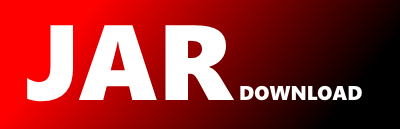
org.json4s.ReaderFunctions.scala Maven / Gradle / Ivy
package org.json4s
trait ReaderFunctions { self: Reader.type =>
def reader[A1, A2, X](f: (A1, A2) => X)(
key1: String, key2: String
)(implicit
A1: Reader[A1], A2: Reader[A2]
): Reader[X] =
reader2[A1, A2, X](f)(key1, key2)
def reader2[A1, A2, X](f: (A1, A2) => X)(
key1: String, key2: String
)(implicit
A1: Reader[A1], A2: Reader[A2]
): Reader[X] = Reader.from {
case json: JObject =>
val obj = json.obj.toMap
val a1 = obj.get(key1).toRight(new MappingException("field " + key1 + " not found")).flatMap(A1 readEither _)
val a2 = obj.get(key2).toRight(new MappingException("field " + key2 + " not found")).flatMap(A2 readEither _)
var lefts = List.empty[MappingException]
a1 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a2 match {
case Left(l) =>
lefts ::= l
case _ =>
}
if (lefts.isEmpty) {
Right(f(a1.asInstanceOf[Right[_, A1]].value, a2.asInstanceOf[Right[_, A2]].value))
} else {
Left(new MappingException.Multi(lefts.reverse, null))
}
case x =>
Left(new MappingException("JObject expected, but got " + x))
}
def reader[A1, A2, A3, X](f: (A1, A2, A3) => X)(
key1: String, key2: String, key3: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3]
): Reader[X] =
reader3[A1, A2, A3, X](f)(key1, key2, key3)
def reader3[A1, A2, A3, X](f: (A1, A2, A3) => X)(
key1: String, key2: String, key3: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3]
): Reader[X] = Reader.from {
case json: JObject =>
val obj = json.obj.toMap
val a1 = obj.get(key1).toRight(new MappingException("field " + key1 + " not found")).flatMap(A1 readEither _)
val a2 = obj.get(key2).toRight(new MappingException("field " + key2 + " not found")).flatMap(A2 readEither _)
val a3 = obj.get(key3).toRight(new MappingException("field " + key3 + " not found")).flatMap(A3 readEither _)
var lefts = List.empty[MappingException]
a1 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a2 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a3 match {
case Left(l) =>
lefts ::= l
case _ =>
}
if (lefts.isEmpty) {
Right(f(a1.asInstanceOf[Right[_, A1]].value, a2.asInstanceOf[Right[_, A2]].value, a3.asInstanceOf[Right[_, A3]].value))
} else {
Left(new MappingException.Multi(lefts.reverse, null))
}
case x =>
Left(new MappingException("JObject expected, but got " + x))
}
def reader[A1, A2, A3, A4, X](f: (A1, A2, A3, A4) => X)(
key1: String, key2: String, key3: String, key4: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4]
): Reader[X] =
reader4[A1, A2, A3, A4, X](f)(key1, key2, key3, key4)
def reader4[A1, A2, A3, A4, X](f: (A1, A2, A3, A4) => X)(
key1: String, key2: String, key3: String, key4: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4]
): Reader[X] = Reader.from {
case json: JObject =>
val obj = json.obj.toMap
val a1 = obj.get(key1).toRight(new MappingException("field " + key1 + " not found")).flatMap(A1 readEither _)
val a2 = obj.get(key2).toRight(new MappingException("field " + key2 + " not found")).flatMap(A2 readEither _)
val a3 = obj.get(key3).toRight(new MappingException("field " + key3 + " not found")).flatMap(A3 readEither _)
val a4 = obj.get(key4).toRight(new MappingException("field " + key4 + " not found")).flatMap(A4 readEither _)
var lefts = List.empty[MappingException]
a1 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a2 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a3 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a4 match {
case Left(l) =>
lefts ::= l
case _ =>
}
if (lefts.isEmpty) {
Right(f(a1.asInstanceOf[Right[_, A1]].value, a2.asInstanceOf[Right[_, A2]].value, a3.asInstanceOf[Right[_, A3]].value, a4.asInstanceOf[Right[_, A4]].value))
} else {
Left(new MappingException.Multi(lefts.reverse, null))
}
case x =>
Left(new MappingException("JObject expected, but got " + x))
}
def reader[A1, A2, A3, A4, A5, X](f: (A1, A2, A3, A4, A5) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5]
): Reader[X] =
reader5[A1, A2, A3, A4, A5, X](f)(key1, key2, key3, key4, key5)
def reader5[A1, A2, A3, A4, A5, X](f: (A1, A2, A3, A4, A5) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5]
): Reader[X] = Reader.from {
case json: JObject =>
val obj = json.obj.toMap
val a1 = obj.get(key1).toRight(new MappingException("field " + key1 + " not found")).flatMap(A1 readEither _)
val a2 = obj.get(key2).toRight(new MappingException("field " + key2 + " not found")).flatMap(A2 readEither _)
val a3 = obj.get(key3).toRight(new MappingException("field " + key3 + " not found")).flatMap(A3 readEither _)
val a4 = obj.get(key4).toRight(new MappingException("field " + key4 + " not found")).flatMap(A4 readEither _)
val a5 = obj.get(key5).toRight(new MappingException("field " + key5 + " not found")).flatMap(A5 readEither _)
var lefts = List.empty[MappingException]
a1 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a2 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a3 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a4 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a5 match {
case Left(l) =>
lefts ::= l
case _ =>
}
if (lefts.isEmpty) {
Right(f(a1.asInstanceOf[Right[_, A1]].value, a2.asInstanceOf[Right[_, A2]].value, a3.asInstanceOf[Right[_, A3]].value, a4.asInstanceOf[Right[_, A4]].value, a5.asInstanceOf[Right[_, A5]].value))
} else {
Left(new MappingException.Multi(lefts.reverse, null))
}
case x =>
Left(new MappingException("JObject expected, but got " + x))
}
def reader[A1, A2, A3, A4, A5, A6, X](f: (A1, A2, A3, A4, A5, A6) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6]
): Reader[X] =
reader6[A1, A2, A3, A4, A5, A6, X](f)(key1, key2, key3, key4, key5, key6)
def reader6[A1, A2, A3, A4, A5, A6, X](f: (A1, A2, A3, A4, A5, A6) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6]
): Reader[X] = Reader.from {
case json: JObject =>
val obj = json.obj.toMap
val a1 = obj.get(key1).toRight(new MappingException("field " + key1 + " not found")).flatMap(A1 readEither _)
val a2 = obj.get(key2).toRight(new MappingException("field " + key2 + " not found")).flatMap(A2 readEither _)
val a3 = obj.get(key3).toRight(new MappingException("field " + key3 + " not found")).flatMap(A3 readEither _)
val a4 = obj.get(key4).toRight(new MappingException("field " + key4 + " not found")).flatMap(A4 readEither _)
val a5 = obj.get(key5).toRight(new MappingException("field " + key5 + " not found")).flatMap(A5 readEither _)
val a6 = obj.get(key6).toRight(new MappingException("field " + key6 + " not found")).flatMap(A6 readEither _)
var lefts = List.empty[MappingException]
a1 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a2 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a3 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a4 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a5 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a6 match {
case Left(l) =>
lefts ::= l
case _ =>
}
if (lefts.isEmpty) {
Right(f(a1.asInstanceOf[Right[_, A1]].value, a2.asInstanceOf[Right[_, A2]].value, a3.asInstanceOf[Right[_, A3]].value, a4.asInstanceOf[Right[_, A4]].value, a5.asInstanceOf[Right[_, A5]].value, a6.asInstanceOf[Right[_, A6]].value))
} else {
Left(new MappingException.Multi(lefts.reverse, null))
}
case x =>
Left(new MappingException("JObject expected, but got " + x))
}
def reader[A1, A2, A3, A4, A5, A6, A7, X](f: (A1, A2, A3, A4, A5, A6, A7) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7]
): Reader[X] =
reader7[A1, A2, A3, A4, A5, A6, A7, X](f)(key1, key2, key3, key4, key5, key6, key7)
def reader7[A1, A2, A3, A4, A5, A6, A7, X](f: (A1, A2, A3, A4, A5, A6, A7) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7]
): Reader[X] = Reader.from {
case json: JObject =>
val obj = json.obj.toMap
val a1 = obj.get(key1).toRight(new MappingException("field " + key1 + " not found")).flatMap(A1 readEither _)
val a2 = obj.get(key2).toRight(new MappingException("field " + key2 + " not found")).flatMap(A2 readEither _)
val a3 = obj.get(key3).toRight(new MappingException("field " + key3 + " not found")).flatMap(A3 readEither _)
val a4 = obj.get(key4).toRight(new MappingException("field " + key4 + " not found")).flatMap(A4 readEither _)
val a5 = obj.get(key5).toRight(new MappingException("field " + key5 + " not found")).flatMap(A5 readEither _)
val a6 = obj.get(key6).toRight(new MappingException("field " + key6 + " not found")).flatMap(A6 readEither _)
val a7 = obj.get(key7).toRight(new MappingException("field " + key7 + " not found")).flatMap(A7 readEither _)
var lefts = List.empty[MappingException]
a1 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a2 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a3 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a4 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a5 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a6 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a7 match {
case Left(l) =>
lefts ::= l
case _ =>
}
if (lefts.isEmpty) {
Right(f(a1.asInstanceOf[Right[_, A1]].value, a2.asInstanceOf[Right[_, A2]].value, a3.asInstanceOf[Right[_, A3]].value, a4.asInstanceOf[Right[_, A4]].value, a5.asInstanceOf[Right[_, A5]].value, a6.asInstanceOf[Right[_, A6]].value, a7.asInstanceOf[Right[_, A7]].value))
} else {
Left(new MappingException.Multi(lefts.reverse, null))
}
case x =>
Left(new MappingException("JObject expected, but got " + x))
}
def reader[A1, A2, A3, A4, A5, A6, A7, A8, X](f: (A1, A2, A3, A4, A5, A6, A7, A8) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8]
): Reader[X] =
reader8[A1, A2, A3, A4, A5, A6, A7, A8, X](f)(key1, key2, key3, key4, key5, key6, key7, key8)
def reader8[A1, A2, A3, A4, A5, A6, A7, A8, X](f: (A1, A2, A3, A4, A5, A6, A7, A8) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8]
): Reader[X] = Reader.from {
case json: JObject =>
val obj = json.obj.toMap
val a1 = obj.get(key1).toRight(new MappingException("field " + key1 + " not found")).flatMap(A1 readEither _)
val a2 = obj.get(key2).toRight(new MappingException("field " + key2 + " not found")).flatMap(A2 readEither _)
val a3 = obj.get(key3).toRight(new MappingException("field " + key3 + " not found")).flatMap(A3 readEither _)
val a4 = obj.get(key4).toRight(new MappingException("field " + key4 + " not found")).flatMap(A4 readEither _)
val a5 = obj.get(key5).toRight(new MappingException("field " + key5 + " not found")).flatMap(A5 readEither _)
val a6 = obj.get(key6).toRight(new MappingException("field " + key6 + " not found")).flatMap(A6 readEither _)
val a7 = obj.get(key7).toRight(new MappingException("field " + key7 + " not found")).flatMap(A7 readEither _)
val a8 = obj.get(key8).toRight(new MappingException("field " + key8 + " not found")).flatMap(A8 readEither _)
var lefts = List.empty[MappingException]
a1 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a2 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a3 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a4 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a5 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a6 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a7 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a8 match {
case Left(l) =>
lefts ::= l
case _ =>
}
if (lefts.isEmpty) {
Right(f(a1.asInstanceOf[Right[_, A1]].value, a2.asInstanceOf[Right[_, A2]].value, a3.asInstanceOf[Right[_, A3]].value, a4.asInstanceOf[Right[_, A4]].value, a5.asInstanceOf[Right[_, A5]].value, a6.asInstanceOf[Right[_, A6]].value, a7.asInstanceOf[Right[_, A7]].value, a8.asInstanceOf[Right[_, A8]].value))
} else {
Left(new MappingException.Multi(lefts.reverse, null))
}
case x =>
Left(new MappingException("JObject expected, but got " + x))
}
def reader[A1, A2, A3, A4, A5, A6, A7, A8, A9, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9]
): Reader[X] =
reader9[A1, A2, A3, A4, A5, A6, A7, A8, A9, X](f)(key1, key2, key3, key4, key5, key6, key7, key8, key9)
def reader9[A1, A2, A3, A4, A5, A6, A7, A8, A9, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9]
): Reader[X] = Reader.from {
case json: JObject =>
val obj = json.obj.toMap
val a1 = obj.get(key1).toRight(new MappingException("field " + key1 + " not found")).flatMap(A1 readEither _)
val a2 = obj.get(key2).toRight(new MappingException("field " + key2 + " not found")).flatMap(A2 readEither _)
val a3 = obj.get(key3).toRight(new MappingException("field " + key3 + " not found")).flatMap(A3 readEither _)
val a4 = obj.get(key4).toRight(new MappingException("field " + key4 + " not found")).flatMap(A4 readEither _)
val a5 = obj.get(key5).toRight(new MappingException("field " + key5 + " not found")).flatMap(A5 readEither _)
val a6 = obj.get(key6).toRight(new MappingException("field " + key6 + " not found")).flatMap(A6 readEither _)
val a7 = obj.get(key7).toRight(new MappingException("field " + key7 + " not found")).flatMap(A7 readEither _)
val a8 = obj.get(key8).toRight(new MappingException("field " + key8 + " not found")).flatMap(A8 readEither _)
val a9 = obj.get(key9).toRight(new MappingException("field " + key9 + " not found")).flatMap(A9 readEither _)
var lefts = List.empty[MappingException]
a1 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a2 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a3 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a4 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a5 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a6 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a7 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a8 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a9 match {
case Left(l) =>
lefts ::= l
case _ =>
}
if (lefts.isEmpty) {
Right(f(a1.asInstanceOf[Right[_, A1]].value, a2.asInstanceOf[Right[_, A2]].value, a3.asInstanceOf[Right[_, A3]].value, a4.asInstanceOf[Right[_, A4]].value, a5.asInstanceOf[Right[_, A5]].value, a6.asInstanceOf[Right[_, A6]].value, a7.asInstanceOf[Right[_, A7]].value, a8.asInstanceOf[Right[_, A8]].value, a9.asInstanceOf[Right[_, A9]].value))
} else {
Left(new MappingException.Multi(lefts.reverse, null))
}
case x =>
Left(new MappingException("JObject expected, but got " + x))
}
def reader[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10]
): Reader[X] =
reader10[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, X](f)(key1, key2, key3, key4, key5, key6, key7, key8, key9, key10)
def reader10[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10]
): Reader[X] = Reader.from {
case json: JObject =>
val obj = json.obj.toMap
val a1 = obj.get(key1).toRight(new MappingException("field " + key1 + " not found")).flatMap(A1 readEither _)
val a2 = obj.get(key2).toRight(new MappingException("field " + key2 + " not found")).flatMap(A2 readEither _)
val a3 = obj.get(key3).toRight(new MappingException("field " + key3 + " not found")).flatMap(A3 readEither _)
val a4 = obj.get(key4).toRight(new MappingException("field " + key4 + " not found")).flatMap(A4 readEither _)
val a5 = obj.get(key5).toRight(new MappingException("field " + key5 + " not found")).flatMap(A5 readEither _)
val a6 = obj.get(key6).toRight(new MappingException("field " + key6 + " not found")).flatMap(A6 readEither _)
val a7 = obj.get(key7).toRight(new MappingException("field " + key7 + " not found")).flatMap(A7 readEither _)
val a8 = obj.get(key8).toRight(new MappingException("field " + key8 + " not found")).flatMap(A8 readEither _)
val a9 = obj.get(key9).toRight(new MappingException("field " + key9 + " not found")).flatMap(A9 readEither _)
val a10 = obj.get(key10).toRight(new MappingException("field " + key10 + " not found")).flatMap(A10 readEither _)
var lefts = List.empty[MappingException]
a1 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a2 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a3 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a4 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a5 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a6 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a7 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a8 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a9 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a10 match {
case Left(l) =>
lefts ::= l
case _ =>
}
if (lefts.isEmpty) {
Right(f(a1.asInstanceOf[Right[_, A1]].value, a2.asInstanceOf[Right[_, A2]].value, a3.asInstanceOf[Right[_, A3]].value, a4.asInstanceOf[Right[_, A4]].value, a5.asInstanceOf[Right[_, A5]].value, a6.asInstanceOf[Right[_, A6]].value, a7.asInstanceOf[Right[_, A7]].value, a8.asInstanceOf[Right[_, A8]].value, a9.asInstanceOf[Right[_, A9]].value, a10.asInstanceOf[Right[_, A10]].value))
} else {
Left(new MappingException.Multi(lefts.reverse, null))
}
case x =>
Left(new MappingException("JObject expected, but got " + x))
}
def reader[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11]
): Reader[X] =
reader11[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, X](f)(key1, key2, key3, key4, key5, key6, key7, key8, key9, key10, key11)
def reader11[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11]
): Reader[X] = Reader.from {
case json: JObject =>
val obj = json.obj.toMap
val a1 = obj.get(key1).toRight(new MappingException("field " + key1 + " not found")).flatMap(A1 readEither _)
val a2 = obj.get(key2).toRight(new MappingException("field " + key2 + " not found")).flatMap(A2 readEither _)
val a3 = obj.get(key3).toRight(new MappingException("field " + key3 + " not found")).flatMap(A3 readEither _)
val a4 = obj.get(key4).toRight(new MappingException("field " + key4 + " not found")).flatMap(A4 readEither _)
val a5 = obj.get(key5).toRight(new MappingException("field " + key5 + " not found")).flatMap(A5 readEither _)
val a6 = obj.get(key6).toRight(new MappingException("field " + key6 + " not found")).flatMap(A6 readEither _)
val a7 = obj.get(key7).toRight(new MappingException("field " + key7 + " not found")).flatMap(A7 readEither _)
val a8 = obj.get(key8).toRight(new MappingException("field " + key8 + " not found")).flatMap(A8 readEither _)
val a9 = obj.get(key9).toRight(new MappingException("field " + key9 + " not found")).flatMap(A9 readEither _)
val a10 = obj.get(key10).toRight(new MappingException("field " + key10 + " not found")).flatMap(A10 readEither _)
val a11 = obj.get(key11).toRight(new MappingException("field " + key11 + " not found")).flatMap(A11 readEither _)
var lefts = List.empty[MappingException]
a1 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a2 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a3 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a4 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a5 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a6 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a7 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a8 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a9 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a10 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a11 match {
case Left(l) =>
lefts ::= l
case _ =>
}
if (lefts.isEmpty) {
Right(f(a1.asInstanceOf[Right[_, A1]].value, a2.asInstanceOf[Right[_, A2]].value, a3.asInstanceOf[Right[_, A3]].value, a4.asInstanceOf[Right[_, A4]].value, a5.asInstanceOf[Right[_, A5]].value, a6.asInstanceOf[Right[_, A6]].value, a7.asInstanceOf[Right[_, A7]].value, a8.asInstanceOf[Right[_, A8]].value, a9.asInstanceOf[Right[_, A9]].value, a10.asInstanceOf[Right[_, A10]].value, a11.asInstanceOf[Right[_, A11]].value))
} else {
Left(new MappingException.Multi(lefts.reverse, null))
}
case x =>
Left(new MappingException("JObject expected, but got " + x))
}
def reader[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String, key12: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11], A12: Reader[A12]
): Reader[X] =
reader12[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, X](f)(key1, key2, key3, key4, key5, key6, key7, key8, key9, key10, key11, key12)
def reader12[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String, key12: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11], A12: Reader[A12]
): Reader[X] = Reader.from {
case json: JObject =>
val obj = json.obj.toMap
val a1 = obj.get(key1).toRight(new MappingException("field " + key1 + " not found")).flatMap(A1 readEither _)
val a2 = obj.get(key2).toRight(new MappingException("field " + key2 + " not found")).flatMap(A2 readEither _)
val a3 = obj.get(key3).toRight(new MappingException("field " + key3 + " not found")).flatMap(A3 readEither _)
val a4 = obj.get(key4).toRight(new MappingException("field " + key4 + " not found")).flatMap(A4 readEither _)
val a5 = obj.get(key5).toRight(new MappingException("field " + key5 + " not found")).flatMap(A5 readEither _)
val a6 = obj.get(key6).toRight(new MappingException("field " + key6 + " not found")).flatMap(A6 readEither _)
val a7 = obj.get(key7).toRight(new MappingException("field " + key7 + " not found")).flatMap(A7 readEither _)
val a8 = obj.get(key8).toRight(new MappingException("field " + key8 + " not found")).flatMap(A8 readEither _)
val a9 = obj.get(key9).toRight(new MappingException("field " + key9 + " not found")).flatMap(A9 readEither _)
val a10 = obj.get(key10).toRight(new MappingException("field " + key10 + " not found")).flatMap(A10 readEither _)
val a11 = obj.get(key11).toRight(new MappingException("field " + key11 + " not found")).flatMap(A11 readEither _)
val a12 = obj.get(key12).toRight(new MappingException("field " + key12 + " not found")).flatMap(A12 readEither _)
var lefts = List.empty[MappingException]
a1 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a2 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a3 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a4 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a5 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a6 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a7 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a8 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a9 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a10 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a11 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a12 match {
case Left(l) =>
lefts ::= l
case _ =>
}
if (lefts.isEmpty) {
Right(f(a1.asInstanceOf[Right[_, A1]].value, a2.asInstanceOf[Right[_, A2]].value, a3.asInstanceOf[Right[_, A3]].value, a4.asInstanceOf[Right[_, A4]].value, a5.asInstanceOf[Right[_, A5]].value, a6.asInstanceOf[Right[_, A6]].value, a7.asInstanceOf[Right[_, A7]].value, a8.asInstanceOf[Right[_, A8]].value, a9.asInstanceOf[Right[_, A9]].value, a10.asInstanceOf[Right[_, A10]].value, a11.asInstanceOf[Right[_, A11]].value, a12.asInstanceOf[Right[_, A12]].value))
} else {
Left(new MappingException.Multi(lefts.reverse, null))
}
case x =>
Left(new MappingException("JObject expected, but got " + x))
}
def reader[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String, key12: String, key13: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11], A12: Reader[A12], A13: Reader[A13]
): Reader[X] =
reader13[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, X](f)(key1, key2, key3, key4, key5, key6, key7, key8, key9, key10, key11, key12, key13)
def reader13[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String, key12: String, key13: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11], A12: Reader[A12], A13: Reader[A13]
): Reader[X] = Reader.from {
case json: JObject =>
val obj = json.obj.toMap
val a1 = obj.get(key1).toRight(new MappingException("field " + key1 + " not found")).flatMap(A1 readEither _)
val a2 = obj.get(key2).toRight(new MappingException("field " + key2 + " not found")).flatMap(A2 readEither _)
val a3 = obj.get(key3).toRight(new MappingException("field " + key3 + " not found")).flatMap(A3 readEither _)
val a4 = obj.get(key4).toRight(new MappingException("field " + key4 + " not found")).flatMap(A4 readEither _)
val a5 = obj.get(key5).toRight(new MappingException("field " + key5 + " not found")).flatMap(A5 readEither _)
val a6 = obj.get(key6).toRight(new MappingException("field " + key6 + " not found")).flatMap(A6 readEither _)
val a7 = obj.get(key7).toRight(new MappingException("field " + key7 + " not found")).flatMap(A7 readEither _)
val a8 = obj.get(key8).toRight(new MappingException("field " + key8 + " not found")).flatMap(A8 readEither _)
val a9 = obj.get(key9).toRight(new MappingException("field " + key9 + " not found")).flatMap(A9 readEither _)
val a10 = obj.get(key10).toRight(new MappingException("field " + key10 + " not found")).flatMap(A10 readEither _)
val a11 = obj.get(key11).toRight(new MappingException("field " + key11 + " not found")).flatMap(A11 readEither _)
val a12 = obj.get(key12).toRight(new MappingException("field " + key12 + " not found")).flatMap(A12 readEither _)
val a13 = obj.get(key13).toRight(new MappingException("field " + key13 + " not found")).flatMap(A13 readEither _)
var lefts = List.empty[MappingException]
a1 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a2 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a3 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a4 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a5 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a6 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a7 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a8 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a9 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a10 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a11 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a12 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a13 match {
case Left(l) =>
lefts ::= l
case _ =>
}
if (lefts.isEmpty) {
Right(f(a1.asInstanceOf[Right[_, A1]].value, a2.asInstanceOf[Right[_, A2]].value, a3.asInstanceOf[Right[_, A3]].value, a4.asInstanceOf[Right[_, A4]].value, a5.asInstanceOf[Right[_, A5]].value, a6.asInstanceOf[Right[_, A6]].value, a7.asInstanceOf[Right[_, A7]].value, a8.asInstanceOf[Right[_, A8]].value, a9.asInstanceOf[Right[_, A9]].value, a10.asInstanceOf[Right[_, A10]].value, a11.asInstanceOf[Right[_, A11]].value, a12.asInstanceOf[Right[_, A12]].value, a13.asInstanceOf[Right[_, A13]].value))
} else {
Left(new MappingException.Multi(lefts.reverse, null))
}
case x =>
Left(new MappingException("JObject expected, but got " + x))
}
def reader[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String, key12: String, key13: String, key14: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11], A12: Reader[A12], A13: Reader[A13], A14: Reader[A14]
): Reader[X] =
reader14[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, X](f)(key1, key2, key3, key4, key5, key6, key7, key8, key9, key10, key11, key12, key13, key14)
def reader14[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String, key12: String, key13: String, key14: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11], A12: Reader[A12], A13: Reader[A13], A14: Reader[A14]
): Reader[X] = Reader.from {
case json: JObject =>
val obj = json.obj.toMap
val a1 = obj.get(key1).toRight(new MappingException("field " + key1 + " not found")).flatMap(A1 readEither _)
val a2 = obj.get(key2).toRight(new MappingException("field " + key2 + " not found")).flatMap(A2 readEither _)
val a3 = obj.get(key3).toRight(new MappingException("field " + key3 + " not found")).flatMap(A3 readEither _)
val a4 = obj.get(key4).toRight(new MappingException("field " + key4 + " not found")).flatMap(A4 readEither _)
val a5 = obj.get(key5).toRight(new MappingException("field " + key5 + " not found")).flatMap(A5 readEither _)
val a6 = obj.get(key6).toRight(new MappingException("field " + key6 + " not found")).flatMap(A6 readEither _)
val a7 = obj.get(key7).toRight(new MappingException("field " + key7 + " not found")).flatMap(A7 readEither _)
val a8 = obj.get(key8).toRight(new MappingException("field " + key8 + " not found")).flatMap(A8 readEither _)
val a9 = obj.get(key9).toRight(new MappingException("field " + key9 + " not found")).flatMap(A9 readEither _)
val a10 = obj.get(key10).toRight(new MappingException("field " + key10 + " not found")).flatMap(A10 readEither _)
val a11 = obj.get(key11).toRight(new MappingException("field " + key11 + " not found")).flatMap(A11 readEither _)
val a12 = obj.get(key12).toRight(new MappingException("field " + key12 + " not found")).flatMap(A12 readEither _)
val a13 = obj.get(key13).toRight(new MappingException("field " + key13 + " not found")).flatMap(A13 readEither _)
val a14 = obj.get(key14).toRight(new MappingException("field " + key14 + " not found")).flatMap(A14 readEither _)
var lefts = List.empty[MappingException]
a1 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a2 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a3 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a4 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a5 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a6 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a7 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a8 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a9 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a10 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a11 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a12 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a13 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a14 match {
case Left(l) =>
lefts ::= l
case _ =>
}
if (lefts.isEmpty) {
Right(f(a1.asInstanceOf[Right[_, A1]].value, a2.asInstanceOf[Right[_, A2]].value, a3.asInstanceOf[Right[_, A3]].value, a4.asInstanceOf[Right[_, A4]].value, a5.asInstanceOf[Right[_, A5]].value, a6.asInstanceOf[Right[_, A6]].value, a7.asInstanceOf[Right[_, A7]].value, a8.asInstanceOf[Right[_, A8]].value, a9.asInstanceOf[Right[_, A9]].value, a10.asInstanceOf[Right[_, A10]].value, a11.asInstanceOf[Right[_, A11]].value, a12.asInstanceOf[Right[_, A12]].value, a13.asInstanceOf[Right[_, A13]].value, a14.asInstanceOf[Right[_, A14]].value))
} else {
Left(new MappingException.Multi(lefts.reverse, null))
}
case x =>
Left(new MappingException("JObject expected, but got " + x))
}
def reader[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String, key12: String, key13: String, key14: String, key15: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11], A12: Reader[A12], A13: Reader[A13], A14: Reader[A14], A15: Reader[A15]
): Reader[X] =
reader15[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, X](f)(key1, key2, key3, key4, key5, key6, key7, key8, key9, key10, key11, key12, key13, key14, key15)
def reader15[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String, key12: String, key13: String, key14: String, key15: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11], A12: Reader[A12], A13: Reader[A13], A14: Reader[A14], A15: Reader[A15]
): Reader[X] = Reader.from {
case json: JObject =>
val obj = json.obj.toMap
val a1 = obj.get(key1).toRight(new MappingException("field " + key1 + " not found")).flatMap(A1 readEither _)
val a2 = obj.get(key2).toRight(new MappingException("field " + key2 + " not found")).flatMap(A2 readEither _)
val a3 = obj.get(key3).toRight(new MappingException("field " + key3 + " not found")).flatMap(A3 readEither _)
val a4 = obj.get(key4).toRight(new MappingException("field " + key4 + " not found")).flatMap(A4 readEither _)
val a5 = obj.get(key5).toRight(new MappingException("field " + key5 + " not found")).flatMap(A5 readEither _)
val a6 = obj.get(key6).toRight(new MappingException("field " + key6 + " not found")).flatMap(A6 readEither _)
val a7 = obj.get(key7).toRight(new MappingException("field " + key7 + " not found")).flatMap(A7 readEither _)
val a8 = obj.get(key8).toRight(new MappingException("field " + key8 + " not found")).flatMap(A8 readEither _)
val a9 = obj.get(key9).toRight(new MappingException("field " + key9 + " not found")).flatMap(A9 readEither _)
val a10 = obj.get(key10).toRight(new MappingException("field " + key10 + " not found")).flatMap(A10 readEither _)
val a11 = obj.get(key11).toRight(new MappingException("field " + key11 + " not found")).flatMap(A11 readEither _)
val a12 = obj.get(key12).toRight(new MappingException("field " + key12 + " not found")).flatMap(A12 readEither _)
val a13 = obj.get(key13).toRight(new MappingException("field " + key13 + " not found")).flatMap(A13 readEither _)
val a14 = obj.get(key14).toRight(new MappingException("field " + key14 + " not found")).flatMap(A14 readEither _)
val a15 = obj.get(key15).toRight(new MappingException("field " + key15 + " not found")).flatMap(A15 readEither _)
var lefts = List.empty[MappingException]
a1 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a2 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a3 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a4 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a5 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a6 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a7 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a8 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a9 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a10 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a11 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a12 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a13 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a14 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a15 match {
case Left(l) =>
lefts ::= l
case _ =>
}
if (lefts.isEmpty) {
Right(f(a1.asInstanceOf[Right[_, A1]].value, a2.asInstanceOf[Right[_, A2]].value, a3.asInstanceOf[Right[_, A3]].value, a4.asInstanceOf[Right[_, A4]].value, a5.asInstanceOf[Right[_, A5]].value, a6.asInstanceOf[Right[_, A6]].value, a7.asInstanceOf[Right[_, A7]].value, a8.asInstanceOf[Right[_, A8]].value, a9.asInstanceOf[Right[_, A9]].value, a10.asInstanceOf[Right[_, A10]].value, a11.asInstanceOf[Right[_, A11]].value, a12.asInstanceOf[Right[_, A12]].value, a13.asInstanceOf[Right[_, A13]].value, a14.asInstanceOf[Right[_, A14]].value, a15.asInstanceOf[Right[_, A15]].value))
} else {
Left(new MappingException.Multi(lefts.reverse, null))
}
case x =>
Left(new MappingException("JObject expected, but got " + x))
}
def reader[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String, key12: String, key13: String, key14: String, key15: String, key16: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11], A12: Reader[A12], A13: Reader[A13], A14: Reader[A14], A15: Reader[A15], A16: Reader[A16]
): Reader[X] =
reader16[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, X](f)(key1, key2, key3, key4, key5, key6, key7, key8, key9, key10, key11, key12, key13, key14, key15, key16)
def reader16[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String, key12: String, key13: String, key14: String, key15: String, key16: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11], A12: Reader[A12], A13: Reader[A13], A14: Reader[A14], A15: Reader[A15], A16: Reader[A16]
): Reader[X] = Reader.from {
case json: JObject =>
val obj = json.obj.toMap
val a1 = obj.get(key1).toRight(new MappingException("field " + key1 + " not found")).flatMap(A1 readEither _)
val a2 = obj.get(key2).toRight(new MappingException("field " + key2 + " not found")).flatMap(A2 readEither _)
val a3 = obj.get(key3).toRight(new MappingException("field " + key3 + " not found")).flatMap(A3 readEither _)
val a4 = obj.get(key4).toRight(new MappingException("field " + key4 + " not found")).flatMap(A4 readEither _)
val a5 = obj.get(key5).toRight(new MappingException("field " + key5 + " not found")).flatMap(A5 readEither _)
val a6 = obj.get(key6).toRight(new MappingException("field " + key6 + " not found")).flatMap(A6 readEither _)
val a7 = obj.get(key7).toRight(new MappingException("field " + key7 + " not found")).flatMap(A7 readEither _)
val a8 = obj.get(key8).toRight(new MappingException("field " + key8 + " not found")).flatMap(A8 readEither _)
val a9 = obj.get(key9).toRight(new MappingException("field " + key9 + " not found")).flatMap(A9 readEither _)
val a10 = obj.get(key10).toRight(new MappingException("field " + key10 + " not found")).flatMap(A10 readEither _)
val a11 = obj.get(key11).toRight(new MappingException("field " + key11 + " not found")).flatMap(A11 readEither _)
val a12 = obj.get(key12).toRight(new MappingException("field " + key12 + " not found")).flatMap(A12 readEither _)
val a13 = obj.get(key13).toRight(new MappingException("field " + key13 + " not found")).flatMap(A13 readEither _)
val a14 = obj.get(key14).toRight(new MappingException("field " + key14 + " not found")).flatMap(A14 readEither _)
val a15 = obj.get(key15).toRight(new MappingException("field " + key15 + " not found")).flatMap(A15 readEither _)
val a16 = obj.get(key16).toRight(new MappingException("field " + key16 + " not found")).flatMap(A16 readEither _)
var lefts = List.empty[MappingException]
a1 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a2 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a3 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a4 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a5 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a6 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a7 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a8 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a9 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a10 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a11 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a12 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a13 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a14 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a15 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a16 match {
case Left(l) =>
lefts ::= l
case _ =>
}
if (lefts.isEmpty) {
Right(f(a1.asInstanceOf[Right[_, A1]].value, a2.asInstanceOf[Right[_, A2]].value, a3.asInstanceOf[Right[_, A3]].value, a4.asInstanceOf[Right[_, A4]].value, a5.asInstanceOf[Right[_, A5]].value, a6.asInstanceOf[Right[_, A6]].value, a7.asInstanceOf[Right[_, A7]].value, a8.asInstanceOf[Right[_, A8]].value, a9.asInstanceOf[Right[_, A9]].value, a10.asInstanceOf[Right[_, A10]].value, a11.asInstanceOf[Right[_, A11]].value, a12.asInstanceOf[Right[_, A12]].value, a13.asInstanceOf[Right[_, A13]].value, a14.asInstanceOf[Right[_, A14]].value, a15.asInstanceOf[Right[_, A15]].value, a16.asInstanceOf[Right[_, A16]].value))
} else {
Left(new MappingException.Multi(lefts.reverse, null))
}
case x =>
Left(new MappingException("JObject expected, but got " + x))
}
def reader[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String, key12: String, key13: String, key14: String, key15: String, key16: String, key17: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11], A12: Reader[A12], A13: Reader[A13], A14: Reader[A14], A15: Reader[A15], A16: Reader[A16], A17: Reader[A17]
): Reader[X] =
reader17[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, X](f)(key1, key2, key3, key4, key5, key6, key7, key8, key9, key10, key11, key12, key13, key14, key15, key16, key17)
def reader17[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String, key12: String, key13: String, key14: String, key15: String, key16: String, key17: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11], A12: Reader[A12], A13: Reader[A13], A14: Reader[A14], A15: Reader[A15], A16: Reader[A16], A17: Reader[A17]
): Reader[X] = Reader.from {
case json: JObject =>
val obj = json.obj.toMap
val a1 = obj.get(key1).toRight(new MappingException("field " + key1 + " not found")).flatMap(A1 readEither _)
val a2 = obj.get(key2).toRight(new MappingException("field " + key2 + " not found")).flatMap(A2 readEither _)
val a3 = obj.get(key3).toRight(new MappingException("field " + key3 + " not found")).flatMap(A3 readEither _)
val a4 = obj.get(key4).toRight(new MappingException("field " + key4 + " not found")).flatMap(A4 readEither _)
val a5 = obj.get(key5).toRight(new MappingException("field " + key5 + " not found")).flatMap(A5 readEither _)
val a6 = obj.get(key6).toRight(new MappingException("field " + key6 + " not found")).flatMap(A6 readEither _)
val a7 = obj.get(key7).toRight(new MappingException("field " + key7 + " not found")).flatMap(A7 readEither _)
val a8 = obj.get(key8).toRight(new MappingException("field " + key8 + " not found")).flatMap(A8 readEither _)
val a9 = obj.get(key9).toRight(new MappingException("field " + key9 + " not found")).flatMap(A9 readEither _)
val a10 = obj.get(key10).toRight(new MappingException("field " + key10 + " not found")).flatMap(A10 readEither _)
val a11 = obj.get(key11).toRight(new MappingException("field " + key11 + " not found")).flatMap(A11 readEither _)
val a12 = obj.get(key12).toRight(new MappingException("field " + key12 + " not found")).flatMap(A12 readEither _)
val a13 = obj.get(key13).toRight(new MappingException("field " + key13 + " not found")).flatMap(A13 readEither _)
val a14 = obj.get(key14).toRight(new MappingException("field " + key14 + " not found")).flatMap(A14 readEither _)
val a15 = obj.get(key15).toRight(new MappingException("field " + key15 + " not found")).flatMap(A15 readEither _)
val a16 = obj.get(key16).toRight(new MappingException("field " + key16 + " not found")).flatMap(A16 readEither _)
val a17 = obj.get(key17).toRight(new MappingException("field " + key17 + " not found")).flatMap(A17 readEither _)
var lefts = List.empty[MappingException]
a1 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a2 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a3 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a4 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a5 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a6 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a7 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a8 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a9 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a10 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a11 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a12 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a13 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a14 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a15 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a16 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a17 match {
case Left(l) =>
lefts ::= l
case _ =>
}
if (lefts.isEmpty) {
Right(f(a1.asInstanceOf[Right[_, A1]].value, a2.asInstanceOf[Right[_, A2]].value, a3.asInstanceOf[Right[_, A3]].value, a4.asInstanceOf[Right[_, A4]].value, a5.asInstanceOf[Right[_, A5]].value, a6.asInstanceOf[Right[_, A6]].value, a7.asInstanceOf[Right[_, A7]].value, a8.asInstanceOf[Right[_, A8]].value, a9.asInstanceOf[Right[_, A9]].value, a10.asInstanceOf[Right[_, A10]].value, a11.asInstanceOf[Right[_, A11]].value, a12.asInstanceOf[Right[_, A12]].value, a13.asInstanceOf[Right[_, A13]].value, a14.asInstanceOf[Right[_, A14]].value, a15.asInstanceOf[Right[_, A15]].value, a16.asInstanceOf[Right[_, A16]].value, a17.asInstanceOf[Right[_, A17]].value))
} else {
Left(new MappingException.Multi(lefts.reverse, null))
}
case x =>
Left(new MappingException("JObject expected, but got " + x))
}
def reader[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String, key12: String, key13: String, key14: String, key15: String, key16: String, key17: String, key18: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11], A12: Reader[A12], A13: Reader[A13], A14: Reader[A14], A15: Reader[A15], A16: Reader[A16], A17: Reader[A17], A18: Reader[A18]
): Reader[X] =
reader18[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, X](f)(key1, key2, key3, key4, key5, key6, key7, key8, key9, key10, key11, key12, key13, key14, key15, key16, key17, key18)
def reader18[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String, key12: String, key13: String, key14: String, key15: String, key16: String, key17: String, key18: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11], A12: Reader[A12], A13: Reader[A13], A14: Reader[A14], A15: Reader[A15], A16: Reader[A16], A17: Reader[A17], A18: Reader[A18]
): Reader[X] = Reader.from {
case json: JObject =>
val obj = json.obj.toMap
val a1 = obj.get(key1).toRight(new MappingException("field " + key1 + " not found")).flatMap(A1 readEither _)
val a2 = obj.get(key2).toRight(new MappingException("field " + key2 + " not found")).flatMap(A2 readEither _)
val a3 = obj.get(key3).toRight(new MappingException("field " + key3 + " not found")).flatMap(A3 readEither _)
val a4 = obj.get(key4).toRight(new MappingException("field " + key4 + " not found")).flatMap(A4 readEither _)
val a5 = obj.get(key5).toRight(new MappingException("field " + key5 + " not found")).flatMap(A5 readEither _)
val a6 = obj.get(key6).toRight(new MappingException("field " + key6 + " not found")).flatMap(A6 readEither _)
val a7 = obj.get(key7).toRight(new MappingException("field " + key7 + " not found")).flatMap(A7 readEither _)
val a8 = obj.get(key8).toRight(new MappingException("field " + key8 + " not found")).flatMap(A8 readEither _)
val a9 = obj.get(key9).toRight(new MappingException("field " + key9 + " not found")).flatMap(A9 readEither _)
val a10 = obj.get(key10).toRight(new MappingException("field " + key10 + " not found")).flatMap(A10 readEither _)
val a11 = obj.get(key11).toRight(new MappingException("field " + key11 + " not found")).flatMap(A11 readEither _)
val a12 = obj.get(key12).toRight(new MappingException("field " + key12 + " not found")).flatMap(A12 readEither _)
val a13 = obj.get(key13).toRight(new MappingException("field " + key13 + " not found")).flatMap(A13 readEither _)
val a14 = obj.get(key14).toRight(new MappingException("field " + key14 + " not found")).flatMap(A14 readEither _)
val a15 = obj.get(key15).toRight(new MappingException("field " + key15 + " not found")).flatMap(A15 readEither _)
val a16 = obj.get(key16).toRight(new MappingException("field " + key16 + " not found")).flatMap(A16 readEither _)
val a17 = obj.get(key17).toRight(new MappingException("field " + key17 + " not found")).flatMap(A17 readEither _)
val a18 = obj.get(key18).toRight(new MappingException("field " + key18 + " not found")).flatMap(A18 readEither _)
var lefts = List.empty[MappingException]
a1 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a2 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a3 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a4 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a5 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a6 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a7 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a8 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a9 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a10 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a11 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a12 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a13 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a14 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a15 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a16 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a17 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a18 match {
case Left(l) =>
lefts ::= l
case _ =>
}
if (lefts.isEmpty) {
Right(f(a1.asInstanceOf[Right[_, A1]].value, a2.asInstanceOf[Right[_, A2]].value, a3.asInstanceOf[Right[_, A3]].value, a4.asInstanceOf[Right[_, A4]].value, a5.asInstanceOf[Right[_, A5]].value, a6.asInstanceOf[Right[_, A6]].value, a7.asInstanceOf[Right[_, A7]].value, a8.asInstanceOf[Right[_, A8]].value, a9.asInstanceOf[Right[_, A9]].value, a10.asInstanceOf[Right[_, A10]].value, a11.asInstanceOf[Right[_, A11]].value, a12.asInstanceOf[Right[_, A12]].value, a13.asInstanceOf[Right[_, A13]].value, a14.asInstanceOf[Right[_, A14]].value, a15.asInstanceOf[Right[_, A15]].value, a16.asInstanceOf[Right[_, A16]].value, a17.asInstanceOf[Right[_, A17]].value, a18.asInstanceOf[Right[_, A18]].value))
} else {
Left(new MappingException.Multi(lefts.reverse, null))
}
case x =>
Left(new MappingException("JObject expected, but got " + x))
}
def reader[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, A19, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, A19) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String, key12: String, key13: String, key14: String, key15: String, key16: String, key17: String, key18: String, key19: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11], A12: Reader[A12], A13: Reader[A13], A14: Reader[A14], A15: Reader[A15], A16: Reader[A16], A17: Reader[A17], A18: Reader[A18], A19: Reader[A19]
): Reader[X] =
reader19[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, A19, X](f)(key1, key2, key3, key4, key5, key6, key7, key8, key9, key10, key11, key12, key13, key14, key15, key16, key17, key18, key19)
def reader19[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, A19, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, A19) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String, key12: String, key13: String, key14: String, key15: String, key16: String, key17: String, key18: String, key19: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11], A12: Reader[A12], A13: Reader[A13], A14: Reader[A14], A15: Reader[A15], A16: Reader[A16], A17: Reader[A17], A18: Reader[A18], A19: Reader[A19]
): Reader[X] = Reader.from {
case json: JObject =>
val obj = json.obj.toMap
val a1 = obj.get(key1).toRight(new MappingException("field " + key1 + " not found")).flatMap(A1 readEither _)
val a2 = obj.get(key2).toRight(new MappingException("field " + key2 + " not found")).flatMap(A2 readEither _)
val a3 = obj.get(key3).toRight(new MappingException("field " + key3 + " not found")).flatMap(A3 readEither _)
val a4 = obj.get(key4).toRight(new MappingException("field " + key4 + " not found")).flatMap(A4 readEither _)
val a5 = obj.get(key5).toRight(new MappingException("field " + key5 + " not found")).flatMap(A5 readEither _)
val a6 = obj.get(key6).toRight(new MappingException("field " + key6 + " not found")).flatMap(A6 readEither _)
val a7 = obj.get(key7).toRight(new MappingException("field " + key7 + " not found")).flatMap(A7 readEither _)
val a8 = obj.get(key8).toRight(new MappingException("field " + key8 + " not found")).flatMap(A8 readEither _)
val a9 = obj.get(key9).toRight(new MappingException("field " + key9 + " not found")).flatMap(A9 readEither _)
val a10 = obj.get(key10).toRight(new MappingException("field " + key10 + " not found")).flatMap(A10 readEither _)
val a11 = obj.get(key11).toRight(new MappingException("field " + key11 + " not found")).flatMap(A11 readEither _)
val a12 = obj.get(key12).toRight(new MappingException("field " + key12 + " not found")).flatMap(A12 readEither _)
val a13 = obj.get(key13).toRight(new MappingException("field " + key13 + " not found")).flatMap(A13 readEither _)
val a14 = obj.get(key14).toRight(new MappingException("field " + key14 + " not found")).flatMap(A14 readEither _)
val a15 = obj.get(key15).toRight(new MappingException("field " + key15 + " not found")).flatMap(A15 readEither _)
val a16 = obj.get(key16).toRight(new MappingException("field " + key16 + " not found")).flatMap(A16 readEither _)
val a17 = obj.get(key17).toRight(new MappingException("field " + key17 + " not found")).flatMap(A17 readEither _)
val a18 = obj.get(key18).toRight(new MappingException("field " + key18 + " not found")).flatMap(A18 readEither _)
val a19 = obj.get(key19).toRight(new MappingException("field " + key19 + " not found")).flatMap(A19 readEither _)
var lefts = List.empty[MappingException]
a1 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a2 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a3 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a4 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a5 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a6 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a7 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a8 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a9 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a10 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a11 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a12 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a13 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a14 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a15 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a16 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a17 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a18 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a19 match {
case Left(l) =>
lefts ::= l
case _ =>
}
if (lefts.isEmpty) {
Right(f(a1.asInstanceOf[Right[_, A1]].value, a2.asInstanceOf[Right[_, A2]].value, a3.asInstanceOf[Right[_, A3]].value, a4.asInstanceOf[Right[_, A4]].value, a5.asInstanceOf[Right[_, A5]].value, a6.asInstanceOf[Right[_, A6]].value, a7.asInstanceOf[Right[_, A7]].value, a8.asInstanceOf[Right[_, A8]].value, a9.asInstanceOf[Right[_, A9]].value, a10.asInstanceOf[Right[_, A10]].value, a11.asInstanceOf[Right[_, A11]].value, a12.asInstanceOf[Right[_, A12]].value, a13.asInstanceOf[Right[_, A13]].value, a14.asInstanceOf[Right[_, A14]].value, a15.asInstanceOf[Right[_, A15]].value, a16.asInstanceOf[Right[_, A16]].value, a17.asInstanceOf[Right[_, A17]].value, a18.asInstanceOf[Right[_, A18]].value, a19.asInstanceOf[Right[_, A19]].value))
} else {
Left(new MappingException.Multi(lefts.reverse, null))
}
case x =>
Left(new MappingException("JObject expected, but got " + x))
}
def reader[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, A19, A20, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, A19, A20) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String, key12: String, key13: String, key14: String, key15: String, key16: String, key17: String, key18: String, key19: String, key20: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11], A12: Reader[A12], A13: Reader[A13], A14: Reader[A14], A15: Reader[A15], A16: Reader[A16], A17: Reader[A17], A18: Reader[A18], A19: Reader[A19], A20: Reader[A20]
): Reader[X] =
reader20[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, A19, A20, X](f)(key1, key2, key3, key4, key5, key6, key7, key8, key9, key10, key11, key12, key13, key14, key15, key16, key17, key18, key19, key20)
def reader20[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, A19, A20, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, A19, A20) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String, key12: String, key13: String, key14: String, key15: String, key16: String, key17: String, key18: String, key19: String, key20: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11], A12: Reader[A12], A13: Reader[A13], A14: Reader[A14], A15: Reader[A15], A16: Reader[A16], A17: Reader[A17], A18: Reader[A18], A19: Reader[A19], A20: Reader[A20]
): Reader[X] = Reader.from {
case json: JObject =>
val obj = json.obj.toMap
val a1 = obj.get(key1).toRight(new MappingException("field " + key1 + " not found")).flatMap(A1 readEither _)
val a2 = obj.get(key2).toRight(new MappingException("field " + key2 + " not found")).flatMap(A2 readEither _)
val a3 = obj.get(key3).toRight(new MappingException("field " + key3 + " not found")).flatMap(A3 readEither _)
val a4 = obj.get(key4).toRight(new MappingException("field " + key4 + " not found")).flatMap(A4 readEither _)
val a5 = obj.get(key5).toRight(new MappingException("field " + key5 + " not found")).flatMap(A5 readEither _)
val a6 = obj.get(key6).toRight(new MappingException("field " + key6 + " not found")).flatMap(A6 readEither _)
val a7 = obj.get(key7).toRight(new MappingException("field " + key7 + " not found")).flatMap(A7 readEither _)
val a8 = obj.get(key8).toRight(new MappingException("field " + key8 + " not found")).flatMap(A8 readEither _)
val a9 = obj.get(key9).toRight(new MappingException("field " + key9 + " not found")).flatMap(A9 readEither _)
val a10 = obj.get(key10).toRight(new MappingException("field " + key10 + " not found")).flatMap(A10 readEither _)
val a11 = obj.get(key11).toRight(new MappingException("field " + key11 + " not found")).flatMap(A11 readEither _)
val a12 = obj.get(key12).toRight(new MappingException("field " + key12 + " not found")).flatMap(A12 readEither _)
val a13 = obj.get(key13).toRight(new MappingException("field " + key13 + " not found")).flatMap(A13 readEither _)
val a14 = obj.get(key14).toRight(new MappingException("field " + key14 + " not found")).flatMap(A14 readEither _)
val a15 = obj.get(key15).toRight(new MappingException("field " + key15 + " not found")).flatMap(A15 readEither _)
val a16 = obj.get(key16).toRight(new MappingException("field " + key16 + " not found")).flatMap(A16 readEither _)
val a17 = obj.get(key17).toRight(new MappingException("field " + key17 + " not found")).flatMap(A17 readEither _)
val a18 = obj.get(key18).toRight(new MappingException("field " + key18 + " not found")).flatMap(A18 readEither _)
val a19 = obj.get(key19).toRight(new MappingException("field " + key19 + " not found")).flatMap(A19 readEither _)
val a20 = obj.get(key20).toRight(new MappingException("field " + key20 + " not found")).flatMap(A20 readEither _)
var lefts = List.empty[MappingException]
a1 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a2 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a3 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a4 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a5 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a6 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a7 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a8 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a9 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a10 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a11 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a12 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a13 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a14 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a15 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a16 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a17 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a18 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a19 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a20 match {
case Left(l) =>
lefts ::= l
case _ =>
}
if (lefts.isEmpty) {
Right(f(a1.asInstanceOf[Right[_, A1]].value, a2.asInstanceOf[Right[_, A2]].value, a3.asInstanceOf[Right[_, A3]].value, a4.asInstanceOf[Right[_, A4]].value, a5.asInstanceOf[Right[_, A5]].value, a6.asInstanceOf[Right[_, A6]].value, a7.asInstanceOf[Right[_, A7]].value, a8.asInstanceOf[Right[_, A8]].value, a9.asInstanceOf[Right[_, A9]].value, a10.asInstanceOf[Right[_, A10]].value, a11.asInstanceOf[Right[_, A11]].value, a12.asInstanceOf[Right[_, A12]].value, a13.asInstanceOf[Right[_, A13]].value, a14.asInstanceOf[Right[_, A14]].value, a15.asInstanceOf[Right[_, A15]].value, a16.asInstanceOf[Right[_, A16]].value, a17.asInstanceOf[Right[_, A17]].value, a18.asInstanceOf[Right[_, A18]].value, a19.asInstanceOf[Right[_, A19]].value, a20.asInstanceOf[Right[_, A20]].value))
} else {
Left(new MappingException.Multi(lefts.reverse, null))
}
case x =>
Left(new MappingException("JObject expected, but got " + x))
}
def reader[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, A19, A20, A21, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, A19, A20, A21) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String, key12: String, key13: String, key14: String, key15: String, key16: String, key17: String, key18: String, key19: String, key20: String, key21: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11], A12: Reader[A12], A13: Reader[A13], A14: Reader[A14], A15: Reader[A15], A16: Reader[A16], A17: Reader[A17], A18: Reader[A18], A19: Reader[A19], A20: Reader[A20], A21: Reader[A21]
): Reader[X] =
reader21[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, A19, A20, A21, X](f)(key1, key2, key3, key4, key5, key6, key7, key8, key9, key10, key11, key12, key13, key14, key15, key16, key17, key18, key19, key20, key21)
def reader21[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, A19, A20, A21, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, A19, A20, A21) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String, key12: String, key13: String, key14: String, key15: String, key16: String, key17: String, key18: String, key19: String, key20: String, key21: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11], A12: Reader[A12], A13: Reader[A13], A14: Reader[A14], A15: Reader[A15], A16: Reader[A16], A17: Reader[A17], A18: Reader[A18], A19: Reader[A19], A20: Reader[A20], A21: Reader[A21]
): Reader[X] = Reader.from {
case json: JObject =>
val obj = json.obj.toMap
val a1 = obj.get(key1).toRight(new MappingException("field " + key1 + " not found")).flatMap(A1 readEither _)
val a2 = obj.get(key2).toRight(new MappingException("field " + key2 + " not found")).flatMap(A2 readEither _)
val a3 = obj.get(key3).toRight(new MappingException("field " + key3 + " not found")).flatMap(A3 readEither _)
val a4 = obj.get(key4).toRight(new MappingException("field " + key4 + " not found")).flatMap(A4 readEither _)
val a5 = obj.get(key5).toRight(new MappingException("field " + key5 + " not found")).flatMap(A5 readEither _)
val a6 = obj.get(key6).toRight(new MappingException("field " + key6 + " not found")).flatMap(A6 readEither _)
val a7 = obj.get(key7).toRight(new MappingException("field " + key7 + " not found")).flatMap(A7 readEither _)
val a8 = obj.get(key8).toRight(new MappingException("field " + key8 + " not found")).flatMap(A8 readEither _)
val a9 = obj.get(key9).toRight(new MappingException("field " + key9 + " not found")).flatMap(A9 readEither _)
val a10 = obj.get(key10).toRight(new MappingException("field " + key10 + " not found")).flatMap(A10 readEither _)
val a11 = obj.get(key11).toRight(new MappingException("field " + key11 + " not found")).flatMap(A11 readEither _)
val a12 = obj.get(key12).toRight(new MappingException("field " + key12 + " not found")).flatMap(A12 readEither _)
val a13 = obj.get(key13).toRight(new MappingException("field " + key13 + " not found")).flatMap(A13 readEither _)
val a14 = obj.get(key14).toRight(new MappingException("field " + key14 + " not found")).flatMap(A14 readEither _)
val a15 = obj.get(key15).toRight(new MappingException("field " + key15 + " not found")).flatMap(A15 readEither _)
val a16 = obj.get(key16).toRight(new MappingException("field " + key16 + " not found")).flatMap(A16 readEither _)
val a17 = obj.get(key17).toRight(new MappingException("field " + key17 + " not found")).flatMap(A17 readEither _)
val a18 = obj.get(key18).toRight(new MappingException("field " + key18 + " not found")).flatMap(A18 readEither _)
val a19 = obj.get(key19).toRight(new MappingException("field " + key19 + " not found")).flatMap(A19 readEither _)
val a20 = obj.get(key20).toRight(new MappingException("field " + key20 + " not found")).flatMap(A20 readEither _)
val a21 = obj.get(key21).toRight(new MappingException("field " + key21 + " not found")).flatMap(A21 readEither _)
var lefts = List.empty[MappingException]
a1 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a2 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a3 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a4 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a5 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a6 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a7 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a8 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a9 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a10 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a11 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a12 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a13 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a14 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a15 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a16 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a17 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a18 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a19 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a20 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a21 match {
case Left(l) =>
lefts ::= l
case _ =>
}
if (lefts.isEmpty) {
Right(f(a1.asInstanceOf[Right[_, A1]].value, a2.asInstanceOf[Right[_, A2]].value, a3.asInstanceOf[Right[_, A3]].value, a4.asInstanceOf[Right[_, A4]].value, a5.asInstanceOf[Right[_, A5]].value, a6.asInstanceOf[Right[_, A6]].value, a7.asInstanceOf[Right[_, A7]].value, a8.asInstanceOf[Right[_, A8]].value, a9.asInstanceOf[Right[_, A9]].value, a10.asInstanceOf[Right[_, A10]].value, a11.asInstanceOf[Right[_, A11]].value, a12.asInstanceOf[Right[_, A12]].value, a13.asInstanceOf[Right[_, A13]].value, a14.asInstanceOf[Right[_, A14]].value, a15.asInstanceOf[Right[_, A15]].value, a16.asInstanceOf[Right[_, A16]].value, a17.asInstanceOf[Right[_, A17]].value, a18.asInstanceOf[Right[_, A18]].value, a19.asInstanceOf[Right[_, A19]].value, a20.asInstanceOf[Right[_, A20]].value, a21.asInstanceOf[Right[_, A21]].value))
} else {
Left(new MappingException.Multi(lefts.reverse, null))
}
case x =>
Left(new MappingException("JObject expected, but got " + x))
}
def reader[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, A19, A20, A21, A22, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, A19, A20, A21, A22) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String, key12: String, key13: String, key14: String, key15: String, key16: String, key17: String, key18: String, key19: String, key20: String, key21: String, key22: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11], A12: Reader[A12], A13: Reader[A13], A14: Reader[A14], A15: Reader[A15], A16: Reader[A16], A17: Reader[A17], A18: Reader[A18], A19: Reader[A19], A20: Reader[A20], A21: Reader[A21], A22: Reader[A22]
): Reader[X] =
reader22[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, A19, A20, A21, A22, X](f)(key1, key2, key3, key4, key5, key6, key7, key8, key9, key10, key11, key12, key13, key14, key15, key16, key17, key18, key19, key20, key21, key22)
def reader22[A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, A19, A20, A21, A22, X](f: (A1, A2, A3, A4, A5, A6, A7, A8, A9, A10, A11, A12, A13, A14, A15, A16, A17, A18, A19, A20, A21, A22) => X)(
key1: String, key2: String, key3: String, key4: String, key5: String, key6: String, key7: String, key8: String, key9: String, key10: String, key11: String, key12: String, key13: String, key14: String, key15: String, key16: String, key17: String, key18: String, key19: String, key20: String, key21: String, key22: String
)(implicit
A1: Reader[A1], A2: Reader[A2], A3: Reader[A3], A4: Reader[A4], A5: Reader[A5], A6: Reader[A6], A7: Reader[A7], A8: Reader[A8], A9: Reader[A9], A10: Reader[A10], A11: Reader[A11], A12: Reader[A12], A13: Reader[A13], A14: Reader[A14], A15: Reader[A15], A16: Reader[A16], A17: Reader[A17], A18: Reader[A18], A19: Reader[A19], A20: Reader[A20], A21: Reader[A21], A22: Reader[A22]
): Reader[X] = Reader.from {
case json: JObject =>
val obj = json.obj.toMap
val a1 = obj.get(key1).toRight(new MappingException("field " + key1 + " not found")).flatMap(A1 readEither _)
val a2 = obj.get(key2).toRight(new MappingException("field " + key2 + " not found")).flatMap(A2 readEither _)
val a3 = obj.get(key3).toRight(new MappingException("field " + key3 + " not found")).flatMap(A3 readEither _)
val a4 = obj.get(key4).toRight(new MappingException("field " + key4 + " not found")).flatMap(A4 readEither _)
val a5 = obj.get(key5).toRight(new MappingException("field " + key5 + " not found")).flatMap(A5 readEither _)
val a6 = obj.get(key6).toRight(new MappingException("field " + key6 + " not found")).flatMap(A6 readEither _)
val a7 = obj.get(key7).toRight(new MappingException("field " + key7 + " not found")).flatMap(A7 readEither _)
val a8 = obj.get(key8).toRight(new MappingException("field " + key8 + " not found")).flatMap(A8 readEither _)
val a9 = obj.get(key9).toRight(new MappingException("field " + key9 + " not found")).flatMap(A9 readEither _)
val a10 = obj.get(key10).toRight(new MappingException("field " + key10 + " not found")).flatMap(A10 readEither _)
val a11 = obj.get(key11).toRight(new MappingException("field " + key11 + " not found")).flatMap(A11 readEither _)
val a12 = obj.get(key12).toRight(new MappingException("field " + key12 + " not found")).flatMap(A12 readEither _)
val a13 = obj.get(key13).toRight(new MappingException("field " + key13 + " not found")).flatMap(A13 readEither _)
val a14 = obj.get(key14).toRight(new MappingException("field " + key14 + " not found")).flatMap(A14 readEither _)
val a15 = obj.get(key15).toRight(new MappingException("field " + key15 + " not found")).flatMap(A15 readEither _)
val a16 = obj.get(key16).toRight(new MappingException("field " + key16 + " not found")).flatMap(A16 readEither _)
val a17 = obj.get(key17).toRight(new MappingException("field " + key17 + " not found")).flatMap(A17 readEither _)
val a18 = obj.get(key18).toRight(new MappingException("field " + key18 + " not found")).flatMap(A18 readEither _)
val a19 = obj.get(key19).toRight(new MappingException("field " + key19 + " not found")).flatMap(A19 readEither _)
val a20 = obj.get(key20).toRight(new MappingException("field " + key20 + " not found")).flatMap(A20 readEither _)
val a21 = obj.get(key21).toRight(new MappingException("field " + key21 + " not found")).flatMap(A21 readEither _)
val a22 = obj.get(key22).toRight(new MappingException("field " + key22 + " not found")).flatMap(A22 readEither _)
var lefts = List.empty[MappingException]
a1 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a2 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a3 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a4 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a5 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a6 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a7 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a8 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a9 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a10 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a11 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a12 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a13 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a14 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a15 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a16 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a17 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a18 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a19 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a20 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a21 match {
case Left(l) =>
lefts ::= l
case _ =>
}
a22 match {
case Left(l) =>
lefts ::= l
case _ =>
}
if (lefts.isEmpty) {
Right(f(a1.asInstanceOf[Right[_, A1]].value, a2.asInstanceOf[Right[_, A2]].value, a3.asInstanceOf[Right[_, A3]].value, a4.asInstanceOf[Right[_, A4]].value, a5.asInstanceOf[Right[_, A5]].value, a6.asInstanceOf[Right[_, A6]].value, a7.asInstanceOf[Right[_, A7]].value, a8.asInstanceOf[Right[_, A8]].value, a9.asInstanceOf[Right[_, A9]].value, a10.asInstanceOf[Right[_, A10]].value, a11.asInstanceOf[Right[_, A11]].value, a12.asInstanceOf[Right[_, A12]].value, a13.asInstanceOf[Right[_, A13]].value, a14.asInstanceOf[Right[_, A14]].value, a15.asInstanceOf[Right[_, A15]].value, a16.asInstanceOf[Right[_, A16]].value, a17.asInstanceOf[Right[_, A17]].value, a18.asInstanceOf[Right[_, A18]].value, a19.asInstanceOf[Right[_, A19]].value, a20.asInstanceOf[Right[_, A20]].value, a21.asInstanceOf[Right[_, A21]].value, a22.asInstanceOf[Right[_, A22]].value))
} else {
Left(new MappingException.Multi(lefts.reverse, null))
}
case x =>
Left(new MappingException("JObject expected, but got " + x))
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy