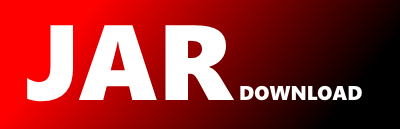
org.jruby.truffle.language.objects.shared.ShareInternalFieldsNodeGen Maven / Gradle / Ivy
The newest version!
// CheckStyle: start generated
package org.jruby.truffle.language.objects.shared;
import com.oracle.truffle.api.Assumption;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.internal.SpecializationNode;
import com.oracle.truffle.api.dsl.internal.SpecializedNode;
import com.oracle.truffle.api.dsl.internal.SuppressFBWarnings;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.nodes.InvalidAssumptionException;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.object.DynamicObject;
import com.oracle.truffle.api.object.Shape;
import com.oracle.truffle.api.profiles.ConditionProfile;
import org.jruby.truffle.core.array.ArrayGuards;
import org.jruby.truffle.language.objects.ShapeCachingGuards;
@GeneratedBy(ShareInternalFieldsNode.class)
@SuppressFBWarnings("SA_LOCAL_SELF_COMPARISON")
public final class ShareInternalFieldsNodeGen extends ShareInternalFieldsNode implements SpecializedNode {
@CompilationFinal private boolean excludeShareCachedObjectArray_;
@CompilationFinal private boolean excludeShareCachedOtherArray_;
@CompilationFinal private boolean excludeShareCachedQueue_;
@CompilationFinal private boolean excludeShareCachedBasicObject_;
@CompilationFinal private boolean excludeUpdateShapeAndShare_;
@Child private BaseNode_ specialization_;
private ShareInternalFieldsNodeGen(int depth) {
super(depth);
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public void executeShare(DynamicObject arg0Value) {
specialization_.executeVoid(arg0Value);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
public static ShareInternalFieldsNode create(int depth) {
return new ShareInternalFieldsNodeGen(depth);
}
@GeneratedBy(ShareInternalFieldsNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected ShareInternalFieldsNodeGen root;
BaseNode_(ShareInternalFieldsNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (ShareInternalFieldsNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {null};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arg0Value) {
this.executeVoid((DynamicObject) arg0Value);
return null;
}
public abstract void executeVoid(DynamicObject arg0Value);
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arg0Value) {
if (arg0Value instanceof DynamicObject) {
DynamicObject arg0Value_ = (DynamicObject) arg0Value;
Shape cachedShape1 = (arg0Value_.getShape());
if ((arg0Value_.getShape() == cachedShape1) && (ShapeCachingGuards.isArrayShape(cachedShape1)) && (ArrayGuards.isObjectArray(arg0Value_))) {
if (!root.excludeShareCachedObjectArray_) {
WriteBarrierNode writeBarrierNode1 = (root.createWriteBarrierNode());
Assumption assumption0_1 = (cachedShape1.getValidAssumption());
if (isValid(assumption0_1)) {
SpecializationNode s = ShareCachedObjectArrayNode_.create(root, cachedShape1, writeBarrierNode1, assumption0_1);
if (countSame(s) < (ShareInternalFieldsNode.CACHE_LIMIT)) {
return s;
}
}
}
}
Shape cachedShape2 = (arg0Value_.getShape());
if ((arg0Value_.getShape() == cachedShape2) && (ShapeCachingGuards.isArrayShape(cachedShape2)) && (!(ArrayGuards.isObjectArray(arg0Value_)))) {
if (!root.excludeShareCachedOtherArray_) {
Assumption assumption0_2 = (cachedShape2.getValidAssumption());
if (isValid(assumption0_2)) {
SpecializationNode s = ShareCachedOtherArrayNode_.create(root, cachedShape2, assumption0_2);
if (countSame(s) < (ShareInternalFieldsNode.CACHE_LIMIT)) {
return s;
}
}
}
}
Shape cachedShape3 = (arg0Value_.getShape());
if ((arg0Value_.getShape() == cachedShape3) && (ShapeCachingGuards.isQueueShape(cachedShape3))) {
if (!root.excludeShareCachedQueue_) {
ConditionProfile profileEmpty3 = (ConditionProfile.createBinaryProfile());
WriteBarrierNode writeBarrierNode3 = (root.createWriteBarrierNode());
Assumption assumption0_3 = (cachedShape3.getValidAssumption());
if (isValid(assumption0_3)) {
SpecializationNode s = ShareCachedQueueNode_.create(root, cachedShape3, profileEmpty3, writeBarrierNode3, assumption0_3);
if (countSame(s) < (ShareInternalFieldsNode.CACHE_LIMIT)) {
return s;
}
}
}
}
Shape cachedShape4 = (arg0Value_.getShape());
if ((arg0Value_.getShape() == cachedShape4) && (ShapeCachingGuards.isBasicObjectShape(cachedShape4))) {
if (!root.excludeShareCachedBasicObject_) {
Assumption assumption0_4 = (cachedShape4.getValidAssumption());
if (isValid(assumption0_4)) {
SpecializationNode s = ShareCachedBasicObjectNode_.create(root, cachedShape4, assumption0_4);
if (countSame(s) < (ShareInternalFieldsNode.CACHE_LIMIT)) {
return s;
}
}
}
}
if ((ShapeCachingGuards.updateShape(arg0Value_))) {
if (!root.excludeUpdateShapeAndShare_) {
return UpdateShapeAndShareNode_.create(root);
}
}
root.excludeShareCachedObjectArray_ = true;
root.excludeShareCachedOtherArray_ = true;
root.excludeShareCachedQueue_ = true;
root.excludeShareCachedBasicObject_ = true;
root.excludeUpdateShapeAndShare_ = true;
return ShareUncachedNode_.create(root);
}
return null;
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(ShareInternalFieldsNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(ShareInternalFieldsNodeGen root) {
super(root, 2147483647);
}
@Override
public void executeVoid(DynamicObject arg0Value) {
uninitialized(null, arg0Value);
return;
}
static BaseNode_ create(ShareInternalFieldsNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(methodName = "shareCachedObjectArray(DynamicObject, Shape, WriteBarrierNode)", value = ShareInternalFieldsNode.class)
private static final class ShareCachedObjectArrayNode_ extends BaseNode_ {
private final Shape cachedShape;
@Child private WriteBarrierNode writeBarrierNode;
@CompilationFinal private final Assumption assumption0_;
ShareCachedObjectArrayNode_(ShareInternalFieldsNodeGen root, Shape cachedShape, WriteBarrierNode writeBarrierNode, Assumption assumption0_) {
super(root, 1);
this.cachedShape = cachedShape;
this.writeBarrierNode = writeBarrierNode;
this.assumption0_ = assumption0_;
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arg0Value) {
if (newNode.getClass() == ShareUncachedNode_.class) {
removeSame("Contained by shareUncached(DynamicObject)");
}
return super.merge(newNode, frameValue, arg0Value);
}
@Override
public boolean isIdentical(SpecializationNode other, Frame frameValue, Object arg0Value) {
if (arg0Value instanceof DynamicObject) {
DynamicObject arg0Value_ = (DynamicObject) arg0Value;
if ((arg0Value_.getShape() == this.cachedShape) && (ArrayGuards.isObjectArray(arg0Value_))) {
assert (ShapeCachingGuards.isArrayShape(this.cachedShape));
return true;
}
}
return false;
}
@Override
public void executeVoid(DynamicObject arg0Value) {
try {
check(this.assumption0_);
} catch (InvalidAssumptionException ae) {
removeThis("Assumption [assumption0] invalidated", null, arg0Value);
return;
}
if ((arg0Value.getShape() == this.cachedShape) && (ArrayGuards.isObjectArray(arg0Value))) {
assert (ShapeCachingGuards.isArrayShape(this.cachedShape));
root.shareCachedObjectArray(arg0Value, this.cachedShape, this.writeBarrierNode);
return;
}
getNext().executeVoid(arg0Value);
return;
}
static BaseNode_ create(ShareInternalFieldsNodeGen root, Shape cachedShape, WriteBarrierNode writeBarrierNode, Assumption assumption0_) {
return new ShareCachedObjectArrayNode_(root, cachedShape, writeBarrierNode, assumption0_);
}
}
@GeneratedBy(methodName = "shareCachedOtherArray(DynamicObject, Shape)", value = ShareInternalFieldsNode.class)
private static final class ShareCachedOtherArrayNode_ extends BaseNode_ {
private final Shape cachedShape;
@CompilationFinal private final Assumption assumption0_;
ShareCachedOtherArrayNode_(ShareInternalFieldsNodeGen root, Shape cachedShape, Assumption assumption0_) {
super(root, 2);
this.cachedShape = cachedShape;
this.assumption0_ = assumption0_;
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arg0Value) {
if (newNode.getClass() == ShareUncachedNode_.class) {
removeSame("Contained by shareUncached(DynamicObject)");
}
return super.merge(newNode, frameValue, arg0Value);
}
@Override
public boolean isIdentical(SpecializationNode other, Frame frameValue, Object arg0Value) {
if (arg0Value instanceof DynamicObject) {
DynamicObject arg0Value_ = (DynamicObject) arg0Value;
if ((arg0Value_.getShape() == this.cachedShape) && (!(ArrayGuards.isObjectArray(arg0Value_)))) {
assert (ShapeCachingGuards.isArrayShape(this.cachedShape));
return true;
}
}
return false;
}
@Override
public void executeVoid(DynamicObject arg0Value) {
try {
check(this.assumption0_);
} catch (InvalidAssumptionException ae) {
removeThis("Assumption [assumption0] invalidated", null, arg0Value);
return;
}
if ((arg0Value.getShape() == this.cachedShape) && (!(ArrayGuards.isObjectArray(arg0Value)))) {
assert (ShapeCachingGuards.isArrayShape(this.cachedShape));
root.shareCachedOtherArray(arg0Value, this.cachedShape);
return;
}
getNext().executeVoid(arg0Value);
return;
}
static BaseNode_ create(ShareInternalFieldsNodeGen root, Shape cachedShape, Assumption assumption0_) {
return new ShareCachedOtherArrayNode_(root, cachedShape, assumption0_);
}
}
@GeneratedBy(methodName = "shareCachedQueue(DynamicObject, Shape, ConditionProfile, WriteBarrierNode)", value = ShareInternalFieldsNode.class)
private static final class ShareCachedQueueNode_ extends BaseNode_ {
private final Shape cachedShape;
private final ConditionProfile profileEmpty;
@Child private WriteBarrierNode writeBarrierNode;
@CompilationFinal private final Assumption assumption0_;
ShareCachedQueueNode_(ShareInternalFieldsNodeGen root, Shape cachedShape, ConditionProfile profileEmpty, WriteBarrierNode writeBarrierNode, Assumption assumption0_) {
super(root, 3);
this.cachedShape = cachedShape;
this.profileEmpty = profileEmpty;
this.writeBarrierNode = writeBarrierNode;
this.assumption0_ = assumption0_;
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arg0Value) {
if (newNode.getClass() == ShareUncachedNode_.class) {
removeSame("Contained by shareUncached(DynamicObject)");
}
return super.merge(newNode, frameValue, arg0Value);
}
@Override
public boolean isIdentical(SpecializationNode other, Frame frameValue, Object arg0Value) {
if (arg0Value instanceof DynamicObject) {
DynamicObject arg0Value_ = (DynamicObject) arg0Value;
if ((arg0Value_.getShape() == this.cachedShape)) {
assert (ShapeCachingGuards.isQueueShape(this.cachedShape));
return true;
}
}
return false;
}
@Override
public void executeVoid(DynamicObject arg0Value) {
try {
check(this.assumption0_);
} catch (InvalidAssumptionException ae) {
removeThis("Assumption [assumption0] invalidated", null, arg0Value);
return;
}
if ((arg0Value.getShape() == this.cachedShape)) {
assert (ShapeCachingGuards.isQueueShape(this.cachedShape));
root.shareCachedQueue(arg0Value, this.cachedShape, this.profileEmpty, this.writeBarrierNode);
return;
}
getNext().executeVoid(arg0Value);
return;
}
static BaseNode_ create(ShareInternalFieldsNodeGen root, Shape cachedShape, ConditionProfile profileEmpty, WriteBarrierNode writeBarrierNode, Assumption assumption0_) {
return new ShareCachedQueueNode_(root, cachedShape, profileEmpty, writeBarrierNode, assumption0_);
}
}
@GeneratedBy(methodName = "shareCachedBasicObject(DynamicObject, Shape)", value = ShareInternalFieldsNode.class)
private static final class ShareCachedBasicObjectNode_ extends BaseNode_ {
private final Shape cachedShape;
@CompilationFinal private final Assumption assumption0_;
ShareCachedBasicObjectNode_(ShareInternalFieldsNodeGen root, Shape cachedShape, Assumption assumption0_) {
super(root, 4);
this.cachedShape = cachedShape;
this.assumption0_ = assumption0_;
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arg0Value) {
if (newNode.getClass() == ShareUncachedNode_.class) {
removeSame("Contained by shareUncached(DynamicObject)");
}
return super.merge(newNode, frameValue, arg0Value);
}
@Override
public boolean isIdentical(SpecializationNode other, Frame frameValue, Object arg0Value) {
if (arg0Value instanceof DynamicObject) {
DynamicObject arg0Value_ = (DynamicObject) arg0Value;
if ((arg0Value_.getShape() == this.cachedShape)) {
assert (ShapeCachingGuards.isBasicObjectShape(this.cachedShape));
return true;
}
}
return false;
}
@Override
public void executeVoid(DynamicObject arg0Value) {
try {
check(this.assumption0_);
} catch (InvalidAssumptionException ae) {
removeThis("Assumption [assumption0] invalidated", null, arg0Value);
return;
}
if ((arg0Value.getShape() == this.cachedShape)) {
assert (ShapeCachingGuards.isBasicObjectShape(this.cachedShape));
root.shareCachedBasicObject(arg0Value, this.cachedShape);
return;
}
getNext().executeVoid(arg0Value);
return;
}
static BaseNode_ create(ShareInternalFieldsNodeGen root, Shape cachedShape, Assumption assumption0_) {
return new ShareCachedBasicObjectNode_(root, cachedShape, assumption0_);
}
}
@GeneratedBy(methodName = "updateShapeAndShare(DynamicObject)", value = ShareInternalFieldsNode.class)
private static final class UpdateShapeAndShareNode_ extends BaseNode_ {
UpdateShapeAndShareNode_(ShareInternalFieldsNodeGen root) {
super(root, 5);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arg0Value) {
if (newNode.getClass() == ShareUncachedNode_.class) {
removeSame("Contained by shareUncached(DynamicObject)");
}
return super.merge(newNode, frameValue, arg0Value);
}
@Override
public void executeVoid(DynamicObject arg0Value) {
if ((ShapeCachingGuards.updateShape(arg0Value))) {
root.updateShapeAndShare(arg0Value);
return;
}
getNext().executeVoid(arg0Value);
return;
}
static BaseNode_ create(ShareInternalFieldsNodeGen root) {
return new UpdateShapeAndShareNode_(root);
}
}
@GeneratedBy(methodName = "shareUncached(DynamicObject)", value = ShareInternalFieldsNode.class)
private static final class ShareUncachedNode_ extends BaseNode_ {
ShareUncachedNode_(ShareInternalFieldsNodeGen root) {
super(root, 6);
}
@Override
public void executeVoid(DynamicObject arg0Value) {
root.shareUncached(arg0Value);
return;
}
static BaseNode_ create(ShareInternalFieldsNodeGen root) {
return new ShareUncachedNode_(root);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy