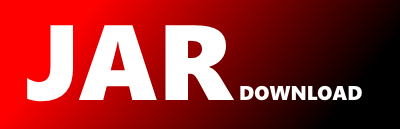
org.jruby.truffle.language.globals.ReadGlobalVariableNodeGen Maven / Gradle / Ivy
The newest version!
// CheckStyle: start generated
package org.jruby.truffle.language.globals;
import com.oracle.truffle.api.Assumption;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.internal.SpecializationNode;
import com.oracle.truffle.api.dsl.internal.SpecializedNode;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.InvalidAssumptionException;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
@GeneratedBy(ReadGlobalVariableNode.class)
public final class ReadGlobalVariableNodeGen extends ReadGlobalVariableNode implements SpecializedNode {
@Child private BaseNode_ specialization_;
private ReadGlobalVariableNodeGen(String name) {
super(name);
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
public static ReadGlobalVariableNode create(String name) {
return new ReadGlobalVariableNodeGen(name);
}
@GeneratedBy(ReadGlobalVariableNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected ReadGlobalVariableNodeGen root;
BaseNode_(ReadGlobalVariableNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (ReadGlobalVariableNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {};
}
@Override
public final Object acceptAndExecute(Frame frameValue) {
return this.execute((VirtualFrame) frameValue);
}
public abstract Object execute(VirtualFrame frameValue);
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue) {
GlobalVariableStorage storage1 = (root.getStorage());
Object value1 = (storage1.getValue());
Assumption assumption0_1 = (storage1.getUnchangedAssumption());
if (isValid(assumption0_1)) {
return ReadConstantNode_.create(root, storage1, value1, assumption0_1);
}
GlobalVariableStorage storage2 = (root.getStorage());
return ReadNode_.create(root, storage2);
}
}
@GeneratedBy(ReadGlobalVariableNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(ReadGlobalVariableNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute(VirtualFrame frameValue) {
return uninitialized(frameValue);
}
static BaseNode_ create(ReadGlobalVariableNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(methodName = "readConstant(GlobalVariableStorage, Object)", value = ReadGlobalVariableNode.class)
private static final class ReadConstantNode_ extends BaseNode_ {
private final GlobalVariableStorage storage;
private final Object value;
@CompilationFinal private final Assumption assumption0_;
ReadConstantNode_(ReadGlobalVariableNodeGen root, GlobalVariableStorage storage, Object value, Assumption assumption0_) {
super(root, 1);
this.storage = storage;
this.value = value;
this.assumption0_ = assumption0_;
}
@Override
public Object execute(VirtualFrame frameValue) {
try {
check(this.assumption0_);
} catch (InvalidAssumptionException ae) {
return removeThis("Assumption [assumption0] invalidated", frameValue);
}
return root.readConstant(this.storage, this.value);
}
static BaseNode_ create(ReadGlobalVariableNodeGen root, GlobalVariableStorage storage, Object value, Assumption assumption0_) {
return new ReadConstantNode_(root, storage, value, assumption0_);
}
}
@GeneratedBy(methodName = "read(GlobalVariableStorage)", value = ReadGlobalVariableNode.class)
private static final class ReadNode_ extends BaseNode_ {
private final GlobalVariableStorage storage;
ReadNode_(ReadGlobalVariableNodeGen root, GlobalVariableStorage storage) {
super(root, 2);
this.storage = storage;
}
@Override
public Object execute(VirtualFrame frameValue) {
return root.read(this.storage);
}
static BaseNode_ create(ReadGlobalVariableNodeGen root, GlobalVariableStorage storage) {
return new ReadNode_(root, storage);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy