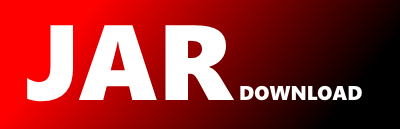
org.jruby.truffle.stdlib.bigdecimal.CreateBigDecimalNodeFactory Maven / Gradle / Ivy
// CheckStyle: start generated
package org.jruby.truffle.stdlib.bigdecimal;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NodeFactory;
import com.oracle.truffle.api.dsl.internal.SpecializationNode;
import com.oracle.truffle.api.dsl.internal.SpecializedNode;
import com.oracle.truffle.api.dsl.internal.SuppressFBWarnings;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.nodes.UnexpectedResultException;
import com.oracle.truffle.api.object.DynamicObject;
import com.oracle.truffle.api.profiles.ConditionProfile;
import java.math.BigDecimal;
import java.util.Arrays;
import java.util.List;
import org.jruby.truffle.core.cast.BooleanCastNode;
import org.jruby.truffle.language.NotProvided;
import org.jruby.truffle.language.RubyGuards;
import org.jruby.truffle.language.RubyNode;
import org.jruby.truffle.language.RubyTypes;
import org.jruby.truffle.language.RubyTypesGen;
import org.jruby.truffle.language.dispatch.CallDispatchHeadNode;
@GeneratedBy(CreateBigDecimalNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public final class CreateBigDecimalNodeFactory implements NodeFactory {
private static CreateBigDecimalNodeFactory instance;
private CreateBigDecimalNodeFactory() {
}
@Override
public Class getNodeClass() {
return CreateBigDecimalNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode.class, RubyNode.class, RubyNode.class));
}
@Override
public CreateBigDecimalNode createNode(Object... arguments) {
if (arguments.length == 3 && (arguments[0] == null || arguments[0] instanceof RubyNode) && (arguments[1] == null || arguments[1] instanceof RubyNode) && (arguments[2] == null || arguments[2] instanceof RubyNode)) {
return create((RubyNode) arguments[0], (RubyNode) arguments[1], (RubyNode) arguments[2]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (instance == null) {
instance = new CreateBigDecimalNodeFactory();
}
return instance;
}
public static CreateBigDecimalNode create(RubyNode value, RubyNode self, RubyNode digits) {
return new CreateBigDecimalNodeGen(value, self, digits);
}
@GeneratedBy(CreateBigDecimalNode.class)
@SuppressFBWarnings("SA_LOCAL_SELF_COMPARISON")
public static final class CreateBigDecimalNodeGen extends CreateBigDecimalNode implements SpecializedNode {
@Child private RubyNode value_;
@Child private RubyNode self_;
@Child private RubyNode digits_;
@CompilationFinal private Class> valueType_;
@CompilationFinal private Class> digitsType_;
@Child private BaseNode_ specialization_;
private CreateBigDecimalNodeGen(RubyNode value, RubyNode self, RubyNode digits) {
this.value_ = value;
this.self_ = self;
this.digits_ = digits;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public DynamicObject executeInitialize(VirtualFrame frameValue, Object valueValue, DynamicObject selfValue, Object digitsValue) {
return specialization_.executeDynamicObject1(frameValue, valueValue, selfValue, digitsValue);
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
return specialization_.executeDynamicObject0(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
private static BigDecimal expectBigDecimal(Object value) throws UnexpectedResultException {
if (value instanceof BigDecimal) {
return (BigDecimal) value;
}
throw new UnexpectedResultException(value);
}
@GeneratedBy(CreateBigDecimalNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected CreateBigDecimalNodeGen root;
BaseNode_(CreateBigDecimalNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (CreateBigDecimalNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.value_, root.self_, root.digits_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object valueValue, Object selfValue, Object digitsValue) {
return this.executeDynamicObject_((VirtualFrame) frameValue, valueValue, selfValue, digitsValue);
}
public abstract DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object valueValue, Object selfValue, Object digitsValue);
public DynamicObject executeDynamicObject1(VirtualFrame frameValue, Object valueValue, DynamicObject selfValue, Object digitsValue) {
return executeDynamicObject_(frameValue, valueValue, selfValue, digitsValue);
}
public Object execute(VirtualFrame frameValue) {
Object valueValue_ = executeValue_(frameValue);
Object selfValue_ = root.self_.execute(frameValue);
Object digitsValue_ = executeDigits_(frameValue);
return executeDynamicObject_(frameValue, valueValue_, selfValue_, digitsValue_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public DynamicObject executeDynamicObject0(VirtualFrame frameValue) {
return (DynamicObject) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object valueValue, Object selfValue, Object digitsValue) {
if (selfValue instanceof DynamicObject) {
if (RubyTypesGen.isImplicitLong(valueValue)) {
if (digitsValue instanceof NotProvided) {
return Create0Node_.create(root, valueValue);
}
if (RubyTypesGen.isImplicitInteger(digitsValue)) {
BigDecimalCastNode bigDecimalCastNode2 = (root.createBigDecimalCastNode());
return Create1Node_.create(root, valueValue, digitsValue, bigDecimalCastNode2);
}
}
if (RubyTypesGen.isImplicitDouble(valueValue)) {
if (digitsValue instanceof NotProvided) {
return Create2Node_.create(root, valueValue);
}
if (RubyTypesGen.isImplicitInteger(digitsValue)) {
BigDecimalCastNode bigDecimalCastNode4 = (root.createBigDecimalCastNode());
return Create3Node_.create(root, valueValue, digitsValue, bigDecimalCastNode4);
}
}
if (valueValue instanceof BigDecimalType) {
BigDecimalType valueValue_ = (BigDecimalType) valueValue;
if ((valueValue_ == BigDecimalType.NEGATIVE_INFINITY || valueValue_ == BigDecimalType.POSITIVE_INFINITY)) {
BooleanCastNode booleanCastNode5 = (root.createBooleanCastNode());
GetIntegerConstantNode getIntegerConstantNode5 = (root.createGetIntegerConstantNode());
CallDispatchHeadNode modeCallNode5 = (CallDispatchHeadNode.createMethodCallIgnoreVisibility());
ConditionProfile raiseProfile5 = (ConditionProfile.createBinaryProfile());
return CreateInfinityNode_.create(root, booleanCastNode5, getIntegerConstantNode5, modeCallNode5, raiseProfile5);
}
if ((valueValue_ == BigDecimalType.NAN)) {
BooleanCastNode booleanCastNode6 = (root.createBooleanCastNode());
GetIntegerConstantNode getIntegerConstantNode6 = (root.createGetIntegerConstantNode());
CallDispatchHeadNode modeCallNode6 = (CallDispatchHeadNode.createMethodCallIgnoreVisibility());
ConditionProfile raiseProfile6 = (ConditionProfile.createBinaryProfile());
return CreateNaNNode_.create(root, booleanCastNode6, getIntegerConstantNode6, modeCallNode6, raiseProfile6);
}
if ((valueValue_ == BigDecimalType.NEGATIVE_ZERO)) {
return CreateNegativeZeroNode_.create(root);
}
}
if (valueValue instanceof BigDecimal) {
if (digitsValue instanceof NotProvided) {
return Create4Node_.create(root);
}
if (RubyTypesGen.isImplicitInteger(digitsValue)) {
return Create5Node_.create(root, digitsValue);
}
}
if (valueValue instanceof DynamicObject) {
DynamicObject valueValue_ = (DynamicObject) valueValue;
if (digitsValue instanceof NotProvided) {
if ((RubyGuards.isRubyBignum(valueValue_))) {
return CreateBignum0Node_.create(root);
}
}
if (RubyTypesGen.isImplicitInteger(digitsValue)) {
if ((RubyGuards.isRubyBignum(valueValue_))) {
return CreateBignum1Node_.create(root, digitsValue);
}
}
if (digitsValue instanceof NotProvided) {
if ((RubyGuards.isRubyBigDecimal(valueValue_))) {
return CreateBigDecimal0Node_.create(root);
}
}
if (RubyTypesGen.isImplicitInteger(digitsValue)) {
if ((RubyGuards.isRubyBigDecimal(valueValue_))) {
return CreateBigDecimal1Node_.create(root, digitsValue);
}
}
if (digitsValue instanceof NotProvided) {
if ((RubyGuards.isRubyString(valueValue_))) {
return CreateString0Node_.create(root);
}
}
if (RubyTypesGen.isImplicitInteger(digitsValue)) {
if ((RubyGuards.isRubyString(valueValue_))) {
return CreateString1Node_.create(root, digitsValue);
}
if ((!(RubyGuards.isRubyBignum(valueValue_))) && (!(RubyGuards.isRubyBigDecimal(valueValue_))) && (!(RubyGuards.isRubyString(valueValue_)))) {
BigDecimalCastNode bigDecimalCastNode16 = (root.createBigDecimalCastNode());
ConditionProfile nilProfile16 = (ConditionProfile.createBinaryProfile());
return Create6Node_.create(root, digitsValue, bigDecimalCastNode16, nilProfile16);
}
}
}
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final long executeValueLong_(Frame frameValue, Class> valueImplicitType) throws UnexpectedResultException {
if (valueImplicitType == long.class) {
return root.value_.executeLong((VirtualFrame) frameValue);
} else if (valueImplicitType == int.class) {
return RubyTypes.promoteToLong(root.value_.executeInteger((VirtualFrame) frameValue));
} else {
Object valueValue_ = executeValue_(frameValue);
return RubyTypesGen.expectImplicitLong(valueValue_, valueImplicitType);
}
}
protected final Object executeValue_(Frame frameValue) {
Class> valueType_ = root.valueType_;
try {
if (valueType_ == double.class) {
return root.value_.executeDouble((VirtualFrame) frameValue);
} else if (valueType_ == int.class) {
return root.value_.executeInteger((VirtualFrame) frameValue);
} else if (valueType_ == long.class) {
return root.value_.executeLong((VirtualFrame) frameValue);
} else if (valueType_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.value_.execute((VirtualFrame) frameValue);
if (_value instanceof Double) {
_type = double.class;
} else if (_value instanceof Integer) {
_type = int.class;
} else if (_value instanceof Long) {
_type = long.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.valueType_ = _type;
}
} else {
return root.value_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.valueType_ = Object.class;
return ex.getResult();
}
}
protected final Object executeDigits_(Frame frameValue) {
Class> digitsType_ = root.digitsType_;
try {
if (digitsType_ == int.class) {
return root.digits_.executeInteger((VirtualFrame) frameValue);
} else if (digitsType_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.digits_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.digitsType_ = _type;
}
} else {
return root.digits_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.digitsType_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(CreateBigDecimalNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(CreateBigDecimalNodeGen root) {
super(root, 2147483647);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object valueValue, Object selfValue, Object digitsValue) {
return (DynamicObject) uninitialized(frameValue, valueValue, selfValue, digitsValue);
}
static BaseNode_ create(CreateBigDecimalNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(CreateBigDecimalNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(CreateBigDecimalNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object valueValue, Object selfValue, Object digitsValue) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, valueValue, selfValue, digitsValue));
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object valueValue, Object selfValue, Object digitsValue) {
return getNext().executeDynamicObject_(frameValue, valueValue, selfValue, digitsValue);
}
static BaseNode_ create(CreateBigDecimalNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "create(VirtualFrame, long, DynamicObject, NotProvided)", value = CreateBigDecimalNode.class)
private static final class Create0Node_ extends BaseNode_ {
private final Class> valueImplicitType;
Create0Node_(CreateBigDecimalNodeGen root, Object valueValue) {
super(root, 1);
this.valueImplicitType = RubyTypesGen.getImplicitLongClass(valueValue);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.valueImplicitType == ((Create0Node_) other).valueImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject0(frameValue);
}
@Override
public DynamicObject executeDynamicObject0(VirtualFrame frameValue) {
long valueValue_;
try {
valueValue_ = executeValueLong_(frameValue, valueImplicitType);
} catch (UnexpectedResultException ex) {
Object selfValue = root.self_.execute(frameValue);
Object digitsValue = executeDigits_(frameValue);
return getNext().executeDynamicObject_(frameValue, ex.getResult(), selfValue, digitsValue);
}
DynamicObject selfValue_;
try {
selfValue_ = root.self_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object digitsValue = executeDigits_(frameValue);
return getNext().executeDynamicObject_(frameValue, valueValue_, ex.getResult(), digitsValue);
}
NotProvided digitsValue_;
try {
digitsValue_ = root.digits_.executeNotProvided(frameValue);
} catch (UnexpectedResultException ex) {
return getNext().executeDynamicObject_(frameValue, valueValue_, selfValue_, ex.getResult());
}
return root.create(frameValue, valueValue_, selfValue_, digitsValue_);
}
@Override
public DynamicObject executeDynamicObject1(VirtualFrame frameValue, Object valueValue, DynamicObject selfValue, Object digitsValue) {
if (RubyTypesGen.isImplicitLong(valueValue, valueImplicitType) && digitsValue instanceof NotProvided) {
long valueValue_ = RubyTypesGen.asImplicitLong(valueValue, valueImplicitType);
NotProvided digitsValue_ = (NotProvided) digitsValue;
return root.create(frameValue, valueValue_, selfValue, digitsValue_);
}
return getNext().executeDynamicObject1(frameValue, valueValue, selfValue, digitsValue);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object valueValue, Object selfValue, Object digitsValue) {
if (RubyTypesGen.isImplicitLong(valueValue, valueImplicitType) && selfValue instanceof DynamicObject && digitsValue instanceof NotProvided) {
long valueValue_ = RubyTypesGen.asImplicitLong(valueValue, valueImplicitType);
DynamicObject selfValue_ = (DynamicObject) selfValue;
NotProvided digitsValue_ = (NotProvided) digitsValue;
return root.create(frameValue, valueValue_, selfValue_, digitsValue_);
}
return getNext().executeDynamicObject_(frameValue, valueValue, selfValue, digitsValue);
}
static BaseNode_ create(CreateBigDecimalNodeGen root, Object valueValue) {
return new Create0Node_(root, valueValue);
}
}
@GeneratedBy(methodName = "create(VirtualFrame, long, DynamicObject, int, BigDecimalCastNode)", value = CreateBigDecimalNode.class)
private static final class Create1Node_ extends BaseNode_ {
@Child private BigDecimalCastNode bigDecimalCastNode;
private final Class> valueImplicitType;
private final Class> digitsImplicitType;
Create1Node_(CreateBigDecimalNodeGen root, Object valueValue, Object digitsValue, BigDecimalCastNode bigDecimalCastNode) {
super(root, 2);
this.valueImplicitType = RubyTypesGen.getImplicitLongClass(valueValue);
this.digitsImplicitType = RubyTypesGen.getImplicitIntegerClass(digitsValue);
this.bigDecimalCastNode = bigDecimalCastNode;
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.valueImplicitType == ((Create1Node_) other).valueImplicitType && this.digitsImplicitType == ((Create1Node_) other).digitsImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject0(frameValue);
}
@Override
public DynamicObject executeDynamicObject0(VirtualFrame frameValue) {
long valueValue_;
try {
valueValue_ = executeValueLong_(frameValue, valueImplicitType);
} catch (UnexpectedResultException ex) {
Object selfValue = root.self_.execute(frameValue);
Object digitsValue = executeDigits_(frameValue);
return getNext().executeDynamicObject_(frameValue, ex.getResult(), selfValue, digitsValue);
}
DynamicObject selfValue_;
try {
selfValue_ = root.self_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object digitsValue = executeDigits_(frameValue);
return getNext().executeDynamicObject_(frameValue, valueValue_, ex.getResult(), digitsValue);
}
int digitsValue_;
try {
if (digitsImplicitType == int.class) {
digitsValue_ = root.digits_.executeInteger(frameValue);
} else {
Object digitsValue__ = executeDigits_(frameValue);
digitsValue_ = RubyTypesGen.expectImplicitInteger(digitsValue__, digitsImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeDynamicObject_(frameValue, valueValue_, selfValue_, ex.getResult());
}
return root.create(frameValue, valueValue_, selfValue_, digitsValue_, this.bigDecimalCastNode);
}
@Override
public DynamicObject executeDynamicObject1(VirtualFrame frameValue, Object valueValue, DynamicObject selfValue, Object digitsValue) {
if (RubyTypesGen.isImplicitLong(valueValue, valueImplicitType) && RubyTypesGen.isImplicitInteger(digitsValue, digitsImplicitType)) {
long valueValue_ = RubyTypesGen.asImplicitLong(valueValue, valueImplicitType);
int digitsValue_ = RubyTypesGen.asImplicitInteger(digitsValue, digitsImplicitType);
return root.create(frameValue, valueValue_, selfValue, digitsValue_, this.bigDecimalCastNode);
}
return getNext().executeDynamicObject1(frameValue, valueValue, selfValue, digitsValue);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object valueValue, Object selfValue, Object digitsValue) {
if (RubyTypesGen.isImplicitLong(valueValue, valueImplicitType) && selfValue instanceof DynamicObject && RubyTypesGen.isImplicitInteger(digitsValue, digitsImplicitType)) {
long valueValue_ = RubyTypesGen.asImplicitLong(valueValue, valueImplicitType);
DynamicObject selfValue_ = (DynamicObject) selfValue;
int digitsValue_ = RubyTypesGen.asImplicitInteger(digitsValue, digitsImplicitType);
return root.create(frameValue, valueValue_, selfValue_, digitsValue_, this.bigDecimalCastNode);
}
return getNext().executeDynamicObject_(frameValue, valueValue, selfValue, digitsValue);
}
static BaseNode_ create(CreateBigDecimalNodeGen root, Object valueValue, Object digitsValue, BigDecimalCastNode bigDecimalCastNode) {
return new Create1Node_(root, valueValue, digitsValue, bigDecimalCastNode);
}
}
@GeneratedBy(methodName = "create(double, DynamicObject, NotProvided)", value = CreateBigDecimalNode.class)
private static final class Create2Node_ extends BaseNode_ {
private final Class> valueImplicitType;
Create2Node_(CreateBigDecimalNodeGen root, Object valueValue) {
super(root, 3);
this.valueImplicitType = RubyTypesGen.getImplicitDoubleClass(valueValue);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.valueImplicitType == ((Create2Node_) other).valueImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject0(frameValue);
}
@Override
public DynamicObject executeDynamicObject0(VirtualFrame frameValue) {
double valueValue_;
try {
if (valueImplicitType == double.class) {
valueValue_ = root.value_.executeDouble(frameValue);
} else {
Object valueValue__ = executeValue_(frameValue);
valueValue_ = RubyTypesGen.expectImplicitDouble(valueValue__, valueImplicitType);
}
} catch (UnexpectedResultException ex) {
Object selfValue = root.self_.execute(frameValue);
Object digitsValue = executeDigits_(frameValue);
return getNext().executeDynamicObject_(frameValue, ex.getResult(), selfValue, digitsValue);
}
DynamicObject selfValue_;
try {
selfValue_ = root.self_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object digitsValue = executeDigits_(frameValue);
return getNext().executeDynamicObject_(frameValue, valueValue_, ex.getResult(), digitsValue);
}
NotProvided digitsValue_;
try {
digitsValue_ = root.digits_.executeNotProvided(frameValue);
} catch (UnexpectedResultException ex) {
return getNext().executeDynamicObject_(frameValue, valueValue_, selfValue_, ex.getResult());
}
return root.create(valueValue_, selfValue_, digitsValue_);
}
@Override
public DynamicObject executeDynamicObject1(VirtualFrame frameValue, Object valueValue, DynamicObject selfValue, Object digitsValue) {
if (RubyTypesGen.isImplicitDouble(valueValue, valueImplicitType) && digitsValue instanceof NotProvided) {
double valueValue_ = RubyTypesGen.asImplicitDouble(valueValue, valueImplicitType);
NotProvided digitsValue_ = (NotProvided) digitsValue;
return root.create(valueValue_, selfValue, digitsValue_);
}
return getNext().executeDynamicObject1(frameValue, valueValue, selfValue, digitsValue);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object valueValue, Object selfValue, Object digitsValue) {
if (RubyTypesGen.isImplicitDouble(valueValue, valueImplicitType) && selfValue instanceof DynamicObject && digitsValue instanceof NotProvided) {
double valueValue_ = RubyTypesGen.asImplicitDouble(valueValue, valueImplicitType);
DynamicObject selfValue_ = (DynamicObject) selfValue;
NotProvided digitsValue_ = (NotProvided) digitsValue;
return root.create(valueValue_, selfValue_, digitsValue_);
}
return getNext().executeDynamicObject_(frameValue, valueValue, selfValue, digitsValue);
}
static BaseNode_ create(CreateBigDecimalNodeGen root, Object valueValue) {
return new Create2Node_(root, valueValue);
}
}
@GeneratedBy(methodName = "create(VirtualFrame, double, DynamicObject, int, BigDecimalCastNode)", value = CreateBigDecimalNode.class)
private static final class Create3Node_ extends BaseNode_ {
@Child private BigDecimalCastNode bigDecimalCastNode;
private final Class> valueImplicitType;
private final Class> digitsImplicitType;
Create3Node_(CreateBigDecimalNodeGen root, Object valueValue, Object digitsValue, BigDecimalCastNode bigDecimalCastNode) {
super(root, 4);
this.valueImplicitType = RubyTypesGen.getImplicitDoubleClass(valueValue);
this.digitsImplicitType = RubyTypesGen.getImplicitIntegerClass(digitsValue);
this.bigDecimalCastNode = bigDecimalCastNode;
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.valueImplicitType == ((Create3Node_) other).valueImplicitType && this.digitsImplicitType == ((Create3Node_) other).digitsImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject0(frameValue);
}
@Override
public DynamicObject executeDynamicObject0(VirtualFrame frameValue) {
double valueValue_;
try {
if (valueImplicitType == double.class) {
valueValue_ = root.value_.executeDouble(frameValue);
} else {
Object valueValue__ = executeValue_(frameValue);
valueValue_ = RubyTypesGen.expectImplicitDouble(valueValue__, valueImplicitType);
}
} catch (UnexpectedResultException ex) {
Object selfValue = root.self_.execute(frameValue);
Object digitsValue = executeDigits_(frameValue);
return getNext().executeDynamicObject_(frameValue, ex.getResult(), selfValue, digitsValue);
}
DynamicObject selfValue_;
try {
selfValue_ = root.self_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object digitsValue = executeDigits_(frameValue);
return getNext().executeDynamicObject_(frameValue, valueValue_, ex.getResult(), digitsValue);
}
int digitsValue_;
try {
if (digitsImplicitType == int.class) {
digitsValue_ = root.digits_.executeInteger(frameValue);
} else {
Object digitsValue__ = executeDigits_(frameValue);
digitsValue_ = RubyTypesGen.expectImplicitInteger(digitsValue__, digitsImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeDynamicObject_(frameValue, valueValue_, selfValue_, ex.getResult());
}
return root.create(frameValue, valueValue_, selfValue_, digitsValue_, this.bigDecimalCastNode);
}
@Override
public DynamicObject executeDynamicObject1(VirtualFrame frameValue, Object valueValue, DynamicObject selfValue, Object digitsValue) {
if (RubyTypesGen.isImplicitDouble(valueValue, valueImplicitType) && RubyTypesGen.isImplicitInteger(digitsValue, digitsImplicitType)) {
double valueValue_ = RubyTypesGen.asImplicitDouble(valueValue, valueImplicitType);
int digitsValue_ = RubyTypesGen.asImplicitInteger(digitsValue, digitsImplicitType);
return root.create(frameValue, valueValue_, selfValue, digitsValue_, this.bigDecimalCastNode);
}
return getNext().executeDynamicObject1(frameValue, valueValue, selfValue, digitsValue);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object valueValue, Object selfValue, Object digitsValue) {
if (RubyTypesGen.isImplicitDouble(valueValue, valueImplicitType) && selfValue instanceof DynamicObject && RubyTypesGen.isImplicitInteger(digitsValue, digitsImplicitType)) {
double valueValue_ = RubyTypesGen.asImplicitDouble(valueValue, valueImplicitType);
DynamicObject selfValue_ = (DynamicObject) selfValue;
int digitsValue_ = RubyTypesGen.asImplicitInteger(digitsValue, digitsImplicitType);
return root.create(frameValue, valueValue_, selfValue_, digitsValue_, this.bigDecimalCastNode);
}
return getNext().executeDynamicObject_(frameValue, valueValue, selfValue, digitsValue);
}
static BaseNode_ create(CreateBigDecimalNodeGen root, Object valueValue, Object digitsValue, BigDecimalCastNode bigDecimalCastNode) {
return new Create3Node_(root, valueValue, digitsValue, bigDecimalCastNode);
}
}
@GeneratedBy(methodName = "createInfinity(VirtualFrame, BigDecimalType, DynamicObject, Object, BooleanCastNode, GetIntegerConstantNode, CallDispatchHeadNode, ConditionProfile)", value = CreateBigDecimalNode.class)
private static final class CreateInfinityNode_ extends BaseNode_ {
@Child private BooleanCastNode booleanCastNode;
@Child private GetIntegerConstantNode getIntegerConstantNode;
@Child private CallDispatchHeadNode modeCallNode;
private final ConditionProfile raiseProfile;
CreateInfinityNode_(CreateBigDecimalNodeGen root, BooleanCastNode booleanCastNode, GetIntegerConstantNode getIntegerConstantNode, CallDispatchHeadNode modeCallNode, ConditionProfile raiseProfile) {
super(root, 5);
this.booleanCastNode = booleanCastNode;
this.getIntegerConstantNode = getIntegerConstantNode;
this.modeCallNode = modeCallNode;
this.raiseProfile = raiseProfile;
}
@Override
public DynamicObject executeDynamicObject1(VirtualFrame frameValue, Object valueValue, DynamicObject selfValue, Object digitsValue) {
if (valueValue instanceof BigDecimalType) {
BigDecimalType valueValue_ = (BigDecimalType) valueValue;
if ((valueValue_ == BigDecimalType.NEGATIVE_INFINITY || valueValue_ == BigDecimalType.POSITIVE_INFINITY)) {
return root.createInfinity(frameValue, valueValue_, selfValue, digitsValue, this.booleanCastNode, this.getIntegerConstantNode, this.modeCallNode, this.raiseProfile);
}
}
return getNext().executeDynamicObject1(frameValue, valueValue, selfValue, digitsValue);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object valueValue, Object selfValue, Object digitsValue) {
if (valueValue instanceof BigDecimalType && selfValue instanceof DynamicObject) {
BigDecimalType valueValue_ = (BigDecimalType) valueValue;
DynamicObject selfValue_ = (DynamicObject) selfValue;
if ((valueValue_ == BigDecimalType.NEGATIVE_INFINITY || valueValue_ == BigDecimalType.POSITIVE_INFINITY)) {
return root.createInfinity(frameValue, valueValue_, selfValue_, digitsValue, this.booleanCastNode, this.getIntegerConstantNode, this.modeCallNode, this.raiseProfile);
}
}
return getNext().executeDynamicObject_(frameValue, valueValue, selfValue, digitsValue);
}
static BaseNode_ create(CreateBigDecimalNodeGen root, BooleanCastNode booleanCastNode, GetIntegerConstantNode getIntegerConstantNode, CallDispatchHeadNode modeCallNode, ConditionProfile raiseProfile) {
return new CreateInfinityNode_(root, booleanCastNode, getIntegerConstantNode, modeCallNode, raiseProfile);
}
}
@GeneratedBy(methodName = "createNaN(VirtualFrame, BigDecimalType, DynamicObject, Object, BooleanCastNode, GetIntegerConstantNode, CallDispatchHeadNode, ConditionProfile)", value = CreateBigDecimalNode.class)
private static final class CreateNaNNode_ extends BaseNode_ {
@Child private BooleanCastNode booleanCastNode;
@Child private GetIntegerConstantNode getIntegerConstantNode;
@Child private CallDispatchHeadNode modeCallNode;
private final ConditionProfile raiseProfile;
CreateNaNNode_(CreateBigDecimalNodeGen root, BooleanCastNode booleanCastNode, GetIntegerConstantNode getIntegerConstantNode, CallDispatchHeadNode modeCallNode, ConditionProfile raiseProfile) {
super(root, 6);
this.booleanCastNode = booleanCastNode;
this.getIntegerConstantNode = getIntegerConstantNode;
this.modeCallNode = modeCallNode;
this.raiseProfile = raiseProfile;
}
@Override
public DynamicObject executeDynamicObject1(VirtualFrame frameValue, Object valueValue, DynamicObject selfValue, Object digitsValue) {
if (valueValue instanceof BigDecimalType) {
BigDecimalType valueValue_ = (BigDecimalType) valueValue;
if ((valueValue_ == BigDecimalType.NAN)) {
return root.createNaN(frameValue, valueValue_, selfValue, digitsValue, this.booleanCastNode, this.getIntegerConstantNode, this.modeCallNode, this.raiseProfile);
}
}
return getNext().executeDynamicObject1(frameValue, valueValue, selfValue, digitsValue);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object valueValue, Object selfValue, Object digitsValue) {
if (valueValue instanceof BigDecimalType && selfValue instanceof DynamicObject) {
BigDecimalType valueValue_ = (BigDecimalType) valueValue;
DynamicObject selfValue_ = (DynamicObject) selfValue;
if ((valueValue_ == BigDecimalType.NAN)) {
return root.createNaN(frameValue, valueValue_, selfValue_, digitsValue, this.booleanCastNode, this.getIntegerConstantNode, this.modeCallNode, this.raiseProfile);
}
}
return getNext().executeDynamicObject_(frameValue, valueValue, selfValue, digitsValue);
}
static BaseNode_ create(CreateBigDecimalNodeGen root, BooleanCastNode booleanCastNode, GetIntegerConstantNode getIntegerConstantNode, CallDispatchHeadNode modeCallNode, ConditionProfile raiseProfile) {
return new CreateNaNNode_(root, booleanCastNode, getIntegerConstantNode, modeCallNode, raiseProfile);
}
}
@GeneratedBy(methodName = "createNegativeZero(VirtualFrame, BigDecimalType, DynamicObject, Object)", value = CreateBigDecimalNode.class)
private static final class CreateNegativeZeroNode_ extends BaseNode_ {
CreateNegativeZeroNode_(CreateBigDecimalNodeGen root) {
super(root, 7);
}
@Override
public DynamicObject executeDynamicObject1(VirtualFrame frameValue, Object valueValue, DynamicObject selfValue, Object digitsValue) {
if (valueValue instanceof BigDecimalType) {
BigDecimalType valueValue_ = (BigDecimalType) valueValue;
if ((valueValue_ == BigDecimalType.NEGATIVE_ZERO)) {
return root.createNegativeZero(frameValue, valueValue_, selfValue, digitsValue);
}
}
return getNext().executeDynamicObject1(frameValue, valueValue, selfValue, digitsValue);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object valueValue, Object selfValue, Object digitsValue) {
if (valueValue instanceof BigDecimalType && selfValue instanceof DynamicObject) {
BigDecimalType valueValue_ = (BigDecimalType) valueValue;
DynamicObject selfValue_ = (DynamicObject) selfValue;
if ((valueValue_ == BigDecimalType.NEGATIVE_ZERO)) {
return root.createNegativeZero(frameValue, valueValue_, selfValue_, digitsValue);
}
}
return getNext().executeDynamicObject_(frameValue, valueValue, selfValue, digitsValue);
}
static BaseNode_ create(CreateBigDecimalNodeGen root) {
return new CreateNegativeZeroNode_(root);
}
}
@GeneratedBy(methodName = "create(VirtualFrame, BigDecimal, DynamicObject, NotProvided)", value = CreateBigDecimalNode.class)
private static final class Create4Node_ extends BaseNode_ {
Create4Node_(CreateBigDecimalNodeGen root) {
super(root, 8);
}
@Override
public DynamicObject executeDynamicObject1(VirtualFrame frameValue, Object valueValue, DynamicObject selfValue, Object digitsValue) {
if (valueValue instanceof BigDecimal && digitsValue instanceof NotProvided) {
BigDecimal valueValue_ = (BigDecimal) valueValue;
NotProvided digitsValue_ = (NotProvided) digitsValue;
return root.create(frameValue, valueValue_, selfValue, digitsValue_);
}
return getNext().executeDynamicObject1(frameValue, valueValue, selfValue, digitsValue);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object valueValue, Object selfValue, Object digitsValue) {
if (valueValue instanceof BigDecimal && selfValue instanceof DynamicObject && digitsValue instanceof NotProvided) {
BigDecimal valueValue_ = (BigDecimal) valueValue;
DynamicObject selfValue_ = (DynamicObject) selfValue;
NotProvided digitsValue_ = (NotProvided) digitsValue;
return root.create(frameValue, valueValue_, selfValue_, digitsValue_);
}
return getNext().executeDynamicObject_(frameValue, valueValue, selfValue, digitsValue);
}
static BaseNode_ create(CreateBigDecimalNodeGen root) {
return new Create4Node_(root);
}
}
@GeneratedBy(methodName = "create(VirtualFrame, BigDecimal, DynamicObject, int)", value = CreateBigDecimalNode.class)
private static final class Create5Node_ extends BaseNode_ {
private final Class> digitsImplicitType;
Create5Node_(CreateBigDecimalNodeGen root, Object digitsValue) {
super(root, 9);
this.digitsImplicitType = RubyTypesGen.getImplicitIntegerClass(digitsValue);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.digitsImplicitType == ((Create5Node_) other).digitsImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject0(frameValue);
}
@Override
public DynamicObject executeDynamicObject0(VirtualFrame frameValue) {
BigDecimal valueValue_;
try {
valueValue_ = expectBigDecimal(root.value_.execute(frameValue));
} catch (UnexpectedResultException ex) {
Object selfValue = root.self_.execute(frameValue);
Object digitsValue = executeDigits_(frameValue);
return getNext().executeDynamicObject_(frameValue, ex.getResult(), selfValue, digitsValue);
}
DynamicObject selfValue_;
try {
selfValue_ = root.self_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object digitsValue = executeDigits_(frameValue);
return getNext().executeDynamicObject_(frameValue, valueValue_, ex.getResult(), digitsValue);
}
int digitsValue_;
try {
if (digitsImplicitType == int.class) {
digitsValue_ = root.digits_.executeInteger(frameValue);
} else {
Object digitsValue__ = executeDigits_(frameValue);
digitsValue_ = RubyTypesGen.expectImplicitInteger(digitsValue__, digitsImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeDynamicObject_(frameValue, valueValue_, selfValue_, ex.getResult());
}
return root.create(frameValue, valueValue_, selfValue_, digitsValue_);
}
@Override
public DynamicObject executeDynamicObject1(VirtualFrame frameValue, Object valueValue, DynamicObject selfValue, Object digitsValue) {
if (valueValue instanceof BigDecimal && RubyTypesGen.isImplicitInteger(digitsValue, digitsImplicitType)) {
BigDecimal valueValue_ = (BigDecimal) valueValue;
int digitsValue_ = RubyTypesGen.asImplicitInteger(digitsValue, digitsImplicitType);
return root.create(frameValue, valueValue_, selfValue, digitsValue_);
}
return getNext().executeDynamicObject1(frameValue, valueValue, selfValue, digitsValue);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object valueValue, Object selfValue, Object digitsValue) {
if (valueValue instanceof BigDecimal && selfValue instanceof DynamicObject && RubyTypesGen.isImplicitInteger(digitsValue, digitsImplicitType)) {
BigDecimal valueValue_ = (BigDecimal) valueValue;
DynamicObject selfValue_ = (DynamicObject) selfValue;
int digitsValue_ = RubyTypesGen.asImplicitInteger(digitsValue, digitsImplicitType);
return root.create(frameValue, valueValue_, selfValue_, digitsValue_);
}
return getNext().executeDynamicObject_(frameValue, valueValue, selfValue, digitsValue);
}
static BaseNode_ create(CreateBigDecimalNodeGen root, Object digitsValue) {
return new Create5Node_(root, digitsValue);
}
}
@GeneratedBy(methodName = "createBignum(VirtualFrame, DynamicObject, DynamicObject, NotProvided)", value = CreateBigDecimalNode.class)
private static final class CreateBignum0Node_ extends BaseNode_ {
CreateBignum0Node_(CreateBigDecimalNodeGen root) {
super(root, 10);
}
@Override
public DynamicObject executeDynamicObject1(VirtualFrame frameValue, Object valueValue, DynamicObject selfValue, Object digitsValue) {
if (valueValue instanceof DynamicObject && digitsValue instanceof NotProvided) {
DynamicObject valueValue_ = (DynamicObject) valueValue;
NotProvided digitsValue_ = (NotProvided) digitsValue;
if ((RubyGuards.isRubyBignum(valueValue_))) {
return root.createBignum(frameValue, valueValue_, selfValue, digitsValue_);
}
}
return getNext().executeDynamicObject1(frameValue, valueValue, selfValue, digitsValue);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object valueValue, Object selfValue, Object digitsValue) {
if (valueValue instanceof DynamicObject && selfValue instanceof DynamicObject && digitsValue instanceof NotProvided) {
DynamicObject valueValue_ = (DynamicObject) valueValue;
DynamicObject selfValue_ = (DynamicObject) selfValue;
NotProvided digitsValue_ = (NotProvided) digitsValue;
if ((RubyGuards.isRubyBignum(valueValue_))) {
return root.createBignum(frameValue, valueValue_, selfValue_, digitsValue_);
}
}
return getNext().executeDynamicObject_(frameValue, valueValue, selfValue, digitsValue);
}
static BaseNode_ create(CreateBigDecimalNodeGen root) {
return new CreateBignum0Node_(root);
}
}
@GeneratedBy(methodName = "createBignum(VirtualFrame, DynamicObject, DynamicObject, int)", value = CreateBigDecimalNode.class)
private static final class CreateBignum1Node_ extends BaseNode_ {
private final Class> digitsImplicitType;
CreateBignum1Node_(CreateBigDecimalNodeGen root, Object digitsValue) {
super(root, 11);
this.digitsImplicitType = RubyTypesGen.getImplicitIntegerClass(digitsValue);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.digitsImplicitType == ((CreateBignum1Node_) other).digitsImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject0(frameValue);
}
@Override
public DynamicObject executeDynamicObject0(VirtualFrame frameValue) {
DynamicObject valueValue_;
try {
valueValue_ = root.value_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object selfValue = root.self_.execute(frameValue);
Object digitsValue = executeDigits_(frameValue);
return getNext().executeDynamicObject_(frameValue, ex.getResult(), selfValue, digitsValue);
}
DynamicObject selfValue_;
try {
selfValue_ = root.self_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object digitsValue = executeDigits_(frameValue);
return getNext().executeDynamicObject_(frameValue, valueValue_, ex.getResult(), digitsValue);
}
int digitsValue_;
try {
if (digitsImplicitType == int.class) {
digitsValue_ = root.digits_.executeInteger(frameValue);
} else {
Object digitsValue__ = executeDigits_(frameValue);
digitsValue_ = RubyTypesGen.expectImplicitInteger(digitsValue__, digitsImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeDynamicObject_(frameValue, valueValue_, selfValue_, ex.getResult());
}
if ((RubyGuards.isRubyBignum(valueValue_))) {
return root.createBignum(frameValue, valueValue_, selfValue_, digitsValue_);
}
return getNext().executeDynamicObject_(frameValue, valueValue_, selfValue_, digitsValue_);
}
@Override
public DynamicObject executeDynamicObject1(VirtualFrame frameValue, Object valueValue, DynamicObject selfValue, Object digitsValue) {
if (valueValue instanceof DynamicObject && RubyTypesGen.isImplicitInteger(digitsValue, digitsImplicitType)) {
DynamicObject valueValue_ = (DynamicObject) valueValue;
int digitsValue_ = RubyTypesGen.asImplicitInteger(digitsValue, digitsImplicitType);
if ((RubyGuards.isRubyBignum(valueValue_))) {
return root.createBignum(frameValue, valueValue_, selfValue, digitsValue_);
}
}
return getNext().executeDynamicObject1(frameValue, valueValue, selfValue, digitsValue);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object valueValue, Object selfValue, Object digitsValue) {
if (valueValue instanceof DynamicObject && selfValue instanceof DynamicObject && RubyTypesGen.isImplicitInteger(digitsValue, digitsImplicitType)) {
DynamicObject valueValue_ = (DynamicObject) valueValue;
DynamicObject selfValue_ = (DynamicObject) selfValue;
int digitsValue_ = RubyTypesGen.asImplicitInteger(digitsValue, digitsImplicitType);
if ((RubyGuards.isRubyBignum(valueValue_))) {
return root.createBignum(frameValue, valueValue_, selfValue_, digitsValue_);
}
}
return getNext().executeDynamicObject_(frameValue, valueValue, selfValue, digitsValue);
}
static BaseNode_ create(CreateBigDecimalNodeGen root, Object digitsValue) {
return new CreateBignum1Node_(root, digitsValue);
}
}
@GeneratedBy(methodName = "createBigDecimal(VirtualFrame, DynamicObject, DynamicObject, NotProvided)", value = CreateBigDecimalNode.class)
private static final class CreateBigDecimal0Node_ extends BaseNode_ {
CreateBigDecimal0Node_(CreateBigDecimalNodeGen root) {
super(root, 12);
}
@Override
public DynamicObject executeDynamicObject1(VirtualFrame frameValue, Object valueValue, DynamicObject selfValue, Object digitsValue) {
if (valueValue instanceof DynamicObject && digitsValue instanceof NotProvided) {
DynamicObject valueValue_ = (DynamicObject) valueValue;
NotProvided digitsValue_ = (NotProvided) digitsValue;
if ((RubyGuards.isRubyBigDecimal(valueValue_))) {
return root.createBigDecimal(frameValue, valueValue_, selfValue, digitsValue_);
}
}
return getNext().executeDynamicObject1(frameValue, valueValue, selfValue, digitsValue);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object valueValue, Object selfValue, Object digitsValue) {
if (valueValue instanceof DynamicObject && selfValue instanceof DynamicObject && digitsValue instanceof NotProvided) {
DynamicObject valueValue_ = (DynamicObject) valueValue;
DynamicObject selfValue_ = (DynamicObject) selfValue;
NotProvided digitsValue_ = (NotProvided) digitsValue;
if ((RubyGuards.isRubyBigDecimal(valueValue_))) {
return root.createBigDecimal(frameValue, valueValue_, selfValue_, digitsValue_);
}
}
return getNext().executeDynamicObject_(frameValue, valueValue, selfValue, digitsValue);
}
static BaseNode_ create(CreateBigDecimalNodeGen root) {
return new CreateBigDecimal0Node_(root);
}
}
@GeneratedBy(methodName = "createBigDecimal(VirtualFrame, DynamicObject, DynamicObject, int)", value = CreateBigDecimalNode.class)
private static final class CreateBigDecimal1Node_ extends BaseNode_ {
private final Class> digitsImplicitType;
CreateBigDecimal1Node_(CreateBigDecimalNodeGen root, Object digitsValue) {
super(root, 13);
this.digitsImplicitType = RubyTypesGen.getImplicitIntegerClass(digitsValue);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.digitsImplicitType == ((CreateBigDecimal1Node_) other).digitsImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject0(frameValue);
}
@Override
public DynamicObject executeDynamicObject0(VirtualFrame frameValue) {
DynamicObject valueValue_;
try {
valueValue_ = root.value_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object selfValue = root.self_.execute(frameValue);
Object digitsValue = executeDigits_(frameValue);
return getNext().executeDynamicObject_(frameValue, ex.getResult(), selfValue, digitsValue);
}
DynamicObject selfValue_;
try {
selfValue_ = root.self_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object digitsValue = executeDigits_(frameValue);
return getNext().executeDynamicObject_(frameValue, valueValue_, ex.getResult(), digitsValue);
}
int digitsValue_;
try {
if (digitsImplicitType == int.class) {
digitsValue_ = root.digits_.executeInteger(frameValue);
} else {
Object digitsValue__ = executeDigits_(frameValue);
digitsValue_ = RubyTypesGen.expectImplicitInteger(digitsValue__, digitsImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeDynamicObject_(frameValue, valueValue_, selfValue_, ex.getResult());
}
if ((RubyGuards.isRubyBigDecimal(valueValue_))) {
return root.createBigDecimal(frameValue, valueValue_, selfValue_, digitsValue_);
}
return getNext().executeDynamicObject_(frameValue, valueValue_, selfValue_, digitsValue_);
}
@Override
public DynamicObject executeDynamicObject1(VirtualFrame frameValue, Object valueValue, DynamicObject selfValue, Object digitsValue) {
if (valueValue instanceof DynamicObject && RubyTypesGen.isImplicitInteger(digitsValue, digitsImplicitType)) {
DynamicObject valueValue_ = (DynamicObject) valueValue;
int digitsValue_ = RubyTypesGen.asImplicitInteger(digitsValue, digitsImplicitType);
if ((RubyGuards.isRubyBigDecimal(valueValue_))) {
return root.createBigDecimal(frameValue, valueValue_, selfValue, digitsValue_);
}
}
return getNext().executeDynamicObject1(frameValue, valueValue, selfValue, digitsValue);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object valueValue, Object selfValue, Object digitsValue) {
if (valueValue instanceof DynamicObject && selfValue instanceof DynamicObject && RubyTypesGen.isImplicitInteger(digitsValue, digitsImplicitType)) {
DynamicObject valueValue_ = (DynamicObject) valueValue;
DynamicObject selfValue_ = (DynamicObject) selfValue;
int digitsValue_ = RubyTypesGen.asImplicitInteger(digitsValue, digitsImplicitType);
if ((RubyGuards.isRubyBigDecimal(valueValue_))) {
return root.createBigDecimal(frameValue, valueValue_, selfValue_, digitsValue_);
}
}
return getNext().executeDynamicObject_(frameValue, valueValue, selfValue, digitsValue);
}
static BaseNode_ create(CreateBigDecimalNodeGen root, Object digitsValue) {
return new CreateBigDecimal1Node_(root, digitsValue);
}
}
@GeneratedBy(methodName = "createString(VirtualFrame, DynamicObject, DynamicObject, NotProvided)", value = CreateBigDecimalNode.class)
private static final class CreateString0Node_ extends BaseNode_ {
CreateString0Node_(CreateBigDecimalNodeGen root) {
super(root, 14);
}
@Override
public DynamicObject executeDynamicObject1(VirtualFrame frameValue, Object valueValue, DynamicObject selfValue, Object digitsValue) {
if (valueValue instanceof DynamicObject && digitsValue instanceof NotProvided) {
DynamicObject valueValue_ = (DynamicObject) valueValue;
NotProvided digitsValue_ = (NotProvided) digitsValue;
if ((RubyGuards.isRubyString(valueValue_))) {
return root.createString(frameValue, valueValue_, selfValue, digitsValue_);
}
}
return getNext().executeDynamicObject1(frameValue, valueValue, selfValue, digitsValue);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object valueValue, Object selfValue, Object digitsValue) {
if (valueValue instanceof DynamicObject && selfValue instanceof DynamicObject && digitsValue instanceof NotProvided) {
DynamicObject valueValue_ = (DynamicObject) valueValue;
DynamicObject selfValue_ = (DynamicObject) selfValue;
NotProvided digitsValue_ = (NotProvided) digitsValue;
if ((RubyGuards.isRubyString(valueValue_))) {
return root.createString(frameValue, valueValue_, selfValue_, digitsValue_);
}
}
return getNext().executeDynamicObject_(frameValue, valueValue, selfValue, digitsValue);
}
static BaseNode_ create(CreateBigDecimalNodeGen root) {
return new CreateString0Node_(root);
}
}
@GeneratedBy(methodName = "createString(VirtualFrame, DynamicObject, DynamicObject, int)", value = CreateBigDecimalNode.class)
private static final class CreateString1Node_ extends BaseNode_ {
private final Class> digitsImplicitType;
CreateString1Node_(CreateBigDecimalNodeGen root, Object digitsValue) {
super(root, 15);
this.digitsImplicitType = RubyTypesGen.getImplicitIntegerClass(digitsValue);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.digitsImplicitType == ((CreateString1Node_) other).digitsImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject0(frameValue);
}
@Override
public DynamicObject executeDynamicObject0(VirtualFrame frameValue) {
DynamicObject valueValue_;
try {
valueValue_ = root.value_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object selfValue = root.self_.execute(frameValue);
Object digitsValue = executeDigits_(frameValue);
return getNext().executeDynamicObject_(frameValue, ex.getResult(), selfValue, digitsValue);
}
DynamicObject selfValue_;
try {
selfValue_ = root.self_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object digitsValue = executeDigits_(frameValue);
return getNext().executeDynamicObject_(frameValue, valueValue_, ex.getResult(), digitsValue);
}
int digitsValue_;
try {
if (digitsImplicitType == int.class) {
digitsValue_ = root.digits_.executeInteger(frameValue);
} else {
Object digitsValue__ = executeDigits_(frameValue);
digitsValue_ = RubyTypesGen.expectImplicitInteger(digitsValue__, digitsImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeDynamicObject_(frameValue, valueValue_, selfValue_, ex.getResult());
}
if ((RubyGuards.isRubyString(valueValue_))) {
return root.createString(frameValue, valueValue_, selfValue_, digitsValue_);
}
return getNext().executeDynamicObject_(frameValue, valueValue_, selfValue_, digitsValue_);
}
@Override
public DynamicObject executeDynamicObject1(VirtualFrame frameValue, Object valueValue, DynamicObject selfValue, Object digitsValue) {
if (valueValue instanceof DynamicObject && RubyTypesGen.isImplicitInteger(digitsValue, digitsImplicitType)) {
DynamicObject valueValue_ = (DynamicObject) valueValue;
int digitsValue_ = RubyTypesGen.asImplicitInteger(digitsValue, digitsImplicitType);
if ((RubyGuards.isRubyString(valueValue_))) {
return root.createString(frameValue, valueValue_, selfValue, digitsValue_);
}
}
return getNext().executeDynamicObject1(frameValue, valueValue, selfValue, digitsValue);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object valueValue, Object selfValue, Object digitsValue) {
if (valueValue instanceof DynamicObject && selfValue instanceof DynamicObject && RubyTypesGen.isImplicitInteger(digitsValue, digitsImplicitType)) {
DynamicObject valueValue_ = (DynamicObject) valueValue;
DynamicObject selfValue_ = (DynamicObject) selfValue;
int digitsValue_ = RubyTypesGen.asImplicitInteger(digitsValue, digitsImplicitType);
if ((RubyGuards.isRubyString(valueValue_))) {
return root.createString(frameValue, valueValue_, selfValue_, digitsValue_);
}
}
return getNext().executeDynamicObject_(frameValue, valueValue, selfValue, digitsValue);
}
static BaseNode_ create(CreateBigDecimalNodeGen root, Object digitsValue) {
return new CreateString1Node_(root, digitsValue);
}
}
@GeneratedBy(methodName = "create(VirtualFrame, DynamicObject, DynamicObject, int, BigDecimalCastNode, ConditionProfile)", value = CreateBigDecimalNode.class)
private static final class Create6Node_ extends BaseNode_ {
@Child private BigDecimalCastNode bigDecimalCastNode;
private final ConditionProfile nilProfile;
private final Class> digitsImplicitType;
Create6Node_(CreateBigDecimalNodeGen root, Object digitsValue, BigDecimalCastNode bigDecimalCastNode, ConditionProfile nilProfile) {
super(root, 16);
this.digitsImplicitType = RubyTypesGen.getImplicitIntegerClass(digitsValue);
this.bigDecimalCastNode = bigDecimalCastNode;
this.nilProfile = nilProfile;
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.digitsImplicitType == ((Create6Node_) other).digitsImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject0(frameValue);
}
@Override
public DynamicObject executeDynamicObject0(VirtualFrame frameValue) {
DynamicObject valueValue_;
try {
valueValue_ = root.value_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object selfValue = root.self_.execute(frameValue);
Object digitsValue = executeDigits_(frameValue);
return getNext().executeDynamicObject_(frameValue, ex.getResult(), selfValue, digitsValue);
}
DynamicObject selfValue_;
try {
selfValue_ = root.self_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object digitsValue = executeDigits_(frameValue);
return getNext().executeDynamicObject_(frameValue, valueValue_, ex.getResult(), digitsValue);
}
int digitsValue_;
try {
if (digitsImplicitType == int.class) {
digitsValue_ = root.digits_.executeInteger(frameValue);
} else {
Object digitsValue__ = executeDigits_(frameValue);
digitsValue_ = RubyTypesGen.expectImplicitInteger(digitsValue__, digitsImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeDynamicObject_(frameValue, valueValue_, selfValue_, ex.getResult());
}
if ((!(RubyGuards.isRubyBignum(valueValue_))) && (!(RubyGuards.isRubyBigDecimal(valueValue_))) && (!(RubyGuards.isRubyString(valueValue_)))) {
return root.create(frameValue, valueValue_, selfValue_, digitsValue_, this.bigDecimalCastNode, this.nilProfile);
}
return getNext().executeDynamicObject_(frameValue, valueValue_, selfValue_, digitsValue_);
}
@Override
public DynamicObject executeDynamicObject1(VirtualFrame frameValue, Object valueValue, DynamicObject selfValue, Object digitsValue) {
if (valueValue instanceof DynamicObject && RubyTypesGen.isImplicitInteger(digitsValue, digitsImplicitType)) {
DynamicObject valueValue_ = (DynamicObject) valueValue;
int digitsValue_ = RubyTypesGen.asImplicitInteger(digitsValue, digitsImplicitType);
if ((!(RubyGuards.isRubyBignum(valueValue_))) && (!(RubyGuards.isRubyBigDecimal(valueValue_))) && (!(RubyGuards.isRubyString(valueValue_)))) {
return root.create(frameValue, valueValue_, selfValue, digitsValue_, this.bigDecimalCastNode, this.nilProfile);
}
}
return getNext().executeDynamicObject1(frameValue, valueValue, selfValue, digitsValue);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object valueValue, Object selfValue, Object digitsValue) {
if (valueValue instanceof DynamicObject && selfValue instanceof DynamicObject && RubyTypesGen.isImplicitInteger(digitsValue, digitsImplicitType)) {
DynamicObject valueValue_ = (DynamicObject) valueValue;
DynamicObject selfValue_ = (DynamicObject) selfValue;
int digitsValue_ = RubyTypesGen.asImplicitInteger(digitsValue, digitsImplicitType);
if ((!(RubyGuards.isRubyBignum(valueValue_))) && (!(RubyGuards.isRubyBigDecimal(valueValue_))) && (!(RubyGuards.isRubyString(valueValue_)))) {
return root.create(frameValue, valueValue_, selfValue_, digitsValue_, this.bigDecimalCastNode, this.nilProfile);
}
}
return getNext().executeDynamicObject_(frameValue, valueValue, selfValue, digitsValue);
}
static BaseNode_ create(CreateBigDecimalNodeGen root, Object digitsValue, BigDecimalCastNode bigDecimalCastNode, ConditionProfile nilProfile) {
return new Create6Node_(root, digitsValue, bigDecimalCastNode, nilProfile);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy