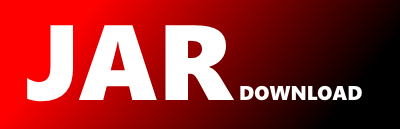
org.jruby.truffle.stdlib.psych.PsychEmitterNodesFactory Maven / Gradle / Ivy
The newest version!
// CheckStyle: start generated
package org.jruby.truffle.stdlib.psych;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NodeFactory;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.internal.SpecializationNode;
import com.oracle.truffle.api.dsl.internal.SpecializedNode;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.nodes.UnexpectedResultException;
import com.oracle.truffle.api.object.DynamicObject;
import java.util.Arrays;
import java.util.List;
import org.jruby.truffle.builtins.CoreMethodArrayArgumentsNode;
import org.jruby.truffle.language.NotProvided;
import org.jruby.truffle.language.RubyGuards;
import org.jruby.truffle.language.RubyNode;
import org.jruby.truffle.language.RubyTypesGen;
import org.jruby.truffle.language.dispatch.CallDispatchHeadNode;
import org.jruby.truffle.stdlib.psych.PsychEmitterNodes.AliasNode;
import org.jruby.truffle.stdlib.psych.PsychEmitterNodes.AllocateNode;
import org.jruby.truffle.stdlib.psych.PsychEmitterNodes.CanonicalNode;
import org.jruby.truffle.stdlib.psych.PsychEmitterNodes.EndDocumentNode;
import org.jruby.truffle.stdlib.psych.PsychEmitterNodes.EndMappingNode;
import org.jruby.truffle.stdlib.psych.PsychEmitterNodes.EndSequenceNode;
import org.jruby.truffle.stdlib.psych.PsychEmitterNodes.EndStreamNode;
import org.jruby.truffle.stdlib.psych.PsychEmitterNodes.IndentationNode;
import org.jruby.truffle.stdlib.psych.PsychEmitterNodes.InitializeNode;
import org.jruby.truffle.stdlib.psych.PsychEmitterNodes.LineWidthNode;
import org.jruby.truffle.stdlib.psych.PsychEmitterNodes.ScalarNode;
import org.jruby.truffle.stdlib.psych.PsychEmitterNodes.SetCanonicalNode;
import org.jruby.truffle.stdlib.psych.PsychEmitterNodes.SetIndentationNode;
import org.jruby.truffle.stdlib.psych.PsychEmitterNodes.SetLineWidthNode;
import org.jruby.truffle.stdlib.psych.PsychEmitterNodes.StartDocumentNode;
import org.jruby.truffle.stdlib.psych.PsychEmitterNodes.StartMappingNode;
import org.jruby.truffle.stdlib.psych.PsychEmitterNodes.StartSequenceNode;
import org.jruby.truffle.stdlib.psych.PsychEmitterNodes.StartStreamNode;
@GeneratedBy(PsychEmitterNodes.class)
public final class PsychEmitterNodesFactory {
public static List> getFactories() {
return Arrays.asList(AllocateNodeFactory.getInstance(), InitializeNodeFactory.getInstance(), StartStreamNodeFactory.getInstance(), EndStreamNodeFactory.getInstance(), StartDocumentNodeFactory.getInstance(), EndDocumentNodeFactory.getInstance(), ScalarNodeFactory.getInstance(), StartSequenceNodeFactory.getInstance(), EndSequenceNodeFactory.getInstance(), StartMappingNodeFactory.getInstance(), EndMappingNodeFactory.getInstance(), AliasNodeFactory.getInstance(), SetCanonicalNodeFactory.getInstance(), CanonicalNodeFactory.getInstance(), SetIndentationNodeFactory.getInstance(), IndentationNodeFactory.getInstance(), SetLineWidthNodeFactory.getInstance(), LineWidthNodeFactory.getInstance());
}
@GeneratedBy(AllocateNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class AllocateNodeFactory implements NodeFactory {
private static AllocateNodeFactory allocateNodeFactoryInstance;
private AllocateNodeFactory() {
}
@Override
public Class getNodeClass() {
return AllocateNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public AllocateNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (allocateNodeFactoryInstance == null) {
allocateNodeFactoryInstance = new AllocateNodeFactory();
}
return allocateNodeFactoryInstance;
}
public static AllocateNode create(RubyNode[] arguments) {
return new AllocateNodeGen(arguments);
}
@GeneratedBy(AllocateNode.class)
public static final class AllocateNodeGen extends AllocateNode {
@Child private RubyNode arguments0_;
@CompilationFinal private boolean seenUnsupported0;
private AllocateNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(ex.getResult());
}
return this.allocate(arguments0Value_);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
private UnsupportedSpecializationException unsupported(Object arguments0Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_}, arguments0Value);
}
}
}
@GeneratedBy(InitializeNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class InitializeNodeFactory implements NodeFactory {
private static InitializeNodeFactory initializeNodeFactoryInstance;
private InitializeNodeFactory() {
}
@Override
public Class getNodeClass() {
return InitializeNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public InitializeNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (initializeNodeFactoryInstance == null) {
initializeNodeFactoryInstance = new InitializeNodeFactory();
}
return initializeNodeFactoryInstance;
}
public static InitializeNode create(RubyNode[] arguments) {
return new InitializeNodeGen(arguments);
}
@GeneratedBy(InitializeNode.class)
public static final class InitializeNodeGen extends InitializeNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@Child private RubyNode arguments2_;
@Child private BaseNode_ specialization_;
private InitializeNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.arguments2_ = arguments != null && 2 < arguments.length ? arguments[2] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(InitializeNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected InitializeNodeGen root;
BaseNode_(InitializeNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (InitializeNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_, root.arguments1_, root.arguments2_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return this.executeDynamicObject_((VirtualFrame) frameValue, arguments0Value, arguments1Value, arguments2Value);
}
public abstract DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = root.arguments1_.execute(frameValue);
Object arguments2Value_ = root.arguments2_.execute(frameValue);
return executeDynamicObject_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof DynamicObject && arguments1Value instanceof DynamicObject) {
if (arguments2Value instanceof NotProvided) {
return Initialize0Node_.create(root);
}
if (arguments2Value instanceof DynamicObject) {
CallDispatchHeadNode lineWidthCallNode2 = (CallDispatchHeadNode.createMethodCall());
CallDispatchHeadNode canonicalCallNode2 = (CallDispatchHeadNode.createMethodCall());
CallDispatchHeadNode indentationCallNode2 = (CallDispatchHeadNode.createMethodCall());
return Initialize1Node_.create(root, lineWidthCallNode2, canonicalCallNode2, indentationCallNode2);
}
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(InitializeNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(InitializeNodeGen root) {
super(root, 2147483647);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return (DynamicObject) uninitialized(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(InitializeNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(InitializeNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(InitializeNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value, arguments1Value, arguments2Value));
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(InitializeNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "initialize(DynamicObject, DynamicObject, NotProvided)", value = InitializeNode.class)
private static final class Initialize0Node_ extends BaseNode_ {
Initialize0Node_(InitializeNodeGen root) {
super(root, 1);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof DynamicObject && arguments1Value instanceof DynamicObject && arguments2Value instanceof NotProvided) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
NotProvided arguments2Value_ = (NotProvided) arguments2Value;
return root.initialize(arguments0Value_, arguments1Value_, arguments2Value_);
}
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(InitializeNodeGen root) {
return new Initialize0Node_(root);
}
}
@GeneratedBy(methodName = "initialize(VirtualFrame, DynamicObject, DynamicObject, DynamicObject, CallDispatchHeadNode, CallDispatchHeadNode, CallDispatchHeadNode)", value = InitializeNode.class)
private static final class Initialize1Node_ extends BaseNode_ {
@Child private CallDispatchHeadNode lineWidthCallNode;
@Child private CallDispatchHeadNode canonicalCallNode;
@Child private CallDispatchHeadNode indentationCallNode;
Initialize1Node_(InitializeNodeGen root, CallDispatchHeadNode lineWidthCallNode, CallDispatchHeadNode canonicalCallNode, CallDispatchHeadNode indentationCallNode) {
super(root, 2);
this.lineWidthCallNode = lineWidthCallNode;
this.canonicalCallNode = canonicalCallNode;
this.indentationCallNode = indentationCallNode;
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof DynamicObject && arguments1Value instanceof DynamicObject && arguments2Value instanceof DynamicObject) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
DynamicObject arguments2Value_ = (DynamicObject) arguments2Value;
return root.initialize(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, this.lineWidthCallNode, this.canonicalCallNode, this.indentationCallNode);
}
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(InitializeNodeGen root, CallDispatchHeadNode lineWidthCallNode, CallDispatchHeadNode canonicalCallNode, CallDispatchHeadNode indentationCallNode) {
return new Initialize1Node_(root, lineWidthCallNode, canonicalCallNode, indentationCallNode);
}
}
}
}
@GeneratedBy(StartStreamNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class StartStreamNodeFactory implements NodeFactory {
private static StartStreamNodeFactory startStreamNodeFactoryInstance;
private StartStreamNodeFactory() {
}
@Override
public Class getNodeClass() {
return StartStreamNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public StartStreamNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (startStreamNodeFactoryInstance == null) {
startStreamNodeFactoryInstance = new StartStreamNodeFactory();
}
return startStreamNodeFactoryInstance;
}
public static StartStreamNode create(RubyNode[] arguments) {
return new StartStreamNodeGen(arguments);
}
@GeneratedBy(StartStreamNode.class)
public static final class StartStreamNodeGen extends StartStreamNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@CompilationFinal private Class> arguments1Type_;
@Child private BaseNode_ specialization_;
private StartStreamNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
return specialization_.executeDynamicObject(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(StartStreamNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected StartStreamNodeGen root;
BaseNode_(StartStreamNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (StartStreamNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_, root.arguments1_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value, Object arguments1Value) {
return this.executeDynamicObject_((VirtualFrame) frameValue, arguments0Value, arguments1Value);
}
public abstract DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = executeArguments1_(frameValue);
return executeDynamicObject_(frameValue, arguments0Value_, arguments1Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
return (DynamicObject) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value)) {
return StartStreamNode_.create(root, arguments1Value);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments1_(Frame frameValue) {
Class> arguments1Type_ = root.arguments1Type_;
try {
if (arguments1Type_ == int.class) {
return root.arguments1_.executeInteger((VirtualFrame) frameValue);
} else if (arguments1Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments1_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments1Type_ = _type;
}
} else {
return root.arguments1_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments1Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(StartStreamNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(StartStreamNodeGen root) {
super(root, 2147483647);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
return (DynamicObject) uninitialized(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(StartStreamNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(StartStreamNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(StartStreamNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value, arguments1Value));
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(StartStreamNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "startStream(DynamicObject, int)", value = StartStreamNode.class)
private static final class StartStreamNode_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
StartStreamNode_(StartStreamNodeGen root, Object arguments1Value) {
super(root, 1);
this.arguments1ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments1Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((StartStreamNode_) other).arguments1ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject(frameValue);
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
return getNext().executeDynamicObject_(frameValue, ex.getResult(), arguments1Value);
}
int arguments1Value_;
try {
if (arguments1ImplicitType == int.class) {
arguments1Value_ = root.arguments1_.executeInteger(frameValue);
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitInteger(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeDynamicObject_(frameValue, arguments0Value_, ex.getResult());
}
return root.startStream(arguments0Value_, arguments1Value_);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value, arguments1ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
int arguments1Value_ = RubyTypesGen.asImplicitInteger(arguments1Value, arguments1ImplicitType);
return root.startStream(arguments0Value_, arguments1Value_);
}
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(StartStreamNodeGen root, Object arguments1Value) {
return new StartStreamNode_(root, arguments1Value);
}
}
}
}
@GeneratedBy(EndStreamNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class EndStreamNodeFactory implements NodeFactory {
private static EndStreamNodeFactory endStreamNodeFactoryInstance;
private EndStreamNodeFactory() {
}
@Override
public Class getNodeClass() {
return EndStreamNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public EndStreamNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (endStreamNodeFactoryInstance == null) {
endStreamNodeFactoryInstance = new EndStreamNodeFactory();
}
return endStreamNodeFactoryInstance;
}
public static EndStreamNode create(RubyNode[] arguments) {
return new EndStreamNodeGen(arguments);
}
@GeneratedBy(EndStreamNode.class)
public static final class EndStreamNodeGen extends EndStreamNode {
@Child private RubyNode arguments0_;
@CompilationFinal private boolean seenUnsupported0;
private EndStreamNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(ex.getResult());
}
return this.endStream(arguments0Value_);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
private UnsupportedSpecializationException unsupported(Object arguments0Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_}, arguments0Value);
}
}
}
@GeneratedBy(StartDocumentNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class StartDocumentNodeFactory implements NodeFactory {
private static StartDocumentNodeFactory startDocumentNodeFactoryInstance;
private StartDocumentNodeFactory() {
}
@Override
public Class getNodeClass() {
return StartDocumentNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class, RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public StartDocumentNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (startDocumentNodeFactoryInstance == null) {
startDocumentNodeFactoryInstance = new StartDocumentNodeFactory();
}
return startDocumentNodeFactoryInstance;
}
public static StartDocumentNode create(RubyNode[] arguments) {
return new StartDocumentNodeGen(arguments);
}
@GeneratedBy(StartDocumentNode.class)
public static final class StartDocumentNodeGen extends StartDocumentNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@Child private RubyNode arguments2_;
@Child private RubyNode arguments3_;
@CompilationFinal private boolean seenUnsupported0;
private StartDocumentNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.arguments2_ = arguments != null && 2 < arguments.length ? arguments[2] : null;
this.arguments3_ = arguments != null && 3 < arguments.length ? arguments[3] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
executeDynamicObject(frameValue);
return;
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = arguments1_.execute(frameValue);
Object arguments2Value = arguments2_.execute(frameValue);
Object arguments3Value = arguments3_.execute(frameValue);
throw unsupported(ex.getResult(), arguments1Value, arguments2Value, arguments3Value);
}
DynamicObject arguments1Value_;
try {
arguments1Value_ = arguments1_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments2Value = arguments2_.execute(frameValue);
Object arguments3Value = arguments3_.execute(frameValue);
throw unsupported(arguments0Value_, ex.getResult(), arguments2Value, arguments3Value);
}
DynamicObject arguments2Value_;
try {
arguments2Value_ = arguments2_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments3Value = arguments3_.execute(frameValue);
throw unsupported(arguments0Value_, arguments1Value_, ex.getResult(), arguments3Value);
}
boolean arguments3Value_;
try {
arguments3Value_ = arguments3_.executeBoolean(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(arguments0Value_, arguments1Value_, arguments2Value_, ex.getResult());
}
if ((RubyGuards.isRubyArray(arguments1Value_)) && (RubyGuards.isRubyArray(arguments2Value_))) {
return this.startDocument(arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_);
}
throw unsupported(arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_);
}
private UnsupportedSpecializationException unsupported(Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_, arguments1_, arguments2_, arguments3_}, arguments0Value, arguments1Value, arguments2Value, arguments3Value);
}
}
}
@GeneratedBy(EndDocumentNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class EndDocumentNodeFactory implements NodeFactory {
private static EndDocumentNodeFactory endDocumentNodeFactoryInstance;
private EndDocumentNodeFactory() {
}
@Override
public Class getNodeClass() {
return EndDocumentNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public EndDocumentNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (endDocumentNodeFactoryInstance == null) {
endDocumentNodeFactoryInstance = new EndDocumentNodeFactory();
}
return endDocumentNodeFactoryInstance;
}
public static EndDocumentNode create(RubyNode[] arguments) {
return new EndDocumentNodeGen(arguments);
}
@GeneratedBy(EndDocumentNode.class)
public static final class EndDocumentNodeGen extends EndDocumentNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@CompilationFinal private boolean seenUnsupported0;
private EndDocumentNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
executeDynamicObject(frameValue);
return;
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = arguments1_.execute(frameValue);
throw unsupported(ex.getResult(), arguments1Value);
}
boolean arguments1Value_;
try {
arguments1Value_ = arguments1_.executeBoolean(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(arguments0Value_, ex.getResult());
}
return this.endDocument(arguments0Value_, arguments1Value_);
}
private UnsupportedSpecializationException unsupported(Object arguments0Value, Object arguments1Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_, arguments1_}, arguments0Value, arguments1Value);
}
}
}
@GeneratedBy(ScalarNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class ScalarNodeFactory implements NodeFactory {
private static ScalarNodeFactory scalarNodeFactoryInstance;
private ScalarNodeFactory() {
}
@Override
public Class getNodeClass() {
return ScalarNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class, RubyNode.class, RubyNode.class, RubyNode.class, RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public ScalarNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (scalarNodeFactoryInstance == null) {
scalarNodeFactoryInstance = new ScalarNodeFactory();
}
return scalarNodeFactoryInstance;
}
public static ScalarNode create(RubyNode[] arguments) {
return new ScalarNodeGen(arguments);
}
@GeneratedBy(ScalarNode.class)
public static final class ScalarNodeGen extends ScalarNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@Child private RubyNode arguments2_;
@Child private RubyNode arguments3_;
@Child private RubyNode arguments4_;
@Child private RubyNode arguments5_;
@Child private RubyNode arguments6_;
@CompilationFinal private Class> arguments4Type_;
@CompilationFinal private Class> arguments5Type_;
@CompilationFinal private Class> arguments6Type_;
@Child private BaseNode_ specialization_;
private ScalarNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.arguments2_ = arguments != null && 2 < arguments.length ? arguments[2] : null;
this.arguments3_ = arguments != null && 3 < arguments.length ? arguments[3] : null;
this.arguments4_ = arguments != null && 4 < arguments.length ? arguments[4] : null;
this.arguments5_ = arguments != null && 5 < arguments.length ? arguments[5] : null;
this.arguments6_ = arguments != null && 6 < arguments.length ? arguments[6] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
return specialization_.executeDynamicObject(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(ScalarNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected ScalarNodeGen root;
BaseNode_(ScalarNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (ScalarNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_, root.arguments1_, root.arguments2_, root.arguments3_, root.arguments4_, root.arguments5_, root.arguments6_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object... args_) {
return this.executeDynamicObject_((VirtualFrame) frameValue, args_[0], args_[1], args_[2], args_[3], args_[4], args_[5], args_[6]);
}
public abstract DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value, Object arguments4Value, Object arguments5Value, Object arguments6Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = root.arguments1_.execute(frameValue);
Object arguments2Value_ = root.arguments2_.execute(frameValue);
Object arguments3Value_ = root.arguments3_.execute(frameValue);
Object arguments4Value_ = executeArguments4_(frameValue);
Object arguments5Value_ = executeArguments5_(frameValue);
Object arguments6Value_ = executeArguments6_(frameValue);
return executeDynamicObject_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_, arguments4Value_, arguments5Value_, arguments6Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
return (DynamicObject) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object... args_) {
if (args_[0] instanceof DynamicObject && args_[1] instanceof DynamicObject && args_[4] instanceof Boolean && args_[5] instanceof Boolean && RubyTypesGen.isImplicitInteger(args_[6])) {
DynamicObject arguments1Value_ = (DynamicObject) args_[1];
if ((RubyGuards.isRubyString(arguments1Value_))) {
return ScalarNode_.create(root, args_[6]);
}
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments4_(Frame frameValue) {
Class> arguments4Type_ = root.arguments4Type_;
try {
if (arguments4Type_ == boolean.class) {
return root.arguments4_.executeBoolean((VirtualFrame) frameValue);
} else if (arguments4Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments4_.execute((VirtualFrame) frameValue);
if (_value instanceof Boolean) {
_type = boolean.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments4Type_ = _type;
}
} else {
return root.arguments4_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments4Type_ = Object.class;
return ex.getResult();
}
}
protected final Object executeArguments5_(Frame frameValue) {
Class> arguments5Type_ = root.arguments5Type_;
try {
if (arguments5Type_ == boolean.class) {
return root.arguments5_.executeBoolean((VirtualFrame) frameValue);
} else if (arguments5Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments5_.execute((VirtualFrame) frameValue);
if (_value instanceof Boolean) {
_type = boolean.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments5Type_ = _type;
}
} else {
return root.arguments5_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments5Type_ = Object.class;
return ex.getResult();
}
}
protected final Object executeArguments6_(Frame frameValue) {
Class> arguments6Type_ = root.arguments6Type_;
try {
if (arguments6Type_ == int.class) {
return root.arguments6_.executeInteger((VirtualFrame) frameValue);
} else if (arguments6Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments6_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments6Type_ = _type;
}
} else {
return root.arguments6_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments6Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(ScalarNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(ScalarNodeGen root) {
super(root, 2147483647);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value, Object arguments4Value, Object arguments5Value, Object arguments6Value) {
return (DynamicObject) uninitialized(frameValue, arguments0Value, arguments1Value, arguments2Value, arguments3Value, arguments4Value, arguments5Value, arguments6Value);
}
static BaseNode_ create(ScalarNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(ScalarNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(ScalarNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object... args_) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, args_[0], args_[1], args_[2], args_[3], args_[4], args_[5], args_[6]));
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value, Object arguments4Value, Object arguments5Value, Object arguments6Value) {
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value, arguments2Value, arguments3Value, arguments4Value, arguments5Value, arguments6Value);
}
static BaseNode_ create(ScalarNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "scalar(DynamicObject, DynamicObject, Object, Object, boolean, boolean, int)", value = ScalarNode.class)
private static final class ScalarNode_ extends BaseNode_ {
private final Class> arguments6ImplicitType;
ScalarNode_(ScalarNodeGen root, Object arguments6Value) {
super(root, 1);
this.arguments6ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments6Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments6ImplicitType == ((ScalarNode_) other).arguments6ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject(frameValue);
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = root.arguments1_.execute(frameValue);
Object arguments2Value = root.arguments2_.execute(frameValue);
Object arguments3Value = root.arguments3_.execute(frameValue);
Object arguments4Value = executeArguments4_(frameValue);
Object arguments5Value = executeArguments5_(frameValue);
Object arguments6Value = executeArguments6_(frameValue);
return getNext().executeDynamicObject_(frameValue, ex.getResult(), arguments1Value, arguments2Value, arguments3Value, arguments4Value, arguments5Value, arguments6Value);
}
DynamicObject arguments1Value_;
try {
arguments1Value_ = root.arguments1_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments2Value = root.arguments2_.execute(frameValue);
Object arguments3Value = root.arguments3_.execute(frameValue);
Object arguments4Value = executeArguments4_(frameValue);
Object arguments5Value = executeArguments5_(frameValue);
Object arguments6Value = executeArguments6_(frameValue);
return getNext().executeDynamicObject_(frameValue, arguments0Value_, ex.getResult(), arguments2Value, arguments3Value, arguments4Value, arguments5Value, arguments6Value);
}
Object arguments2Value_ = root.arguments2_.execute(frameValue);
Object arguments3Value_ = root.arguments3_.execute(frameValue);
boolean arguments4Value_;
try {
arguments4Value_ = root.arguments4_.executeBoolean(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments5Value = executeArguments5_(frameValue);
Object arguments6Value = executeArguments6_(frameValue);
return getNext().executeDynamicObject_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_, ex.getResult(), arguments5Value, arguments6Value);
}
boolean arguments5Value_;
try {
arguments5Value_ = root.arguments5_.executeBoolean(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments6Value = executeArguments6_(frameValue);
return getNext().executeDynamicObject_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_, arguments4Value_, ex.getResult(), arguments6Value);
}
int arguments6Value_;
try {
if (arguments6ImplicitType == int.class) {
arguments6Value_ = root.arguments6_.executeInteger(frameValue);
} else {
Object arguments6Value__ = executeArguments6_(frameValue);
arguments6Value_ = RubyTypesGen.expectImplicitInteger(arguments6Value__, arguments6ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeDynamicObject_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_, arguments4Value_, arguments5Value_, ex.getResult());
}
if ((RubyGuards.isRubyString(arguments1Value_))) {
return root.scalar(arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_, arguments4Value_, arguments5Value_, arguments6Value_);
}
return getNext().executeDynamicObject_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_, arguments4Value_, arguments5Value_, arguments6Value_);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value, Object arguments4Value, Object arguments5Value, Object arguments6Value) {
if (arguments0Value instanceof DynamicObject && arguments1Value instanceof DynamicObject && arguments4Value instanceof Boolean && arguments5Value instanceof Boolean && RubyTypesGen.isImplicitInteger(arguments6Value, arguments6ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
boolean arguments4Value_ = (boolean) arguments4Value;
boolean arguments5Value_ = (boolean) arguments5Value;
int arguments6Value_ = RubyTypesGen.asImplicitInteger(arguments6Value, arguments6ImplicitType);
if ((RubyGuards.isRubyString(arguments1Value_))) {
return root.scalar(arguments0Value_, arguments1Value_, arguments2Value, arguments3Value, arguments4Value_, arguments5Value_, arguments6Value_);
}
}
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value, arguments2Value, arguments3Value, arguments4Value, arguments5Value, arguments6Value);
}
static BaseNode_ create(ScalarNodeGen root, Object arguments6Value) {
return new ScalarNode_(root, arguments6Value);
}
}
}
}
@GeneratedBy(StartSequenceNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class StartSequenceNodeFactory implements NodeFactory {
private static StartSequenceNodeFactory startSequenceNodeFactoryInstance;
private StartSequenceNodeFactory() {
}
@Override
public Class getNodeClass() {
return StartSequenceNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class, RubyNode.class, RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public StartSequenceNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (startSequenceNodeFactoryInstance == null) {
startSequenceNodeFactoryInstance = new StartSequenceNodeFactory();
}
return startSequenceNodeFactoryInstance;
}
public static StartSequenceNode create(RubyNode[] arguments) {
return new StartSequenceNodeGen(arguments);
}
@GeneratedBy(StartSequenceNode.class)
public static final class StartSequenceNodeGen extends StartSequenceNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@Child private RubyNode arguments2_;
@Child private RubyNode arguments3_;
@Child private RubyNode arguments4_;
@CompilationFinal private Class> arguments3Type_;
@CompilationFinal private Class> arguments4Type_;
@Child private BaseNode_ specialization_;
private StartSequenceNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.arguments2_ = arguments != null && 2 < arguments.length ? arguments[2] : null;
this.arguments3_ = arguments != null && 3 < arguments.length ? arguments[3] : null;
this.arguments4_ = arguments != null && 4 < arguments.length ? arguments[4] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
return specialization_.executeDynamicObject(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(StartSequenceNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected StartSequenceNodeGen root;
BaseNode_(StartSequenceNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (StartSequenceNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_, root.arguments1_, root.arguments2_, root.arguments3_, root.arguments4_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value, Object arguments4Value) {
return this.executeDynamicObject_((VirtualFrame) frameValue, arguments0Value, arguments1Value, arguments2Value, arguments3Value, arguments4Value);
}
public abstract DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value, Object arguments4Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = root.arguments1_.execute(frameValue);
Object arguments2Value_ = root.arguments2_.execute(frameValue);
Object arguments3Value_ = executeArguments3_(frameValue);
Object arguments4Value_ = executeArguments4_(frameValue);
return executeDynamicObject_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_, arguments4Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
return (DynamicObject) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value, Object arguments4Value) {
if (arguments0Value instanceof DynamicObject && arguments3Value instanceof Boolean && RubyTypesGen.isImplicitInteger(arguments4Value)) {
return StartSequenceNode_.create(root, arguments4Value);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments3_(Frame frameValue) {
Class> arguments3Type_ = root.arguments3Type_;
try {
if (arguments3Type_ == boolean.class) {
return root.arguments3_.executeBoolean((VirtualFrame) frameValue);
} else if (arguments3Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments3_.execute((VirtualFrame) frameValue);
if (_value instanceof Boolean) {
_type = boolean.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments3Type_ = _type;
}
} else {
return root.arguments3_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments3Type_ = Object.class;
return ex.getResult();
}
}
protected final Object executeArguments4_(Frame frameValue) {
Class> arguments4Type_ = root.arguments4Type_;
try {
if (arguments4Type_ == int.class) {
return root.arguments4_.executeInteger((VirtualFrame) frameValue);
} else if (arguments4Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments4_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments4Type_ = _type;
}
} else {
return root.arguments4_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments4Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(StartSequenceNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(StartSequenceNodeGen root) {
super(root, 2147483647);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value, Object arguments4Value) {
return (DynamicObject) uninitialized(frameValue, arguments0Value, arguments1Value, arguments2Value, arguments3Value, arguments4Value);
}
static BaseNode_ create(StartSequenceNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(StartSequenceNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(StartSequenceNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value, Object arguments4Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value, arguments1Value, arguments2Value, arguments3Value, arguments4Value));
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value, Object arguments4Value) {
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value, arguments2Value, arguments3Value, arguments4Value);
}
static BaseNode_ create(StartSequenceNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "startSequence(DynamicObject, Object, Object, boolean, int)", value = StartSequenceNode.class)
private static final class StartSequenceNode_ extends BaseNode_ {
private final Class> arguments4ImplicitType;
StartSequenceNode_(StartSequenceNodeGen root, Object arguments4Value) {
super(root, 1);
this.arguments4ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments4Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments4ImplicitType == ((StartSequenceNode_) other).arguments4ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject(frameValue);
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = root.arguments1_.execute(frameValue);
Object arguments2Value = root.arguments2_.execute(frameValue);
Object arguments3Value = executeArguments3_(frameValue);
Object arguments4Value = executeArguments4_(frameValue);
return getNext().executeDynamicObject_(frameValue, ex.getResult(), arguments1Value, arguments2Value, arguments3Value, arguments4Value);
}
Object arguments1Value_ = root.arguments1_.execute(frameValue);
Object arguments2Value_ = root.arguments2_.execute(frameValue);
boolean arguments3Value_;
try {
arguments3Value_ = root.arguments3_.executeBoolean(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments4Value = executeArguments4_(frameValue);
return getNext().executeDynamicObject_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, ex.getResult(), arguments4Value);
}
int arguments4Value_;
try {
if (arguments4ImplicitType == int.class) {
arguments4Value_ = root.arguments4_.executeInteger(frameValue);
} else {
Object arguments4Value__ = executeArguments4_(frameValue);
arguments4Value_ = RubyTypesGen.expectImplicitInteger(arguments4Value__, arguments4ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeDynamicObject_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_, ex.getResult());
}
return root.startSequence(arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_, arguments4Value_);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value, Object arguments4Value) {
if (arguments0Value instanceof DynamicObject && arguments3Value instanceof Boolean && RubyTypesGen.isImplicitInteger(arguments4Value, arguments4ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
boolean arguments3Value_ = (boolean) arguments3Value;
int arguments4Value_ = RubyTypesGen.asImplicitInteger(arguments4Value, arguments4ImplicitType);
return root.startSequence(arguments0Value_, arguments1Value, arguments2Value, arguments3Value_, arguments4Value_);
}
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value, arguments2Value, arguments3Value, arguments4Value);
}
static BaseNode_ create(StartSequenceNodeGen root, Object arguments4Value) {
return new StartSequenceNode_(root, arguments4Value);
}
}
}
}
@GeneratedBy(EndSequenceNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class EndSequenceNodeFactory implements NodeFactory {
private static EndSequenceNodeFactory endSequenceNodeFactoryInstance;
private EndSequenceNodeFactory() {
}
@Override
public Class getNodeClass() {
return EndSequenceNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public EndSequenceNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (endSequenceNodeFactoryInstance == null) {
endSequenceNodeFactoryInstance = new EndSequenceNodeFactory();
}
return endSequenceNodeFactoryInstance;
}
public static EndSequenceNode create(RubyNode[] arguments) {
return new EndSequenceNodeGen(arguments);
}
@GeneratedBy(EndSequenceNode.class)
public static final class EndSequenceNodeGen extends EndSequenceNode {
@Child private RubyNode arguments0_;
@CompilationFinal private boolean seenUnsupported0;
private EndSequenceNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(ex.getResult());
}
return this.endSequence(arguments0Value_);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
private UnsupportedSpecializationException unsupported(Object arguments0Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_}, arguments0Value);
}
}
}
@GeneratedBy(StartMappingNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class StartMappingNodeFactory implements NodeFactory {
private static StartMappingNodeFactory startMappingNodeFactoryInstance;
private StartMappingNodeFactory() {
}
@Override
public Class getNodeClass() {
return StartMappingNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class, RubyNode.class, RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public StartMappingNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (startMappingNodeFactoryInstance == null) {
startMappingNodeFactoryInstance = new StartMappingNodeFactory();
}
return startMappingNodeFactoryInstance;
}
public static StartMappingNode create(RubyNode[] arguments) {
return new StartMappingNodeGen(arguments);
}
@GeneratedBy(StartMappingNode.class)
public static final class StartMappingNodeGen extends StartMappingNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@Child private RubyNode arguments2_;
@Child private RubyNode arguments3_;
@Child private RubyNode arguments4_;
@CompilationFinal private Class> arguments3Type_;
@CompilationFinal private Class> arguments4Type_;
@Child private BaseNode_ specialization_;
private StartMappingNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.arguments2_ = arguments != null && 2 < arguments.length ? arguments[2] : null;
this.arguments3_ = arguments != null && 3 < arguments.length ? arguments[3] : null;
this.arguments4_ = arguments != null && 4 < arguments.length ? arguments[4] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
return specialization_.executeDynamicObject(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(StartMappingNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected StartMappingNodeGen root;
BaseNode_(StartMappingNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (StartMappingNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_, root.arguments1_, root.arguments2_, root.arguments3_, root.arguments4_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value, Object arguments4Value) {
return this.executeDynamicObject_((VirtualFrame) frameValue, arguments0Value, arguments1Value, arguments2Value, arguments3Value, arguments4Value);
}
public abstract DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value, Object arguments4Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = root.arguments1_.execute(frameValue);
Object arguments2Value_ = root.arguments2_.execute(frameValue);
Object arguments3Value_ = executeArguments3_(frameValue);
Object arguments4Value_ = executeArguments4_(frameValue);
return executeDynamicObject_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_, arguments4Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
return (DynamicObject) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value, Object arguments4Value) {
if (arguments0Value instanceof DynamicObject && arguments3Value instanceof Boolean && RubyTypesGen.isImplicitInteger(arguments4Value)) {
return StartMappingNode_.create(root, arguments4Value);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments3_(Frame frameValue) {
Class> arguments3Type_ = root.arguments3Type_;
try {
if (arguments3Type_ == boolean.class) {
return root.arguments3_.executeBoolean((VirtualFrame) frameValue);
} else if (arguments3Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments3_.execute((VirtualFrame) frameValue);
if (_value instanceof Boolean) {
_type = boolean.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments3Type_ = _type;
}
} else {
return root.arguments3_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments3Type_ = Object.class;
return ex.getResult();
}
}
protected final Object executeArguments4_(Frame frameValue) {
Class> arguments4Type_ = root.arguments4Type_;
try {
if (arguments4Type_ == int.class) {
return root.arguments4_.executeInteger((VirtualFrame) frameValue);
} else if (arguments4Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments4_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments4Type_ = _type;
}
} else {
return root.arguments4_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments4Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(StartMappingNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(StartMappingNodeGen root) {
super(root, 2147483647);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value, Object arguments4Value) {
return (DynamicObject) uninitialized(frameValue, arguments0Value, arguments1Value, arguments2Value, arguments3Value, arguments4Value);
}
static BaseNode_ create(StartMappingNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(StartMappingNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(StartMappingNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value, Object arguments4Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value, arguments1Value, arguments2Value, arguments3Value, arguments4Value));
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value, Object arguments4Value) {
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value, arguments2Value, arguments3Value, arguments4Value);
}
static BaseNode_ create(StartMappingNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "startMapping(DynamicObject, Object, Object, boolean, int)", value = StartMappingNode.class)
private static final class StartMappingNode_ extends BaseNode_ {
private final Class> arguments4ImplicitType;
StartMappingNode_(StartMappingNodeGen root, Object arguments4Value) {
super(root, 1);
this.arguments4ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments4Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments4ImplicitType == ((StartMappingNode_) other).arguments4ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeDynamicObject(frameValue);
}
@Override
public DynamicObject executeDynamicObject(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = root.arguments1_.execute(frameValue);
Object arguments2Value = root.arguments2_.execute(frameValue);
Object arguments3Value = executeArguments3_(frameValue);
Object arguments4Value = executeArguments4_(frameValue);
return getNext().executeDynamicObject_(frameValue, ex.getResult(), arguments1Value, arguments2Value, arguments3Value, arguments4Value);
}
Object arguments1Value_ = root.arguments1_.execute(frameValue);
Object arguments2Value_ = root.arguments2_.execute(frameValue);
boolean arguments3Value_;
try {
arguments3Value_ = root.arguments3_.executeBoolean(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments4Value = executeArguments4_(frameValue);
return getNext().executeDynamicObject_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, ex.getResult(), arguments4Value);
}
int arguments4Value_;
try {
if (arguments4ImplicitType == int.class) {
arguments4Value_ = root.arguments4_.executeInteger(frameValue);
} else {
Object arguments4Value__ = executeArguments4_(frameValue);
arguments4Value_ = RubyTypesGen.expectImplicitInteger(arguments4Value__, arguments4ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeDynamicObject_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_, ex.getResult());
}
return root.startMapping(arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_, arguments4Value_);
}
@Override
public DynamicObject executeDynamicObject_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value, Object arguments4Value) {
if (arguments0Value instanceof DynamicObject && arguments3Value instanceof Boolean && RubyTypesGen.isImplicitInteger(arguments4Value, arguments4ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
boolean arguments3Value_ = (boolean) arguments3Value;
int arguments4Value_ = RubyTypesGen.asImplicitInteger(arguments4Value, arguments4ImplicitType);
return root.startMapping(arguments0Value_, arguments1Value, arguments2Value, arguments3Value_, arguments4Value_);
}
return getNext().executeDynamicObject_(frameValue, arguments0Value, arguments1Value, arguments2Value, arguments3Value, arguments4Value);
}
static BaseNode_ create(StartMappingNodeGen root, Object arguments4Value) {
return new StartMappingNode_(root, arguments4Value);
}
}
}
}
@GeneratedBy(EndMappingNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class EndMappingNodeFactory implements NodeFactory {
private static EndMappingNodeFactory endMappingNodeFactoryInstance;
private EndMappingNodeFactory() {
}
@Override
public Class getNodeClass() {
return EndMappingNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public EndMappingNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (endMappingNodeFactoryInstance == null) {
endMappingNodeFactoryInstance = new EndMappingNodeFactory();
}
return endMappingNodeFactoryInstance;
}
public static EndMappingNode create(RubyNode[] arguments) {
return new EndMappingNodeGen(arguments);
}
@GeneratedBy(EndMappingNode.class)
public static final class EndMappingNodeGen extends EndMappingNode {
@Child private RubyNode arguments0_;
@CompilationFinal private boolean seenUnsupported0;
private EndMappingNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(ex.getResult());
}
return this.endMapping(arguments0Value_);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
private UnsupportedSpecializationException unsupported(Object arguments0Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_}, arguments0Value);
}
}
}
@GeneratedBy(AliasNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class AliasNodeFactory implements NodeFactory {
private static AliasNodeFactory aliasNodeFactoryInstance;
private AliasNodeFactory() {
}
@Override
public Class getNodeClass() {
return AliasNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public AliasNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (aliasNodeFactoryInstance == null) {
aliasNodeFactoryInstance = new AliasNodeFactory();
}
return aliasNodeFactoryInstance;
}
public static AliasNode create(RubyNode[] arguments) {
return new AliasNodeGen(arguments);
}
@GeneratedBy(AliasNode.class)
public static final class AliasNodeGen extends AliasNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@CompilationFinal private boolean seenUnsupported0;
private AliasNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = arguments1_.execute(frameValue);
throw unsupported(ex.getResult(), arguments1Value);
}
Object arguments1Value_ = arguments1_.execute(frameValue);
return this.alias(arguments0Value_, arguments1Value_);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
private UnsupportedSpecializationException unsupported(Object arguments0Value, Object arguments1Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_, arguments1_}, arguments0Value, arguments1Value);
}
}
}
@GeneratedBy(SetCanonicalNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class SetCanonicalNodeFactory implements NodeFactory {
private static SetCanonicalNodeFactory setCanonicalNodeFactoryInstance;
private SetCanonicalNodeFactory() {
}
@Override
public Class getNodeClass() {
return SetCanonicalNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public SetCanonicalNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (setCanonicalNodeFactoryInstance == null) {
setCanonicalNodeFactoryInstance = new SetCanonicalNodeFactory();
}
return setCanonicalNodeFactoryInstance;
}
public static SetCanonicalNode create(RubyNode[] arguments) {
return new SetCanonicalNodeGen(arguments);
}
@GeneratedBy(SetCanonicalNode.class)
public static final class SetCanonicalNodeGen extends SetCanonicalNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@CompilationFinal private boolean seenUnsupported0;
private SetCanonicalNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeBoolean(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
executeBoolean(frameValue);
return;
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = arguments1_.execute(frameValue);
throw unsupported(ex.getResult(), arguments1Value);
}
boolean arguments1Value_;
try {
arguments1Value_ = arguments1_.executeBoolean(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(arguments0Value_, ex.getResult());
}
return this.setCanonical(arguments0Value_, arguments1Value_);
}
private UnsupportedSpecializationException unsupported(Object arguments0Value, Object arguments1Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_, arguments1_}, arguments0Value, arguments1Value);
}
}
}
@GeneratedBy(CanonicalNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class CanonicalNodeFactory implements NodeFactory {
private static CanonicalNodeFactory canonicalNodeFactoryInstance;
private CanonicalNodeFactory() {
}
@Override
public Class getNodeClass() {
return CanonicalNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public CanonicalNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (canonicalNodeFactoryInstance == null) {
canonicalNodeFactoryInstance = new CanonicalNodeFactory();
}
return canonicalNodeFactoryInstance;
}
public static CanonicalNode create(RubyNode[] arguments) {
return new CanonicalNodeGen(arguments);
}
@GeneratedBy(CanonicalNode.class)
public static final class CanonicalNodeGen extends CanonicalNode {
@Child private RubyNode arguments0_;
@CompilationFinal private boolean seenUnsupported0;
private CanonicalNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeBoolean(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
executeBoolean(frameValue);
return;
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(ex.getResult());
}
return this.canonical(arguments0Value_);
}
private UnsupportedSpecializationException unsupported(Object arguments0Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_}, arguments0Value);
}
}
}
@GeneratedBy(SetIndentationNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class SetIndentationNodeFactory implements NodeFactory {
private static SetIndentationNodeFactory setIndentationNodeFactoryInstance;
private SetIndentationNodeFactory() {
}
@Override
public Class getNodeClass() {
return SetIndentationNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public SetIndentationNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (setIndentationNodeFactoryInstance == null) {
setIndentationNodeFactoryInstance = new SetIndentationNodeFactory();
}
return setIndentationNodeFactoryInstance;
}
public static SetIndentationNode create(RubyNode[] arguments) {
return new SetIndentationNodeGen(arguments);
}
@GeneratedBy(SetIndentationNode.class)
public static final class SetIndentationNodeGen extends SetIndentationNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@CompilationFinal private Class> arguments1Type_;
@Child private BaseNode_ specialization_;
private SetIndentationNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public int executeInteger(VirtualFrame frameValue) {
return specialization_.executeInt(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(SetIndentationNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected SetIndentationNodeGen root;
BaseNode_(SetIndentationNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (SetIndentationNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_, root.arguments1_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value, Object arguments1Value) {
return this.executeInt_((VirtualFrame) frameValue, arguments0Value, arguments1Value);
}
public abstract int executeInt_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = executeArguments1_(frameValue);
return executeInt_(frameValue, arguments0Value_, arguments1Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public int executeInt(VirtualFrame frameValue) {
return (int) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value)) {
return SetIndentationNode_.create(root, arguments1Value);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments1_(Frame frameValue) {
Class> arguments1Type_ = root.arguments1Type_;
try {
if (arguments1Type_ == int.class) {
return root.arguments1_.executeInteger((VirtualFrame) frameValue);
} else if (arguments1Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments1_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments1Type_ = _type;
}
} else {
return root.arguments1_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments1Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(SetIndentationNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(SetIndentationNodeGen root) {
super(root, 2147483647);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
return (int) uninitialized(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(SetIndentationNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(SetIndentationNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(SetIndentationNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value, arguments1Value));
}
@Override
public int executeInt(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = executeArguments1_(frameValue);
return getNext().executeInt_(frameValue, arguments0Value_, arguments1Value_);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
return getNext().executeInt_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(SetIndentationNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "setIndentation(DynamicObject, int)", value = SetIndentationNode.class)
private static final class SetIndentationNode_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
SetIndentationNode_(SetIndentationNodeGen root, Object arguments1Value) {
super(root, 1);
this.arguments1ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments1Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((SetIndentationNode_) other).arguments1ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeInt(frameValue);
}
@Override
public int executeInt(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
return getNext().executeInt_(frameValue, ex.getResult(), arguments1Value);
}
int arguments1Value_;
try {
if (arguments1ImplicitType == int.class) {
arguments1Value_ = root.arguments1_.executeInteger(frameValue);
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitInteger(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeInt_(frameValue, arguments0Value_, ex.getResult());
}
return root.setIndentation(arguments0Value_, arguments1Value_);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value, arguments1ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
int arguments1Value_ = RubyTypesGen.asImplicitInteger(arguments1Value, arguments1ImplicitType);
return root.setIndentation(arguments0Value_, arguments1Value_);
}
return getNext().executeInt_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(SetIndentationNodeGen root, Object arguments1Value) {
return new SetIndentationNode_(root, arguments1Value);
}
}
}
}
@GeneratedBy(IndentationNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class IndentationNodeFactory implements NodeFactory {
private static IndentationNodeFactory indentationNodeFactoryInstance;
private IndentationNodeFactory() {
}
@Override
public Class getNodeClass() {
return IndentationNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public IndentationNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (indentationNodeFactoryInstance == null) {
indentationNodeFactoryInstance = new IndentationNodeFactory();
}
return indentationNodeFactoryInstance;
}
public static IndentationNode create(RubyNode[] arguments) {
return new IndentationNodeGen(arguments);
}
@GeneratedBy(IndentationNode.class)
public static final class IndentationNodeGen extends IndentationNode {
@Child private RubyNode arguments0_;
@CompilationFinal private boolean seenUnsupported0;
private IndentationNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeInteger(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
executeInteger(frameValue);
return;
}
@Override
public int executeInteger(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(ex.getResult());
}
return this.indentation(arguments0Value_);
}
private UnsupportedSpecializationException unsupported(Object arguments0Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_}, arguments0Value);
}
}
}
@GeneratedBy(SetLineWidthNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class SetLineWidthNodeFactory implements NodeFactory {
private static SetLineWidthNodeFactory setLineWidthNodeFactoryInstance;
private SetLineWidthNodeFactory() {
}
@Override
public Class getNodeClass() {
return SetLineWidthNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public SetLineWidthNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (setLineWidthNodeFactoryInstance == null) {
setLineWidthNodeFactoryInstance = new SetLineWidthNodeFactory();
}
return setLineWidthNodeFactoryInstance;
}
public static SetLineWidthNode create(RubyNode[] arguments) {
return new SetLineWidthNodeGen(arguments);
}
@GeneratedBy(SetLineWidthNode.class)
public static final class SetLineWidthNodeGen extends SetLineWidthNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@CompilationFinal private Class> arguments1Type_;
@Child private BaseNode_ specialization_;
private SetLineWidthNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public int executeInteger(VirtualFrame frameValue) {
return specialization_.executeInt(frameValue);
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(SetLineWidthNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected SetLineWidthNodeGen root;
BaseNode_(SetLineWidthNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (SetLineWidthNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_, root.arguments1_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value, Object arguments1Value) {
return this.executeInt_((VirtualFrame) frameValue, arguments0Value, arguments1Value);
}
public abstract int executeInt_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value);
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = executeArguments1_(frameValue);
return executeInt_(frameValue, arguments0Value_, arguments1Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
public int executeInt(VirtualFrame frameValue) {
return (int) execute(frameValue);
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value)) {
return SetLineWidthNode_.create(root, arguments1Value);
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeArguments1_(Frame frameValue) {
Class> arguments1Type_ = root.arguments1Type_;
try {
if (arguments1Type_ == int.class) {
return root.arguments1_.executeInteger((VirtualFrame) frameValue);
} else if (arguments1Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.arguments1_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.arguments1Type_ = _type;
}
} else {
return root.arguments1_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.arguments1Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(SetLineWidthNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(SetLineWidthNodeGen root) {
super(root, 2147483647);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
return (int) uninitialized(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(SetLineWidthNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(SetLineWidthNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(SetLineWidthNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value, arguments1Value));
}
@Override
public int executeInt(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = executeArguments1_(frameValue);
return getNext().executeInt_(frameValue, arguments0Value_, arguments1Value_);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
return getNext().executeInt_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(SetLineWidthNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "setLineWidth(DynamicObject, int)", value = SetLineWidthNode.class)
private static final class SetLineWidthNode_ extends BaseNode_ {
private final Class> arguments1ImplicitType;
SetLineWidthNode_(SetLineWidthNodeGen root, Object arguments1Value) {
super(root, 1);
this.arguments1ImplicitType = RubyTypesGen.getImplicitIntegerClass(arguments1Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.arguments1ImplicitType == ((SetLineWidthNode_) other).arguments1ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeInt(frameValue);
}
@Override
public int executeInt(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = root.arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
Object arguments1Value = executeArguments1_(frameValue);
return getNext().executeInt_(frameValue, ex.getResult(), arguments1Value);
}
int arguments1Value_;
try {
if (arguments1ImplicitType == int.class) {
arguments1Value_ = root.arguments1_.executeInteger(frameValue);
} else {
Object arguments1Value__ = executeArguments1_(frameValue);
arguments1Value_ = RubyTypesGen.expectImplicitInteger(arguments1Value__, arguments1ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().executeInt_(frameValue, arguments0Value_, ex.getResult());
}
return root.setLineWidth(arguments0Value_, arguments1Value_);
}
@Override
public int executeInt_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value) {
if (arguments0Value instanceof DynamicObject && RubyTypesGen.isImplicitInteger(arguments1Value, arguments1ImplicitType)) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
int arguments1Value_ = RubyTypesGen.asImplicitInteger(arguments1Value, arguments1ImplicitType);
return root.setLineWidth(arguments0Value_, arguments1Value_);
}
return getNext().executeInt_(frameValue, arguments0Value, arguments1Value);
}
static BaseNode_ create(SetLineWidthNodeGen root, Object arguments1Value) {
return new SetLineWidthNode_(root, arguments1Value);
}
}
}
}
@GeneratedBy(LineWidthNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class LineWidthNodeFactory implements NodeFactory {
private static LineWidthNodeFactory lineWidthNodeFactoryInstance;
private LineWidthNodeFactory() {
}
@Override
public Class getNodeClass() {
return LineWidthNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public LineWidthNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (lineWidthNodeFactoryInstance == null) {
lineWidthNodeFactoryInstance = new LineWidthNodeFactory();
}
return lineWidthNodeFactoryInstance;
}
public static LineWidthNode create(RubyNode[] arguments) {
return new LineWidthNodeGen(arguments);
}
@GeneratedBy(LineWidthNode.class)
public static final class LineWidthNodeGen extends LineWidthNode {
@Child private RubyNode arguments0_;
@CompilationFinal private boolean seenUnsupported0;
private LineWidthNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
@Override
public Object execute(VirtualFrame frameValue) {
return executeInteger(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
executeInteger(frameValue);
return;
}
@Override
public int executeInteger(VirtualFrame frameValue) {
DynamicObject arguments0Value_;
try {
arguments0Value_ = arguments0_.executeDynamicObject(frameValue);
} catch (UnexpectedResultException ex) {
throw unsupported(ex.getResult());
}
return this.lineWidth(arguments0Value_);
}
private UnsupportedSpecializationException unsupported(Object arguments0Value) {
if (!seenUnsupported0) {
CompilerDirectives.transferToInterpreterAndInvalidate();
seenUnsupported0 = true;
}
return new UnsupportedSpecializationException(this, new Node[] {arguments0_}, arguments0Value);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy