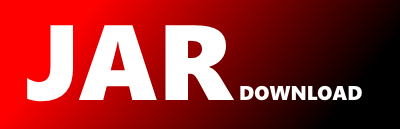
org.jruby.truffle.stdlib.bigdecimal.BigDecimalLayoutImpl Maven / Gradle / Ivy
The newest version!
package org.jruby.truffle.stdlib.bigdecimal;
import java.util.EnumSet;
import com.oracle.truffle.api.CompilerAsserts;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.object.DynamicObject;
import com.oracle.truffle.api.object.DynamicObjectFactory;
import com.oracle.truffle.api.object.FinalLocationException;
import com.oracle.truffle.api.object.HiddenKey;
import com.oracle.truffle.api.object.IncompatibleLocationException;
import com.oracle.truffle.api.object.LocationModifier;
import com.oracle.truffle.api.object.ObjectType;
import com.oracle.truffle.api.object.Property;
import com.oracle.truffle.api.object.Shape;
import org.jruby.truffle.stdlib.bigdecimal.BigDecimalLayout;
import org.jruby.truffle.core.basicobject.BasicObjectLayoutImpl;
@GeneratedBy(org.jruby.truffle.stdlib.bigdecimal.BigDecimalLayout.class)
public class BigDecimalLayoutImpl extends BasicObjectLayoutImpl implements BigDecimalLayout {
public static final BigDecimalLayout INSTANCE = new BigDecimalLayoutImpl();
public static class BigDecimalType extends BasicObjectLayoutImpl.BasicObjectType {
public BigDecimalType(
com.oracle.truffle.api.object.DynamicObject logicalClass,
com.oracle.truffle.api.object.DynamicObject metaClass) {
super(
logicalClass,
metaClass);
}
@Override
public BigDecimalType setLogicalClass(com.oracle.truffle.api.object.DynamicObject logicalClass) {
return new BigDecimalType(
logicalClass,
metaClass);
}
@Override
public BigDecimalType setMetaClass(com.oracle.truffle.api.object.DynamicObject metaClass) {
return new BigDecimalType(
logicalClass,
metaClass);
}
}
protected static final Shape.Allocator BIG_DECIMAL_ALLOCATOR = LAYOUT.createAllocator();
protected static final HiddenKey VALUE_IDENTIFIER = new HiddenKey("value");
protected static final Property VALUE_PROPERTY = Property.create(VALUE_IDENTIFIER, BIG_DECIMAL_ALLOCATOR.locationForType(java.math.BigDecimal.class, EnumSet.of(LocationModifier.NonNull)), 0);
protected static final HiddenKey TYPE_IDENTIFIER = new HiddenKey("type");
protected static final Property TYPE_PROPERTY = Property.create(TYPE_IDENTIFIER, BIG_DECIMAL_ALLOCATOR.locationForType(org.jruby.truffle.stdlib.bigdecimal.BigDecimalType.class, EnumSet.of(LocationModifier.NonNull)), 0);
protected BigDecimalLayoutImpl() {
}
@Override
public DynamicObjectFactory createBigDecimalShape(
com.oracle.truffle.api.object.DynamicObject logicalClass,
com.oracle.truffle.api.object.DynamicObject metaClass) {
return LAYOUT.createShape(new BigDecimalType(
logicalClass,
metaClass))
.addProperty(VALUE_PROPERTY)
.addProperty(TYPE_PROPERTY)
.createFactory();
}
@Override
public DynamicObject createBigDecimal(
DynamicObjectFactory factory,
java.math.BigDecimal value,
org.jruby.truffle.stdlib.bigdecimal.BigDecimalType type) {
assert factory != null;
CompilerAsserts.partialEvaluationConstant(factory);
assert createsBigDecimal(factory);
assert factory.getShape().hasProperty(VALUE_IDENTIFIER);
assert factory.getShape().hasProperty(TYPE_IDENTIFIER);
assert value != null;
assert type != null;
return factory.newInstance(
value,
type);
}
@Override
public boolean isBigDecimal(DynamicObject object) {
return isBigDecimal(object.getShape().getObjectType());
}
private static boolean isBigDecimal(ObjectType objectType) {
return objectType instanceof BigDecimalType;
}
private static boolean createsBigDecimal(DynamicObjectFactory factory) {
return isBigDecimal(factory.getShape().getObjectType());
}
@Override
public java.math.BigDecimal getValue(DynamicObject object) {
assert isBigDecimal(object);
assert object.getShape().hasProperty(VALUE_IDENTIFIER);
return (java.math.BigDecimal) VALUE_PROPERTY.get(object, isBigDecimal(object));
}
@Override
public void setValue(DynamicObject object, java.math.BigDecimal value) {
assert isBigDecimal(object);
assert object.getShape().hasProperty(VALUE_IDENTIFIER);
assert value != null;
try {
VALUE_PROPERTY.set(object, value, object.getShape());
} catch (IncompatibleLocationException | FinalLocationException e) {
throw new UnsupportedOperationException(e);
}
}
@Override
public org.jruby.truffle.stdlib.bigdecimal.BigDecimalType getType(DynamicObject object) {
assert isBigDecimal(object);
assert object.getShape().hasProperty(TYPE_IDENTIFIER);
return (org.jruby.truffle.stdlib.bigdecimal.BigDecimalType) TYPE_PROPERTY.get(object, isBigDecimal(object));
}
@Override
public void setType(DynamicObject object, org.jruby.truffle.stdlib.bigdecimal.BigDecimalType value) {
assert isBigDecimal(object);
assert object.getShape().hasProperty(TYPE_IDENTIFIER);
assert value != null;
try {
TYPE_PROPERTY.set(object, value, object.getShape());
} catch (IncompatibleLocationException | FinalLocationException e) {
throw new UnsupportedOperationException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy