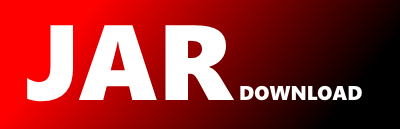
org.jruby.truffle.language.methods.LookupMethodNodeGen Maven / Gradle / Ivy
The newest version!
// CheckStyle: start generated
package org.jruby.truffle.language.methods;
import com.oracle.truffle.api.Assumption;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.internal.SpecializationNode;
import com.oracle.truffle.api.dsl.internal.SpecializedNode;
import com.oracle.truffle.api.dsl.internal.SuppressFBWarnings;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.InvalidAssumptionException;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.object.DynamicObject;
import org.jruby.truffle.language.RubyNode;
@GeneratedBy(LookupMethodNode.class)
@SuppressFBWarnings("SA_LOCAL_SELF_COMPARISON")
public final class LookupMethodNodeGen extends LookupMethodNode implements SpecializedNode {
@Child private RubyNode self_;
@Child private RubyNode name_;
@Child private BaseNode_ specialization_;
private LookupMethodNodeGen(boolean ignoreVisibility, boolean onlyLookupPublic, RubyNode self, RubyNode name) {
super(ignoreVisibility, onlyLookupPublic);
this.self_ = self;
this.name_ = name;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public InternalMethod executeLookupMethod(VirtualFrame frameValue, Object selfValue, String nameValue) {
return specialization_.executeInternalMethod(frameValue, selfValue, nameValue);
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
public static LookupMethodNode create(boolean ignoreVisibility, boolean onlyLookupPublic, RubyNode self, RubyNode name) {
return new LookupMethodNodeGen(ignoreVisibility, onlyLookupPublic, self, name);
}
@GeneratedBy(LookupMethodNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected LookupMethodNodeGen root;
BaseNode_(LookupMethodNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (LookupMethodNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.self_, root.name_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object selfValue, Object nameValue) {
return this.executeInternalMethod_((VirtualFrame) frameValue, selfValue, nameValue);
}
public abstract InternalMethod executeInternalMethod_(VirtualFrame frameValue, Object selfValue, Object nameValue);
public InternalMethod executeInternalMethod(VirtualFrame frameValue, Object selfValue, String nameValue) {
return executeInternalMethod_(frameValue, selfValue, nameValue);
}
public Object execute(VirtualFrame frameValue) {
Object selfValue_ = root.self_.execute(frameValue);
Object nameValue_ = root.name_.execute(frameValue);
return executeInternalMethod_(frameValue, selfValue_, nameValue_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object selfValue, Object nameValue) {
if (nameValue instanceof String) {
String nameValue_ = (String) nameValue;
DynamicObject selfMetaClass1 = (root.metaClass(selfValue));
String cachedName1 = (nameValue_);
if ((root.metaClass(selfValue) == selfMetaClass1) && (nameValue_ == cachedName1)) {
CompilerDirectives.transferToInterpreterAndInvalidate();
InternalMethod method1 = (root.doLookup((VirtualFrame) frameValue, selfValue, nameValue_));
Assumption assumption0_1 = (root.getUnmodifiedAssumption(selfMetaClass1));
if (isValid(assumption0_1)) {
SpecializationNode s = LookupMethodCachedNode_.create(root, selfMetaClass1, cachedName1, method1, assumption0_1);
if (countSame(s) < (root.getCacheLimit())) {
return s;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return LookupMethodUncachedNode_.create(root);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(LookupMethodNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(LookupMethodNodeGen root) {
super(root, 2147483647);
}
@Override
public InternalMethod executeInternalMethod_(VirtualFrame frameValue, Object selfValue, Object nameValue) {
return (InternalMethod) uninitialized(frameValue, selfValue, nameValue);
}
static BaseNode_ create(LookupMethodNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(LookupMethodNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(LookupMethodNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object selfValue, Object nameValue) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, selfValue, nameValue));
}
@Override
public InternalMethod executeInternalMethod_(VirtualFrame frameValue, Object selfValue, Object nameValue) {
return getNext().executeInternalMethod_(frameValue, selfValue, nameValue);
}
static BaseNode_ create(LookupMethodNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "lookupMethodCached(VirtualFrame, Object, String, DynamicObject, String, InternalMethod)", value = LookupMethodNode.class)
private static final class LookupMethodCachedNode_ extends BaseNode_ {
private final DynamicObject selfMetaClass;
private final String cachedName;
private final InternalMethod method;
@CompilationFinal private final Assumption assumption0_;
LookupMethodCachedNode_(LookupMethodNodeGen root, DynamicObject selfMetaClass, String cachedName, InternalMethod method, Assumption assumption0_) {
super(root, 1);
this.selfMetaClass = selfMetaClass;
this.cachedName = cachedName;
this.method = method;
this.assumption0_ = assumption0_;
}
@Override
public boolean isIdentical(SpecializationNode other, Frame frameValue, Object selfValue, Object nameValue) {
if (nameValue instanceof String) {
String nameValue_ = (String) nameValue;
if ((root.metaClass(selfValue) == this.selfMetaClass) && (nameValue_ == this.cachedName)) {
return true;
}
}
return false;
}
@Override
public InternalMethod executeInternalMethod(VirtualFrame frameValue, Object selfValue, String nameValue) {
try {
check(this.assumption0_);
} catch (InvalidAssumptionException ae) {
return (InternalMethod) removeThis("Assumption [assumption0] invalidated", frameValue, selfValue, nameValue);
}
if ((root.metaClass(selfValue) == this.selfMetaClass) && (nameValue == this.cachedName)) {
return root.lookupMethodCached(frameValue, selfValue, nameValue, this.selfMetaClass, this.cachedName, this.method);
}
return getNext().executeInternalMethod(frameValue, selfValue, nameValue);
}
@Override
public InternalMethod executeInternalMethod_(VirtualFrame frameValue, Object selfValue, Object nameValue) {
try {
check(this.assumption0_);
} catch (InvalidAssumptionException ae) {
return (InternalMethod) removeThis("Assumption [assumption0] invalidated", frameValue, selfValue, nameValue);
}
if (nameValue instanceof String) {
String nameValue_ = (String) nameValue;
if ((root.metaClass(selfValue) == this.selfMetaClass) && (nameValue_ == this.cachedName)) {
return root.lookupMethodCached(frameValue, selfValue, nameValue_, this.selfMetaClass, this.cachedName, this.method);
}
}
return getNext().executeInternalMethod_(frameValue, selfValue, nameValue);
}
static BaseNode_ create(LookupMethodNodeGen root, DynamicObject selfMetaClass, String cachedName, InternalMethod method, Assumption assumption0_) {
return new LookupMethodCachedNode_(root, selfMetaClass, cachedName, method, assumption0_);
}
}
@GeneratedBy(methodName = "lookupMethodUncached(VirtualFrame, Object, String)", value = LookupMethodNode.class)
private static final class LookupMethodUncachedNode_ extends BaseNode_ {
LookupMethodUncachedNode_(LookupMethodNodeGen root) {
super(root, 2);
}
@Override
public InternalMethod executeInternalMethod(VirtualFrame frameValue, Object selfValue, String nameValue) {
return root.lookupMethodUncached(frameValue, selfValue, nameValue);
}
@Override
public InternalMethod executeInternalMethod_(VirtualFrame frameValue, Object selfValue, Object nameValue) {
if (nameValue instanceof String) {
String nameValue_ = (String) nameValue;
return root.lookupMethodUncached(frameValue, selfValue, nameValue_);
}
return getNext().executeInternalMethod_(frameValue, selfValue, nameValue);
}
static BaseNode_ create(LookupMethodNodeGen root) {
return new LookupMethodUncachedNode_(root);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy