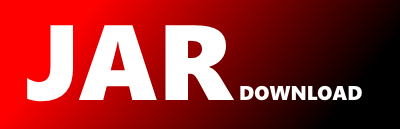
org.jsl.collider.DataBlockCache Maven / Gradle / Ivy
/*
* Copyright (C) 2015 Sergey Zubarev, [email protected]
*
* This file is a part of JS-Collider framework tests.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.jsl.collider;
import java.nio.ByteBuffer;
import java.util.concurrent.locks.ReentrantLock;
import java.util.logging.Level;
import java.util.logging.Logger;
public class DataBlockCache
{
private final boolean m_useDirectBuffers;
private final int m_blockSize;
private final int m_initialSize;
private final int m_maxSize;
private final ReentrantLock m_lock;
private DataBlock m_dataBlock;
private int m_size;
private DataBlock createDataBlock()
{
final ByteBuffer byteBuffer =
m_useDirectBuffers
? ByteBuffer.allocateDirect( m_blockSize )
: ByteBuffer.allocate( m_blockSize );
return new DataBlock( byteBuffer );
}
public DataBlockCache( boolean useDirectBuffers, int blockSize, int initialSize, int maxSize )
{
m_useDirectBuffers = useDirectBuffers;
m_blockSize = blockSize;
m_initialSize = initialSize;
m_maxSize = maxSize;
m_lock = new ReentrantLock();
m_dataBlock = null;
m_size = initialSize;
for (int idx=0; idx0; cnt--)
{
dataBlock.next = createDataBlock();
dataBlock = dataBlock.next;
}
return ret;
}
public final DataBlock getByDataSize( int dataSize )
{
int blocks = (dataSize / m_blockSize);
if ((dataSize % m_blockSize) > 0)
blocks++;
return get( blocks );
}
public final void clear( Logger logger )
{
int size = 0;
while (m_dataBlock != null)
{
final DataBlock next = m_dataBlock.next;
m_dataBlock.next = null;
m_dataBlock = next;
size++;
}
if (size != m_size)
{
if (logger.isLoggable(Level.WARNING))
{
logger.warning(
getClass().getSimpleName() +
"[" + m_blockSize + "] internal error: real size " +
size + " != " + m_size + "." );
}
}
if (size < m_initialSize)
{
if (logger.isLoggable(Level.WARNING))
{
logger.warning(
getClass().getSimpleName() +
"[" + m_blockSize + "] resource leak detected: current size " +
size + " less than initial size (" + m_initialSize + ")." );
}
}
else if (size > m_maxSize)
{
if (logger.isLoggable(Level.WARNING))
{
logger.warning(
getClass().getSimpleName() +
"[" + m_blockSize + "] internal error: current size " +
size + " is greater than maximum size (" + m_maxSize + ")." );
}
}
else
{
if (logger.isLoggable(Level.FINE))
{
logger.fine(
getClass().getSimpleName() +
"[" + m_blockSize + "] size=" + size + "." );
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy