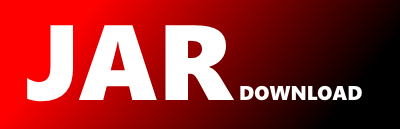
org.jruby.truffle.stdlib.psych.PsychParserNodesFactory Maven / Gradle / Ivy
The newest version!
// CheckStyle: start generated
package org.jruby.truffle.stdlib.psych;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NodeFactory;
import com.oracle.truffle.api.dsl.internal.SpecializationNode;
import com.oracle.truffle.api.dsl.internal.SpecializedNode;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.object.DynamicObject;
import com.oracle.truffle.api.profiles.BranchProfile;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import org.jruby.truffle.language.NotProvided;
import org.jruby.truffle.language.RubyNode;
import org.jruby.truffle.language.SnippetNode;
import org.jruby.truffle.language.dispatch.CallDispatchHeadNode;
import org.jruby.truffle.language.dispatch.DoesRespondDispatchHeadNode;
import org.jruby.truffle.language.objects.ReadObjectFieldNode;
import org.jruby.truffle.language.objects.TaintNode;
import org.jruby.truffle.stdlib.psych.PsychParserNodes.ParseNode;
@GeneratedBy(PsychParserNodes.class)
public final class PsychParserNodesFactory {
public static List> getFactories() {
return Collections.singletonList(ParseNodeFactory.getInstance());
}
@GeneratedBy(ParseNode.class)
@SuppressWarnings({"unchecked", "rawtypes"})
public static final class ParseNodeFactory implements NodeFactory {
private static ParseNodeFactory parseNodeFactoryInstance;
private ParseNodeFactory() {
}
@Override
public Class getNodeClass() {
return ParseNode.class;
}
@Override
public List getExecutionSignature() {
return Arrays.asList(RubyNode.class, RubyNode.class, RubyNode.class);
}
@Override
public List getNodeSignatures() {
return Arrays.asList(Arrays.asList(RubyNode[].class));
}
@Override
public ParseNode createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof RubyNode[])) {
return create((RubyNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
if (parseNodeFactoryInstance == null) {
parseNodeFactoryInstance = new ParseNodeFactory();
}
return parseNodeFactoryInstance;
}
public static ParseNode create(RubyNode[] arguments) {
return new ParseNodeGen(arguments);
}
@GeneratedBy(ParseNode.class)
public static final class ParseNodeGen extends ParseNode implements SpecializedNode {
@Child private RubyNode arguments0_;
@Child private RubyNode arguments1_;
@Child private RubyNode arguments2_;
@Child private BaseNode_ specialization_;
private ParseNodeGen(RubyNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.arguments2_ = arguments != null && 2 < arguments.length ? arguments[2] : null;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object executeParse(VirtualFrame frameValue, DynamicObject arguments0Value, DynamicObject arguments1Value, Object arguments2Value) {
return specialization_.execute1(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute0(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
@GeneratedBy(ParseNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected ParseNodeGen root;
BaseNode_(ParseNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (ParseNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.arguments0_, root.arguments1_, root.arguments2_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return this.execute_((VirtualFrame) frameValue, arguments0Value, arguments1Value, arguments2Value);
}
public abstract Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value);
public Object execute1(VirtualFrame frameValue, DynamicObject arguments0Value, DynamicObject arguments1Value, Object arguments2Value) {
return execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
public Object execute0(VirtualFrame frameValue) {
Object arguments0Value_ = root.arguments0_.execute(frameValue);
Object arguments1Value_ = root.arguments1_.execute(frameValue);
Object arguments2Value_ = root.arguments2_.execute(frameValue);
return execute_(frameValue, arguments0Value_, arguments1Value_, arguments2Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute0(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof DynamicObject && arguments1Value instanceof DynamicObject) {
if (arguments2Value instanceof NotProvided) {
return Parse0Node_.create(root);
}
if (arguments2Value instanceof DynamicObject) {
SnippetNode taintedNode2 = (new SnippetNode());
DoesRespondDispatchHeadNode respondToReadNode2 = (DoesRespondDispatchHeadNode.create());
DoesRespondDispatchHeadNode respondToPathNode2 = (DoesRespondDispatchHeadNode.create());
CallDispatchHeadNode callPathNode2 = (CallDispatchHeadNode.createMethodCall());
ReadObjectFieldNode readHandlerNode2 = (root.createReadHandlerNode());
CallDispatchHeadNode callStartStreamNode2 = (CallDispatchHeadNode.createMethodCall());
CallDispatchHeadNode callStartDocumentNode2 = (CallDispatchHeadNode.createMethodCall());
CallDispatchHeadNode callEndDocumentNode2 = (CallDispatchHeadNode.createMethodCall());
CallDispatchHeadNode callAliasNode2 = (CallDispatchHeadNode.createMethodCall());
CallDispatchHeadNode callScalarNode2 = (CallDispatchHeadNode.createMethodCall());
CallDispatchHeadNode callStartSequenceNode2 = (CallDispatchHeadNode.createMethodCall());
CallDispatchHeadNode callEndSequenceNode2 = (CallDispatchHeadNode.createMethodCall());
CallDispatchHeadNode callStartMappingNode2 = (CallDispatchHeadNode.createMethodCall());
CallDispatchHeadNode callEndMappingNode2 = (CallDispatchHeadNode.createMethodCall());
CallDispatchHeadNode callEndStreamNode2 = (CallDispatchHeadNode.createMethodCall());
SnippetNode raiseSyntaxErrorSnippetNode2 = (new SnippetNode());
SnippetNode tagPushNode2 = (new SnippetNode());
TaintNode taintNode2 = (TaintNode.create());
BranchProfile errorProfile2 = (BranchProfile.create());
return Parse1Node_.create(root, taintedNode2, respondToReadNode2, respondToPathNode2, callPathNode2, readHandlerNode2, callStartStreamNode2, callStartDocumentNode2, callEndDocumentNode2, callAliasNode2, callScalarNode2, callStartSequenceNode2, callEndSequenceNode2, callStartMappingNode2, callEndMappingNode2, callEndStreamNode2, raiseSyntaxErrorSnippetNode2, tagPushNode2, taintNode2, errorProfile2);
}
}
return null;
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
}
@GeneratedBy(ParseNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(ParseNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return uninitialized(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(ParseNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(ParseNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(ParseNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, arguments0Value, arguments1Value, arguments2Value));
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(ParseNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "parse(VirtualFrame, DynamicObject, DynamicObject, NotProvided)", value = ParseNode.class)
private static final class Parse0Node_ extends BaseNode_ {
Parse0Node_(ParseNodeGen root) {
super(root, 1);
}
@Override
public Object execute1(VirtualFrame frameValue, DynamicObject arguments0Value, DynamicObject arguments1Value, Object arguments2Value) {
if (arguments2Value instanceof NotProvided) {
NotProvided arguments2Value_ = (NotProvided) arguments2Value;
return root.parse(frameValue, arguments0Value, arguments1Value, arguments2Value_);
}
return getNext().execute1(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof DynamicObject && arguments1Value instanceof DynamicObject && arguments2Value instanceof NotProvided) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
NotProvided arguments2Value_ = (NotProvided) arguments2Value;
return root.parse(frameValue, arguments0Value_, arguments1Value_, arguments2Value_);
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(ParseNodeGen root) {
return new Parse0Node_(root);
}
}
@GeneratedBy(methodName = "parse(VirtualFrame, DynamicObject, DynamicObject, DynamicObject, SnippetNode, DoesRespondDispatchHeadNode, DoesRespondDispatchHeadNode, CallDispatchHeadNode, ReadObjectFieldNode, CallDispatchHeadNode, CallDispatchHeadNode, CallDispatchHeadNode, CallDispatchHeadNode, CallDispatchHeadNode, CallDispatchHeadNode, CallDispatchHeadNode, CallDispatchHeadNode, CallDispatchHeadNode, CallDispatchHeadNode, SnippetNode, SnippetNode, TaintNode, BranchProfile)", value = ParseNode.class)
private static final class Parse1Node_ extends BaseNode_ {
@Child private SnippetNode taintedNode;
@Child private DoesRespondDispatchHeadNode respondToReadNode;
@Child private DoesRespondDispatchHeadNode respondToPathNode;
@Child private CallDispatchHeadNode callPathNode;
@Child private ReadObjectFieldNode readHandlerNode;
@Child private CallDispatchHeadNode callStartStreamNode;
@Child private CallDispatchHeadNode callStartDocumentNode;
@Child private CallDispatchHeadNode callEndDocumentNode;
@Child private CallDispatchHeadNode callAliasNode;
@Child private CallDispatchHeadNode callScalarNode;
@Child private CallDispatchHeadNode callStartSequenceNode;
@Child private CallDispatchHeadNode callEndSequenceNode;
@Child private CallDispatchHeadNode callStartMappingNode;
@Child private CallDispatchHeadNode callEndMappingNode;
@Child private CallDispatchHeadNode callEndStreamNode;
@Child private SnippetNode raiseSyntaxErrorSnippetNode;
@Child private SnippetNode tagPushNode;
@Child private TaintNode taintNode;
private final BranchProfile errorProfile;
Parse1Node_(ParseNodeGen root, SnippetNode taintedNode, DoesRespondDispatchHeadNode respondToReadNode, DoesRespondDispatchHeadNode respondToPathNode, CallDispatchHeadNode callPathNode, ReadObjectFieldNode readHandlerNode, CallDispatchHeadNode callStartStreamNode, CallDispatchHeadNode callStartDocumentNode, CallDispatchHeadNode callEndDocumentNode, CallDispatchHeadNode callAliasNode, CallDispatchHeadNode callScalarNode, CallDispatchHeadNode callStartSequenceNode, CallDispatchHeadNode callEndSequenceNode, CallDispatchHeadNode callStartMappingNode, CallDispatchHeadNode callEndMappingNode, CallDispatchHeadNode callEndStreamNode, SnippetNode raiseSyntaxErrorSnippetNode, SnippetNode tagPushNode, TaintNode taintNode, BranchProfile errorProfile) {
super(root, 2);
this.taintedNode = taintedNode;
this.respondToReadNode = respondToReadNode;
this.respondToPathNode = respondToPathNode;
this.callPathNode = callPathNode;
this.readHandlerNode = readHandlerNode;
this.callStartStreamNode = callStartStreamNode;
this.callStartDocumentNode = callStartDocumentNode;
this.callEndDocumentNode = callEndDocumentNode;
this.callAliasNode = callAliasNode;
this.callScalarNode = callScalarNode;
this.callStartSequenceNode = callStartSequenceNode;
this.callEndSequenceNode = callEndSequenceNode;
this.callStartMappingNode = callStartMappingNode;
this.callEndMappingNode = callEndMappingNode;
this.callEndStreamNode = callEndStreamNode;
this.raiseSyntaxErrorSnippetNode = raiseSyntaxErrorSnippetNode;
this.tagPushNode = tagPushNode;
this.taintNode = taintNode;
this.errorProfile = errorProfile;
}
@Override
public Object execute1(VirtualFrame frameValue, DynamicObject arguments0Value, DynamicObject arguments1Value, Object arguments2Value) {
if (arguments2Value instanceof DynamicObject) {
DynamicObject arguments2Value_ = (DynamicObject) arguments2Value;
return root.parse(frameValue, arguments0Value, arguments1Value, arguments2Value_, this.taintedNode, this.respondToReadNode, this.respondToPathNode, this.callPathNode, this.readHandlerNode, this.callStartStreamNode, this.callStartDocumentNode, this.callEndDocumentNode, this.callAliasNode, this.callScalarNode, this.callStartSequenceNode, this.callEndSequenceNode, this.callStartMappingNode, this.callEndMappingNode, this.callEndStreamNode, this.raiseSyntaxErrorSnippetNode, this.tagPushNode, this.taintNode, this.errorProfile);
}
return getNext().execute1(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
@Override
public Object execute_(VirtualFrame frameValue, Object arguments0Value, Object arguments1Value, Object arguments2Value) {
if (arguments0Value instanceof DynamicObject && arguments1Value instanceof DynamicObject && arguments2Value instanceof DynamicObject) {
DynamicObject arguments0Value_ = (DynamicObject) arguments0Value;
DynamicObject arguments1Value_ = (DynamicObject) arguments1Value;
DynamicObject arguments2Value_ = (DynamicObject) arguments2Value;
return root.parse(frameValue, arguments0Value_, arguments1Value_, arguments2Value_, this.taintedNode, this.respondToReadNode, this.respondToPathNode, this.callPathNode, this.readHandlerNode, this.callStartStreamNode, this.callStartDocumentNode, this.callEndDocumentNode, this.callAliasNode, this.callScalarNode, this.callStartSequenceNode, this.callEndSequenceNode, this.callStartMappingNode, this.callEndMappingNode, this.callEndStreamNode, this.raiseSyntaxErrorSnippetNode, this.tagPushNode, this.taintNode, this.errorProfile);
}
return getNext().execute_(frameValue, arguments0Value, arguments1Value, arguments2Value);
}
static BaseNode_ create(ParseNodeGen root, SnippetNode taintedNode, DoesRespondDispatchHeadNode respondToReadNode, DoesRespondDispatchHeadNode respondToPathNode, CallDispatchHeadNode callPathNode, ReadObjectFieldNode readHandlerNode, CallDispatchHeadNode callStartStreamNode, CallDispatchHeadNode callStartDocumentNode, CallDispatchHeadNode callEndDocumentNode, CallDispatchHeadNode callAliasNode, CallDispatchHeadNode callScalarNode, CallDispatchHeadNode callStartSequenceNode, CallDispatchHeadNode callEndSequenceNode, CallDispatchHeadNode callStartMappingNode, CallDispatchHeadNode callEndMappingNode, CallDispatchHeadNode callEndStreamNode, SnippetNode raiseSyntaxErrorSnippetNode, SnippetNode tagPushNode, TaintNode taintNode, BranchProfile errorProfile) {
return new Parse1Node_(root, taintedNode, respondToReadNode, respondToPathNode, callPathNode, readHandlerNode, callStartStreamNode, callStartDocumentNode, callEndDocumentNode, callAliasNode, callScalarNode, callStartSequenceNode, callEndSequenceNode, callStartMappingNode, callEndMappingNode, callEndStreamNode, raiseSyntaxErrorSnippetNode, tagPushNode, taintNode, errorProfile);
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy