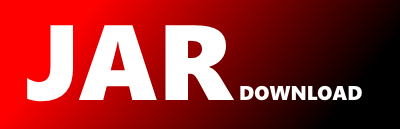
org.jscience.mathematics.function.RationalFunction Maven / Gradle / Ivy
The newest version!
/*
* JScience - Java(TM) Tools and Libraries for the Advancement of Sciences.
* Copyright (C) 2006 - JScience (http://jscience.org/)
* All rights reserved.
*
* Permission to use, copy, modify, and distribute this software is
* freely granted, provided that this notice is preserved.
*/
package org.jscience.mathematics.function;
import java.util.List;
import org.jscience.mathematics.structure.Field;
import javolution.context.ObjectFactory;
import javolution.text.Text;
import javolution.text.TextBuilder;
/**
* This class represents the quotient of two {@link Polynomial},
* it is also a {@link Field field} (invertible).
*
* @author Jean-Marie Dautelle
* @version 3.1, April 1, 2006
*/
public class RationalFunction> extends Function
implements Field> {
/**
* Holds the factory for rational functions.
*/
/**
* Holds the dividend.
*/
private Polynomial _dividend;
/**
* Holds the divisor.
*/
private Polynomial _divisor;
/**
* Default constructor.
*/
private RationalFunction() {
}
/**
* Returns the dividend of this rational function.
*
* @return this rational function dividend.
*/
public Polynomial getDividend() {
return _dividend;
}
/**
* Returns the divisor of this rational function.
*
* @return this rational function divisor.
*/
public Polynomial getDivisor() {
return _divisor;
}
/**
* Returns the rational function from the specified dividend and divisor.
*
* @param dividend the dividend value.
* @param divisor the divisor value.
* @return dividend / divisor
*/
@SuppressWarnings("unchecked")
public static > RationalFunction valueOf(
Polynomial dividend, Polynomial divisor) {
RationalFunction rf = FACTORY.object();
rf._dividend = dividend;
rf._divisor = divisor;
return rf;
}
@SuppressWarnings("unchecked")
private static final ObjectFactory FACTORY = new ObjectFactory() {
protected RationalFunction create() {
return new RationalFunction();
}
@SuppressWarnings("unchecked")
protected void cleanup(RationalFunction rf) {
rf._dividend = null;
rf._divisor = null;
}
};
/**
* Returns the sum of two rational functions.
*
* @param that the rational function being added.
* @return this + that
*/
public RationalFunction plus(RationalFunction that) {
return valueOf(this._dividend.times(that._divisor).plus(
this._divisor.times(that._dividend)), this._divisor
.times(that._divisor));
}
/**
* Returns the opposite of this rational function.
*
* @return - this
*/
public RationalFunction opposite() {
return valueOf(_dividend.opposite(), _divisor);
}
/**
* Returns the difference of two rational functions.
*
* @param that the rational function being subtracted.
* @return this - that
*/
public RationalFunction minus(RationalFunction that) {
return this.plus(that.opposite());
}
/**
* Returns the product of two rational functions.
*
* @param that the rational function multiplier.
* @return this · that
*/
public RationalFunction times(RationalFunction that) {
return valueOf(this._dividend.times(that._dividend), this._divisor
.times(that._divisor));
}
/**
* Returns the inverse of this rational function.
*
* @return 1 / this
*/
public RationalFunction inverse() {
return valueOf(_divisor, _dividend);
}
/**
* Returns the quotient of two rational functions.
*
* @param that the rational function divisor.
* @return this / that
*/
public RationalFunction divide(RationalFunction that) {
return this.times(that.inverse());
}
@SuppressWarnings("unchecked")
@Override
public List> getVariables() {
return merge(_dividend.getVariables(), _divisor.getVariables());
}
@SuppressWarnings("unchecked")
@Override
public F evaluate() {
return _dividend.evaluate().times(_divisor.evaluate().inverse());
}
@Override
public Text toText() {
TextBuilder tb = TextBuilder.newInstance();
tb.append('(');
tb.append(_dividend);
tb.append(")/(");
tb.append(_divisor);
tb.append(')');
return tb.toText();
}
@Override
public boolean equals(Object obj) {
if (obj instanceof RationalFunction) {
RationalFunction> that = (RationalFunction>) obj;
return this._dividend.equals(this._dividend)
&& this._divisor.equals(that._divisor);
} else {
return false;
}
}
@Override
public int hashCode() {
return _dividend.hashCode() - _divisor.hashCode();
}
//////////////////////////////////////////////////////////////////////
// Overrides parent method potentially returning rational functions //
//////////////////////////////////////////////////////////////////////
@Override
public RationalFunction differentiate(Variable v) {
return valueOf(_divisor.times(_dividend.differentiate(v)).plus(
_dividend.times(_divisor.differentiate(v)).opposite()),
_dividend.pow(2));
}
@SuppressWarnings("unchecked")
@Override
public Function plus(Function that) {
return (that instanceof RationalFunction) ?
this.plus((RationalFunction)that) : super.plus(that);
}
@SuppressWarnings("unchecked")
@Override
public Function minus(Function that) {
return (that instanceof RationalFunction) ?
this.minus((RationalFunction)that) : super.minus(that);
}
@SuppressWarnings("unchecked")
@Override
public Function times(Function that) {
return (that instanceof RationalFunction) ?
this.times((RationalFunction)that) : super.times(that);
}
@SuppressWarnings("unchecked")
@Override
public Function divide(Function that) {
return (that instanceof RationalFunction) ?
this.divide((RationalFunction)that) : super.divide(that);
}
@SuppressWarnings("unchecked")
@Override
public RationalFunction pow(int n) {
return (RationalFunction) super.pow(n);
}
/**
* Returns a copy of this rational function.
* {@link javolution.context.AllocatorContext allocated}
* by the calling thread (possibly on the stack).
*
* @return an identical and independant copy of this rational function.
*/
public RationalFunction copy() {
return RationalFunction.valueOf(_dividend.copy(), _divisor.copy());
}
private static final long serialVersionUID = 1L;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy