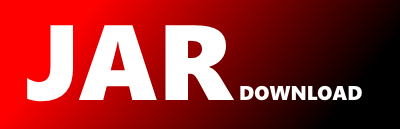
javax.measure.VectorMeasure Maven / Gradle / Ivy
/*
* JScience - Java(TM) Tools and Libraries for the Advancement of Sciences.
* Copyright (C) 2007 - JScience (http://jscience.org/)
* All rights reserved.
*
* Permission to use, copy, modify, and distribute this software is
* freely granted, provided that this notice is preserved.
*/
package javax.measure;
import javax.measure.converter.UnitConverter;
import javax.measure.quantity.Quantity;
import javax.measure.unit.CompoundUnit;
import javax.measure.unit.Unit;
/**
* This class represents a measurement vector of two or more dimensions.
* For example:[code]
* VectorMeasure dimension = VectorMeasure.valueOf(12.0, 30.0, 40.0, MILLIMETER);
* VectorMeasure v2d = VectorMeasure.valueOf(-2.2, -3.0, KNOTS);
* VectorMeasure c2d = VectorMeasure.valueOf(-7.3, 3.5, NANOAMPERE);
* [/code]
*
*
* Subclasses may provide fixed dimensions specializations:[code]
* class Velocity2D extends VectorMeasure {
* public Velocity2D(double x, double y, Unit unit) {
* ...
* }
* }
* [/code]
*
* Measurement vectors may use {@link CompoundUnit compound units}:[code]
* VectorMeasure latLong = VectorMeasure.valueOf(12.345, 22.23, DEGREE_ANGLE);
* Unit HOUR_MINUTE_SECOND_ANGLE = DEGREE_ANGLE.compound(MINUTE_ANGLE).compound(SECOND_ANGLE);
* System.out.println(latLong.to(HOUR_MINUTE_SECOND_ANGLE));
*
* > [12°19'42", 22°12'48"] [/code]
*
* Instances of this class (and sub-classes) are immutable.
*
* @author Jean-Marie Dautelle
* @version 4.3, October 3, 2007
*/
public abstract class VectorMeasure extends Measure {
/**
* Default constructor (for sub-classes).
*/
protected VectorMeasure() {
}
/**
* Returns a 2-dimensional measurement vector.
*
* @param x the first vector component value.
* @param y the second vector component value.
* @param unit the measurement unit.
*/
public static VectorMeasure valueOf(
double x, double y, Unit unit) {
return new TwoDimensional(x, y, unit);
}
/**
* Returns a 3-dimensional measurement vector.
*
* @param x the first vector component value.
* @param y the second vector component value.
* @param z the third vector component value.
* @param unit the measurement unit.
*/
public static VectorMeasure valueOf(
double x, double y, double z, Unit unit) {
return new ThreeDimensional(x, y, z, unit);
}
/**
* Returns a multi-dimensional measurement vector.
*
* @param components the vector component values.
* @param unit the measurement unit.
*/
public static VectorMeasure valueOf(double[] components,
Unit unit) {
return new MultiDimensional(components, unit);
}
/**
* Returns the measurement vector equivalent to this one but stated in the
* specified unit.
*
* @param unit the new measurement unit.
* @return the vector measure stated in the specified unit.
*/
public abstract VectorMeasure to(Unit unit);
/**
* Returns the norm of this measurement vector stated in the specified
* unit.
*
* @param unit the unit in which the norm is stated.
* @return |this|
*/
public abstract double doubleValue(Unit unit);
/**
* Returns the String
representation of this measurement
* vector (for example [2.3 m/s, 5.6 m/s]
).
*
* @return the textual representation of the measurement vector.
*/
public String toString() {
double[] values = getValue();
Unit unit = getUnit();
StringBuffer tmp = new StringBuffer();
tmp.append('[');
for (double v : values) {
if (tmp.length() > 1) {
tmp.append(", ");
}
if (unit instanceof CompoundUnit) {
MeasureFormat.DEFAULT.formatCompound(v, unit, tmp, null);
} else {
tmp.append(v).append(" ").append(unit);
}
}
tmp.append("] ");
return tmp.toString();
}
// Holds 2-dimensional implementation.
private static class TwoDimensional extends VectorMeasure {
private final double _x;
private final double _y;
private final Unit _unit;
private TwoDimensional(double x, double y, Unit unit) {
_x = x;
_y = y;
_unit = unit;
}
@Override
public double doubleValue(Unit unit) {
double norm = Math.sqrt(_x * _x + _y * _y);
if ((unit == _unit) || (unit.equals(_unit)))
return norm;
return _unit.getConverterTo(unit).convert(norm);
}
@Override
public Unit getUnit() {
return _unit;
}
@Override
public double[] getValue() {
return new double[] { _x, _y };
}
@Override
public TwoDimensional to(Unit unit) {
if ((unit == _unit) || (unit.equals(_unit)))
return this;
UnitConverter cvtr = _unit.getConverterTo(unit);
return new TwoDimensional(cvtr.convert(_x), cvtr.convert(_y), unit);
}
private static final long serialVersionUID = 1L;
}
// Holds 3-dimensional implementation.
private static class ThreeDimensional extends VectorMeasure {
private final double _x;
private final double _y;
private final double _z;
private final Unit _unit;
private ThreeDimensional(double x, double y, double z, Unit unit) {
_x = x;
_y = y;
_z = z;
_unit = unit;
}
@Override
public double doubleValue(Unit unit) {
double norm = Math.sqrt(_x * _x + _y * _y + _z * _z);
if ((unit == _unit) || (unit.equals(_unit)))
return norm;
return _unit.getConverterTo(unit).convert(norm);
}
@Override
public Unit getUnit() {
return _unit;
}
@Override
public double[] getValue() {
return new double[] { _x, _y, _z };
}
@Override
public ThreeDimensional to(Unit unit) {
if ((unit == _unit) || (unit.equals(_unit)))
return this;
UnitConverter cvtr = _unit.getConverterTo(unit);
return new ThreeDimensional(cvtr.convert(_x), cvtr.convert(_y), cvtr.convert(_z), unit);
}
private static final long serialVersionUID = 1L;
}
// Holds multi-dimensional implementation.
private static class MultiDimensional extends VectorMeasure {
private final double[] _components;
private final Unit _unit;
private MultiDimensional(double[] components, Unit unit) {
_components = components.clone();
_unit = unit;
}
@Override
public double doubleValue(Unit unit) {
double normSquare = _components[0] * _components[0];
for (int i=1, n=_components.length; i < n;) {
double d = _components[i++];
normSquare += d * d;
}
if ((unit == _unit) || (unit.equals(_unit)))
return Math.sqrt(normSquare);
return _unit.getConverterTo(unit).convert(Math.sqrt(normSquare));
}
@Override
public Unit getUnit() {
return _unit;
}
@Override
public double[] getValue() {
return _components.clone();
}
@Override
public MultiDimensional to(Unit unit) {
if ((unit == _unit) || (unit.equals(_unit)))
return this;
UnitConverter cvtr = _unit.getConverterTo(unit);
double[] newValues = new double[_components.length];
for (int i=0; i < _components.length; i++) {
newValues[i] = cvtr.convert(_components[i]);
}
return new MultiDimensional(newValues, unit);
}
private static final long serialVersionUID = 1L;
}
}