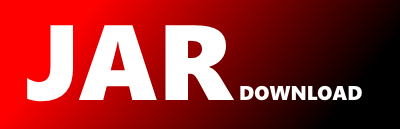
org.jruby.truffle.language.methods.CanBindMethodToModuleNodeGen Maven / Gradle / Ivy
The newest version!
// CheckStyle: start generated
package org.jruby.truffle.language.methods;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.internal.SpecializationNode;
import com.oracle.truffle.api.dsl.internal.SpecializedNode;
import com.oracle.truffle.api.dsl.internal.SuppressFBWarnings;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.nodes.UnexpectedResultException;
import com.oracle.truffle.api.object.DynamicObject;
import org.jruby.truffle.language.RubyGuards;
import org.jruby.truffle.language.RubyNode;
@GeneratedBy(CanBindMethodToModuleNode.class)
@SuppressFBWarnings("SA_LOCAL_SELF_COMPARISON")
public final class CanBindMethodToModuleNodeGen extends CanBindMethodToModuleNode implements SpecializedNode {
@Child private RubyNode method_;
@Child private RubyNode module_;
@Child private BaseNode_ specialization_;
private CanBindMethodToModuleNodeGen(RubyNode method, RubyNode module) {
this.method_ = method;
this.module_ = module;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public boolean executeCanBindMethodToModule(InternalMethod methodValue, DynamicObject moduleValue) {
return specialization_.executeBoolean1(methodValue, moduleValue);
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
return specialization_.executeBoolean0(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
private static InternalMethod expectInternalMethod(Object value) throws UnexpectedResultException {
if (value instanceof InternalMethod) {
return (InternalMethod) value;
}
throw new UnexpectedResultException(value);
}
public static CanBindMethodToModuleNode create(RubyNode method, RubyNode module) {
return new CanBindMethodToModuleNodeGen(method, module);
}
@GeneratedBy(CanBindMethodToModuleNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected CanBindMethodToModuleNodeGen root;
BaseNode_(CanBindMethodToModuleNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (CanBindMethodToModuleNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.method_, root.module_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object methodValue, Object moduleValue) {
return this.executeBoolean_(methodValue, moduleValue);
}
public abstract boolean executeBoolean_(Object methodValue, Object moduleValue);
public boolean executeBoolean1(InternalMethod methodValue, DynamicObject moduleValue) {
return executeBoolean_(methodValue, moduleValue);
}
public Object execute(VirtualFrame frameValue) {
Object methodValue_ = root.method_.execute(frameValue);
Object moduleValue_ = root.module_.execute(frameValue);
return executeBoolean_(methodValue_, moduleValue_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public boolean executeBoolean0(VirtualFrame frameValue) {
return (boolean) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object methodValue, Object moduleValue) {
if (methodValue instanceof InternalMethod && moduleValue instanceof DynamicObject) {
InternalMethod methodValue_ = (InternalMethod) methodValue;
DynamicObject moduleValue_ = (DynamicObject) moduleValue;
DynamicObject declaringModule1 = (methodValue_.getDeclaringModule());
DynamicObject cachedModule1 = (moduleValue_);
if ((RubyGuards.isRubyModule(moduleValue_)) && (methodValue_.getDeclaringModule() == declaringModule1) && (moduleValue_ == cachedModule1)) {
boolean canBindMethodTo1 = (root.canBindMethodTo(declaringModule1, cachedModule1));
SpecializationNode s = CanBindMethodToCachedNode_.create(root, declaringModule1, cachedModule1, canBindMethodTo1);
if (countSame(s) < (root.getCacheLimit())) {
return s;
}
}
if ((RubyGuards.isRubyModule(moduleValue_))) {
return CanBindMethodToUncachedNode_.create(root);
}
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(CanBindMethodToModuleNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(CanBindMethodToModuleNodeGen root) {
super(root, 2147483647);
}
@Override
public boolean executeBoolean_(Object methodValue, Object moduleValue) {
return (boolean) uninitialized(null, methodValue, moduleValue);
}
static BaseNode_ create(CanBindMethodToModuleNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(CanBindMethodToModuleNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(CanBindMethodToModuleNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object methodValue, Object moduleValue) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, methodValue, moduleValue));
}
@Override
public boolean executeBoolean0(VirtualFrame frameValue) {
Object methodValue_ = root.method_.execute(frameValue);
Object moduleValue_ = root.module_.execute(frameValue);
return getNext().executeBoolean_(methodValue_, moduleValue_);
}
@Override
public boolean executeBoolean1(InternalMethod methodValue, DynamicObject moduleValue) {
return getNext().executeBoolean1(methodValue, moduleValue);
}
@Override
public boolean executeBoolean_(Object methodValue, Object moduleValue) {
return getNext().executeBoolean_(methodValue, moduleValue);
}
static BaseNode_ create(CanBindMethodToModuleNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "canBindMethodToCached(InternalMethod, DynamicObject, DynamicObject, DynamicObject, boolean)", value = CanBindMethodToModuleNode.class)
private static final class CanBindMethodToCachedNode_ extends BaseNode_ {
private final DynamicObject declaringModule;
private final DynamicObject cachedModule;
private final boolean canBindMethodTo;
CanBindMethodToCachedNode_(CanBindMethodToModuleNodeGen root, DynamicObject declaringModule, DynamicObject cachedModule, boolean canBindMethodTo) {
super(root, 1);
this.declaringModule = declaringModule;
this.cachedModule = cachedModule;
this.canBindMethodTo = canBindMethodTo;
}
@Override
public boolean isIdentical(SpecializationNode other, Frame frameValue, Object methodValue, Object moduleValue) {
if (methodValue instanceof InternalMethod && moduleValue instanceof DynamicObject) {
InternalMethod methodValue_ = (InternalMethod) methodValue;
DynamicObject moduleValue_ = (DynamicObject) moduleValue;
if ((RubyGuards.isRubyModule(moduleValue_)) && (methodValue_.getDeclaringModule() == this.declaringModule) && (moduleValue_ == this.cachedModule)) {
return true;
}
}
return false;
}
@Override
public boolean executeBoolean0(VirtualFrame frameValue) {
InternalMethod methodValue_;
try {
methodValue_ = expectInternalMethod(root.method_.execute(frameValue));
} catch (UnexpectedResultException ex) {
Object moduleValue = root.module_.execute(frameValue);
return getNext().executeBoolean_(ex.getResult(), moduleValue);
}
DynamicObject moduleValue_;
try {
moduleValue_ = root.module_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
return getNext().executeBoolean_(methodValue_, ex.getResult());
}
if ((RubyGuards.isRubyModule(moduleValue_)) && (methodValue_.getDeclaringModule() == this.declaringModule) && (moduleValue_ == this.cachedModule)) {
return root.canBindMethodToCached(methodValue_, moduleValue_, this.declaringModule, this.cachedModule, this.canBindMethodTo);
}
return getNext().executeBoolean_(methodValue_, moduleValue_);
}
@Override
public boolean executeBoolean1(InternalMethod methodValue, DynamicObject moduleValue) {
if ((RubyGuards.isRubyModule(moduleValue)) && (methodValue.getDeclaringModule() == this.declaringModule) && (moduleValue == this.cachedModule)) {
return root.canBindMethodToCached(methodValue, moduleValue, this.declaringModule, this.cachedModule, this.canBindMethodTo);
}
return getNext().executeBoolean1(methodValue, moduleValue);
}
@Override
public boolean executeBoolean_(Object methodValue, Object moduleValue) {
if (methodValue instanceof InternalMethod && moduleValue instanceof DynamicObject) {
InternalMethod methodValue_ = (InternalMethod) methodValue;
DynamicObject moduleValue_ = (DynamicObject) moduleValue;
if ((RubyGuards.isRubyModule(moduleValue_)) && (methodValue_.getDeclaringModule() == this.declaringModule) && (moduleValue_ == this.cachedModule)) {
return root.canBindMethodToCached(methodValue_, moduleValue_, this.declaringModule, this.cachedModule, this.canBindMethodTo);
}
}
return getNext().executeBoolean_(methodValue, moduleValue);
}
static BaseNode_ create(CanBindMethodToModuleNodeGen root, DynamicObject declaringModule, DynamicObject cachedModule, boolean canBindMethodTo) {
return new CanBindMethodToCachedNode_(root, declaringModule, cachedModule, canBindMethodTo);
}
}
@GeneratedBy(methodName = "canBindMethodToUncached(InternalMethod, DynamicObject)", value = CanBindMethodToModuleNode.class)
private static final class CanBindMethodToUncachedNode_ extends BaseNode_ {
CanBindMethodToUncachedNode_(CanBindMethodToModuleNodeGen root) {
super(root, 2);
}
@Override
public boolean executeBoolean0(VirtualFrame frameValue) {
InternalMethod methodValue_;
try {
methodValue_ = expectInternalMethod(root.method_.execute(frameValue));
} catch (UnexpectedResultException ex) {
Object moduleValue = root.module_.execute(frameValue);
return getNext().executeBoolean_(ex.getResult(), moduleValue);
}
DynamicObject moduleValue_;
try {
moduleValue_ = root.module_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
return getNext().executeBoolean_(methodValue_, ex.getResult());
}
if ((RubyGuards.isRubyModule(moduleValue_))) {
return root.canBindMethodToUncached(methodValue_, moduleValue_);
}
return getNext().executeBoolean_(methodValue_, moduleValue_);
}
@Override
public boolean executeBoolean1(InternalMethod methodValue, DynamicObject moduleValue) {
if ((RubyGuards.isRubyModule(moduleValue))) {
return root.canBindMethodToUncached(methodValue, moduleValue);
}
return getNext().executeBoolean1(methodValue, moduleValue);
}
@Override
public boolean executeBoolean_(Object methodValue, Object moduleValue) {
if (methodValue instanceof InternalMethod && moduleValue instanceof DynamicObject) {
InternalMethod methodValue_ = (InternalMethod) methodValue;
DynamicObject moduleValue_ = (DynamicObject) moduleValue;
if ((RubyGuards.isRubyModule(moduleValue_))) {
return root.canBindMethodToUncached(methodValue_, moduleValue_);
}
}
return getNext().executeBoolean_(methodValue, moduleValue);
}
static BaseNode_ create(CanBindMethodToModuleNodeGen root) {
return new CanBindMethodToUncachedNode_(root);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy