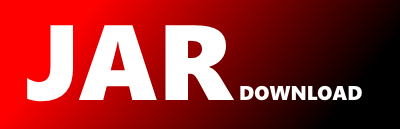
org.jruby.truffle.language.objects.ObjectIVarSetNodeGen Maven / Gradle / Ivy
The newest version!
// CheckStyle: start generated
package org.jruby.truffle.language.objects;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.internal.SpecializationNode;
import com.oracle.truffle.api.dsl.internal.SpecializedNode;
import com.oracle.truffle.api.dsl.internal.SuppressFBWarnings;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.object.DynamicObject;
import org.jruby.truffle.language.RubyNode;
@GeneratedBy(ObjectIVarSetNode.class)
@SuppressFBWarnings("SA_LOCAL_SELF_COMPARISON")
public final class ObjectIVarSetNodeGen extends ObjectIVarSetNode implements SpecializedNode {
@Child private RubyNode object_;
@Child private RubyNode name_;
@Child private RubyNode value_;
@CompilationFinal private boolean excludeIvarSetCached_;
@Child private BaseNode_ specialization_;
private ObjectIVarSetNodeGen(boolean checkName, RubyNode object, RubyNode name, RubyNode value) {
super(checkName);
this.object_ = object;
this.name_ = name;
this.value_ = value;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object executeIVarSet(DynamicObject objectValue, String nameValue, Object valueValue) {
return specialization_.execute1(objectValue, nameValue, valueValue);
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute0(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
public static ObjectIVarSetNode create(boolean checkName, RubyNode object, RubyNode name, RubyNode value) {
return new ObjectIVarSetNodeGen(checkName, object, name, value);
}
@GeneratedBy(ObjectIVarSetNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected ObjectIVarSetNodeGen root;
BaseNode_(ObjectIVarSetNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (ObjectIVarSetNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.object_, root.name_, root.value_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object objectValue, Object nameValue, Object valueValue) {
return this.execute_(objectValue, nameValue, valueValue);
}
public abstract Object execute_(Object objectValue, Object nameValue, Object valueValue);
public Object execute1(DynamicObject objectValue, String nameValue, Object valueValue) {
return execute_(objectValue, nameValue, valueValue);
}
public Object execute0(VirtualFrame frameValue) {
Object objectValue_ = root.object_.execute(frameValue);
Object nameValue_ = root.name_.execute(frameValue);
Object valueValue_ = root.value_.execute(frameValue);
return execute_(objectValue_, nameValue_, valueValue_);
}
public void executeVoid(VirtualFrame frameValue) {
execute0(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object objectValue, Object nameValue, Object valueValue) {
if (objectValue instanceof DynamicObject && nameValue instanceof String) {
DynamicObject objectValue_ = (DynamicObject) objectValue;
String nameValue_ = (String) nameValue;
String cachedName1 = (nameValue_);
if ((nameValue_ == cachedName1)) {
if (!root.excludeIvarSetCached_) {
WriteObjectFieldNode writeObjectFieldNode1 = (root.createWriteFieldNode(root.checkName(cachedName1, objectValue_)));
SpecializationNode s = IvarSetCachedNode_.create(root, cachedName1, writeObjectFieldNode1);
if (countSame(s) < (root.getCacheLimit())) {
return s;
}
}
}
root.excludeIvarSetCached_ = true;
return IvarSetUncachedNode_.create(root);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(ObjectIVarSetNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(ObjectIVarSetNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute_(Object objectValue, Object nameValue, Object valueValue) {
return uninitialized(null, objectValue, nameValue, valueValue);
}
static BaseNode_ create(ObjectIVarSetNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(ObjectIVarSetNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(ObjectIVarSetNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object objectValue, Object nameValue, Object valueValue) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, objectValue, nameValue, valueValue));
}
@Override
public Object execute_(Object objectValue, Object nameValue, Object valueValue) {
return getNext().execute_(objectValue, nameValue, valueValue);
}
static BaseNode_ create(ObjectIVarSetNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "ivarSetCached(DynamicObject, String, Object, String, WriteObjectFieldNode)", value = ObjectIVarSetNode.class)
private static final class IvarSetCachedNode_ extends BaseNode_ {
private final String cachedName;
@Child private WriteObjectFieldNode writeObjectFieldNode;
IvarSetCachedNode_(ObjectIVarSetNodeGen root, String cachedName, WriteObjectFieldNode writeObjectFieldNode) {
super(root, 1);
this.cachedName = cachedName;
this.writeObjectFieldNode = writeObjectFieldNode;
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object objectValue, Object nameValue, Object valueValue) {
if (newNode.getClass() == IvarSetUncachedNode_.class) {
removeSame("Contained by ivarSetUncached(DynamicObject, String, Object)");
}
return super.merge(newNode, frameValue, objectValue, nameValue, valueValue);
}
@Override
public boolean isIdentical(SpecializationNode other, Frame frameValue, Object objectValue, Object nameValue, Object valueValue) {
if (objectValue instanceof DynamicObject && nameValue instanceof String) {
String nameValue_ = (String) nameValue;
if ((nameValue_ == this.cachedName)) {
return true;
}
}
return false;
}
@Override
public Object execute1(DynamicObject objectValue, String nameValue, Object valueValue) {
if ((nameValue == this.cachedName)) {
return root.ivarSetCached(objectValue, nameValue, valueValue, this.cachedName, this.writeObjectFieldNode);
}
return getNext().execute1(objectValue, nameValue, valueValue);
}
@Override
public Object execute_(Object objectValue, Object nameValue, Object valueValue) {
if (objectValue instanceof DynamicObject && nameValue instanceof String) {
DynamicObject objectValue_ = (DynamicObject) objectValue;
String nameValue_ = (String) nameValue;
if ((nameValue_ == this.cachedName)) {
return root.ivarSetCached(objectValue_, nameValue_, valueValue, this.cachedName, this.writeObjectFieldNode);
}
}
return getNext().execute_(objectValue, nameValue, valueValue);
}
static BaseNode_ create(ObjectIVarSetNodeGen root, String cachedName, WriteObjectFieldNode writeObjectFieldNode) {
return new IvarSetCachedNode_(root, cachedName, writeObjectFieldNode);
}
}
@GeneratedBy(methodName = "ivarSetUncached(DynamicObject, String, Object)", value = ObjectIVarSetNode.class)
private static final class IvarSetUncachedNode_ extends BaseNode_ {
IvarSetUncachedNode_(ObjectIVarSetNodeGen root) {
super(root, 2);
}
@Override
public Object execute1(DynamicObject objectValue, String nameValue, Object valueValue) {
return root.ivarSetUncached(objectValue, nameValue, valueValue);
}
@Override
public Object execute_(Object objectValue, Object nameValue, Object valueValue) {
if (objectValue instanceof DynamicObject && nameValue instanceof String) {
DynamicObject objectValue_ = (DynamicObject) objectValue;
String nameValue_ = (String) nameValue;
return root.ivarSetUncached(objectValue_, nameValue_, valueValue);
}
return getNext().execute_(objectValue, nameValue, valueValue);
}
static BaseNode_ create(ObjectIVarSetNodeGen root) {
return new IvarSetUncachedNode_(root);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy