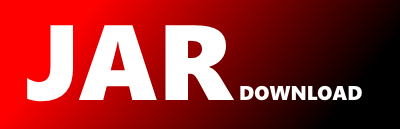
org.jruby.truffle.language.objects.IsANodeGen Maven / Gradle / Ivy
The newest version!
// CheckStyle: start generated
package org.jruby.truffle.language.objects;
import com.oracle.truffle.api.Assumption;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.internal.SpecializationNode;
import com.oracle.truffle.api.dsl.internal.SpecializedNode;
import com.oracle.truffle.api.dsl.internal.SuppressFBWarnings;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.InvalidAssumptionException;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.nodes.UnexpectedResultException;
import com.oracle.truffle.api.object.DynamicObject;
import org.jruby.truffle.language.RubyGuards;
import org.jruby.truffle.language.RubyNode;
@GeneratedBy(IsANode.class)
@SuppressFBWarnings("SA_LOCAL_SELF_COMPARISON")
public final class IsANodeGen extends IsANode implements SpecializedNode {
@Child private RubyNode instance_;
@Child private RubyNode module_;
@CompilationFinal private boolean excludeIsACached_;
@Child private BaseNode_ specialization_;
private IsANodeGen(RubyNode instance, RubyNode module) {
this.instance_ = instance;
this.module_ = module;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public boolean executeIsA(Object instanceValue, DynamicObject moduleValue) {
return specialization_.executeBoolean1(instanceValue, moduleValue);
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
return specialization_.executeBoolean0(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
public static IsANode create(RubyNode instance, RubyNode module) {
return new IsANodeGen(instance, module);
}
@GeneratedBy(IsANode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected IsANodeGen root;
BaseNode_(IsANodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (IsANodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.instance_, root.module_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object instanceValue, Object moduleValue) {
return this.executeBoolean_(instanceValue, moduleValue);
}
public abstract boolean executeBoolean_(Object instanceValue, Object moduleValue);
public boolean executeBoolean1(Object instanceValue, DynamicObject moduleValue) {
return executeBoolean_(instanceValue, moduleValue);
}
public Object execute(VirtualFrame frameValue) {
Object instanceValue_ = root.instance_.execute(frameValue);
Object moduleValue_ = root.module_.execute(frameValue);
return executeBoolean_(instanceValue_, moduleValue_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public boolean executeBoolean0(VirtualFrame frameValue) {
return (boolean) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object instanceValue, Object moduleValue) {
if (moduleValue instanceof DynamicObject) {
DynamicObject moduleValue_ = (DynamicObject) moduleValue;
DynamicObject cachedMetaClass1 = (root.getMetaClass(instanceValue));
DynamicObject cachedModule1 = (moduleValue_);
if ((RubyGuards.isRubyModule(cachedModule1)) && (root.getMetaClass(instanceValue) == cachedMetaClass1) && (moduleValue_ == cachedModule1)) {
if (!root.excludeIsACached_) {
boolean result1 = (root.isA(cachedMetaClass1, cachedModule1));
Assumption assumption0_1 = (root.getUnmodifiedAssumption(cachedModule1));
if (isValid(assumption0_1)) {
SpecializationNode s = IsACachedNode_.create(root, cachedMetaClass1, cachedModule1, result1, assumption0_1);
if (countSame(s) < (root.getCacheLimit())) {
return s;
}
}
}
}
if ((RubyGuards.isRubyModule(moduleValue_))) {
root.excludeIsACached_ = true;
return IsAUncachedNode_.create(root);
} else {
return IsATypeErrorNode_.create(root);
}
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(IsANode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(IsANodeGen root) {
super(root, 2147483647);
}
@Override
public boolean executeBoolean_(Object instanceValue, Object moduleValue) {
return (boolean) uninitialized(null, instanceValue, moduleValue);
}
static BaseNode_ create(IsANodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(IsANode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(IsANodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object instanceValue, Object moduleValue) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, instanceValue, moduleValue));
}
@Override
public boolean executeBoolean0(VirtualFrame frameValue) {
Object instanceValue_ = root.instance_.execute(frameValue);
Object moduleValue_ = root.module_.execute(frameValue);
return getNext().executeBoolean_(instanceValue_, moduleValue_);
}
@Override
public boolean executeBoolean1(Object instanceValue, DynamicObject moduleValue) {
return getNext().executeBoolean1(instanceValue, moduleValue);
}
@Override
public boolean executeBoolean_(Object instanceValue, Object moduleValue) {
return getNext().executeBoolean_(instanceValue, moduleValue);
}
static BaseNode_ create(IsANodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "isACached(Object, DynamicObject, DynamicObject, DynamicObject, boolean)", value = IsANode.class)
private static final class IsACachedNode_ extends BaseNode_ {
private final DynamicObject cachedMetaClass;
private final DynamicObject cachedModule;
private final boolean result;
@CompilationFinal private final Assumption assumption0_;
IsACachedNode_(IsANodeGen root, DynamicObject cachedMetaClass, DynamicObject cachedModule, boolean result, Assumption assumption0_) {
super(root, 1);
this.cachedMetaClass = cachedMetaClass;
this.cachedModule = cachedModule;
this.result = result;
this.assumption0_ = assumption0_;
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object instanceValue, Object moduleValue) {
if (newNode.getClass() == IsAUncachedNode_.class) {
removeSame("Contained by isAUncached(Object, DynamicObject)");
}
return super.merge(newNode, frameValue, instanceValue, moduleValue);
}
@Override
public boolean isIdentical(SpecializationNode other, Frame frameValue, Object instanceValue, Object moduleValue) {
if (moduleValue instanceof DynamicObject) {
DynamicObject moduleValue_ = (DynamicObject) moduleValue;
if ((root.getMetaClass(instanceValue) == this.cachedMetaClass) && (moduleValue_ == this.cachedModule)) {
assert (RubyGuards.isRubyModule(this.cachedModule));
return true;
}
}
return false;
}
@Override
public boolean executeBoolean0(VirtualFrame frameValue) {
Object instanceValue_ = root.instance_.execute(frameValue);
DynamicObject moduleValue_;
try {
moduleValue_ = root.module_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
return getNext().executeBoolean_(instanceValue_, ex.getResult());
}
try {
check(this.assumption0_);
} catch (InvalidAssumptionException ae) {
return (boolean) removeThis("Assumption [assumption0] invalidated", frameValue, instanceValue_, moduleValue_);
}
if ((root.getMetaClass(instanceValue_) == this.cachedMetaClass) && (moduleValue_ == this.cachedModule)) {
assert (RubyGuards.isRubyModule(this.cachedModule));
return root.isACached(instanceValue_, moduleValue_, this.cachedMetaClass, this.cachedModule, this.result);
}
return getNext().executeBoolean_(instanceValue_, moduleValue_);
}
@Override
public boolean executeBoolean1(Object instanceValue, DynamicObject moduleValue) {
try {
check(this.assumption0_);
} catch (InvalidAssumptionException ae) {
return (boolean) removeThis("Assumption [assumption0] invalidated", null, instanceValue, moduleValue);
}
if ((root.getMetaClass(instanceValue) == this.cachedMetaClass) && (moduleValue == this.cachedModule)) {
assert (RubyGuards.isRubyModule(this.cachedModule));
return root.isACached(instanceValue, moduleValue, this.cachedMetaClass, this.cachedModule, this.result);
}
return getNext().executeBoolean1(instanceValue, moduleValue);
}
@Override
public boolean executeBoolean_(Object instanceValue, Object moduleValue) {
try {
check(this.assumption0_);
} catch (InvalidAssumptionException ae) {
return (boolean) removeThis("Assumption [assumption0] invalidated", null, instanceValue, moduleValue);
}
if (moduleValue instanceof DynamicObject) {
DynamicObject moduleValue_ = (DynamicObject) moduleValue;
if ((root.getMetaClass(instanceValue) == this.cachedMetaClass) && (moduleValue_ == this.cachedModule)) {
assert (RubyGuards.isRubyModule(this.cachedModule));
return root.isACached(instanceValue, moduleValue_, this.cachedMetaClass, this.cachedModule, this.result);
}
}
return getNext().executeBoolean_(instanceValue, moduleValue);
}
static BaseNode_ create(IsANodeGen root, DynamicObject cachedMetaClass, DynamicObject cachedModule, boolean result, Assumption assumption0_) {
return new IsACachedNode_(root, cachedMetaClass, cachedModule, result, assumption0_);
}
}
@GeneratedBy(methodName = "isAUncached(Object, DynamicObject)", value = IsANode.class)
private static final class IsAUncachedNode_ extends BaseNode_ {
IsAUncachedNode_(IsANodeGen root) {
super(root, 2);
}
@Override
public boolean executeBoolean0(VirtualFrame frameValue) {
Object instanceValue_ = root.instance_.execute(frameValue);
DynamicObject moduleValue_;
try {
moduleValue_ = root.module_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
return getNext().executeBoolean_(instanceValue_, ex.getResult());
}
if ((RubyGuards.isRubyModule(moduleValue_))) {
return root.isAUncached(instanceValue_, moduleValue_);
}
return getNext().executeBoolean_(instanceValue_, moduleValue_);
}
@Override
public boolean executeBoolean1(Object instanceValue, DynamicObject moduleValue) {
if ((RubyGuards.isRubyModule(moduleValue))) {
return root.isAUncached(instanceValue, moduleValue);
}
return getNext().executeBoolean1(instanceValue, moduleValue);
}
@Override
public boolean executeBoolean_(Object instanceValue, Object moduleValue) {
if (moduleValue instanceof DynamicObject) {
DynamicObject moduleValue_ = (DynamicObject) moduleValue;
if ((RubyGuards.isRubyModule(moduleValue_))) {
return root.isAUncached(instanceValue, moduleValue_);
}
}
return getNext().executeBoolean_(instanceValue, moduleValue);
}
static BaseNode_ create(IsANodeGen root) {
return new IsAUncachedNode_(root);
}
}
@GeneratedBy(methodName = "isATypeError(Object, DynamicObject)", value = IsANode.class)
private static final class IsATypeErrorNode_ extends BaseNode_ {
IsATypeErrorNode_(IsANodeGen root) {
super(root, 3);
}
@Override
public boolean executeBoolean0(VirtualFrame frameValue) {
Object instanceValue_ = root.instance_.execute(frameValue);
DynamicObject moduleValue_;
try {
moduleValue_ = root.module_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
return getNext().executeBoolean_(instanceValue_, ex.getResult());
}
if ((!(RubyGuards.isRubyModule(moduleValue_)))) {
return root.isATypeError(instanceValue_, moduleValue_);
}
return getNext().executeBoolean_(instanceValue_, moduleValue_);
}
@Override
public boolean executeBoolean1(Object instanceValue, DynamicObject moduleValue) {
if ((!(RubyGuards.isRubyModule(moduleValue)))) {
return root.isATypeError(instanceValue, moduleValue);
}
return getNext().executeBoolean1(instanceValue, moduleValue);
}
@Override
public boolean executeBoolean_(Object instanceValue, Object moduleValue) {
if (moduleValue instanceof DynamicObject) {
DynamicObject moduleValue_ = (DynamicObject) moduleValue;
if ((!(RubyGuards.isRubyModule(moduleValue_)))) {
return root.isATypeError(instanceValue, moduleValue_);
}
}
return getNext().executeBoolean_(instanceValue, moduleValue);
}
static BaseNode_ create(IsANodeGen root) {
return new IsATypeErrorNode_(root);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy