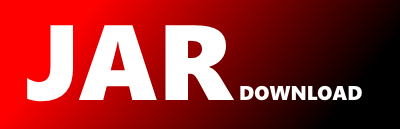
org.jruby.truffle.language.globals.WriteGlobalVariableNodeGen Maven / Gradle / Ivy
The newest version!
// CheckStyle: start generated
package org.jruby.truffle.language.globals;
import com.oracle.truffle.api.Assumption;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.internal.SpecializationNode;
import com.oracle.truffle.api.dsl.internal.SpecializedNode;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.InvalidAssumptionException;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import org.jruby.truffle.language.RubyNode;
@GeneratedBy(WriteGlobalVariableNode.class)
public final class WriteGlobalVariableNodeGen extends WriteGlobalVariableNode implements SpecializedNode {
@Child private RubyNode value_;
@CompilationFinal private boolean excludeWriteAssumeConstant_;
@Child private BaseNode_ specialization_;
private WriteGlobalVariableNodeGen(String name, RubyNode value) {
super(name);
this.value_ = value;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
public static WriteGlobalVariableNode create(String name, RubyNode value) {
return new WriteGlobalVariableNodeGen(name, value);
}
@GeneratedBy(WriteGlobalVariableNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected WriteGlobalVariableNodeGen root;
BaseNode_(WriteGlobalVariableNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (WriteGlobalVariableNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.value_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object valueValue) {
return this.execute_((VirtualFrame) frameValue, valueValue);
}
public abstract Object execute_(VirtualFrame frameValue, Object valueValue);
public Object execute(VirtualFrame frameValue) {
Object valueValue_ = root.value_.execute(frameValue);
return execute_(frameValue, valueValue_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object valueValue) {
GlobalVariableStorage storage1 = (root.getStorage());
Object previousValue1 = (storage1.getValue());
if ((root.referenceEqualNode.executeReferenceEqual(valueValue, previousValue1))) {
Assumption assumption0_1 = (storage1.getUnchangedAssumption());
if (isValid(assumption0_1)) {
SpecializationNode s = WriteTryToKeepConstantNode_.create(root, storage1, previousValue1, assumption0_1);
if (countSame(s) < (3)) {
return s;
}
}
}
GlobalVariableStorage storage2 = (root.getStorage());
if ((storage2.isAssumeConstant())) {
if (!root.excludeWriteAssumeConstant_) {
Assumption assumption0_2 = (storage2.getUnchangedAssumption());
if (isValid(assumption0_2)) {
return WriteAssumeConstantNode_.create(root, storage2, assumption0_2);
}
}
}
GlobalVariableStorage storage3 = (root.getStorage());
if ((root.workaround()) && (!(storage3.isAssumeConstant()))) {
root.excludeWriteAssumeConstant_ = true;
return WriteNode_.create(root, storage3);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(WriteGlobalVariableNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(WriteGlobalVariableNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute_(VirtualFrame frameValue, Object valueValue) {
return uninitialized(frameValue, valueValue);
}
static BaseNode_ create(WriteGlobalVariableNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(WriteGlobalVariableNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(WriteGlobalVariableNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object valueValue) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, valueValue));
}
@Override
public Object execute_(VirtualFrame frameValue, Object valueValue) {
return getNext().execute_(frameValue, valueValue);
}
static BaseNode_ create(WriteGlobalVariableNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "writeTryToKeepConstant(Object, GlobalVariableStorage, Object)", value = WriteGlobalVariableNode.class)
private static final class WriteTryToKeepConstantNode_ extends BaseNode_ {
private final GlobalVariableStorage storage;
private final Object previousValue;
@CompilationFinal private final Assumption assumption0_;
WriteTryToKeepConstantNode_(WriteGlobalVariableNodeGen root, GlobalVariableStorage storage, Object previousValue, Assumption assumption0_) {
super(root, 1);
this.storage = storage;
this.previousValue = previousValue;
this.assumption0_ = assumption0_;
}
@Override
public boolean isIdentical(SpecializationNode other, Frame frameValue, Object valueValue) {
if ((root.referenceEqualNode.executeReferenceEqual(valueValue, this.previousValue))) {
return true;
}
return false;
}
@Override
public Object execute_(VirtualFrame frameValue, Object valueValue) {
try {
check(this.assumption0_);
} catch (InvalidAssumptionException ae) {
return removeThis("Assumption [assumption0] invalidated", frameValue, valueValue);
}
if ((root.referenceEqualNode.executeReferenceEqual(valueValue, this.previousValue))) {
return root.writeTryToKeepConstant(valueValue, this.storage, this.previousValue);
}
return getNext().execute_(frameValue, valueValue);
}
static BaseNode_ create(WriteGlobalVariableNodeGen root, GlobalVariableStorage storage, Object previousValue, Assumption assumption0_) {
return new WriteTryToKeepConstantNode_(root, storage, previousValue, assumption0_);
}
}
@GeneratedBy(methodName = "writeAssumeConstant(Object, GlobalVariableStorage)", value = WriteGlobalVariableNode.class)
private static final class WriteAssumeConstantNode_ extends BaseNode_ {
private final GlobalVariableStorage storage;
@CompilationFinal private final Assumption assumption0_;
WriteAssumeConstantNode_(WriteGlobalVariableNodeGen root, GlobalVariableStorage storage, Assumption assumption0_) {
super(root, 2);
this.storage = storage;
this.assumption0_ = assumption0_;
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object valueValue) {
if (newNode.getClass() == WriteNode_.class) {
removeSame("Contained by write(Object, GlobalVariableStorage)");
}
return super.merge(newNode, frameValue, valueValue);
}
@Override
public Object execute_(VirtualFrame frameValue, Object valueValue) {
try {
check(this.assumption0_);
} catch (InvalidAssumptionException ae) {
return removeThis("Assumption [assumption0] invalidated", frameValue, valueValue);
}
assert (this.storage.isAssumeConstant());
return root.writeAssumeConstant(valueValue, this.storage);
}
static BaseNode_ create(WriteGlobalVariableNodeGen root, GlobalVariableStorage storage, Assumption assumption0_) {
return new WriteAssumeConstantNode_(root, storage, assumption0_);
}
}
@GeneratedBy(methodName = "write(Object, GlobalVariableStorage)", value = WriteGlobalVariableNode.class)
private static final class WriteNode_ extends BaseNode_ {
private final GlobalVariableStorage storage;
WriteNode_(WriteGlobalVariableNodeGen root, GlobalVariableStorage storage) {
super(root, 3);
this.storage = storage;
}
@Override
public Object execute_(VirtualFrame frameValue, Object valueValue) {
assert (root.workaround());
assert (!(this.storage.isAssumeConstant()));
return root.write(valueValue, this.storage);
}
static BaseNode_ create(WriteGlobalVariableNodeGen root, GlobalVariableStorage storage) {
return new WriteNode_(root, storage);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy