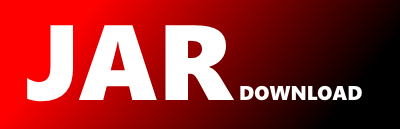
org.jruby.truffle.tools.ChaosNodeGen Maven / Gradle / Ivy
The newest version!
// CheckStyle: start generated
package org.jruby.truffle.tools;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.internal.SpecializationNode;
import com.oracle.truffle.api.dsl.internal.SpecializedNode;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.nodes.UnexpectedResultException;
import org.jruby.truffle.language.RubyNode;
import org.jruby.truffle.language.RubyTypesGen;
@GeneratedBy(ChaosNode.class)
public final class ChaosNodeGen extends ChaosNode implements SpecializedNode {
@Child private RubyNode child0_;
@CompilationFinal private Class> child0Type_;
@Child private BaseNode_ specialization_;
private ChaosNodeGen(RubyNode child0) {
this.child0_ = child0;
this.specialization_ = UninitializedNode_.create(this);
}
@Override
public NodeCost getCost() {
return specialization_.getNodeCost();
}
@Override
public Object execute(VirtualFrame frameValue) {
return specialization_.execute(frameValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
specialization_.executeVoid(frameValue);
return;
}
@Override
public SpecializationNode getSpecializationNode() {
return specialization_;
}
@Override
public Node deepCopy() {
return SpecializationNode.updateRoot(super.deepCopy());
}
public static ChaosNode create(RubyNode child0) {
return new ChaosNodeGen(child0);
}
@GeneratedBy(ChaosNode.class)
private abstract static class BaseNode_ extends SpecializationNode {
@CompilationFinal protected ChaosNodeGen root;
BaseNode_(ChaosNodeGen root, int index) {
super(index);
this.root = root;
}
@Override
protected final void setRoot(Node root) {
this.root = (ChaosNodeGen) root;
}
@Override
protected final Node[] getSuppliedChildren() {
return new Node[] {root.child0_};
}
@Override
public final Object acceptAndExecute(Frame frameValue, Object child0Value) {
return this.execute_((VirtualFrame) frameValue, child0Value);
}
public abstract Object execute_(VirtualFrame frameValue, Object child0Value);
public Object execute(VirtualFrame frameValue) {
Object child0Value_ = executeChild0_(frameValue);
return execute_(frameValue, child0Value_);
}
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
@Override
protected final SpecializationNode createNext(Frame frameValue, Object child0Value) {
if (RubyTypesGen.isImplicitInteger(child0Value)) {
return ChaosNode_.create(root, child0Value);
}
return null;
}
@Override
protected final SpecializationNode createFallback() {
return FallbackNode_.create(root);
}
@Override
protected final SpecializationNode createPolymorphic() {
return PolymorphicNode_.create(root);
}
protected final BaseNode_ getNext() {
return (BaseNode_) this.next;
}
protected final Object executeChild0_(Frame frameValue) {
Class> child0Type_ = root.child0Type_;
try {
if (child0Type_ == int.class) {
return root.child0_.executeInteger((VirtualFrame) frameValue);
} else if (child0Type_ == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Class> _type = Object.class;
try {
Object _value = root.child0_.execute((VirtualFrame) frameValue);
if (_value instanceof Integer) {
_type = int.class;
} else {
_type = Object.class;
}
return _value;
} finally {
root.child0Type_ = _type;
}
} else {
return root.child0_.execute((VirtualFrame) frameValue);
}
} catch (UnexpectedResultException ex) {
root.child0Type_ = Object.class;
return ex.getResult();
}
}
}
@GeneratedBy(ChaosNode.class)
private static final class UninitializedNode_ extends BaseNode_ {
UninitializedNode_(ChaosNodeGen root) {
super(root, 2147483647);
}
@Override
public Object execute_(VirtualFrame frameValue, Object child0Value) {
return uninitialized(frameValue, child0Value);
}
static BaseNode_ create(ChaosNodeGen root) {
return new UninitializedNode_(root);
}
}
@GeneratedBy(ChaosNode.class)
private static final class PolymorphicNode_ extends BaseNode_ {
PolymorphicNode_(ChaosNodeGen root) {
super(root, 0);
}
@Override
public SpecializationNode merge(SpecializationNode newNode, Frame frameValue, Object child0Value) {
return polymorphicMerge(newNode, super.merge(newNode, frameValue, child0Value));
}
@Override
public Object execute_(VirtualFrame frameValue, Object child0Value) {
return getNext().execute_(frameValue, child0Value);
}
static BaseNode_ create(ChaosNodeGen root) {
return new PolymorphicNode_(root);
}
}
@GeneratedBy(methodName = "chaos(int)", value = ChaosNode.class)
private static final class ChaosNode_ extends BaseNode_ {
private final Class> child0ImplicitType;
ChaosNode_(ChaosNodeGen root, Object child0Value) {
super(root, 1);
this.child0ImplicitType = RubyTypesGen.getImplicitIntegerClass(child0Value);
}
@Override
public boolean isSame(SpecializationNode other) {
return super.isSame(other) && this.child0ImplicitType == ((ChaosNode_) other).child0ImplicitType;
}
@Override
public Object execute(VirtualFrame frameValue) {
int child0Value_;
try {
if (child0ImplicitType == int.class) {
child0Value_ = root.child0_.executeInteger(frameValue);
} else {
Object child0Value__ = executeChild0_(frameValue);
child0Value_ = RubyTypesGen.expectImplicitInteger(child0Value__, child0ImplicitType);
}
} catch (UnexpectedResultException ex) {
return getNext().execute_(frameValue, ex.getResult());
}
return root.chaos(child0Value_);
}
@Override
public Object execute_(VirtualFrame frameValue, Object child0Value) {
if (RubyTypesGen.isImplicitInteger(child0Value, child0ImplicitType)) {
int child0Value_ = RubyTypesGen.asImplicitInteger(child0Value, child0ImplicitType);
return root.chaos(child0Value_);
}
return getNext().execute_(frameValue, child0Value);
}
static BaseNode_ create(ChaosNodeGen root, Object child0Value) {
return new ChaosNode_(root, child0Value);
}
}
@GeneratedBy(methodName = "chaos(Object)", value = ChaosNode.class)
private static final class FallbackNode_ extends BaseNode_ {
FallbackNode_(ChaosNodeGen root) {
super(root, 2147483646);
}
@TruffleBoundary
private boolean guardFallback(Object child0Value) {
return createNext(null, child0Value) == null;
}
@Override
public Object execute_(VirtualFrame frameValue, Object child0Value) {
if (guardFallback(child0Value)) {
return root.chaos(child0Value);
}
return getNext().execute_(frameValue, child0Value);
}
static BaseNode_ create(ChaosNodeGen root) {
return new FallbackNode_(root);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy