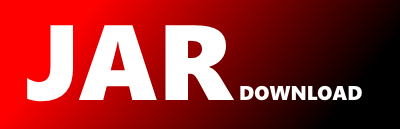
org.jsimpledb.cli.CliSession Maven / Gradle / Ivy
Show all versions of jsimpledb-cli Show documentation
/*
* Copyright (C) 2015 Archie L. Cobbs. All rights reserved.
*/
package org.jsimpledb.cli;
import com.google.common.base.Preconditions;
import java.io.PrintWriter;
import java.util.ArrayList;
import java.util.Map;
import java.util.SortedMap;
import java.util.TreeMap;
import org.jsimpledb.JSimpleDB;
import org.jsimpledb.Session;
import org.jsimpledb.cli.cmd.Command;
import org.jsimpledb.core.Database;
import org.jsimpledb.kv.KVDatabase;
import org.jsimpledb.parse.ParseException;
import org.jsimpledb.parse.ParseSession;
import org.jsimpledb.util.ImplementationsReader;
/**
* Represents one CLI console session.
*/
public class CliSession extends ParseSession {
/**
* Classpath XML file resource describing available {@link Command}s: {@value #CLI_COMMANDS_DESCRIPTOR_RESOURCE}.
*
*
* Example:
*
* <cli-command-implementations>
* <cli-command-implementation class="com.example.MyCliCommand"/>
* </cli-command-implementations>
*
*
*
* Instances must have a public constructor taking either zero parameters or one {@link CliSession} parameter.
*
* @see #loadCommandsFromClasspath
*/
public static final String CLI_COMMANDS_DESCRIPTOR_RESOURCE = "META-INF/jsimpledb/cli-command-implementations.xml";
private final Console console;
private final PrintWriter writer;
private final TreeMap commands = new TreeMap<>();
private boolean done;
private boolean verbose;
private int lineLimit = 16;
private String errorMessagePrefix = "Error: ";
// Constructors
/**
* Constructor for {@link org.jsimpledb.SessionMode#KEY_VALUE} mode.
*
* @param kvdb key/value database
* @param writer CLI output
* @param console associated console if any, otherwise null
* @throws IllegalArgumentException if {@code kvdb} or {@code writer} is null
*/
public CliSession(KVDatabase kvdb, PrintWriter writer, Console console) {
super(kvdb);
Preconditions.checkArgument(writer != null, "null writer");
this.writer = writer;
this.console = console;
}
/**
* Constructor for {@link org.jsimpledb.SessionMode#CORE_API} mode.
*
* @param db core database
* @param writer CLI output
* @param console associated console if any, otherwise null
* @throws IllegalArgumentException if {@code db} or {@code writer} is null
*/
public CliSession(Database db, PrintWriter writer, Console console) {
super(db);
Preconditions.checkArgument(writer != null, "null writer");
this.writer = writer;
this.console = console;
}
/**
* Constructor for {@link org.jsimpledb.SessionMode#JSIMPLEDB} mode.
*
* @param jdb database
* @param writer CLI output
* @param console associated console if any, otherwise null
* @throws IllegalArgumentException if {@code jdb} or {@code writer} is null
*/
public CliSession(JSimpleDB jdb, PrintWriter writer, Console console) {
super(jdb);
Preconditions.checkArgument(writer != null, "null writer");
this.writer = writer;
this.console = console;
}
// Accessors
/**
* Get the associated {@link Console}, if any.
*
* @return associated {@link Console}, or null if there is no console associated with this instance
*/
public Console getConsole() {
return this.console;
}
/**
* Get the output {@link PrintWriter} for this CLI session.
*
* @return output writer
*/
public PrintWriter getWriter() {
return this.writer;
}
/**
* Get the {@link Command}s registered with this instance.
*
* @return registered commands indexed by name
*/
public SortedMap getCommands() {
return this.commands;
}
public int getLineLimit() {
return this.lineLimit;
}
public void setLineLimit(int lineLimit) {
this.lineLimit = lineLimit;
}
public boolean isVerbose() {
return this.verbose;
}
public void setVerbose(boolean verbose) {
this.verbose = verbose;
}
public boolean isDone() {
return this.done;
}
public void setDone(boolean done) {
this.done = done;
}
// Command registration
/**
* Scan the classpath for {@link Command}s and register them.
*
* @see #CLI_COMMANDS_DESCRIPTOR_RESOURCE
*/
public void loadCommandsFromClasspath() {
final ImplementationsReader reader = new ImplementationsReader("cli-command");
final ArrayList