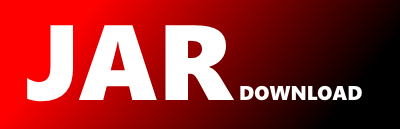
org.jruby.truffle.language.objects.shared.IsSharedNodeGen Maven / Gradle / Ivy
The newest version!
// CheckStyle: start generated
package org.jruby.truffle.language.objects.shared;
import com.oracle.truffle.api.Assumption;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.internal.SpecializationNode;
import com.oracle.truffle.api.dsl.internal.SpecializedNode;
import com.oracle.truffle.api.dsl.internal.SuppressFBWarnings;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.nodes.InvalidAssumptionException;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.object.DynamicObject;
import com.oracle.truffle.api.object.Shape;
import org.jruby.truffle.language.objects.ShapeCachingGuards;
@GeneratedBy(IsSharedNode.class)
@SuppressFBWarnings("SA_LOCAL_SELF_COMPARISON")
public final class IsSharedNodeGen extends IsSharedNode implements SpecializedNode {
@CompilationFinal private boolean excludeIsShareCached_;
@CompilationFinal private boolean excludeUpdateShapeAndIsShared_;
@Child private BaseNode_ specialization_;
private IsSharedNodeGen() {
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public boolean executeIsShared(DynamicObject arg0Value) {
return specialization_.executeBoolean(arg0Value);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
public static IsSharedNode create() {
return new IsSharedNodeGen();
}
@GeneratedBy(IsSharedNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected IsSharedNodeGen root;
BaseNode_(IsSharedNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (IsSharedNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {null};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arg0Value) {
return this.executeBoolean((DynamicObject) arg0Value);
}
public abstract boolean executeBoolean(DynamicObject arg0Value);
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arg0Value) {
if (arg0Value instanceof DynamicObject) {
DynamicObject arg0Value_ = (DynamicObject) arg0Value;
Shape cachedShape1 = (arg0Value_.getShape());
if ((arg0Value_.getShape() == cachedShape1)) {
if (!root.excludeIsShareCached_) {
boolean shared1 = (root.isShared(cachedShape1));
Assumption assumption0_1 = (cachedShape1.getValidAssumption());
if (isValid(assumption0_1)) {
SpecializationNode s = IsShareCachedNode_.create(root, cachedShape1, shared1, assumption0_1);
if (countSame(s) < (IsSharedNode.CACHE_LIMIT)) {
return s;
}
}
}
}
if ((ShapeCachingGuards.updateShape(arg0Value_))) {
if (!root.excludeUpdateShapeAndIsShared_) {
return UpdateShapeAndIsSharedNode_.create(root);
}
}
root.excludeIsShareCached_ = true;
root.excludeUpdateShapeAndIsShared_ = true;
return IsSharedUncachedNode_.create(root);
}
return null;
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(IsSharedNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(IsSharedNodeGen root) {
super(root, 2147483647);
}
@Override
public boolean executeBoolean(DynamicObject arg0Value) {
return (boolean) uninitialized(null, arg0Value);
}
static BaseNode_ create(IsSharedNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(methodName = "isShareCached(DynamicObject, Shape, boolean)", value = IsSharedNode.class)
private static final class IsShareCachedNode_ extends BaseNode_ {
private final Shape cachedShape;
private final boolean shared;
@CompilationFinal private final Assumption assumption0_;
IsShareCachedNode_(IsSharedNodeGen root, Shape cachedShape, boolean shared, Assumption assumption0_) {
super(root, 1);
this.cachedShape = cachedShape;
this.shared = shared;
this.assumption0_ = assumption0_;
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arg0Value) {
if (newNode.getClass() == IsSharedUncachedNode_.class) {
removeSame("Contained by isSharedUncached(DynamicObject)");
}
return super.merge(newNode, frameValue, arg0Value);
}
@Override
public boolean isIdentical(SpecializationNode other, Frame frameValue, Object arg0Value) {
if (arg0Value instanceof DynamicObject) {
DynamicObject arg0Value_ = (DynamicObject) arg0Value;
if ((arg0Value_.getShape() == this.cachedShape)) {
return true;
}
}
return false;
}
@Override
public boolean executeBoolean(DynamicObject arg0Value) {
try {
check(this.assumption0_);
} catch (InvalidAssumptionException ae) {
return (boolean) removeThis("Assumption [assumption0] invalidated", null, arg0Value);
}
if ((arg0Value.getShape() == this.cachedShape)) {
return root.isShareCached(arg0Value, this.cachedShape, this.shared);
}
return getNext().executeBoolean(arg0Value);
}
static BaseNode_ create(IsSharedNodeGen root, Shape cachedShape, boolean shared, Assumption assumption0_) {
return new IsShareCachedNode_(root, cachedShape, shared, assumption0_);
}
}
@GeneratedBy(methodName = "updateShapeAndIsShared(DynamicObject)", value = IsSharedNode.class)
private static final class UpdateShapeAndIsSharedNode_ extends BaseNode_ {
UpdateShapeAndIsSharedNode_(IsSharedNodeGen root) {
super(root, 2);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arg0Value) {
if (newNode.getClass() == IsSharedUncachedNode_.class) {
removeSame("Contained by isSharedUncached(DynamicObject)");
}
return super.merge(newNode, frameValue, arg0Value);
}
@Override
public boolean executeBoolean(DynamicObject arg0Value) {
if ((ShapeCachingGuards.updateShape(arg0Value))) {
return root.updateShapeAndIsShared(arg0Value);
}
return getNext().executeBoolean(arg0Value);
}
static BaseNode_ create(IsSharedNodeGen root) {
return new UpdateShapeAndIsSharedNode_(root);
}
}
@GeneratedBy(methodName = "isSharedUncached(DynamicObject)", value = IsSharedNode.class)
private static final class IsSharedUncachedNode_ extends BaseNode_ {
IsSharedUncachedNode_(IsSharedNodeGen root) {
super(root, 3);
}
@Override
public boolean executeBoolean(DynamicObject arg0Value) {
return root.isSharedUncached(arg0Value);
}
static BaseNode_ create(IsSharedNodeGen root) {
return new IsSharedUncachedNode_(root);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy