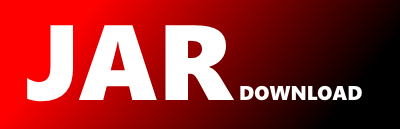
org.jsimpledb.util.ConvertedEntrySet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jsimpledb-util Show documentation
Show all versions of jsimpledb-util Show documentation
Common utility classes used by JSimpleDB.
The newest version!
/*
* Copyright (C) 2015 Archie L. Cobbs. All rights reserved.
*/
package org.jsimpledb.util;
import com.google.common.base.Converter;
import com.google.common.base.Preconditions;
import java.util.Map;
/**
* Converts a map's entry set.
*
* @param key type of this map
* @param value type of this map
* @param key type of wrapped map
* @param value type of wrapped map
*/
class ConvertedEntrySet extends ConvertedSet, Map.Entry> {
private final Map map;
private final Converter keyConverter;
private final Converter valueConverter;
/**
* Constructor.
*
* @param set wrapped set
* @param converter element converter
* @throws IllegalArgumentException if any parameter is null
*/
ConvertedEntrySet(Map map, Converter keyConverter, Converter valueConverter) {
super(map.entrySet(), new MapEntryConverter(keyConverter, valueConverter));
Preconditions.checkArgument(keyConverter != null, "null keyConverter");
Preconditions.checkArgument(valueConverter != null, "null valueConverter");
this.map = map;
this.keyConverter = keyConverter;
this.valueConverter = valueConverter;
}
public Converter getKeyConverter() {
return this.keyConverter;
}
public Converter getValueConverter() {
return this.valueConverter;
}
@Override
@SuppressWarnings("unchecked")
public boolean contains(Object obj) {
// Check type
if (!(obj instanceof Map.Entry))
return false;
final Map.Entry, ?> entry = (Map.Entry, ?>)obj;
// Convert key to wrapped key
final K key = (K)entry.getKey();
WK wkey = null;
if (key != null) {
try {
wkey = this.keyConverter.convert(key);
} catch (ClassCastException e) {
return false;
}
}
// Get corresponding wrapped value, if any, and unwrap it
final WV wvalue = this.map.get(wkey);
if (wvalue == null && !this.map.containsKey(wkey))
return false;
final V value = this.valueConverter.reverse().convert(wvalue);
// Compare original value to unwrapped value
return value != null ? value.equals(entry.getValue()) : entry.getValue() == null;
}
@Override
public boolean add(Map.Entry entry) {
throw new UnsupportedOperationException();
}
@Override
@SuppressWarnings("unchecked")
public boolean remove(Object obj) {
// Check type
if (!(obj instanceof Map.Entry))
return false;
final Map.Entry, ?> entry = (Map.Entry, ?>)obj;
// Convert key to wrapped key
final K key = (K)entry.getKey();
WK wkey = null;
if (key != null) {
try {
wkey = this.keyConverter.convert(key);
} catch (ClassCastException e) {
return false;
}
}
// Get corresponding wrapped value, if any, and unwrap it
final WV wvalue = this.map.get(wkey);
if (wvalue == null && !this.map.containsKey(wkey))
return false;
final V value = this.valueConverter.reverse().convert(wvalue);
// Compare original value to unwrapped value and remove entry if it matches
if (value != null ? value.equals(entry.getValue()) : entry.getValue() == null) {
this.map.remove(wkey);
return true;
}
// Not found
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy