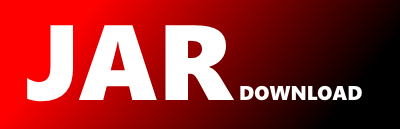
org.jsoftware.utils.chain.Chain Maven / Gradle / Ivy
The newest version!
package org.jsoftware.utils.chain;
/**
* Chain responsibility pattern implementation.
* @author m-szalik
* @param invocation context type
*/
public class Chain {
private final ChainCommandHolder first;
public Chain(ChainCommand... commands) {
if (commands != null && commands.length > 0) {
ChainCommandHolder prev = null;
for (int i = commands.length -1; i >= 0; i--) {
ChainCommand command = commands[i];
ChainCommandHolder current = new ChainCommandHolder<>(command, prev);
prev = current;
}
first = prev;
} else {
first = null;
}
}
public void execute(C context) throws Exception {
if (first != null) {
first.execute(context);
}
}
}
/**
* Holds command and it's successor
* @param Invocation context type
*/
class ChainCommandHolder implements DoChain {
private final ChainCommand command;
private final ChainCommandHolder next;
public ChainCommandHolder(ChainCommand command, ChainCommandHolder next) {
this.command = command;
this.next = next;
}
@Override
public void doContinue(C context) throws Exception {
if (next != null) {
next.execute(context);
}
}
void execute(C context) throws Exception {
command.execute(context, this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy