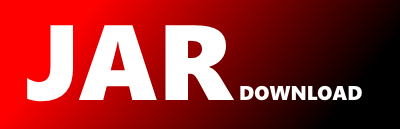
org.jruby.truffle.language.objects.AllocateObjectNodeWrapper Maven / Gradle / Ivy
The newest version!
// CheckStyle: start generated
package org.jruby.truffle.language.objects;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.instrumentation.InstrumentableFactory;
import com.oracle.truffle.api.instrumentation.ProbeNode;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.nodes.UnexpectedResultException;
import com.oracle.truffle.api.object.DynamicObject;
import org.jruby.truffle.language.NotProvided;
@GeneratedBy(AllocateObjectNode.class)
public final class AllocateObjectNodeWrapper implements InstrumentableFactory {
@Override
public WrapperNode createWrapper(AllocateObjectNode delegateNode, ProbeNode probeNode) {
return new AllocateObjectNodeWrapper0(delegateNode, probeNode);
}
@GeneratedBy(AllocateObjectNode.class)
private static final class AllocateObjectNodeWrapper0 extends AllocateObjectNode implements WrapperNode {
@Child private AllocateObjectNode delegateNode;
@Child private ProbeNode probeNode;
private AllocateObjectNodeWrapper0(AllocateObjectNode delegateNode, ProbeNode probeNode) {
this.delegateNode = delegateNode;
this.probeNode = probeNode;
}
@Override
public AllocateObjectNode getDelegateNode() {
return delegateNode;
}
@Override
public ProbeNode getProbeNode() {
return probeNode;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
@Override
public Object execute(VirtualFrame frame) {
try {
probeNode.onEnter(frame);
Object returnValue = delegateNode.execute(frame);
probeNode.onReturnValue(frame, returnValue);
return returnValue;
} catch (Throwable t) {
probeNode.onReturnExceptional(frame, t);
throw t;
}
}
@Override
public boolean executeBoolean(VirtualFrame frame) throws UnexpectedResultException {
try {
probeNode.onEnter(frame);
boolean returnValue = delegateNode.executeBoolean(frame);
probeNode.onReturnValue(frame, returnValue);
return returnValue;
} catch (Throwable t) {
probeNode.onReturnExceptional(frame, t);
throw t;
}
}
@Override
public double executeDouble(VirtualFrame frame) throws UnexpectedResultException {
try {
probeNode.onEnter(frame);
double returnValue = delegateNode.executeDouble(frame);
probeNode.onReturnValue(frame, returnValue);
return returnValue;
} catch (Throwable t) {
probeNode.onReturnExceptional(frame, t);
throw t;
}
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frame) throws UnexpectedResultException {
try {
probeNode.onEnter(frame);
DynamicObject returnValue = delegateNode.executeDynamicObject(frame);
probeNode.onReturnValue(frame, returnValue);
return returnValue;
} catch (Throwable t) {
probeNode.onReturnExceptional(frame, t);
throw t;
}
}
@Override
public int executeInteger(VirtualFrame frame) throws UnexpectedResultException {
try {
probeNode.onEnter(frame);
int returnValue = delegateNode.executeInteger(frame);
probeNode.onReturnValue(frame, returnValue);
return returnValue;
} catch (Throwable t) {
probeNode.onReturnExceptional(frame, t);
throw t;
}
}
@Override
public long executeLong(VirtualFrame frame) throws UnexpectedResultException {
try {
probeNode.onEnter(frame);
long returnValue = delegateNode.executeLong(frame);
probeNode.onReturnValue(frame, returnValue);
return returnValue;
} catch (Throwable t) {
probeNode.onReturnExceptional(frame, t);
throw t;
}
}
@Override
public NotProvided executeNotProvided(VirtualFrame frame) throws UnexpectedResultException {
try {
probeNode.onEnter(frame);
NotProvided returnValue = delegateNode.executeNotProvided(frame);
probeNode.onReturnValue(frame, returnValue);
return returnValue;
} catch (Throwable t) {
probeNode.onReturnExceptional(frame, t);
throw t;
}
}
@Override
public Object[] executeObjectArray(VirtualFrame frame) throws UnexpectedResultException {
try {
probeNode.onEnter(frame);
Object[] returnValue = delegateNode.executeObjectArray(frame);
probeNode.onReturnValue(frame, returnValue);
return returnValue;
} catch (Throwable t) {
probeNode.onReturnExceptional(frame, t);
throw t;
}
}
@Override
public void executeVoid(VirtualFrame frame) {
try {
probeNode.onEnter(frame);
delegateNode.executeVoid(frame);
probeNode.onReturnValue(frame, null);
} catch (Throwable t) {
probeNode.onReturnExceptional(frame, t);
throw t;
}
}
@Override
protected DynamicObject executeAllocate(DynamicObject classToAllocate, Object[] values) {
try {
probeNode.onEnter(null);
DynamicObject returnValue = delegateNode.executeAllocate(classToAllocate, values);
probeNode.onReturnValue(null, returnValue);
return returnValue;
} catch (Throwable t) {
probeNode.onReturnExceptional(null, t);
throw t;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy