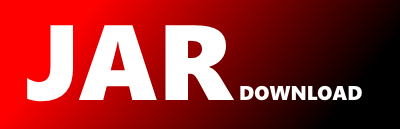
org.jruby.truffle.language.locals.WriteFrameSlotNodeGen Maven / Gradle / Ivy
The newest version!
// CheckStyle: start generated
package org.jruby.truffle.language.locals;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.internal.SpecializationNode;
import com.oracle.truffle.api.dsl.internal.SpecializedNode;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.FrameSlot;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
@GeneratedBy(WriteFrameSlotNode.class)
public final class WriteFrameSlotNodeGen extends WriteFrameSlotNode implements SpecializedNode {
@CompilationFinal private boolean excludeWriteBoolean_;
@CompilationFinal private boolean excludeWriteInteger_;
@CompilationFinal private boolean excludeWriteLong_;
@CompilationFinal private boolean excludeWriteDouble_;
@Child private BaseNode_ specialization_;
private WriteFrameSlotNodeGen(FrameSlot frameSlot) {
super(frameSlot);
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object executeWrite(Frame frameValue, Object arg0Value) {
return specialization_.execute(frameValue, arg0Value);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
public static WriteFrameSlotNode create(FrameSlot frameSlot) {
return new WriteFrameSlotNodeGen(frameSlot);
}
@GeneratedBy(WriteFrameSlotNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected WriteFrameSlotNodeGen root;
BaseNode_(WriteFrameSlotNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (WriteFrameSlotNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {null};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arg0Value) {
return this.execute(frameValue, arg0Value);
}
public abstract Object execute(Frame frameValue, Object arg0Value);
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arg0Value) {
if (arg0Value instanceof Boolean) {
if ((root.checkBooleanKind(frameValue))) {
if (!root.excludeWriteBoolean_) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return WriteBooleanNode_.create(root);
}
}
}
if (arg0Value instanceof Integer) {
if ((root.checkIntegerKind(frameValue))) {
if (!root.excludeWriteInteger_) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return WriteIntegerNode_.create(root);
}
}
}
if (arg0Value instanceof Long) {
if ((root.checkLongKind(frameValue))) {
if (!root.excludeWriteLong_) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return WriteLongNode_.create(root);
}
}
}
if (arg0Value instanceof Double) {
if ((root.checkDoubleKind(frameValue))) {
if (!root.excludeWriteDouble_) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return WriteDoubleNode_.create(root);
}
}
}
if ((root.checkObjectKind(frameValue))) {
CompilerDirectives.transferToInterpreterAndInvalidate();
root.excludeWriteBoolean_ = true;
root.excludeWriteInteger_ = true;
root.excludeWriteLong_ = true;
root.excludeWriteDouble_ = true;
return WriteObjectNode_.create(root);
}
return null;
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(WriteFrameSlotNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(WriteFrameSlotNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute(Frame frameValue, Object arg0Value) {
return uninitialized(frameValue, arg0Value);
}
static BaseNode_ create(WriteFrameSlotNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(methodName = "writeBoolean(Frame, boolean)", value = WriteFrameSlotNode.class)
private static final class WriteBooleanNode_ extends BaseNode_ {
WriteBooleanNode_(WriteFrameSlotNodeGen root) {
super(root, 1);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arg0Value) {
if (newNode.getClass() == WriteObjectNode_.class) {
removeSame("Contained by writeObject(Frame, Object)");
}
return super.merge(newNode, frameValue, arg0Value);
}
@Override
public Object execute(Frame frameValue, Object arg0Value) {
if (arg0Value instanceof Boolean) {
boolean arg0Value_ = (boolean) arg0Value;
if ((root.checkBooleanKind(frameValue))) {
return root.writeBoolean(frameValue, arg0Value_);
}
}
return getNext().execute(frameValue, arg0Value);
}
static BaseNode_ create(WriteFrameSlotNodeGen root) {
return new WriteBooleanNode_(root);
}
}
@GeneratedBy(methodName = "writeInteger(Frame, int)", value = WriteFrameSlotNode.class)
private static final class WriteIntegerNode_ extends BaseNode_ {
WriteIntegerNode_(WriteFrameSlotNodeGen root) {
super(root, 2);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arg0Value) {
if (newNode.getClass() == WriteObjectNode_.class) {
removeSame("Contained by writeObject(Frame, Object)");
}
return super.merge(newNode, frameValue, arg0Value);
}
@Override
public Object execute(Frame frameValue, Object arg0Value) {
if (arg0Value instanceof Integer) {
int arg0Value_ = (int) arg0Value;
if ((root.checkIntegerKind(frameValue))) {
return root.writeInteger(frameValue, arg0Value_);
}
}
return getNext().execute(frameValue, arg0Value);
}
static BaseNode_ create(WriteFrameSlotNodeGen root) {
return new WriteIntegerNode_(root);
}
}
@GeneratedBy(methodName = "writeLong(Frame, long)", value = WriteFrameSlotNode.class)
private static final class WriteLongNode_ extends BaseNode_ {
WriteLongNode_(WriteFrameSlotNodeGen root) {
super(root, 3);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arg0Value) {
if (newNode.getClass() == WriteObjectNode_.class) {
removeSame("Contained by writeObject(Frame, Object)");
}
return super.merge(newNode, frameValue, arg0Value);
}
@Override
public Object execute(Frame frameValue, Object arg0Value) {
if (arg0Value instanceof Long) {
long arg0Value_ = (long) arg0Value;
if ((root.checkLongKind(frameValue))) {
return root.writeLong(frameValue, arg0Value_);
}
}
return getNext().execute(frameValue, arg0Value);
}
static BaseNode_ create(WriteFrameSlotNodeGen root) {
return new WriteLongNode_(root);
}
}
@GeneratedBy(methodName = "writeDouble(Frame, double)", value = WriteFrameSlotNode.class)
private static final class WriteDoubleNode_ extends BaseNode_ {
WriteDoubleNode_(WriteFrameSlotNodeGen root) {
super(root, 4);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arg0Value) {
if (newNode.getClass() == WriteObjectNode_.class) {
removeSame("Contained by writeObject(Frame, Object)");
}
return super.merge(newNode, frameValue, arg0Value);
}
@Override
public Object execute(Frame frameValue, Object arg0Value) {
if (arg0Value instanceof Double) {
double arg0Value_ = (double) arg0Value;
if ((root.checkDoubleKind(frameValue))) {
return root.writeDouble(frameValue, arg0Value_);
}
}
return getNext().execute(frameValue, arg0Value);
}
static BaseNode_ create(WriteFrameSlotNodeGen root) {
return new WriteDoubleNode_(root);
}
}
@GeneratedBy(methodName = "writeObject(Frame, Object)", value = WriteFrameSlotNode.class)
private static final class WriteObjectNode_ extends BaseNode_ {
WriteObjectNode_(WriteFrameSlotNodeGen root) {
super(root, 5);
}
@Override
public Object execute(Frame frameValue, Object arg0Value) {
if ((root.checkObjectKind(frameValue))) {
return root.writeObject(frameValue, arg0Value);
}
return getNext().execute(frameValue, arg0Value);
}
static BaseNode_ create(WriteFrameSlotNodeGen root) {
return new WriteObjectNode_(root);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy