeFactoryInstance = new WriteNodeFactory();
}
return writeNodeFactoryInstance;
}
public static WriteNode create(RubyNode[] arguments) {
return new WriteNodeGen(arguments);
}
@GeneratedBy(WriteNode.class)
@SuppressFBWarnings("SA_LOCAL_SELF_COMPARISON")
public static final class WriteNodeGen extends WriteNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@Child private RubyNode arguments2_;
@CompilationFinal private boolean excludeWriteCached_;
@Child private BaseNode_ specialization_;
private WriteNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.arguments2_ = arguments != null && 2 < arguments.length ? arguments[2] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(WriteNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected WriteNodeGen root;
BaseNode_(WriteNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (WriteNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_, root.arguments1_, root.arguments2_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return this.execute_((VirtualFrame) frameValue, arguments0Value, arguments1Value, arguments2Value);
}
public abstract Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = root.arguments1_.execute(frameValue);
Object arguments2Value_ = root.arguments2_.execute(frameValue);
return execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof TruffleObject) {
if ((!(RubyGuards.isRubySymbol(arguments1Value))) && (!(RubyGuards.isRubyString(arguments1Value)))) {
Node writeNode1 = (WriteNode.createWriteNode());
BranchProfile exceptionProfile1 = (BranchProfile.create());
return Write0Node_.create(root, writeNode1, exceptionProfile1);
}
if (arguments1Value instanceof DynamicObject) {
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
DynamicObject cachedIdentifier2 = (arguments1Value_);
if ((arguments1Value_ == cachedIdentifier2) && (RubyGuards.isRubySymbol(cachedIdentifier2))) {
String identifierString2 = (cachedIdentifier2.toString());
Node writeNode2 = (WriteNode.createWriteNode());
BranchProfile exceptionProfile2 = (BranchProfile.create());
SpecializationNode s = Write1Node_.create(root, cachedIdentifier2, identifierString2, writeNode2, exceptionProfile2);
if (countSame(s) < (3)) {
return s;
}
}
Rope cachedIdentifier3 = (StringCachingGuards.privatizeRope(arguments1Value_));
if ((RubyGuards.isRubyString(arguments1Value_)) && (StringCachingGuards.ropesEqual(arguments1Value_, cachedIdentifier3))) {
if (!root.excludeWriteCached_) {
String identifierString3 = (cachedIdentifier3.toString());
Node writeNode3 = (WriteNode.createWriteNode());
BranchProfile exceptionProfile3 = (BranchProfile.create());
SpecializationNode s = WriteCachedNode_.create(root, cachedIdentifier3, identifierString3, writeNode3, exceptionProfile3);
if (countSame(s) < (root.getCacheLimit())) {
return s;
}
}
}
if ((RubyGuards.isRubyString(arguments1Value_))) {
Node writeNode4 = (WriteNode.createWriteNode());
BranchProfile exceptionProfile4 = (BranchProfile.create());
root.excludeWriteCached_ = true;
return WriteUncachedNode_.create(root, writeNode4, exceptionProfile4);
}
}
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(WriteNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(WriteNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return uninitialized(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(WriteNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(WriteNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(WriteNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value, arguments1Value, arguments2Value));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(WriteNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "write(VirtualFrame, TruffleObject, Object, Object, Node, BranchProfile)", value = WriteNode.class)
private static final class Write0Node_ extends BaseNode_ {
@Child private Node writeNode;
private final BranchProfile exceptionProfile;
Write0Node_(WriteNodeGen root, Node writeNode, BranchProfile exceptionProfile) {
super(root, 1);
this.writeNode = writeNode;
this.exceptionProfile = exceptionProfile;
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof TruffleObject) {
TruffleObject arguments0Value_ = (TruffleObject) arguments0Value;
if ((!(RubyGuards.isRubySymbol(arguments1Value))) && (!(RubyGuards.isRubyString(arguments1Value)))) {
return root.write(frameValue, arguments0Value_, arguments1Value, arguments2Value, this.writeNode, this.exceptionProfile);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(WriteNodeGen root, Node writeNode, BranchProfile exceptionProfile) {
return new Write0Node_(root, writeNode, exceptionProfile);
}
}
@GeneratedBy(methodName = "write(VirtualFrame, TruffleObject, DynamicObject, Object, DynamicObject, String, Node, BranchProfile)", value = WriteNode.class)
private static final class Write1Node_ extends BaseNode_ {
private final DynamicObject cachedIdentifier;
private final String identifierString;
@Child private Node writeNode;
private final BranchProfile exceptionProfile;
Write1Node_(WriteNodeGen root, DynamicObject cachedIdentifier, String identifierString, Node writeNode, BranchProfile exceptionProfile) {
super(root, 2);
this.cachedIdentifier = cachedIdentifier;
this.identifierString = identifierString;
this.writeNode = writeNode;
this.exceptionProfile = exceptionProfile;
}
@Override
public boolean isIdentical(SpecializationNode other, Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof TruffleObject && arguments1Value instanceof DynamicObject) {
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
if ((arguments1Value_ == this.cachedIdentifier)) {
assert (RubyGuards.isRubySymbol(this.cachedIdentifier));
return true;
}
}
return false;
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof TruffleObject && arguments1Value instanceof DynamicObject) {
TruffleObject arguments0Value_ = (TruffleObject) arguments0Value;
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
if ((arguments1Value_ == this.cachedIdentifier)) {
assert (RubyGuards.isRubySymbol(this.cachedIdentifier));
return root.write(frameValue, arguments0Value_, arguments1Value_, arguments2Value, this.cachedIdentifier, this.identifierString, this.writeNode, this.exceptionProfile);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(WriteNodeGen root, DynamicObject cachedIdentifier, String identifierString, Node writeNode, BranchProfile exceptionProfile) {
return new Write1Node_(root, cachedIdentifier, identifierString, writeNode, exceptionProfile);
}
}
@GeneratedBy(methodName = "writeCached(VirtualFrame, TruffleObject, DynamicObject, Object, Rope, String, Node, BranchProfile)", value = WriteNode.class)
private static final class WriteCachedNode_ extends BaseNode_ {
private final Rope cachedIdentifier;
private final String identifierString;
@Child private Node writeNode;
private final BranchProfile exceptionProfile;
WriteCachedNode_(WriteNodeGen root, Rope cachedIdentifier, String identifierString, Node writeNode, BranchProfile exceptionProfile) {
super(root, 3);
this.cachedIdentifier = cachedIdentifier;
this.identifierString = identifierString;
this.writeNode = writeNode;
this.exceptionProfile = exceptionProfile;
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (newNode.getClass() == WriteUncachedNode_.class) {
removeSame("Contained by writeUncached(VirtualFrame, TruffleObject, DynamicObject, Object, Node, BranchProfile)");
}
return super.merge(newNode, frameValue, arguments0Value, arguments1Value, arguments2Value);
}
@Override
public boolean isIdentical(SpecializationNode other, Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof TruffleObject && arguments1Value instanceof DynamicObject) {
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
if ((RubyGuards.isRubyString(arguments1Value_)) && (StringCachingGuards.ropesEqual(arguments1Value_, this.cachedIdentifier))) {
return true;
}
}
return false;
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof TruffleObject && arguments1Value instanceof DynamicObject) {
TruffleObject arguments0Value_ = (TruffleObject) arguments0Value;
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
if ((RubyGuards.isRubyString(arguments1Value_)) && (StringCachingGuards.ropesEqual(arguments1Value_, this.cachedIdentifier))) {
return root.writeCached(frameValue, arguments0Value_, arguments1Value_, arguments2Value, this.cachedIdentifier, this.identifierString, this.writeNode, this.exceptionProfile);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(WriteNodeGen root, Rope cachedIdentifier, String identifierString, Node writeNode, BranchProfile exceptionProfile) {
return new WriteCachedNode_(root, cachedIdentifier, identifierString, writeNode, exceptionProfile);
}
}
@GeneratedBy(methodName = "writeUncached(VirtualFrame, TruffleObject, DynamicObject, Object, Node, BranchProfile)", value = WriteNode.class)
private static final class WriteUncachedNode_ extends BaseNode_ {
@Child private Node writeNode;
private final BranchProfile exceptionProfile;
WriteUncachedNode_(WriteNodeGen root, Node writeNode, BranchProfile exceptionProfile) {
super(root, 4);
this.writeNode = writeNode;
this.exceptionProfile = exceptionProfile;
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof TruffleObject && arguments1Value instanceof DynamicObject) {
TruffleObject arguments0Value_ = (TruffleObject) arguments0Value;
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
if ((RubyGuards.isRubyString(arguments1Value_))) {
return root.writeUncached(frameValue, arguments0Value_, arguments1Value_, arguments2Value, this.writeNode, this.exceptionProfile);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(WriteNodeGen root, Node writeNode, BranchProfile exceptionProfile) {
return new WriteUncachedNode_(root, writeNode, exceptionProfile);
}
}
}
}
@GeneratedBy(KeysNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class KeysNodeFactory implements NodeFactory {
private static KeysNodeFactory keysNodeFactoryInstance;
private KeysNodeFactory() {
}
@Override
public Class getNodeClass() {
return KeysNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public KeysNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (keysNodeFactoryInstance == null) {
keysNodeFactoryInstance = new KeysNodeFactory();
}
return keysNodeFactoryInstance;
}
public static KeysNode create(RubyNode[] arguments) {
return new KeysNodeGen(arguments);
}
@GeneratedBy(KeysNode.class)
public static final class KeysNodeGen extends KeysNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private BaseNode_ specialization_;
private KeysNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(KeysNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected KeysNodeGen root;
BaseNode_(KeysNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (KeysNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.execute_((VirtualFrame) frameValue, arguments0Value);
}
public abstract Object execute_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
return execute_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
if (arguments0Value instanceof TruffleObject) {
Node keysNode1 = (root.createKeysNode());
SnippetNode snippetNode1 = (new SnippetNode());
BranchProfile exceptionProfile1 = (BranchProfile.create());
return SizeNode_.create(root, keysNode1, snippetNode1, exceptionProfile1);
}
return null;
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(KeysNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(KeysNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
return uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(KeysNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(methodName = "size(VirtualFrame, TruffleObject, Node, SnippetNode, BranchProfile)", value = KeysNode.class)
private static final class SizeNode_ extends BaseNode_ {
@Child private Node keysNode;
@Child private SnippetNode snippetNode;
private final BranchProfile exceptionProfile;
SizeNode_(KeysNodeGen root, Node keysNode, SnippetNode snippetNode, BranchProfile exceptionProfile) {
super(root, 1);
this.keysNode = keysNode;
this.snippetNode = snippetNode;
this.exceptionProfile = exceptionProfile;
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
if (arguments0Value instanceof TruffleObject) {
TruffleObject arguments0Value_ = (TruffleObject) arguments0Value;
return root.size(frameValue, arguments0Value_, this.keysNode, this.snippetNode, this.exceptionProfile);
}
return getNext().execute_(frameValue, arguments0Value);
}
static BaseNode_ create(KeysNodeGen root, Node keysNode, SnippetNode snippetNode, BranchProfile exceptionProfile) {
return new SizeNode_(root, keysNode, snippetNode, exceptionProfile);
}
}
}
}
@GeneratedBy(ExportNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class ExportNodeFactory implements NodeFactory {
private static ExportNodeFactory exportNodeFactoryInstance;
private ExportNodeFactory() {
}
@Override
public Class getNodeClass() {
return ExportNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public ExportNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (exportNodeFactoryInstance == null) {
exportNodeFactoryInstance = new ExportNodeFactory();
}
return exportNodeFactoryInstance;
}
public static ExportNode create(RubyNode[] arguments) {
return new ExportNodeGen(arguments);
}
@GeneratedBy(ExportNode.class)
public static final class ExportNodeGen extends ExportNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@CompilationFinal private boolean seenUnsupported0;
private ExportNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = arguments1_.execute(frameValue);
throw unsupported(ex.getResult(), arguments1Value);
}
TruffleObject arguments1Value_;
try {
arguments1Value_ = expectTruffleObject(arguments1_.execute(frameValue));
} catch (UnexpectedResultException ex) {
throw unsupported(arguments0Value_, ex.getResult());
}
if ((RubyGuards.isRubyString(arguments0Value_) || RubyGuards.isRubySymbol(arguments0Value_))) {
return this.export(arguments0Value_, arguments1Value_);
}
throw unsupported(arguments0Value_, arguments1Value_);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
private UnsupportedSpecializationException unsupported(Object arguments0Value, Object arguments1Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_, arguments1_}, arguments0Value, arguments1Value);
}
private static TruffleObject expectTruffleObject(Object value) throws UnexpectedResultException {
if (value instanceof TruffleObject) {
return (TruffleObject) value;
}
throw new UnexpectedResultException(value);
}
}
}
@GeneratedBy(ImportNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class ImportNodeFactory implements NodeFactory {
private static ImportNodeFactory importNodeFactoryInstance;
private ImportNodeFactory() {
}
@Override
public Class getNodeClass() {
return ImportNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode.class));
}
@Override
public ImportNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode)) {
return create((RubyNode) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (importNodeFactoryInstance == null) {
importNodeFactoryInstance = new ImportNodeFactory();
}
return importNodeFactoryInstance;
}
public static ImportNode create(RubyNode name) {
return new ImportNodeGen(name);
}
@GeneratedBy(ImportNode.class)
public static final class ImportNodeGen extends ImportNode implements SpecializedNode {
@Child private RubyNode name_;
@Child private BaseNode_ specialization_;
private ImportNodeGen(RubyNode name) {
this.name_ = coercetNameToString(name);
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(ImportNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected ImportNodeGen root;
BaseNode_(ImportNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (ImportNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.name_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object nameValue) {
return this.execute_((VirtualFrame) frameValue, nameValue);
}
public abstract Object execute_(VirtualFrame frameValue, Object nameValue);
public Object execute(VirtualFrame frameValue) {
Object nameValue_ = root.name_.execute(frameValue);
return execute_(frameValue, nameValue_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object nameValue) {
if (nameValue instanceof String) {
BranchProfile errorProfile1 = (BranchProfile.create());
return ImportObjectNode_.create(root, errorProfile1);
}
return null;
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(ImportNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(ImportNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute_(VirtualFrame frameValue, Object nameValue) {
return uninitialized(frameValue, nameValue);
}
static BaseNode_ create(ImportNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(methodName = "importObject(String, BranchProfile)", value = ImportNode.class)
private static final class ImportObjectNode_ extends BaseNode_ {
private final BranchProfile errorProfile;
ImportObjectNode_(ImportNodeGen root, BranchProfile errorProfile) {
super(root, 1);
this.errorProfile = errorProfile;
}
@Override
public Object execute_(VirtualFrame frameValue, Object nameValue) {
if (nameValue instanceof String) {
String nameValue_ = (String) nameValue;
return root.importObject(nameValue_, this.errorProfile);
}
return getNext().execute_(frameValue, nameValue);
}
static BaseNode_ create(ImportNodeGen root, BranchProfile errorProfile) {
return new ImportObjectNode_(root, errorProfile);
}
}
}
}
@GeneratedBy(MimeTypeSupportedNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class MimeTypeSupportedNodeFactory implements NodeFactory {
private static MimeTypeSupportedNodeFactory mimeTypeSupportedNodeFactoryInstance;
private MimeTypeSupportedNodeFactory() {
}
@Override
public Class getNodeClass() {
return MimeTypeSupportedNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public MimeTypeSupportedNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (mimeTypeSupportedNodeFactoryInstance == null) {
mimeTypeSupportedNodeFactoryInstance = new MimeTypeSupportedNodeFactory();
}
return mimeTypeSupportedNodeFactoryInstance;
}
public static MimeTypeSupportedNode create(RubyNode[] arguments) {
return new MimeTypeSupportedNodeGen(arguments);
}
@GeneratedBy(MimeTypeSupportedNode.class)
public static final class MimeTypeSupportedNodeGen extends MimeTypeSupportedNode {
@Child private RubyNode arguments0_;
@CompilationFinal private boolean seenUnsupported0;
private MimeTypeSupportedNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeBoolean(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
executeBoolean(frameValue);
return;
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(ex.getResult());
}
if ((RubyGuards.isRubyString(arguments0Value_))) {
return this.isMimeTypeSupported(arguments0Value_);
}
throw unsupported(arguments0Value_);
}
private UnsupportedSpecializationException unsupported(Object arguments0Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_}, arguments0Value);
}
}
}
@GeneratedBy(EvalNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class EvalNodeFactory implements NodeFactory {
private static EvalNodeFactory evalNodeFactoryInstance;
private EvalNodeFactory() {
}
@Override
public Class getNodeClass() {
return EvalNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public EvalNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (evalNodeFactoryInstance == null) {
evalNodeFactoryInstance = new EvalNodeFactory();
}
return evalNodeFactoryInstance;
}
public static EvalNode create(RubyNode[] arguments) {
return new EvalNodeGen(arguments);
}
@GeneratedBy(EvalNode.class)
public static final class EvalNodeGen extends EvalNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@CompilationFinal private boolean excludeEvalCached_;
@Child private BaseNode_ specialization_;
private EvalNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(EvalNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected EvalNodeGen root;
BaseNode_(EvalNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (EvalNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_, root.arguments1_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value, Object arguments1Value) {
return this.execute_((VirtualFrame) frameValue, arguments0Value, arguments1Value);
}
public abstract Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = root.arguments1_.execute(frameValue);
return execute_(frameValue, arguments0Value_, arguments1Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject && arguments1Value instanceof DynamicObject) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
Rope cachedMimeType1 = (StringCachingGuards.privatizeRope(arguments0Value_));
Rope cachedSource1 = (StringCachingGuards.privatizeRope(arguments1Value_));
if ((RubyGuards.isRubyString(arguments0Value_)) && (RubyGuards.isRubyString(arguments1Value_)) && (StringCachingGuards.ropesEqual(arguments0Value_, cachedMimeType1)) && (StringCachingGuards.ropesEqual(arguments1Value_, cachedSource1))) {
if (!root.excludeEvalCached_) {
DirectCallNode callNode1 = (DirectCallNode.create(root.parse(arguments0Value_, arguments1Value_)));
SpecializationNode s = EvalCachedNode_.create(root, cachedMimeType1, cachedSource1, callNode1);
if (countSame(s) < (root.getCacheLimit())) {
return s;
}
}
}
if ((RubyGuards.isRubyString(arguments0Value_)) && (RubyGuards.isRubyString(arguments1Value_))) {
IndirectCallNode callNode2 = (IndirectCallNode.create());
root.excludeEvalCached_ = true;
return EvalUncachedNode_.create(root, callNode2);
}
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(EvalNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(EvalNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
return uninitialized(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(EvalNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(EvalNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(EvalNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value, arguments1Value));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
return getNext().execute_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(EvalNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "evalCached(VirtualFrame, DynamicObject, DynamicObject, Rope, Rope, DirectCallNode)", value = EvalNode.class)
private static final class EvalCachedNode_ extends BaseNode_ {
private final Rope cachedMimeType;
private final Rope cachedSource;
@Child private DirectCallNode callNode;
EvalCachedNode_(EvalNodeGen root, Rope cachedMimeType, Rope cachedSource, DirectCallNode callNode) {
super(root, 1);
this.cachedMimeType = cachedMimeType;
this.cachedSource = cachedSource;
this.callNode = callNode;
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value) {
if (newNode.getClass() == EvalUncachedNode_.class) {
removeSame("Contained by evalUncached(VirtualFrame, DynamicObject, DynamicObject, IndirectCallNode)");
}
return super.merge(newNode, frameValue, arguments0Value, arguments1Value);
}
@Override
public boolean isIdentical(SpecializationNode other, Frame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject && arguments1Value instanceof DynamicObject) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
if ((RubyGuards.isRubyString(arguments0Value_)) && (RubyGuards.isRubyString(arguments1Value_)) && (StringCachingGuards.ropesEqual(arguments0Value_, this.cachedMimeType)) && (StringCachingGuards.ropesEqual(arguments1Value_, this.cachedSource))) {
return true;
}
}
return false;
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject && arguments1Value instanceof DynamicObject) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
if ((RubyGuards.isRubyString(arguments0Value_)) && (RubyGuards.isRubyString(arguments1Value_)) && (StringCachingGuards.ropesEqual(arguments0Value_, this.cachedMimeType)) && (StringCachingGuards.ropesEqual(arguments1Value_, this.cachedSource))) {
return root.evalCached(frameValue, arguments0Value_, arguments1Value_, this.cachedMimeType, this.cachedSource, this.callNode);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(EvalNodeGen root, Rope cachedMimeType, Rope cachedSource, DirectCallNode callNode) {
return new EvalCachedNode_(root, cachedMimeType, cachedSource, callNode);
}
}
@GeneratedBy(methodName = "evalUncached(VirtualFrame, DynamicObject, DynamicObject, IndirectCallNode)", value = EvalNode.class)
private static final class EvalUncachedNode_ extends BaseNode_ {
@Child private IndirectCallNode callNode;
EvalUncachedNode_(EvalNodeGen root, IndirectCallNode callNode) {
super(root, 2);
this.callNode = callNode;
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject && arguments1Value instanceof DynamicObject) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
if ((RubyGuards.isRubyString(arguments0Value_)) && (RubyGuards.isRubyString(arguments1Value_))) {
return root.evalUncached(frameValue, arguments0Value_, arguments1Value_, this.callNode);
}
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(EvalNodeGen root, IndirectCallNode callNode) {
return new EvalUncachedNode_(root, callNode);
}
}
}
}
@GeneratedBy(InteropToJavaStringNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class InteropToJavaStringNodeFactory implements NodeFactory {
private static InteropToJavaStringNodeFactory interopToJavaStringNodeFactoryInstance;
private InteropToJavaStringNodeFactory() {
}
@Override
public Class getNodeClass() {
return InteropToJavaStringNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public InteropToJavaStringNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (interopToJavaStringNodeFactoryInstance == null) {
interopToJavaStringNodeFactoryInstance = new InteropToJavaStringNodeFactory();
}
return interopToJavaStringNodeFactoryInstance;
}
public static InteropToJavaStringNode create(RubyNode[] arguments) {
return new InteropToJavaStringNodeGen(arguments);
}
@GeneratedBy(InteropToJavaStringNode.class)
public static final class InteropToJavaStringNodeGen extends InteropToJavaStringNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private BaseNode_ specialization_;
private InteropToJavaStringNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(InteropToJavaStringNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected InteropToJavaStringNodeGen root;
BaseNode_(InteropToJavaStringNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (InteropToJavaStringNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.execute_((VirtualFrame) frameValue, arguments0Value);
}
public abstract Object execute_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
return execute_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
NameToJavaStringNode toJavaStringNode1 = (NameToJavaStringNode.create());
return ToJavaStringNode_.create(root, toJavaStringNode1);
}
}
@GeneratedBy(InteropToJavaStringNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(InteropToJavaStringNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
return uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(InteropToJavaStringNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(methodName = "toJavaString(VirtualFrame, Object, NameToJavaStringNode)", value = InteropToJavaStringNode.class)
private static final class ToJavaStringNode_ extends BaseNode_ {
@Child private NameToJavaStringNode toJavaStringNode;
ToJavaStringNode_(InteropToJavaStringNodeGen root, NameToJavaStringNode toJavaStringNode) {
super(root, 1);
this.toJavaStringNode = toJavaStringNode;
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
return root.toJavaString(frameValue, arguments0Value, this.toJavaStringNode);
}
static BaseNode_ create(InteropToJavaStringNodeGen root, NameToJavaStringNode toJavaStringNode) {
return new ToJavaStringNode_(root, toJavaStringNode);
}
}
}
}
@GeneratedBy(InteropFromJavaStringNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class InteropFromJavaStringNodeFactory implements NodeFactory {
private static InteropFromJavaStringNodeFactory interopFromJavaStringNodeFactoryInstance;
private InteropFromJavaStringNodeFactory() {
}
@Override
public Class getNodeClass() {
return InteropFromJavaStringNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public InteropFromJavaStringNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (interopFromJavaStringNodeFactoryInstance == null) {
interopFromJavaStringNodeFactoryInstance = new InteropFromJavaStringNodeFactory();
}
return interopFromJavaStringNodeFactoryInstance;
}
public static InteropFromJavaStringNode create(RubyNode[] arguments) {
return new InteropFromJavaStringNodeGen(arguments);
}
@GeneratedBy(InteropFromJavaStringNode.class)
public static final class InteropFromJavaStringNodeGen extends InteropFromJavaStringNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private BaseNode_ specialization_;
private InteropFromJavaStringNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(InteropFromJavaStringNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected InteropFromJavaStringNodeGen root;
BaseNode_(InteropFromJavaStringNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (InteropFromJavaStringNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value) {
return this.execute_((VirtualFrame) frameValue, arguments0Value);
}
public abstract Object execute_(VirtualFrame frameValue, Object arguments0Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
return execute_(frameValue, arguments0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value) {
ForeignToRubyNode foreignToRubyNode1 = (root.createForeignToRubyNode());
return FromJavaStringNode_.create(root, foreignToRubyNode1);
}
}
@GeneratedBy(InteropFromJavaStringNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(InteropFromJavaStringNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
return uninitialized(frameValue, arguments0Value);
}
static BaseNode_ create(InteropFromJavaStringNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(methodName = "fromJavaString(VirtualFrame, Object, ForeignToRubyNode)", value = InteropFromJavaStringNode.class)
private static final class FromJavaStringNode_ extends BaseNode_ {
@Child private ForeignToRubyNode foreignToRubyNode;
FromJavaStringNode_(InteropFromJavaStringNodeGen root, ForeignToRubyNode foreignToRubyNode) {
super(root, 1);
this.foreignToRubyNode = foreignToRubyNode;
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value) {
return root.fromJavaString(frameValue, arguments0Value, this.foreignToRubyNode);
}
static BaseNode_ create(InteropFromJavaStringNodeGen root, ForeignToRubyNode foreignToRubyNode) {
return new FromJavaStringNode_(root, foreignToRubyNode);
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy