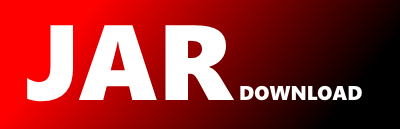
org.jruby.truffle.language.constants.LookupConstantNodeGen Maven / Gradle / Ivy
The newest version!
// CheckStyle: start generated
package org.jruby.truffle.language.constants;
import com.oracle.truffle.api.Assumption;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.internal.SpecializationNode;
import com.oracle.truffle.api.dsl.internal.SpecializedNode;
import com.oracle.truffle.api.dsl.internal.SuppressFBWarnings;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.InvalidAssumptionException;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.object.DynamicObject;
import com.oracle.truffle.api.profiles.ConditionProfile;
import org.jruby.truffle.language.RubyConstant;
import org.jruby.truffle.language.RubyGuards;
import org.jruby.truffle.language.RubyNode;
@GeneratedBy(LookupConstantNode.class)
@SuppressFBWarnings("SA_LOCAL_SELF_COMPARISON")
public final class LookupConstantNodeGen extends LookupConstantNode implements SpecializedNode {
@Child private RubyNode module_;
@Child private RubyNode name_;
@Child private BaseNode_ specialization_;
private LookupConstantNodeGen(boolean ignoreVisibility, boolean lookInObject, RubyNode module, RubyNode name) {
super(ignoreVisibility, lookInObject);
this.module_ = module;
this.name_ = name;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public RubyConstant executeLookupConstant(VirtualFrame frameValue, Object moduleValue, String nameValue) {
return specialization_.executeRubyConstant(frameValue, moduleValue, nameValue);
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
public static LookupConstantNode create(boolean ignoreVisibility, boolean lookInObject, RubyNode module, RubyNode name) {
return new LookupConstantNodeGen(ignoreVisibility, lookInObject, module, name);
}
@GeneratedBy(LookupConstantNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected LookupConstantNodeGen root;
BaseNode_(LookupConstantNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (LookupConstantNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.module_, root.name_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object moduleValue, Object nameValue) {
return this.executeRubyConstant_((VirtualFrame) frameValue, moduleValue, nameValue);
}
public abstract RubyConstant executeRubyConstant_(VirtualFrame frameValue, Object moduleValue, Object nameValue);
public RubyConstant executeRubyConstant(VirtualFrame frameValue, Object moduleValue, String nameValue) {
return executeRubyConstant_(frameValue, moduleValue, nameValue);
}
public Object execute(VirtualFrame frameValue) {
Object moduleValue_ = root.module_.execute(frameValue);
Object nameValue_ = root.name_.execute(frameValue);
return executeRubyConstant_(frameValue, moduleValue_, nameValue_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object moduleValue, Object nameValue) {
if (nameValue instanceof String) {
String nameValue_ = (String) nameValue;
if (moduleValue instanceof DynamicObject) {
DynamicObject moduleValue_ = (DynamicObject) moduleValue;
DynamicObject cachedModule1 = (moduleValue_);
String cachedName1 = (nameValue_);
ConditionProfile sameNameProfile1 = (ConditionProfile.createBinaryProfile());
if ((moduleValue_ == cachedModule1) && (RubyGuards.isRubyModule(cachedModule1)) && (root.guardName(nameValue_, cachedName1, sameNameProfile1))) {
RubyConstant constant1 = (root.doLookup(cachedModule1, cachedName1));
boolean isVisible1 = (root.isVisible(cachedModule1, constant1));
Assumption assumption0_1 = (root.getUnmodifiedAssumption(cachedModule1));
if (isValid(assumption0_1)) {
SpecializationNode s = LookupConstantNode_.create(root, cachedModule1, cachedName1, constant1, isVisible1, sameNameProfile1, assumption0_1);
if (countSame(s) < (root.getCacheLimit())) {
return s;
}
}
}
