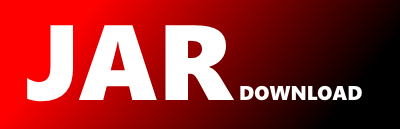
org.jsimpledb.vaadin.ReferenceMethodInfoCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jsimpledb-vaadin Show documentation
Show all versions of jsimpledb-vaadin Show documentation
JSimpleDB classes supporting Vaadin applications.
/*
* Copyright (C) 2015 Archie L. Cobbs. All rights reserved.
*/
package org.jsimpledb.vaadin;
import com.google.common.base.Preconditions;
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.CacheLoader;
import com.google.common.cache.LoadingCache;
import com.google.common.collect.Iterables;
import org.dellroad.stuff.vaadin7.PropertyDef;
import org.dellroad.stuff.vaadin7.PropertyExtractor;
import org.dellroad.stuff.vaadin7.ProvidesPropertyScanner;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Caches {@link org.dellroad.stuff.vaadin7.ProvidesProperty @ProvidesProperty}{@code(}{@link
* JObjectContainer#REFERENCE_LABEL_PROPERTY}{@code )} methods for Java classes.
*/
final class ReferenceMethodInfoCache {
static final PropertyInfo NOT_FOUND = new PropertyInfo<>(null, null);
private static final ReferenceMethodInfoCache INSTANCE = new ReferenceMethodInfoCache();
private final Logger log = LoggerFactory.getLogger(this.getClass());
private final LoadingCache, PropertyInfo>> cache = CacheBuilder.newBuilder().weakKeys().build(
new CacheLoader, PropertyInfo>>() {
@Override
public PropertyInfo> load(Class> type) {
return ReferenceMethodInfoCache.this.findReferenceLablePropertyInfo(type);
}
});
private ReferenceMethodInfoCache() {
}
/**
* Get the singleton instance.
*/
public static ReferenceMethodInfoCache getInstance() {
return ReferenceMethodInfoCache.INSTANCE;
}
/**
* Get property definition and extractor for the reference label method in the specified class, if any.
*
* @throws IllegalArgumentException if {@code type} is null
*/
@SuppressWarnings("unchecked")
public PropertyInfo getReferenceMethodInfo(Class type) {
return (PropertyInfo)this.cache.getUnchecked(type);
}
@SuppressWarnings("unchecked")
private PropertyInfo findReferenceLablePropertyInfo(Class type) {
Preconditions.checkArgument(type != null, "null type");
final ProvidesPropertyScanner scanner = new ProvidesPropertyScanner(type);
final PropertyDef> propertyDef = Iterables.find(scanner.getPropertyDefs(),
pdef -> pdef.getName().equals(JObjectContainer.REFERENCE_LABEL_PROPERTY), null);
return propertyDef != null ? new PropertyInfo(propertyDef, scanner.getPropertyExtractor()) : (PropertyInfo)NOT_FOUND;
}
// PropertyInfo
public static class PropertyInfo {
private final PropertyDef> propertyDef;
private final PropertyExtractor propertyExtractor;
PropertyInfo(PropertyDef> propertyDef, PropertyExtractor propertyExtractor) {
this.propertyDef = propertyDef;
this.propertyExtractor = propertyExtractor;
}
public PropertyDef> getPropertyDef() {
return this.propertyDef;
}
public PropertyExtractor getPropertyExtractor() {
return this.propertyExtractor;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy