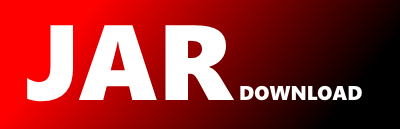
org.jruby.truffle.language.objects.AllocateObjectNodeGen Maven / Gradle / Ivy
The newest version!
// CheckStyle: start generated
package org.jruby.truffle.language.objects;
import com.oracle.truffle.api.Assumption;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.internal.SpecializationNode;
import com.oracle.truffle.api.dsl.internal.SpecializedNode;
import com.oracle.truffle.api.dsl.internal.SuppressFBWarnings;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.InvalidAssumptionException;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.object.DynamicObject;
import com.oracle.truffle.api.object.DynamicObjectFactory;
import org.jruby.truffle.language.RubyNode;
@GeneratedBy(AllocateObjectNode.class)
@SuppressFBWarnings("SA_LOCAL_SELF_COMPARISON")
public final class AllocateObjectNodeGen extends AllocateObjectNode implements SpecializedNode {
@Child private RubyNode classToAllocate_;
@Child private RubyNode values_;
@CompilationFinal private boolean excludeAllocateCached_;
@Child private BaseNode_ specialization_;
private AllocateObjectNodeGen(RubyNode classToAllocate, RubyNode values) {
this.classToAllocate_ = classToAllocate;
this.values_ = values;
this.specialization_ = UninitializedNode_.create(this);
}
private AllocateObjectNodeGen(boolean useCallerFrameForTracing, RubyNode classToAllocate, RubyNode values) {
super(useCallerFrameForTracing);
this.classToAllocate_ = classToAllocate;
this.values_ = values;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
protected DynamicObject executeAllocate(DynamicObject classToAllocateValue, Object[] valuesValue) {
return specialization_.executeDynamicObject1(classToAllocateValue, valuesValue);
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
public static AllocateObjectNode create(RubyNode classToAllocate, RubyNode values) {
return new AllocateObjectNodeGen(classToAllocate, values);
}
public static AllocateObjectNode create(boolean useCallerFrameForTracing, RubyNode classToAllocate, RubyNode values) {
return new AllocateObjectNodeGen(useCallerFrameForTracing, classToAllocate, values);
}
@GeneratedBy(AllocateObjectNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected AllocateObjectNodeGen root;
BaseNode_(AllocateObjectNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (AllocateObjectNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.classToAllocate_, root.values_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object classToAllocateValue, Object valuesValue) {
return this.executeDynamicObject_(classToAllocateValue, valuesValue);
}
public abstract DynamicObject executeDynamicObject_(Object classToAllocateValue, Object valuesValue);
public DynamicObject executeDynamicObject1(DynamicObject classToAllocateValue, Object[] valuesValue) {
return executeDynamicObject_(classToAllocateValue, valuesValue);
}
public Object execute(VirtualFrame frameValue) {
Object classToAllocateValue_ = root.classToAllocate_.execute(frameValue);
Object valuesValue_ = root.values_.execute(frameValue);
return executeDynamicObject_(classToAllocateValue_, valuesValue_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object classToAllocateValue, Object valuesValue) {
if (classToAllocateValue instanceof DynamicObject && valuesValue instanceof Object[]) {
DynamicObject classToAllocateValue_ = (DynamicObject) classToAllocateValue;
DynamicObject cachedClassToAllocate1 = (classToAllocateValue_);
boolean cachedIsSingleton1 = (root.isSingleton(classToAllocateValue_));
if ((cachedClassToAllocate1 == classToAllocateValue_) && (!(cachedIsSingleton1)) && (!(root.isTracing()))) {
if (!root.excludeAllocateCached_) {
DynamicObjectFactory factory1 = (root.getInstanceFactory(classToAllocateValue_));
Assumption assumption0_1 = (root.getTracingAssumption());
if (isValid(assumption0_1)) {
SpecializationNode s = AllocateCachedNode_.create(root, cachedClassToAllocate1, cachedIsSingleton1, factory1, assumption0_1);
if (countSame(s) < (root.getCacheLimit())) {
return s;
}
}
}
}
if ((!(root.isSingleton(classToAllocateValue_))) && (!(root.isTracing()))) {
Assumption assumption0_2 = (root.getTracingAssumption());
if (isValid(assumption0_2)) {
root.excludeAllocateCached_ = true;
return AllocateUncachedNode_.create(root, assumption0_2);
}
}
if ((!(root.isSingleton(classToAllocateValue_))) && (root.isTracing())) {
Assumption assumption0_3 = (root.getTracingAssumption());
if (isValid(assumption0_3)) {
return AllocateTracingNode_.create(root, assumption0_3);
}
}
if ((root.isSingleton(classToAllocateValue_))) {
return AllocateSingletonNode_.create(root);
}
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(AllocateObjectNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(AllocateObjectNodeGen root) {
super(root, 2147483647);
}
@Override
public DynamicObject executeDynamicObject_(Object classToAllocateValue, Object valuesValue) {
return (DynamicObject) uninitialized(null, classToAllocateValue, valuesValue);
}
static BaseNode_ create(AllocateObjectNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(AllocateObjectNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(AllocateObjectNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object classToAllocateValue, Object valuesValue) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, classToAllocateValue, valuesValue));
}
@Override
public DynamicObject executeDynamicObject_(Object classToAllocateValue, Object valuesValue) {
return getNext().executeDynamicObject_(classToAllocateValue, valuesValue);
}
static BaseNode_ create(AllocateObjectNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "allocateCached(DynamicObject, Object[], DynamicObject, boolean, DynamicObjectFactory)", value = AllocateObjectNode.class)
private static final class AllocateCachedNode_ extends BaseNode_ {
private final DynamicObject cachedClassToAllocate;
private final boolean cachedIsSingleton;
private final DynamicObjectFactory factory;
@CompilationFinal private final Assumption assumption0_;
AllocateCachedNode_(AllocateObjectNodeGen root, DynamicObject cachedClassToAllocate, boolean cachedIsSingleton, DynamicObjectFactory factory, Assumption assumption0_) {
super(root, 1);
this.cachedClassToAllocate = cachedClassToAllocate;
this.cachedIsSingleton = cachedIsSingleton;
this.factory = factory;
this.assumption0_ = assumption0_;
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object classToAllocateValue, Object valuesValue) {
if (newNode.getClass() == AllocateUncachedNode_.class) {
removeSame("Contained by allocateUncached(DynamicObject, Object[])");
}
return super.merge(newNode, frameValue, classToAllocateValue, valuesValue);
}
@Override
public boolean isIdentical(SpecializationNode other, Frame frameValue, Object classToAllocateValue, Object valuesValue) {
if (classToAllocateValue instanceof DynamicObject && valuesValue instanceof Object[]) {
DynamicObject classToAllocateValue_ = (DynamicObject) classToAllocateValue;
if ((this.cachedClassToAllocate == classToAllocateValue_)) {
assert (!(this.cachedIsSingleton));
assert (!(root.isTracing()));
return true;
}
}
return false;
}
@Override
public DynamicObject executeDynamicObject1(DynamicObject classToAllocateValue, Object[] valuesValue) {
try {
check(this.assumption0_);
} catch (InvalidAssumptionException ae) {
return (DynamicObject) removeThis("Assumption [assumption0] invalidated", null, classToAllocateValue, valuesValue);
}
if ((this.cachedClassToAllocate == classToAllocateValue)) {
assert (!(this.cachedIsSingleton));
assert (!(root.isTracing()));
return root.allocateCached(classToAllocateValue, valuesValue, this.cachedClassToAllocate, this.cachedIsSingleton, this.factory);
}
return getNext().executeDynamicObject1(classToAllocateValue, valuesValue);
}
@Override
public DynamicObject executeDynamicObject_(Object classToAllocateValue, Object valuesValue) {
try {
check(this.assumption0_);
} catch (InvalidAssumptionException ae) {
return (DynamicObject) removeThis("Assumption [assumption0] invalidated", null, classToAllocateValue, valuesValue);
}
if (classToAllocateValue instanceof DynamicObject && valuesValue instanceof Object[]) {
DynamicObject classToAllocateValue_ = (DynamicObject) classToAllocateValue;
Object[] valuesValue_ = (Object[]) valuesValue;
if ((this.cachedClassToAllocate == classToAllocateValue_)) {
assert (!(this.cachedIsSingleton));
assert (!(root.isTracing()));
return root.allocateCached(classToAllocateValue_, valuesValue_, this.cachedClassToAllocate, this.cachedIsSingleton, this.factory);
}
}
return getNext().executeDynamicObject_(classToAllocateValue, valuesValue);
}
static BaseNode_ create(AllocateObjectNodeGen root, DynamicObject cachedClassToAllocate, boolean cachedIsSingleton, DynamicObjectFactory factory, Assumption assumption0_) {
return new AllocateCachedNode_(root, cachedClassToAllocate, cachedIsSingleton, factory, assumption0_);
}
}
@GeneratedBy(methodName = "allocateUncached(DynamicObject, Object[])", value = AllocateObjectNode.class)
private static final class AllocateUncachedNode_ extends BaseNode_ {
@CompilationFinal private final Assumption assumption0_;
AllocateUncachedNode_(AllocateObjectNodeGen root, Assumption assumption0_) {
super(root, 2);
this.assumption0_ = assumption0_;
}
@Override
public DynamicObject executeDynamicObject1(DynamicObject classToAllocateValue, Object[] valuesValue) {
try {
check(this.assumption0_);
} catch (InvalidAssumptionException ae) {
return (DynamicObject) removeThis("Assumption [assumption0] invalidated", null, classToAllocateValue, valuesValue);
}
if ((!(root.isSingleton(classToAllocateValue)))) {
assert (!(root.isTracing()));
return root.allocateUncached(classToAllocateValue, valuesValue);
}
return getNext().executeDynamicObject1(classToAllocateValue, valuesValue);
}
@Override
public DynamicObject executeDynamicObject_(Object classToAllocateValue, Object valuesValue) {
try {
check(this.assumption0_);
} catch (InvalidAssumptionException ae) {
return (DynamicObject) removeThis("Assumption [assumption0] invalidated", null, classToAllocateValue, valuesValue);
}
if (classToAllocateValue instanceof DynamicObject && valuesValue instanceof Object[]) {
DynamicObject classToAllocateValue_ = (DynamicObject) classToAllocateValue;
Object[] valuesValue_ = (Object[]) valuesValue;
if ((!(root.isSingleton(classToAllocateValue_)))) {
assert (!(root.isTracing()));
return root.allocateUncached(classToAllocateValue_, valuesValue_);
}
}
return getNext().executeDynamicObject_(classToAllocateValue, valuesValue);
}
static BaseNode_ create(AllocateObjectNodeGen root, Assumption assumption0_) {
return new AllocateUncachedNode_(root, assumption0_);
}
}
@GeneratedBy(methodName = "allocateTracing(DynamicObject, Object[])", value = AllocateObjectNode.class)
private static final class AllocateTracingNode_ extends BaseNode_ {
@CompilationFinal private final Assumption assumption0_;
AllocateTracingNode_(AllocateObjectNodeGen root, Assumption assumption0_) {
super(root, 3);
this.assumption0_ = assumption0_;
}
@Override
public DynamicObject executeDynamicObject1(DynamicObject classToAllocateValue, Object[] valuesValue) {
try {
check(this.assumption0_);
} catch (InvalidAssumptionException ae) {
return (DynamicObject) removeThis("Assumption [assumption0] invalidated", null, classToAllocateValue, valuesValue);
}
if ((!(root.isSingleton(classToAllocateValue)))) {
assert (root.isTracing());
return root.allocateTracing(classToAllocateValue, valuesValue);
}
return getNext().executeDynamicObject1(classToAllocateValue, valuesValue);
}
@Override
public DynamicObject executeDynamicObject_(Object classToAllocateValue, Object valuesValue) {
try {
check(this.assumption0_);
} catch (InvalidAssumptionException ae) {
return (DynamicObject) removeThis("Assumption [assumption0] invalidated", null, classToAllocateValue, valuesValue);
}
if (classToAllocateValue instanceof DynamicObject && valuesValue instanceof Object[]) {
DynamicObject classToAllocateValue_ = (DynamicObject) classToAllocateValue;
Object[] valuesValue_ = (Object[]) valuesValue;
if ((!(root.isSingleton(classToAllocateValue_)))) {
assert (root.isTracing());
return root.allocateTracing(classToAllocateValue_, valuesValue_);
}
}
return getNext().executeDynamicObject_(classToAllocateValue, valuesValue);
}
static BaseNode_ create(AllocateObjectNodeGen root, Assumption assumption0_) {
return new AllocateTracingNode_(root, assumption0_);
}
}
@GeneratedBy(methodName = "allocateSingleton(DynamicObject, Object[])", value = AllocateObjectNode.class)
private static final class AllocateSingletonNode_ extends BaseNode_ {
AllocateSingletonNode_(AllocateObjectNodeGen root) {
super(root, 4);
}
@Override
public DynamicObject executeDynamicObject1(DynamicObject classToAllocateValue, Object[] valuesValue) {
if ((root.isSingleton(classToAllocateValue))) {
return root.allocateSingleton(classToAllocateValue, valuesValue);
}
return getNext().executeDynamicObject1(classToAllocateValue, valuesValue);
}
@Override
public DynamicObject executeDynamicObject_(Object classToAllocateValue, Object valuesValue) {
if (classToAllocateValue instanceof DynamicObject && valuesValue instanceof Object[]) {
DynamicObject classToAllocateValue_ = (DynamicObject) classToAllocateValue;
Object[] valuesValue_ = (Object[]) valuesValue;
if ((root.isSingleton(classToAllocateValue_))) {
return root.allocateSingleton(classToAllocateValue_, valuesValue_);
}
}
return getNext().executeDynamicObject_(classToAllocateValue, valuesValue);
}
static BaseNode_ create(AllocateObjectNodeGen root) {
return new AllocateSingletonNode_(root);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy