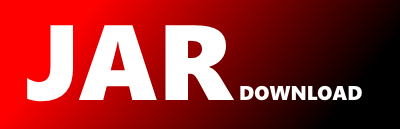
org.jruby.truffle.language.locals.ReadFrameSlotNodeGen Maven / Gradle / Ivy
The newest version!
// CheckStyle: start generated
package org.jruby.truffle.language.locals;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.internal.SpecializationNode;
import com.oracle.truffle.api.dsl.internal.SpecializedNode;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.FrameSlot;
import com.oracle.truffle.api.frame.FrameSlotTypeException;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
@GeneratedBy(ReadFrameSlotNode.class)
public final class ReadFrameSlotNodeGen extends ReadFrameSlotNode implements SpecializedNode {
@CompilationFinal private boolean excludeReadBoolean_;
@CompilationFinal private boolean excludeReadInt_;
@CompilationFinal private boolean excludeReadLong_;
@CompilationFinal private boolean excludeReadDouble_;
@CompilationFinal private boolean excludeReadObject_;
@Child private BaseNode_ specialization_;
private ReadFrameSlotNodeGen(FrameSlot slot) {
super(slot);
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object executeRead(Frame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
public static ReadFrameSlotNode create(FrameSlot slot) {
return new ReadFrameSlotNodeGen(slot);
}
@GeneratedBy(ReadFrameSlotNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected ReadFrameSlotNodeGen root;
BaseNode_(ReadFrameSlotNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (ReadFrameSlotNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {};
}
@Override
public final Object acceptAndExecute(Frame frameValue) {
return this.execute(frameValue);
}
public abstract Object execute(Frame frameValue);
@Override
protected final SpecializationNode createNext(Frame frameValue) {
if (!root.excludeReadBoolean_) {
return ReadBooleanNode_.create(root);
}
if (!root.excludeReadInt_) {
return ReadIntNode_.create(root);
}
if (!root.excludeReadLong_) {
return ReadLongNode_.create(root);
}
if (!root.excludeReadDouble_) {
return ReadDoubleNode_.create(root);
}
if (!root.excludeReadObject_) {
return ReadObjectNode_.create(root);
}
root.excludeReadBoolean_ = true;
root.excludeReadInt_ = true;
root.excludeReadLong_ = true;
root.excludeReadDouble_ = true;
root.excludeReadObject_ = true;
return ReadAnyNode_.create(root);
}
}
@GeneratedBy(ReadFrameSlotNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(ReadFrameSlotNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute(Frame frameValue) {
return uninitialized(frameValue);
}
static BaseNode_ create(ReadFrameSlotNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(methodName = "readBoolean(Frame)", value = ReadFrameSlotNode.class)
private static final class ReadBooleanNode_ extends BaseNode_ {
ReadBooleanNode_(ReadFrameSlotNodeGen root) {
super(root, 1);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue) {
if (newNode.getClass() == ReadAnyNode_.class) {
removeSame("Contained by readAny(Frame)");
}
return super.merge(newNode, frameValue);
}
@Override
public Object execute(Frame frameValue) {
try {
return root.readBoolean(frameValue);
} catch (FrameSlotTypeException ex) {
root.excludeReadBoolean_ = true;
return remove("threw rewrite exception", frameValue);
}
}
static BaseNode_ create(ReadFrameSlotNodeGen root) {
return new ReadBooleanNode_(root);
}
}
@GeneratedBy(methodName = "readInt(Frame)", value = ReadFrameSlotNode.class)
private static final class ReadIntNode_ extends BaseNode_ {
ReadIntNode_(ReadFrameSlotNodeGen root) {
super(root, 2);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue) {
if (newNode.getClass() == ReadAnyNode_.class) {
removeSame("Contained by readAny(Frame)");
}
return super.merge(newNode, frameValue);
}
@Override
public Object execute(Frame frameValue) {
try {
return root.readInt(frameValue);
} catch (FrameSlotTypeException ex) {
root.excludeReadInt_ = true;
return remove("threw rewrite exception", frameValue);
}
}
static BaseNode_ create(ReadFrameSlotNodeGen root) {
return new ReadIntNode_(root);
}
}
@GeneratedBy(methodName = "readLong(Frame)", value = ReadFrameSlotNode.class)
private static final class ReadLongNode_ extends BaseNode_ {
ReadLongNode_(ReadFrameSlotNodeGen root) {
super(root, 3);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue) {
if (newNode.getClass() == ReadAnyNode_.class) {
removeSame("Contained by readAny(Frame)");
}
return super.merge(newNode, frameValue);
}
@Override
public Object execute(Frame frameValue) {
try {
return root.readLong(frameValue);
} catch (FrameSlotTypeException ex) {
root.excludeReadLong_ = true;
return remove("threw rewrite exception", frameValue);
}
}
static BaseNode_ create(ReadFrameSlotNodeGen root) {
return new ReadLongNode_(root);
}
}
@GeneratedBy(methodName = "readDouble(Frame)", value = ReadFrameSlotNode.class)
private static final class ReadDoubleNode_ extends BaseNode_ {
ReadDoubleNode_(ReadFrameSlotNodeGen root) {
super(root, 4);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue) {
if (newNode.getClass() == ReadAnyNode_.class) {
removeSame("Contained by readAny(Frame)");
}
return super.merge(newNode, frameValue);
}
@Override
public Object execute(Frame frameValue) {
try {
return root.readDouble(frameValue);
} catch (FrameSlotTypeException ex) {
root.excludeReadDouble_ = true;
return remove("threw rewrite exception", frameValue);
}
}
static BaseNode_ create(ReadFrameSlotNodeGen root) {
return new ReadDoubleNode_(root);
}
}
@GeneratedBy(methodName = "readObject(Frame)", value = ReadFrameSlotNode.class)
private static final class ReadObjectNode_ extends BaseNode_ {
ReadObjectNode_(ReadFrameSlotNodeGen root) {
super(root, 5);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue) {
if (newNode.getClass() == ReadAnyNode_.class) {
removeSame("Contained by readAny(Frame)");
}
return super.merge(newNode, frameValue);
}
@Override
public Object execute(Frame frameValue) {
try {
return root.readObject(frameValue);
} catch (FrameSlotTypeException ex) {
root.excludeReadObject_ = true;
return remove("threw rewrite exception", frameValue);
}
}
static BaseNode_ create(ReadFrameSlotNodeGen root) {
return new ReadObjectNode_(root);
}
}
@GeneratedBy(methodName = "readAny(Frame)", value = ReadFrameSlotNode.class)
private static final class ReadAnyNode_ extends BaseNode_ {
ReadAnyNode_(ReadFrameSlotNodeGen root) {
super(root, 6);
}
@Override
public Object execute(Frame frameValue) {
return root.readAny(frameValue);
}
static BaseNode_ create(ReadFrameSlotNodeGen root) {
return new ReadAnyNode_(root);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy