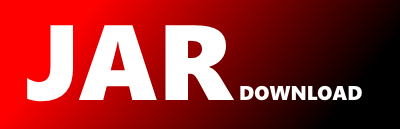
org.jsimpledb.spring.package-info Maven / Gradle / Ivy
Show all versions of jsimpledb-spring Show documentation
/*
* Copyright (C) 2015 Archie L. Cobbs. All rights reserved.
*/
/**
* Spring Framework integration classes.
*
* Overview
*
*
* This package provides the following features to facilitate use with
* Spring:
*
* - Custom XML tags for Spring declarative XML (see below)
* - A Spring {@link org.springframework.transaction.PlatformTransactionManager PlatformTransactionManager} that integrates
* into Spring's transaction infrastructure and enables the
* {@link org.springframework.transaction.annotation.Transactional @Transactional} annotation for
* {@link org.jsimpledb.JSimpleDB} transactions.
* - A {@link org.springframework.dao.support.PersistenceExceptionTranslator}
* {@linkplain org.jsimpledb.spring.JSimpleDBExceptionTranslator implementation} suitable for use with JSimpleDB
* - {@link org.jsimpledb.spring.OpenTransactionInViewFilter}, which allows {@link org.jsimpledb.JSimpleDB}
* transactions to span an entire web request.
* - Various {@link org.springframework.http.converter.HttpMessageConverter}'s that bring JSimpleDB's encoding,
* indexing, and schema management features to data being sent over a network:
*
* - {@link org.jsimpledb.spring.KVStoreHttpMessageConverter} for encoding/decoding a raw
* {@link org.jsimpledb.kv.KVStore}
* - {@link org.jsimpledb.spring.SnapshotJTransactionHttpMessageConverter} for encoding/decoding an entire
* {@link org.jsimpledb.SnapshotJTransaction}
* - {@link org.jsimpledb.spring.JObjectHttpMessageConverter} for encoding/decoding a specific
* {@link org.jsimpledb.JObject} within a {@link org.jsimpledb.SnapshotJTransaction}
*
*
*
*
* JSimpleDB XML Tags
*
*
* JSimpleDB makes available the following XML tags to Spring declarative XML. All elements live in the
* {@code http://jsimpledb.googlecode.com/schema/jsimpledb} XML namespace:
*
*
*
*
* Element
* Description
*
*
* {@code }
* Works just like Spring's {@code } but finds
* {@link org.jsimpledb.annotation.JSimpleClass @JSimpleClass}-annotated Java model classes.
* Returns the classes found in a {@link java.util.List}.
*
*
* {@code }
* Works just like Spring's {@code } but finds
* {@link org.jsimpledb.annotation.JFieldType @JFieldType}-annotated custom {@link org.jsimpledb.core.FieldType} classes.
* Returns the classes found in a {@link java.util.List}.
*
*
* {@code }
* Simplifies defining and configuring a {@link org.jsimpledb.JSimpleDB} database.
*
*
*
*
*
* The {@code } element requires a nested {@code } element to configure
* the Java model classes. A nested {@code } may also be included.
* The {@code } element supports the following attributes:
*
*
*
*
* Attribute
* Type
* Required?
* Description
*
*
* {@code kvstore}
* Bean reference
* No
* The underlying key/value store database. This should be the name of a Spring bean that implements
* the {@link org.jsimpledb.kv.KVDatabase} interface. If unset, defaults to a new
* {@link org.jsimpledb.kv.simple.SimpleKVDatabase} instance.
*
*
* {@code schema-version}
* Integer
* No
* The schema version corresponding to the configured Java model classes. A value of zero means to use
* whatever is the highest schema version already recorded in the database. A value of -1 (the default)
* means to {@linkplain org.jsimpledb.schema.SchemaModel#autogenerateVersion auto-generate} a version number
* based on the {@linkplain org.jsimpledb.schema.SchemaModel#compatibilityHash compatibility hash} of the
* {@link org.jsimpledb.schema.SchemaModel} generated from the Java model classes.
*
*
* {@code storage-id-generator}
* Bean reference
* No
* To use a custom {@link org.jsimpledb.StorageIdGenerator}, specify the name of a Spring bean that
* implements the {@link org.jsimpledb.StorageIdGenerator} interface. By default, a
* {@link org.jsimpledb.DefaultStorageIdGenerator} is used. If this attribute is set, then
* {@code auto-generate-storage-ids} must not be set to false.
*
*
* {@code auto-generate-storage-ids}
* Boolean
* No
* Whether to auto-generate storage ID's. Default is true
*
*
*
*
*
* An example Spring XML configuration using an in-memory key/value store and supporting Spring's
* {@link org.springframework.transaction.annotation.Transactional @Transactional} annotation:
*
* <beans xmlns="http://www.springframework.org/schema/beans"
* xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
* xmlns:jsimpledb="http://jsimpledb.googlecode.com/schema/jsimpledb"
* xmlns:tx="http://www.springframework.org/schema/tx"
* xmlns:p="http://www.springframework.org/schema/p"
* xmlns:c="http://www.springframework.org/schema/c"
* xsi:schemaLocation="
* http://jsimpledb.googlecode.com/schema/jsimpledb
* http://archiecobbs.github.io/jsimpledb/src/java/org/jsimpledb/spring/jsimpledb-1.0.xsd
* http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
* http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx.xsd">
*
* <!-- Define the underlying key/value database -->
* <bean id="kvdb" class="org.jsimpledb.kv.simple.SimpleKVDatabase" p:waitTimeout="5000" p:holdTimeout="10000"/>
*
* <!-- Define the JSimpleDB database on top of that -->
* <jsimpledb:jsimpledb id="jsimpledb" kvstore="kvdb">
*
* <!-- These are our Java model classes -->
* <jsimpledb:scan-classes base-package="com.example.myapp">
* <jsimpledb:exclude-filter type="regex" expression="com\.example\.myapp\.test\..*"/>
* </jsimpledb:scan-classes>
*
* <!-- We have some custom FieldType's here too -->
* <jsimpledb:scan-field-types base-package="com.example.myapp.fieldtype"/>
* </jsimpledb:jsimpledb>
*
* <!-- Create a JSimpleDB transaction manager -->
* <bean id="transactionManager" class="org.jsimpledb.spring.JSimpleDBTransactionManager"
* p:JSimpleDB-ref="jsimpledb" p:allowNewSchema="true"/>
*
* <!-- Enable @Transactional annotations -->
* <tx:annotation-driven transaction-manager="transactionManager"/>
*
* </beans>
*
*
* @see Spring Framework
*/
package org.jsimpledb.spring;