if ((RubyGuards.isRubyModule(moduleValue_))) {
ConditionProfile isVisibleProfile2 = (ConditionProfile.createBinaryProfile());
ConditionProfile isDeprecatedProfile2 = (ConditionProfile.createBinaryProfile());
return LookupConstantUncachedNode_.create(root, isVisibleProfile2, isDeprecatedProfile2);
}
}
if ((!(RubyGuards.isRubyModule(moduleValue)))) {
return LookupNotModuleNode_.create(root);
}
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(LookupConstantNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(LookupConstantNodeGen root) {
super(root, 2147483647);
}
@Override
public RubyConstant executeRubyConstant_(VirtualFrame frameValue, Object moduleValue, Object nameValue) {
return (RubyConstant) uninitialized(frameValue, moduleValue, nameValue);
}
static BaseNode_ create(LookupConstantNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(LookupConstantNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(LookupConstantNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object moduleValue, Object nameValue) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, moduleValue, nameValue));
}
@Override
public RubyConstant executeRubyConstant_(VirtualFrame frameValue, Object moduleValue, Object nameValue) {
return getNext().executeRubyConstant_(frameValue, moduleValue, nameValue);
}
static BaseNode_ create(LookupConstantNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "lookupConstant(VirtualFrame, DynamicObject, String, DynamicObject, String, RubyConstant, boolean, ConditionProfile)", value = LookupConstantNode.class)
private static final class LookupConstantNode_ extends BaseNode_ {
private final DynamicObject cachedModule;
private final String cachedName;
private final RubyConstant constant;
private final boolean isVisible;
private final ConditionProfile sameNameProfile;
@CompilationFinal private final Assumption assumption0_;
LookupConstantNode_(LookupConstantNodeGen root, DynamicObject cachedModule, String cachedName, RubyConstant constant, boolean isVisible, ConditionProfile sameNameProfile, Assumption assumption0_) {
super(root, 1);
this.cachedModule = cachedModule;
this.cachedName = cachedName;
this.constant = constant;
this.isVisible = isVisible;
this.sameNameProfile = sameNameProfile;
this.assumption0_ = assumption0_;
}
@Override
public boolean isIdentical(SpecializationNode other, Frame frameValue, Object moduleValue, Object nameValue) {
if (moduleValue instanceof DynamicObject && nameValue instanceof String) {
DynamicObject moduleValue_ = (DynamicObject) moduleValue;
String nameValue_ = (String) nameValue;
if ((moduleValue_ == this.cachedModule) && (root.guardName(nameValue_, this.cachedName, this.sameNameProfile))) {
assert (RubyGuards.isRubyModule(this.cachedModule));
return true;
}
}
return false;
}
@Override
public RubyConstant executeRubyConstant(VirtualFrame frameValue, Object moduleValue, String nameValue) {
try {
check(this.assumption0_);
} catch (InvalidAssumptionException ae) {
return (RubyConstant) removeThis("Assumption [assumption0] invalidated", frameValue, moduleValue, nameValue);
}
if (moduleValue instanceof DynamicObject) {
DynamicObject moduleValue_ = (DynamicObject) moduleValue;
if ((moduleValue_ == this.cachedModule) && (root.guardName(nameValue, this.cachedName, this.sameNameProfile))) {
assert (RubyGuards.isRubyModule(this.cachedModule));
return root.lookupConstant(frameValue, moduleValue_, nameValue, this.cachedModule, this.cachedName, this.constant, this.isVisible, this.sameNameProfile);
}
}
return getNext().executeRubyConstant(frameValue, moduleValue, nameValue);
}
@Override
public RubyConstant executeRubyConstant_(VirtualFrame frameValue, Object moduleValue, Object nameValue) {
try {
check(this.assumption0_);
} catch (InvalidAssumptionException ae) {
return (RubyConstant) removeThis("Assumption [assumption0] invalidated", frameValue, moduleValue, nameValue);
}
if (moduleValue instanceof DynamicObject && nameValue instanceof String) {
DynamicObject moduleValue_ = (DynamicObject) moduleValue;
String nameValue_ = (String) nameValue;
if ((moduleValue_ == this.cachedModule) && (root.guardName(nameValue_, this.cachedName, this.sameNameProfile))) {
assert (RubyGuards.isRubyModule(this.cachedModule));
return root.lookupConstant(frameValue, moduleValue_, nameValue_, this.cachedModule, this.cachedName, this.constant, this.isVisible, this.sameNameProfile);
}
}
return getNext().executeRubyConstant_(frameValue, moduleValue, nameValue);
}
static BaseNode_ create(LookupConstantNodeGen root, DynamicObject cachedModule, String cachedName, RubyConstant constant, boolean isVisible, ConditionProfile sameNameProfile, Assumption assumption0_) {
return new LookupConstantNode_(root, cachedModule, cachedName, constant, isVisible, sameNameProfile, assumption0_);
}
}
@GeneratedBy(methodName = "lookupConstantUncached(VirtualFrame, DynamicObject, String, ConditionProfile, ConditionProfile)", value = LookupConstantNode.class)
private static final class LookupConstantUncachedNode_ extends BaseNode_ {
private final ConditionProfile isVisibleProfile;
private final ConditionProfile isDeprecatedProfile;
LookupConstantUncachedNode_(LookupConstantNodeGen root, ConditionProfile isVisibleProfile, ConditionProfile isDeprecatedProfile) {
super(root, 2);
this.isVisibleProfile = isVisibleProfile;
this.isDeprecatedProfile = isDeprecatedProfile;
}
@Override
public RubyConstant executeRubyConstant(VirtualFrame frameValue, Object moduleValue, String nameValue) {
if (moduleValue instanceof DynamicObject) {
DynamicObject moduleValue_ = (DynamicObject) moduleValue;
if ((RubyGuards.isRubyModule(moduleValue_))) {
return root.lookupConstantUncached(frameValue, moduleValue_, nameValue, this.isVisibleProfile, this.isDeprecatedProfile);
}
}
return getNext().executeRubyConstant(frameValue, moduleValue, nameValue);
}
@Override
public RubyConstant executeRubyConstant_(VirtualFrame frameValue, Object moduleValue, Object nameValue) {
if (moduleValue instanceof DynamicObject && nameValue instanceof String) {
DynamicObject moduleValue_ = (DynamicObject) moduleValue;
String nameValue_ = (String) nameValue;
if ((RubyGuards.isRubyModule(moduleValue_))) {
return root.lookupConstantUncached(frameValue, moduleValue_, nameValue_, this.isVisibleProfile, this.isDeprecatedProfile);
}
}
return getNext().executeRubyConstant_(frameValue, moduleValue, nameValue);
}
static BaseNode_ create(LookupConstantNodeGen root, ConditionProfile isVisibleProfile, ConditionProfile isDeprecatedProfile) {
return new LookupConstantUncachedNode_(root, isVisibleProfile, isDeprecatedProfile);
}
}
@GeneratedBy(methodName = "lookupNotModule(Object, String)", value = LookupConstantNode.class)
private static final class LookupNotModuleNode_ extends BaseNode_ {
LookupNotModuleNode_(LookupConstantNodeGen root) {
super(root, 3);
}
@Override
public RubyConstant executeRubyConstant(VirtualFrame frameValue, Object moduleValue, String nameValue) {
if ((!(RubyGuards.isRubyModule(moduleValue)))) {
return root.lookupNotModule(moduleValue, nameValue);
}
return getNext().executeRubyConstant(frameValue, moduleValue, nameValue);
}
@Override
public RubyConstant executeRubyConstant_(VirtualFrame frameValue, Object moduleValue, Object nameValue) {
if (nameValue instanceof String) {
String nameValue_ = (String) nameValue;
if ((!(RubyGuards.isRubyModule(moduleValue)))) {
return root.lookupNotModule(moduleValue, nameValue_);
}
}
return getNext().executeRubyConstant_(frameValue, moduleValue, nameValue);
}
static BaseNode_ create(LookupConstantNodeGen root) {
return new LookupNotModuleNode_(root);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy